Introduction to Self-Balancing Binary Search Trees
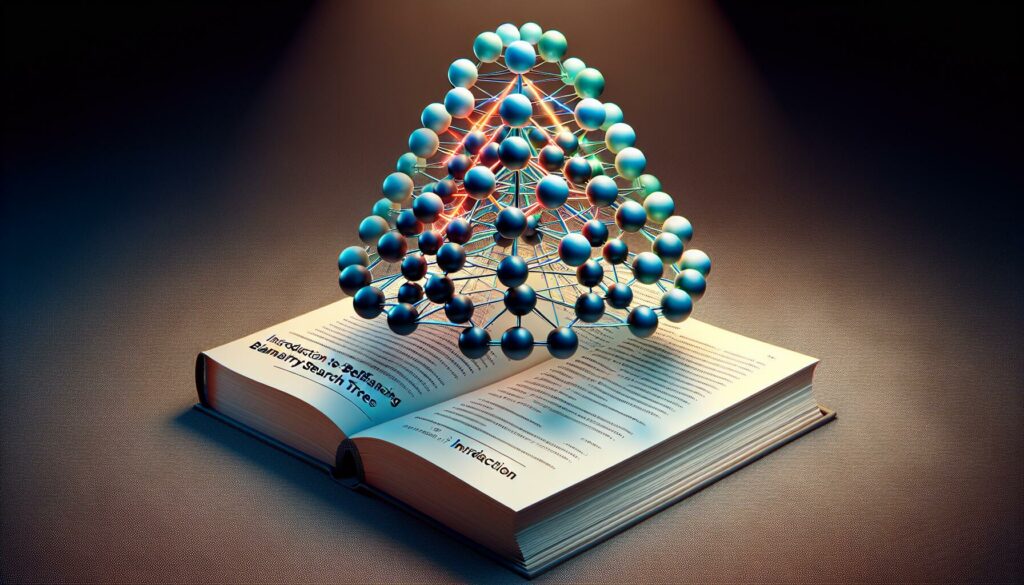
In the world of data structures and algorithms, binary search trees (BSTs) play a crucial role in efficient data storage and retrieval. However, standard BSTs can become unbalanced, leading to degraded performance. This is where self-balancing binary search trees come into play. In this comprehensive guide, we’ll explore the concept of self-balancing BSTs, their importance, and some popular implementations.
Table of Contents
- What are Binary Search Trees?
- The Need for Balancing
- Self-Balancing Binary Search Trees
- AVL Trees
- Red-Black Trees
- Splay Trees
- B-Trees
- Comparison of Self-Balancing BSTs
- Implementing a Self-Balancing BST
- Real-world Applications
- Conclusion
1. What are Binary Search Trees?
Before diving into self-balancing BSTs, let’s quickly recap what a binary search tree is. A binary search tree is a hierarchical data structure where each node has at most two children, referred to as the left child and the right child. The key property of a BST is that for any given node:
- All nodes in its left subtree have keys less than the node’s key
- All nodes in its right subtree have keys greater than the node’s key
This property allows for efficient searching, insertion, and deletion operations, typically with an average time complexity of O(log n), where n is the number of nodes in the tree.
Here’s a simple implementation of a BST node in Python:
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
2. The Need for Balancing
While BSTs offer efficient operations in many cases, their performance can degrade significantly if the tree becomes unbalanced. An unbalanced tree occurs when the heights of the left and right subtrees differ significantly for many nodes in the tree.
Consider the following scenario:
10
/ \
5 15
/ \
3 20
/ \
1 25
This BST is highly unbalanced, resembling a linked list. In this case, searching for the value 25 would take O(n) time, where n is the number of nodes, instead of the expected O(log n) for a balanced tree.
The worst-case scenario occurs when we insert elements in sorted order, resulting in a tree that’s essentially a linked list:
1
\
2
\
3
\
4
\
5
In such cases, the time complexity for operations degrades to O(n), losing the logarithmic time advantage of BSTs.
3. Self-Balancing Binary Search Trees
Self-balancing binary search trees are a class of BSTs that automatically keep their height (maximal number of levels below the root) small in the face of arbitrary item insertions and deletions. These data structures modify the tree at critical times, ensuring that it remains balanced and maintains its efficiency.
The primary goals of self-balancing BSTs are:
- Maintain balance: Ensure that the tree remains approximately balanced after insertions and deletions
- Preserve BST properties: Maintain the ordering property of BSTs
- Efficient operations: Keep insertion, deletion, and search operations efficient, typically O(log n)
Let’s explore some popular self-balancing BST implementations.
4. AVL Trees
AVL trees, named after their inventors Adelson-Velsky and Landis, were the first self-balancing BST to be invented. In an AVL tree, the heights of the left and right subtrees of any node differ by at most one.
Key properties of AVL trees:
- Balance Factor: For each node, the balance factor (height of left subtree – height of right subtree) must be -1, 0, or 1
- Rebalancing: When this balance is violated, the tree is rebalanced using rotation operations
- Height: The height of an AVL tree is always O(log n)
AVL trees use four types of rotations to maintain balance:
- Left rotation
- Right rotation
- Left-Right rotation
- Right-Left rotation
Here’s a basic implementation of an AVL tree node in Python:
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1 # Height of the node
def get_height(node):
if not node:
return 0
return node.height
def get_balance(node):
if not node:
return 0
return get_height(node.left) - get_height(node.right)
def update_height(node):
node.height = 1 + max(get_height(node.left), get_height(node.right))
AVL trees are particularly useful when frequent lookups are required, as they provide faster retrievals than other self-balancing BSTs.
5. Red-Black Trees
Red-Black trees are another type of self-balancing BST that uses color properties to maintain balance. Each node in a Red-Black tree has an extra bit representing color, either red or black.
Key properties of Red-Black trees:
- Root Property: The root is always black
- External Property: Every leaf (NIL) is black
- Internal Property: If a node is red, then both its children are black
- Depth Property: For each node, any simple path from this node to any of its descendant leaves contains the same number of black nodes
These properties ensure that the tree remains approximately balanced. The height of a Red-Black tree is always O(log n), where n is the number of nodes in the tree.
Here’s a basic implementation of a Red-Black tree node in Python:
class RBNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.parent = None
self.color = 1 # 1 for red, 0 for black
class RedBlackTree:
def __init__(self):
self.TNULL = RBNode(0)
self.TNULL.color = 0
self.TNULL.left = None
self.TNULL.right = None
self.root = self.TNULL
Red-Black trees are widely used in many software libraries. For example, they are used in the implementation of associative arrays in many programming languages, including C++’s std::map and Java’s TreeMap.
6. Splay Trees
Splay trees are a unique type of self-balancing BST that doesn’t explicitly maintain balance. Instead, they use a “splaying” operation that moves a given element to the root of the tree.
Key properties of Splay trees:
- Splaying: After each access, the accessed element is moved to the root using a series of rotations
- Amortized Performance: While individual operations can take O(n) time, the amortized time for any sequence of m operations is O(m log n)
- Adaptivity: Frequently accessed elements stay near the root, providing faster access
Here’s a basic implementation of a Splay tree node in Python:
class SplayNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
def splay(root, key):
if not root or root.key == key:
return root
if root.key > key:
if not root.left:
return root
if root.left.key > key:
root.left.left = splay(root.left.left, key)
root = right_rotate(root)
elif root.left.key < key:
root.left.right = splay(root.left.right, key)
if root.left.right:
root.left = left_rotate(root.left)
return right_rotate(root) if root.left else root
else:
# Similar logic for right subtree
pass
Splay trees are particularly useful in applications where certain elements are accessed more frequently than others, such as caching systems or in the implementation of memory allocators.
7. B-Trees
While not strictly a binary tree, B-trees are an important self-balancing search tree used primarily in database and file systems. B-trees generalize the concept of BSTs to allow nodes with more than two children.
Key properties of B-trees:
- Order: A B-tree of order m has a maximum of m children per node
- Node Properties: Each internal node (except root) has at least ⌈m/2⌉ children
- Leaf Level: All leaves appear on the same level
- Height: The height of a B-tree is O(log n)
Here’s a basic implementation of a B-tree node in Python:
class BTreeNode:
def __init__(self, leaf=False):
self.leaf = leaf
self.keys = []
self.child = []
class BTree:
def __init__(self, t):
self.root = BTreeNode(True)
self.t = t # Minimum degree
def insert(self, k):
root = self.root
if len(root.keys) == (2 * self.t) - 1:
temp = BTreeNode()
self.root = temp
temp.child.insert(0, root)
self.split_child(temp, 0)
self.insert_non_full(temp, k)
else:
self.insert_non_full(root, k)
B-trees are extensively used in databases and file systems due to their ability to minimize disk I/O operations.
8. Comparison of Self-Balancing BSTs
Each type of self-balancing BST has its strengths and use cases. Here’s a brief comparison:
Tree Type | Insertion | Deletion | Search | Space | Balancing Method |
---|---|---|---|---|---|
AVL Trees | O(log n) | O(log n) | O(log n) | O(n) | Strict balancing |
Red-Black Trees | O(log n) | O(log n) | O(log n) | O(n) | Relaxed balancing |
Splay Trees | O(log n) amortized | O(log n) amortized | O(log n) amortized | O(n) | Splaying |
B-Trees | O(log n) | O(log n) | O(log n) | O(n) | Multi-way nodes |
- AVL Trees: Best for lookup-intensive applications
- Red-Black Trees: Good balance between lookup and insertion/deletion performance
- Splay Trees: Excellent for applications with locality of reference
- B-Trees: Ideal for systems that read and write large blocks of data
9. Implementing a Self-Balancing BST
Let’s implement a simple AVL tree in Python to demonstrate the concepts of self-balancing BSTs:
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def __init__(self):
self.root = None
def height(self, node):
if not node:
return 0
return node.height
def balance(self, node):
if not node:
return 0
return self.height(node.left) - self.height(node.right)
def update_height(self, node):
if not node:
return
node.height = 1 + max(self.height(node.left), self.height(node.right))
def right_rotate(self, y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
self.update_height(y)
self.update_height(x)
return x
def left_rotate(self, x):
y = x.right
T2 = y.left
y.left = x
x.right = T2
self.update_height(x)
self.update_height(y)
return y
def insert(self, root, key):
if not root:
return AVLNode(key)
if key < root.key:
root.left = self.insert(root.left, key)
else:
root.right = self.insert(root.right, key)
self.update_height(root)
balance = self.balance(root)
# Left Left Case
if balance > 1 and key < root.left.key:
return self.right_rotate(root)
# Right Right Case
if balance < -1 and key > root.right.key:
return self.left_rotate(root)
# Left Right Case
if balance > 1 and key > root.left.key:
root.left = self.left_rotate(root.left)
return self.right_rotate(root)
# Right Left Case
if balance < -1 and key < root.right.key:
root.right = self.right_rotate(root.right)
return self.left_rotate(root)
return root
def insert_key(self, key):
self.root = self.insert(self.root, key)
def inorder_traversal(self, root):
if not root:
return
self.inorder_traversal(root.left)
print(root.key, end=' ')
self.inorder_traversal(root.right)
# Usage
avl = AVLTree()
keys = [10, 20, 30, 40, 50, 25]
for key in keys:
avl.insert_key(key)
print("Inorder traversal of the AVL tree:")
avl.inorder_traversal(avl.root)
This implementation demonstrates the key concepts of AVL trees, including height maintenance, balance checking, and rotation operations to maintain balance.
10. Real-world Applications
Self-balancing BSTs find applications in various areas of computer science and software development:
- Database Systems: B-trees are extensively used in database indexing to speed up data retrieval.
- File Systems: Many file systems use B-trees or variants for directory structures.
- Memory Allocation: Splay trees are used in memory allocators for efficient memory management.
- Compiler Design: Symbol tables in compilers often use self-balancing BSTs for fast lookups.
- Network Routing Tables: Red-Black trees are used in some implementations of IP routing tables.
- Game Development: Scene graphs in 3D games often use self-balancing BSTs for spatial partitioning.
- Machine Learning: Decision trees, which are fundamental in many machine learning algorithms, can benefit from balancing techniques.
11. Conclusion
Self-balancing binary search trees are powerful data structures that maintain efficiency even under dynamic operations. They offer a perfect balance between fast searches and efficient insertions and deletions, making them invaluable in many applications.
Key takeaways:
- Self-balancing BSTs automatically adjust their structure to maintain balance.
- Different types of self-balancing BSTs (AVL, Red-Black, Splay, B-trees) offer various trade-offs between strict balance and operation efficiency.
- These data structures guarantee logarithmic time complexity for basic operations in the worst case (or amortized case for Splay trees).
- Understanding these structures is crucial for designing efficient algorithms and systems, especially in areas like databases, file systems, and memory management.
As you continue your journey in computer science and software development, mastering self-balancing BSTs will provide you with powerful tools to solve complex problems efficiently. Remember, the choice of which self-balancing BST to use depends on the specific requirements of your application, such as the frequency of different operations and the nature of the data being stored.
Keep practicing implementing these data structures, and don’t hesitate to use them in your projects. They might just be the key to unlocking significant performance improvements in your applications!