Introduction to Rust: Should You Learn It in 2024?
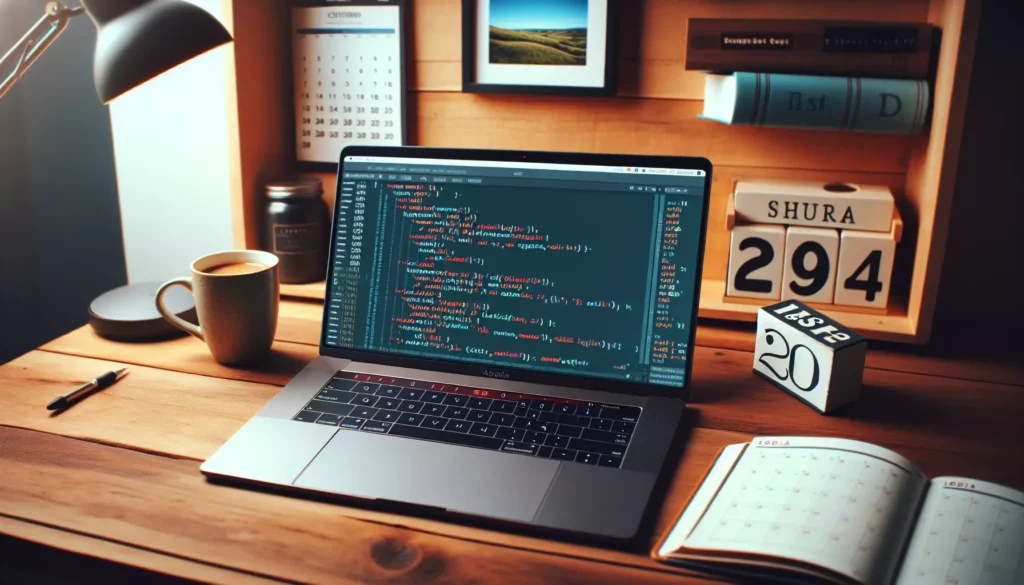
As we step into 2024, the programming landscape continues to evolve, and one language that has been gaining significant traction is Rust. Whether you’re a seasoned developer looking to expand your skill set or a newcomer to the world of programming, you might be wondering if Rust is worth your time and effort. In this comprehensive guide, we’ll explore Rust’s features, its growing ecosystem, and why it might be the perfect language to learn in 2024.
What is Rust?
Rust is a systems programming language that focuses on safety, concurrency, and performance. Created by Mozilla Research, Rust was first released in 2010 and has since grown to become one of the most loved programming languages among developers, according to Stack Overflow’s annual Developer Survey for multiple years running.
Key features of Rust include:
- Memory safety without garbage collection
- Concurrency without data races
- Zero-cost abstractions
- Pattern matching
- Type inference
- Guaranteed thread safety
- Trait-based generics
- Efficient C bindings
Why Rust is Gaining Popularity
Rust’s popularity has been on a steady rise, and there are several reasons for this trend:
1. Safety and Reliability
Rust’s primary selling point is its focus on safety. The language’s ownership model and borrow checker ensure memory safety and thread safety at compile-time, eliminating entire classes of bugs that plague other systems programming languages like C and C++.
2. Performance
Rust offers performance comparable to C and C++, making it an excellent choice for systems programming, game development, and other performance-critical applications.
3. Modern Language Features
Rust combines low-level control with high-level abstractions, offering features like pattern matching, closures, and iterators that are typically associated with higher-level languages.
4. Growing Ecosystem
The Rust ecosystem is rapidly expanding, with a rich set of libraries (crates) available through the Cargo package manager. This makes it easier for developers to build complex applications without reinventing the wheel.
5. Industry Adoption
Major tech companies like Microsoft, Google, Amazon, and Facebook are increasingly adopting Rust for critical infrastructure and systems programming tasks.
Rust in Action: A Simple Example
Let’s take a look at a simple Rust program to get a feel for the language’s syntax:
fn main() {
// Declare a mutable variable
let mut x = 5;
println!("The value of x is: {}", x);
// Modify the variable
x = 6;
println!("The value of x is now: {}", x);
// Use a for loop to iterate over a range
for i in 1..5 {
println!("{}", i);
}
// Define and call a function
fn add(a: i32, b: i32) -> i32 {
a + b
}
let sum = add(3, 7);
println!("The sum is: {}", sum);
}
This simple program demonstrates variable declaration, mutation, loops, and function definitions in Rust. As you can see, the syntax is clean and expressive, with some similarities to other popular languages.
Key Concepts in Rust
To truly appreciate Rust’s power and understand why it’s worth learning in 2024, let’s delve into some of its key concepts:
1. Ownership and Borrowing
Rust’s ownership system is one of its most distinctive features. It ensures memory safety without the need for a garbage collector. Here’s a brief overview:
- Each value in Rust has an owner.
- There can only be one owner at a time.
- When the owner goes out of scope, the value is dropped.
Borrowing allows you to reference data without taking ownership. This concept is crucial for writing efficient and safe code in Rust.
2. Lifetimes
Lifetimes are Rust’s way of ensuring that references are valid for the duration they’re used. While the borrow checker often infers lifetimes, explicit lifetime annotations are sometimes necessary, especially in complex scenarios.
3. Traits
Traits in Rust are similar to interfaces in other languages. They define shared behavior across types, enabling powerful abstractions and generic programming.
4. Error Handling
Rust encourages explicit error handling through the Result
and Option
types, promoting robust and reliable code.
Rust’s Ecosystem and Tools
One of the reasons to consider learning Rust in 2024 is its maturing ecosystem and excellent tooling:
1. Cargo
Cargo is Rust’s package manager and build system. It handles dependencies, compiles your code, runs tests, and generates documentation. Its ease of use significantly improves the development experience.
2. Rustfmt
Rustfmt is an automatic code formatter that ensures consistent style across Rust projects, reducing the cognitive load of maintaining code style manually.
3. Clippy
Clippy is a collection of lints to catch common mistakes and improve your Rust code. It’s an invaluable tool for learning Rust best practices and writing idiomatic code.
4. Rust Analyzer
Rust Analyzer is a powerful language server that provides IDE-like features for Rust in various text editors and IDEs, enhancing the development experience with features like code completion, go-to-definition, and inline error messages.
Where is Rust Being Used?
Understanding where Rust is being applied can help you gauge its relevance and potential career opportunities:
1. Systems Programming
Rust is increasingly being used for operating systems, file systems, and other low-level system components. For example, Microsoft is exploring Rust for parts of Windows, and Google is using it in the Android operating system.
2. Web Development
While not as common as JavaScript or Python, Rust is gaining traction in web development, particularly for backend services and WebAssembly applications.
3. Game Development
Rust’s performance characteristics make it an attractive option for game development, especially for game engines and performance-critical components.
4. Blockchain and Cryptocurrencies
Several blockchain projects, including Solana and Polkadot, use Rust for its safety and performance benefits.
5. Embedded Systems
Rust’s ability to run without a runtime or garbage collector makes it suitable for embedded systems and IoT devices.
Learning Rust: Challenges and Resources
While Rust offers numerous benefits, it does come with a learning curve, especially for developers accustomed to garbage-collected languages or those new to systems programming concepts.
Challenges:
- Understanding ownership and borrowing
- Dealing with lifetimes
- Adapting to Rust’s strict compiler
- Learning to think in terms of Rust’s safety guarantees
Resources for Learning Rust:
- The Rust Programming Language Book: Often referred to as “The Book,” this free online resource is the official guide to learning Rust.
- Rust by Example: An online collection of runnable examples that illustrate various Rust concepts and standard libraries.
- Rustlings: A project of small exercises to get you used to reading and writing Rust code.
- Exercism’s Rust Track: A series of coding exercises with mentorship to help you improve your Rust skills.
- This Week in Rust: A weekly newsletter keeping you updated with the latest Rust news, articles, and projects.
The Future of Rust
As we look towards 2024 and beyond, Rust’s future appears bright:
- Growing Adoption: More companies are adopting Rust for critical infrastructure and systems programming tasks.
- Expanding Ecosystem: The number and quality of Rust libraries and tools continue to grow, making it easier to build complex applications.
- Improved Learning Resources: As the Rust community grows, so does the availability of learning resources, making it easier for newcomers to pick up the language.
- Potential for Web Development: With improvements in the Rust web ecosystem and growing support for WebAssembly, Rust could become more prominent in web development.
Should You Learn Rust in 2024?
The decision to learn Rust in 2024 depends on your goals and circumstances. Here are some scenarios where learning Rust could be particularly beneficial:
- Systems Programmers: If you’re interested in low-level programming, operating systems, or embedded systems, Rust offers a modern and safe alternative to C and C++.
- Performance-Critical Applications: For developers working on applications where performance is crucial, Rust’s speed and low overhead make it an excellent choice.
- Safety-Critical Systems: In fields where software failures can have severe consequences, Rust’s focus on safety is a significant advantage.
- Expanding Your Programming Horizons: Even if you don’t plan to use Rust professionally, learning it can improve your understanding of concepts like ownership, borrowing, and low-level memory management.
- Preparing for Future Trends: As Rust continues to gain adoption in industry, having Rust skills could open up new career opportunities.
Conclusion
Rust represents a significant step forward in systems programming languages, offering a unique combination of performance, safety, and modern language features. As we move into 2024, Rust’s growing ecosystem, increasing industry adoption, and potential for expanding into new domains make it an attractive language to learn.
While Rust does come with a learning curve, particularly for those new to systems programming, the investment in learning Rust can pay off in terms of writing more reliable, efficient, and safe code. The robust tooling and supportive community around Rust also make the learning process more manageable and enjoyable.
Ultimately, whether you should learn Rust in 2024 depends on your personal goals and interests. If you’re intrigued by low-level programming, passionate about writing safe and efficient code, or simply looking to add a powerful tool to your programming toolkit, Rust could be an excellent choice. As with any programming language, the key is to align your learning with your objectives and to enjoy the process of discovery and mastery.
Remember, in the ever-evolving world of technology, continuous learning is crucial. Whether you choose to dive into Rust or explore other languages and technologies, staying curious and open to new ideas will serve you well in your programming journey. Happy coding!