Introduction to Ruby on Rails for Aspiring Web Developers
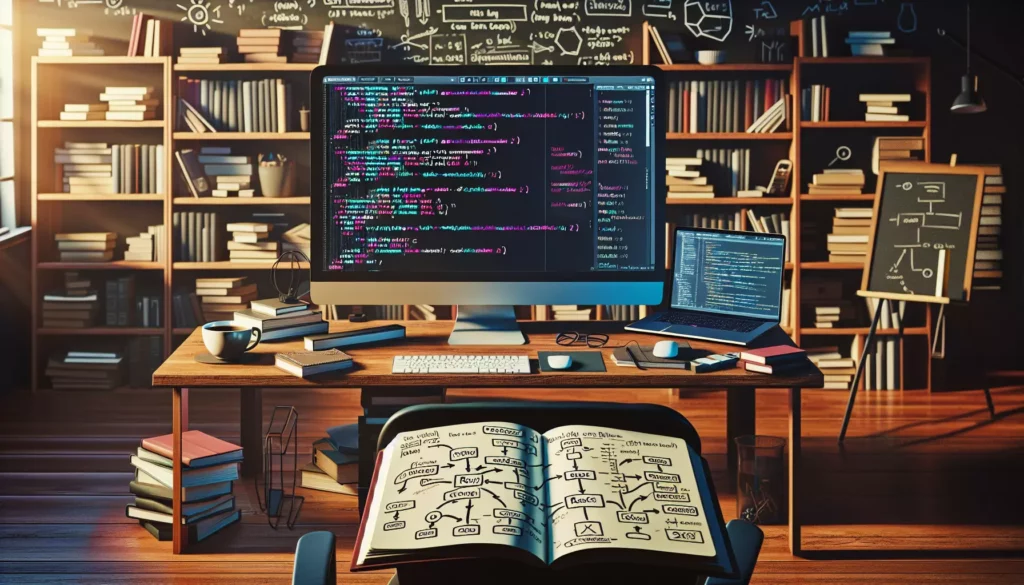
Welcome to the world of Ruby on Rails! If you’re an aspiring web developer looking to dive into a powerful and efficient framework for building web applications, you’ve come to the right place. In this comprehensive guide, we’ll explore the fundamentals of Ruby on Rails, its key features, and why it’s still a popular choice among developers in 2023. Whether you’re a complete beginner or have some programming experience, this article will provide you with a solid foundation to start your journey with Rails.
What is Ruby on Rails?
Ruby on Rails, often simply called Rails, is an open-source web application framework written in Ruby. It was created by David Heinemeier Hansson and released in 2004. Rails follows the model-view-controller (MVC) architectural pattern and emphasizes the use of well-known software engineering patterns and principles, such as convention over configuration (CoC), don’t repeat yourself (DRY), and the active record pattern.
The framework is designed to make programming web applications easier by making assumptions about what every developer needs to get started. It allows you to write less code while accomplishing more than many other languages and frameworks. Rails is used by companies of all sizes, from startups to large enterprises, including Airbnb, GitHub, Shopify, and Twitch.
Key Features of Ruby on Rails
Let’s explore some of the key features that make Ruby on Rails a popular choice for web development:
1. Convention over Configuration (CoC)
Rails follows the principle of “Convention over Configuration,” which means it makes assumptions about what you want to do and how you’re going to do it, rather than requiring you to specify every little thing through endless configuration files. This approach significantly reduces the amount of code you need to write without losing flexibility.
2. Don’t Repeat Yourself (DRY)
The DRY principle is a core part of Rails philosophy. It encourages developers to reduce repetition of information of all kinds, particularly in a multi-tiered web application. By adhering to this principle, your code becomes more maintainable, extensible, and less buggy.
3. Active Record
Rails uses the Active Record pattern, which is an approach to accessing data in a database. It’s the M in MVC – the model – which is the layer of the system responsible for representing business data and logic.
4. RESTful Application Design
Rails encourages the use of RESTful (Representational State Transfer) web service. This is an architectural style for designing networked applications, making it easier to build APIs and web services.
5. Built-in Testing
Rails has built-in support for automated testing. It creates a test directory for you as soon as you create a Rails project, encouraging test-driven development.
6. Security Features
Rails comes with several security features out of the box, such as protection against SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), and more.
Setting Up Your Development Environment
Before we dive into coding, let’s set up your development environment. Here’s a step-by-step guide to get you started:
Step 1: Install Ruby
First, you need to install Ruby on your system. The process varies depending on your operating system:
- For macOS: macOS comes with Ruby pre-installed, but it’s often an older version. It’s recommended to use a version manager like RVM or rbenv to install and manage Ruby versions.
- For Windows: Download and install Ruby from the official Ruby website or use a tool like RubyInstaller.
- For Linux: Use your distribution’s package manager to install Ruby, or use a version manager like RVM or rbenv.
Step 2: Install Rails
Once Ruby is installed, you can install Rails using the following command in your terminal:
gem install rails
Step 3: Install a Database
Rails works with various databases, but SQLite is the default and easiest to set up. It comes pre-installed on macOS and many Linux distributions. For Windows, you might need to install it separately.
Step 4: Install a Text Editor or IDE
You’ll need a good text editor or Integrated Development Environment (IDE) to write your code. Some popular choices include:
- Visual Studio Code
- RubyMine
- Sublime Text
- Atom
Creating Your First Rails Application
Now that your environment is set up, let’s create your first Rails application:
Step 1: Generate a New Rails Application
Open your terminal and run the following command:
rails new my_first_app
This command creates a new Rails application in a directory called “my_first_app”.
Step 2: Navigate to Your Application Directory
cd my_first_app
Step 3: Start the Rails Server
rails server
This command starts your Rails application. You can now visit http://localhost:3000 in your web browser to see your application running.
Understanding the Rails Directory Structure
When you create a new Rails application, it generates a standard directory structure. Understanding this structure is crucial for developing with Rails. Here’s an overview of the main directories:
- app/: Contains the core of your application, including models, views, controllers, and helpers.
- config/: Configuration files for your application, including database configuration and routing.
- db/: Database files and migrations.
- lib/: Extended modules for your application.
- log/: Application log files.
- public/: The only folder seen by the world as-is. Contains static files and compiled assets.
- test/: Unit tests, fixtures, and other test apparatus.
- vendor/: Place for all third-party code.
The Model-View-Controller (MVC) Architecture
Rails follows the Model-View-Controller (MVC) architectural pattern, which separates the application logic into three interconnected elements. This separation of concerns provides a better division of labor and improved maintenance. Let’s break down each component:
Model
The Model represents the data and business logic of the application. It’s responsible for:
- Maintaining the relationship between objects and the database
- Validating models before they get used
- Performing database operations
Here’s a simple example of a model in Rails:
class User < ApplicationRecord
validates :name, presence: true
validates :email, presence: true, uniqueness: true
end
View
The View is responsible for presenting data to the user. In Rails, views are typically written using ERB (Embedded Ruby) templates. They handle the application’s user interface and presentation logic. For example:
<h1>Welcome, <%= @user.name %>!</h1>
<p>Your email is: <%= @user.email %></p>
Controller
The Controller acts as an intermediary between the Model and the View. It receives user requests, interacts with the Model to fetch or update data, and then renders the appropriate View. Here’s a simple controller example:
class UsersController < ApplicationController
def show
@user = User.find(params[:id])
end
def create
@user = User.new(user_params)
if @user.save
redirect_to @user
else
render 'new'
end
end
private
def user_params
params.require(:user).permit(:name, :email)
end
end
Routing in Rails
Routing in Rails is the mechanism by which URLs are mapped to controller actions. The routes are defined in the config/routes.rb
file. Here’s an example of a simple route:
Rails.application.routes.draw do
get '/users/:id', to: 'users#show'
end
This route maps GET requests to “/users/1” to the show
action in the UsersController
.
Active Record: Object-Relational Mapping in Rails
Active Record is the M in MVC – the model – which is the layer of the system responsible for representing business data and logic. It facilitates the creation and use of business objects whose data requires persistent storage to a database.
Here are some key features of Active Record:
1. Object-Relational Mapping (ORM)
Active Record provides an intuitive way to:
- Represent models and their data
- Represent associations between these models
- Represent inheritance hierarchies through related models
- Validate models before they get persisted to the database
- Perform database operations in an object-oriented fashion
2. Associations
Active Record supports several types of associations between models:
- belongs_to
- has_one
- has_many
- has_many :through
- has_one :through
- has_and_belongs_to_many
For example:
class User < ApplicationRecord
has_many :posts
end
class Post < ApplicationRecord
belongs_to :user
end
3. Validations
Active Record allows you to validate the state of a model before it gets written to the database. For example:
class User < ApplicationRecord
validates :name, presence: true
validates :email, presence: true, uniqueness: true
validates :age, numericality: { greater_than_or_equal_to: 18 }
end
4. Callbacks
Active Record callbacks allow you to attach code to certain events in the life-cycle of your models. For example:
class User < ApplicationRecord
before_save :encrypt_password
private
def encrypt_password
self.password = BCrypt::Password.create(password)
end
end
Views and Asset Pipeline
Views in Rails are responsible for presenting your application’s data and user interface. They are typically written in ERB (Embedded Ruby), which allows you to embed Ruby code within HTML.
Layouts
Layouts in Rails allow you to define a common structure for your pages. The default layout is located at app/views/layouts/application.html.erb
. Here’s a simple example:
<!DOCTYPE html>
<html>
<head>
<title><%= yield(:title) %></title>
<%= csrf_meta_tags %>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
</head>
<body>
<%= yield %>
</body>
</html>
Partials
Partials allow you to extract common view code into separate files to keep your views DRY. Partials are prefixed with an underscore. For example, _form.html.erb
:
<%= form_for @user do |f| %>
<%= f.label :name %>
<%= f.text_field :name %>
<%= f.label :email %>
<%= f.email_field :email %>
<%= f.submit %>
<% end %>
You can render this partial in another view like this:
<h1>New User</h1>
<%= render 'form' %>
Asset Pipeline
The Asset Pipeline is a framework in Rails that concatenates, minifies and compresses JavaScript and CSS assets. It also adds fingerprinting to the filenames so that the file that is downloaded will be cached by the web browser. This can significantly improve your application’s load time.
Testing in Rails
Testing is a crucial part of developing robust and maintainable applications. Rails provides built-in support for testing, making it easy to write and run tests for your application.
Types of Tests
Rails supports several types of tests:
- Unit Tests: For testing models and other Ruby classes.
- Functional Tests: For testing controllers.
- Integration Tests: For testing the interaction between controllers.
- System Tests: For full browser-based tests of your application.
Writing Tests
Here’s an example of a simple model test:
require 'test_helper'
class UserTest < ActiveSupport::TestCase
test "should not save user without email" do
user = User.new(name: "John Doe")
assert_not user.save, "Saved the user without an email"
end
end
And here’s an example of a controller test:
require 'test_helper'
class UsersControllerTest < ActionDispatch::IntegrationTest
test "should get index" do
get users_url
assert_response :success
end
test "should create user" do
assert_difference('User.count') do
post users_url, params: { user: { name: "John Doe", email: "john@example.com" } }
end
assert_redirected_to user_url(User.last)
end
end
Deploying a Rails Application
Once you’ve developed your Rails application, you’ll want to deploy it so others can use it. Here are some popular options for deploying Rails applications:
1. Heroku
Heroku is a cloud platform that lets you deploy, run and manage applications written in Ruby, Node.js, Java, Python, Clojure, Scala, Go and PHP. It’s one of the easiest platforms to deploy Rails applications.
To deploy to Heroku:
- Sign up for a Heroku account
- Install the Heroku CLI
- From your Rails app directory, run:
heroku create git push heroku main heroku run rake db:migrate
2. Amazon Web Services (AWS)
AWS offers several services that can be used to deploy Rails applications, including Elastic Beanstalk, EC2, and ECS. While more complex than Heroku, AWS offers more control and can be more cost-effective for larger applications.
3. Digital Ocean
Digital Ocean provides virtual private servers (they call them “droplets”) where you can deploy your Rails application. This option requires more server administration knowledge but offers more flexibility and can be cost-effective.
Conclusion
Ruby on Rails is a powerful and efficient framework for building web applications. Its emphasis on convention over configuration and the DRY principle makes it an excellent choice for rapid application development. As you’ve seen in this introduction, Rails provides a robust set of tools and conventions that can help you build complex web applications with ease.
Remember, the best way to learn Rails is by doing. Start with small projects, experiment with different features, and gradually build more complex applications. The Rails community is large and supportive, so don’t hesitate to seek help when you need it.
As you continue your journey with Rails, you’ll discover more advanced topics like Action Cable for real-time features, Active Storage for file uploads, and Action Mailer for sending emails. You might also want to explore popular gems (Ruby libraries) that can extend Rails’ functionality.
Keep coding, keep learning, and enjoy your journey with Ruby on Rails!