Introduction to Test-Driven Development (TDD): A Comprehensive Guide
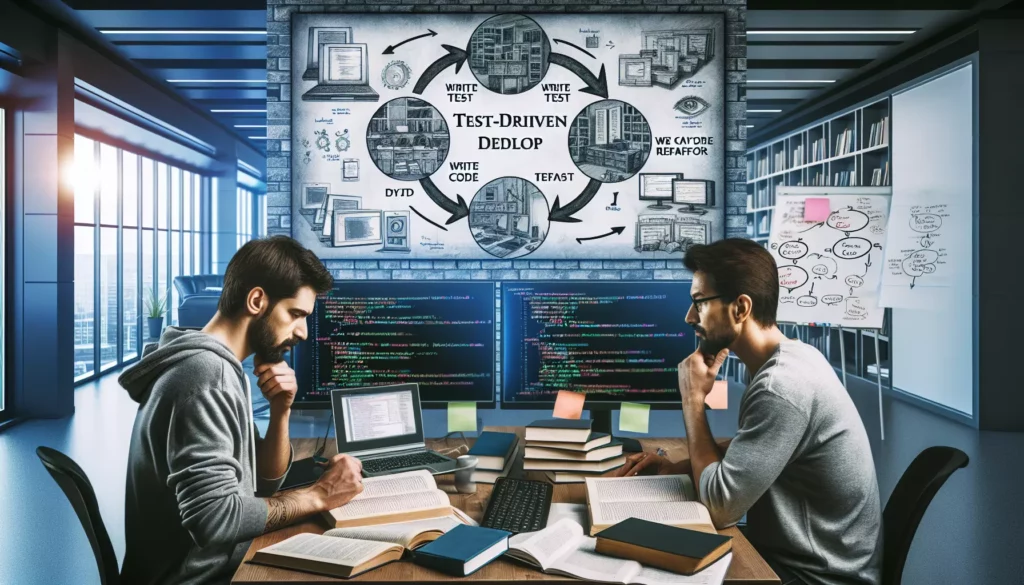
In the world of software development, writing clean, efficient, and bug-free code is paramount. One methodology that has gained significant traction in recent years is Test-Driven Development (TDD). This approach not only helps developers create more robust code but also encourages better design practices and increases overall software quality. In this comprehensive guide, we’ll dive deep into the world of TDD, exploring its principles, benefits, and how to implement it in your development workflow.
What is Test-Driven Development?
Test-Driven Development is a software development process that relies on the repetition of a very short development cycle. It follows these simple steps:
- Write a test for the next bit of functionality you want to add.
- Write the functional code until the test passes.
- Refactor both new and old code to make it well-structured.
The idea is to write tests before writing the actual code. This approach might seem counterintuitive at first, but it offers numerous benefits that we’ll explore in this article.
The TDD Cycle: Red, Green, Refactor
The TDD cycle is often described as “Red, Green, Refactor”. Let’s break down what each of these steps means:
1. Red: Write a Failing Test
In this phase, you write a test that defines a function or improvements of a function, which should fail initially because the function isn’t implemented yet. This step helps you think through the design of the code and how it should work.
2. Green: Make the Test Pass
Here, you write the minimal amount of code necessary to make the test pass. The focus is on getting the functionality working, not on writing perfect code.
3. Refactor: Improve the Code
Once the test passes, you can refactor your code. This means cleaning it up, removing duplication, and making sure it follows good coding practices. The key is that you can do this confidently because you have tests that prove your code still works correctly.
Benefits of Test-Driven Development
TDD offers numerous advantages to developers and development teams:
1. Better Program Design and Higher Code Quality
Writing tests first requires you to really consider what you want your code to do. It forces you to name classes, functions, and methods properly and to think about inputs and outputs. This leads to better-designed, cleaner code.
2. Detailed Project Documentation
The tests you write serve as documentation for your code. They describe how the code should work, making it easier for other developers (or yourself in the future) to understand the codebase.
3. Easier Maintenance and Refactoring
With a comprehensive test suite, you can refactor your code with confidence. If you break something, the tests will let you know immediately.
4. Faster Debugging
When a test fails, you know exactly where in your code the problem is. This significantly reduces debugging time.
5. Reduces Time Spent on Rework
By catching bugs early in development, TDD helps reduce the time spent on rework and bug fixes later in the project lifecycle.
Implementing TDD: A Step-by-Step Guide
Now that we understand the principles and benefits of TDD, let’s walk through a practical example of how to implement it. We’ll use Python and the built-in unittest
module for this example.
Step 1: Write a Failing Test
Let’s say we want to create a simple function that adds two numbers. First, we’ll write a test for this function:
import unittest
class TestMathFunctions(unittest.TestCase):
def test_add_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
self.assertEqual(add_numbers(-1, 1), 0)
self.assertEqual(add_numbers(0, 0), 0)
if __name__ == '__main__':
unittest.main()
If we run this test now, it will fail because we haven’t implemented the add_numbers
function yet.
Step 2: Write the Minimal Code to Pass the Test
Now, let’s implement the add_numbers
function:
def add_numbers(a, b):
return a + b
If we run our test again, it should now pass.
Step 3: Refactor (if necessary)
In this simple example, there’s not much to refactor. However, in more complex scenarios, this is where you would clean up your code, remove any duplication, and ensure it follows best practices.
Step 4: Repeat
Now that we have a working function and passing tests, we can add more functionality. For example, we might want to ensure our function can handle floating-point numbers:
def test_add_float_numbers(self):
self.assertAlmostEqual(add_numbers(2.5, 3.7), 6.2)
self.assertAlmostEqual(add_numbers(-1.1, 1.1), 0)
We would then run the tests, implement the necessary changes, and refactor as needed.
Common TDD Patterns and Best Practices
As you become more comfortable with TDD, you’ll start to recognize common patterns and best practices:
1. Keep Tests Small and Focused
Each test should focus on a single behavior or aspect of your code. This makes tests easier to write, understand, and maintain.
2. Use Descriptive Test Names
Your test names should clearly describe what they’re testing. For example, test_add_positive_numbers
is more descriptive than test_add
.
3. Follow the AAA Pattern
Arrange: Set up the test conditions.
Act: Perform the action being tested.
Assert: Verify the results.
4. Don’t Test Private Methods Directly
Focus on testing the public interface of your classes. Private methods should be tested indirectly through public methods.
5. Use Test Doubles
Learn to use mocks, stubs, and fakes to isolate the code you’re testing and to simulate various scenarios.
TDD in Different Programming Languages
While we used Python in our example, TDD can be applied to any programming language. Here’s a quick overview of TDD frameworks in other popular languages:
JavaScript
For JavaScript, popular testing frameworks include Jest, Mocha, and Jasmine. Here’s a simple example using Jest:
// add.js
function add(a, b) {
return a + b;
}
module.exports = add;
// add.test.js
const add = require('./add');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
Java
JUnit is the most widely used testing framework for Java. Here’s a basic example:
import org.junit.Test;
import static org.junit.Assert.*;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
C#
For C#, NUnit is a popular choice. Here’s how you might write a test:
using NUnit.Framework;
[TestFixture]
public class CalculatorTests
{
[Test]
public void Add_SimpleValues_Calculated()
{
Calculator calculator = new Calculator();
Assert.AreEqual(5, calculator.Add(2, 3));
}
}
TDD and Agile Development
Test-Driven Development aligns well with Agile development methodologies. Both emphasize iterative development, continuous improvement, and delivering working software. Here’s how TDD supports Agile principles:
1. Continuous Integration
TDD provides a suite of tests that can be run automatically whenever code is integrated, supporting continuous integration practices.
2. Rapid Feedback
The quick cycle of writing a test, implementing code, and running tests provides rapid feedback, which is crucial in Agile development.
3. Embracing Change
With a comprehensive test suite, teams can more confidently make changes and refactor code, which supports the Agile principle of embracing change.
4. Sustainable Development
By focusing on writing clean, well-tested code from the start, TDD supports the Agile aim of maintaining a constant pace indefinitely.
Challenges and Criticisms of TDD
While TDD offers many benefits, it’s not without its challenges and criticisms:
1. Learning Curve
TDD requires a shift in mindset and can be challenging for developers who are used to writing code first and tests later (if at all).
2. Time Investment
Writing tests before code can slow down initial development. However, proponents argue that this time is made up later through reduced debugging and maintenance time.
3. Overemphasis on Unit Tests
Some critics argue that TDD places too much emphasis on unit tests at the expense of integration and system tests.
4. Difficulty with Legacy Code
Applying TDD to existing codebases can be challenging, especially if the code wasn’t designed with testability in mind.
5. Maintaining Test Suites
As projects grow, maintaining a large suite of tests can become time-consuming and complex.
TDD and Code Coverage
Code coverage is a metric that measures how much of your code is executed when your tests run. While TDD often leads to high code coverage, it’s important to remember that high coverage doesn’t necessarily mean high-quality tests or bug-free code.
Here are some points to consider about TDD and code coverage:
1. Coverage as a Byproduct
In TDD, high code coverage is a natural byproduct of the process, not the goal itself.
2. Quality Over Quantity
It’s more important to have meaningful tests that verify behavior than to aim for 100% coverage.
3. Uncovering Untested Scenarios
Code coverage tools can help identify parts of your code that aren’t being tested, which can guide further test writing.
4. Balancing Act
While aiming for high coverage is good, it’s important to balance the effort of writing tests with the value they provide.
Advanced TDD Concepts
As you become more proficient with TDD, you might want to explore some advanced concepts:
1. Behavior-Driven Development (BDD)
BDD is an extension of TDD that focuses on the behavior of the system from the user’s perspective. It often uses a specific language (like Gherkin) to describe tests in a way that non-technical stakeholders can understand.
2. Property-Based Testing
Instead of writing individual test cases, property-based testing involves defining properties that your code should satisfy and then automatically generating test cases to verify these properties.
3. Mutation Testing
Mutation testing involves intentionally changing small parts of your code to see if your tests catch these “mutations”. It’s a way to test the quality of your tests.
4. Test-Driven Development in Microservices
Applying TDD in a microservices architecture presents unique challenges and opportunities, particularly around testing service interactions and maintaining independence between services.
Conclusion
Test-Driven Development is a powerful methodology that can significantly improve the quality of your code and the efficiency of your development process. While it requires a shift in mindset and some upfront investment, the long-term benefits in terms of code quality, maintainability, and developer confidence are substantial.
Remember, TDD is not a silver bullet, and like any methodology, it should be applied judiciously. The key is to understand its principles, start small, and gradually incorporate it into your development workflow. With practice, you’ll find that writing tests becomes second nature, and you’ll wonder how you ever developed without it.
As you continue your journey in software development, consider how TDD might fit into your process. Whether you’re working on a personal project or as part of a large team, the principles of Test-Driven Development can help you write better, more reliable code. Happy coding!