Introduction to Reinforcement Learning Algorithms
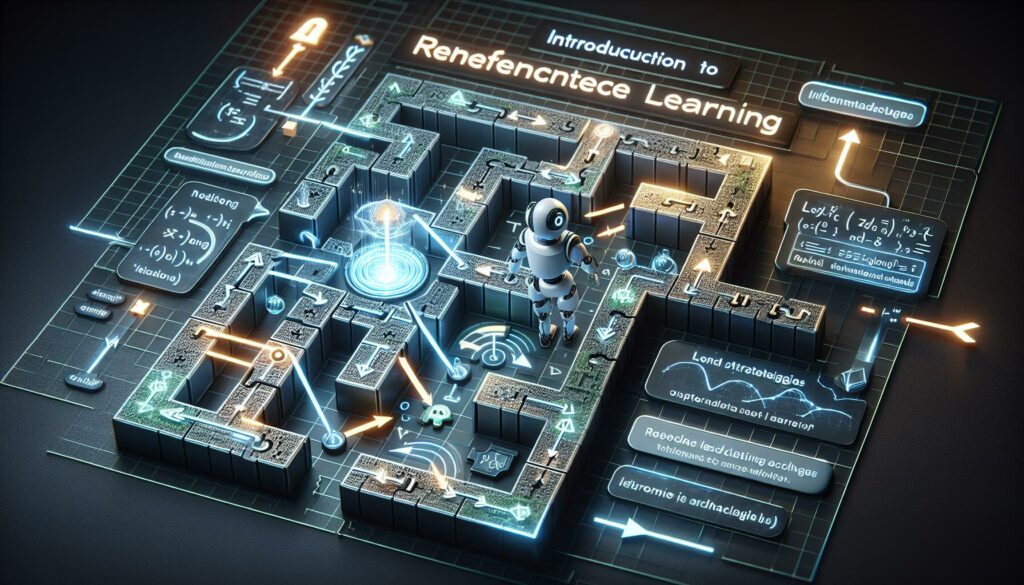
Reinforcement Learning (RL) is a fascinating subset of machine learning that has gained significant traction in recent years. It’s a powerful approach that enables machines to learn through interaction with their environment, much like how humans and animals learn from experience. In this comprehensive guide, we’ll dive deep into the world of reinforcement learning algorithms, exploring their fundamentals, key concepts, and popular implementations.
What is Reinforcement Learning?
Reinforcement Learning is a type of machine learning where an agent learns to make decisions by interacting with an environment. The agent receives feedback in the form of rewards or penalties for its actions, allowing it to learn which actions are most beneficial in different situations. This trial-and-error approach enables the agent to develop strategies to maximize its cumulative reward over time.
The core components of a reinforcement learning system include:
- Agent: The entity that learns and makes decisions
- Environment: The world in which the agent operates
- State: The current situation or condition of the environment
- Action: A decision made by the agent that affects the environment
- Reward: Feedback received by the agent based on its actions
- Policy: The strategy employed by the agent to make decisions
Key Concepts in Reinforcement Learning
Before diving into specific algorithms, it’s essential to understand some fundamental concepts in reinforcement learning:
1. Markov Decision Process (MDP)
The Markov Decision Process is a mathematical framework used to model decision-making in situations where outcomes are partly random and partly under the control of a decision-maker. In RL, the environment is often modeled as an MDP, which consists of:
- A set of states (S)
- A set of actions (A)
- Transition probabilities between states
- Rewards associated with state transitions
- A discount factor (γ) for future rewards
2. Value Function
The value function estimates the expected cumulative reward an agent can obtain from a given state. It helps the agent evaluate the desirability of different states and guides decision-making. There are two types of value functions:
- State-Value Function (V(s)): Estimates the expected return starting from a state s and following a particular policy
- Action-Value Function (Q(s,a)): Estimates the expected return starting from a state s, taking action a, and then following a particular policy
3. Policy
A policy (Ï€) is a strategy or a set of rules that the agent follows to make decisions. It maps states to actions, determining the agent’s behavior in different situations. Policies can be deterministic (always choosing the same action in a given state) or stochastic (choosing actions based on probabilities).
4. Exploration vs. Exploitation
One of the key challenges in reinforcement learning is balancing exploration (trying new actions to gather more information) and exploitation (using known information to maximize rewards). Striking the right balance is crucial for effective learning and optimal performance.
Popular Reinforcement Learning Algorithms
Now that we’ve covered the basics, let’s explore some of the most popular reinforcement learning algorithms:
1. Q-Learning
Q-Learning is a model-free reinforcement learning algorithm that learns the optimal action-value function (Q-function) without requiring a model of the environment. It’s an off-policy algorithm, meaning it can learn from actions not directly resulting from the current policy.
The Q-learning update rule is:
Q(s,a) = Q(s,a) + α * (r + γ * max(Q(s',a')) - Q(s,a))
Where:
- Q(s,a) is the current Q-value for state s and action a
- α is the learning rate
- r is the immediate reward
- γ is the discount factor
- max(Q(s’,a’)) is the maximum Q-value for the next state s’
Here’s a simple implementation of Q-learning in Python:
import numpy as np
def q_learning(env, num_episodes, learning_rate, discount_factor, epsilon):
q_table = np.zeros((env.observation_space.n, env.action_space.n))
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
if np.random.random() < epsilon:
action = env.action_space.sample() # Explore
else:
action = np.argmax(q_table[state]) # Exploit
next_state, reward, done, _ = env.step(action)
# Q-learning update
old_q = q_table[state, action]
next_max_q = np.max(q_table[next_state])
new_q = (1 - learning_rate) * old_q + learning_rate * (reward + discount_factor * next_max_q)
q_table[state, action] = new_q
state = next_state
return q_table
2. SARSA (State-Action-Reward-State-Action)
SARSA is another popular reinforcement learning algorithm that is similar to Q-learning. The main difference is that SARSA is an on-policy algorithm, meaning it learns from actions taken according to the current policy. The update rule for SARSA is:
Q(s,a) = Q(s,a) + α * (r + γ * Q(s',a') - Q(s,a))
Where Q(s’,a’) is the Q-value of the next state-action pair, rather than the maximum Q-value used in Q-learning.
Here’s a Python implementation of SARSA:
import numpy as np
def sarsa(env, num_episodes, learning_rate, discount_factor, epsilon):
q_table = np.zeros((env.observation_space.n, env.action_space.n))
for episode in range(num_episodes):
state = env.reset()
action = epsilon_greedy(q_table, state, epsilon)
done = False
while not done:
next_state, reward, done, _ = env.step(action)
next_action = epsilon_greedy(q_table, next_state, epsilon)
# SARSA update
old_q = q_table[state, action]
next_q = q_table[next_state, next_action]
new_q = (1 - learning_rate) * old_q + learning_rate * (reward + discount_factor * next_q)
q_table[state, action] = new_q
state = next_state
action = next_action
return q_table
def epsilon_greedy(q_table, state, epsilon):
if np.random.random() < epsilon:
return np.random.randint(q_table.shape[1])
else:
return np.argmax(q_table[state])
3. Deep Q-Network (DQN)
Deep Q-Network (DQN) is an extension of Q-learning that uses deep neural networks to approximate the Q-function. This allows the algorithm to handle high-dimensional state spaces and continuous action spaces, making it suitable for more complex environments.
Key features of DQN include:
- Experience Replay: Storing and randomly sampling past experiences to break correlations between consecutive samples
- Target Network: Using a separate network for generating target values to improve stability
- Convolutional layers: For processing image-based state representations
Here’s a simplified implementation of DQN using TensorFlow:
import tensorflow as tf
import numpy as np
class DQN:
def __init__(self, state_dim, action_dim, learning_rate):
self.model = self._build_model(state_dim, action_dim)
self.target_model = self._build_model(state_dim, action_dim)
self.optimizer = tf.keras.optimizers.Adam(learning_rate)
def _build_model(self, state_dim, action_dim):
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(state_dim,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(action_dim)
])
return model
def update_target_model(self):
self.target_model.set_weights(self.model.get_weights())
def get_action(self, state, epsilon):
if np.random.random() < epsilon:
return np.random.randint(self.model.output.shape[1])
else:
q_values = self.model(np.array([state]))
return np.argmax(q_values[0])
def train(self, states, actions, rewards, next_states, dones, discount_factor):
with tf.GradientTape() as tape:
q_values = self.model(states)
target_q_values = self.target_model(next_states)
max_next_q_values = tf.reduce_max(target_q_values, axis=1)
targets = rewards + (1 - dones) * discount_factor * max_next_q_values
q_values_selected = tf.reduce_sum(q_values * tf.one_hot(actions, q_values.shape[1]), axis=1)
loss = tf.keras.losses.MSE(targets, q_values_selected)
gradients = tape.gradient(loss, self.model.trainable_variables)
self.optimizer.apply_gradients(zip(gradients, self.model.trainable_variables))
return loss
4. Policy Gradient Methods
Policy Gradient methods are a class of reinforcement learning algorithms that directly optimize the policy without using a value function. These methods are particularly useful for continuous action spaces and can learn stochastic policies. Some popular policy gradient algorithms include:
- REINFORCE
- Actor-Critic
- Proximal Policy Optimization (PPO)
- Trust Region Policy Optimization (TRPO)
Here’s a simple implementation of the REINFORCE algorithm:
import tensorflow as tf
import numpy as np
class REINFORCE:
def __init__(self, state_dim, action_dim, learning_rate):
self.model = self._build_model(state_dim, action_dim)
self.optimizer = tf.keras.optimizers.Adam(learning_rate)
def _build_model(self, state_dim, action_dim):
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(state_dim,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(action_dim, activation='softmax')
])
return model
def get_action(self, state):
probabilities = self.model(np.array([state]))
action = np.random.choice(len(probabilities[0]), p=probabilities[0])
return action
def train(self, states, actions, rewards):
discounted_rewards = self._discount_rewards(rewards)
discounted_rewards = (discounted_rewards - np.mean(discounted_rewards)) / np.std(discounted_rewards)
with tf.GradientTape() as tape:
logits = self.model(np.array(states))
neg_log_prob = tf.nn.sparse_softmax_cross_entropy_with_logits(logits=logits, labels=actions)
loss = tf.reduce_mean(neg_log_prob * discounted_rewards)
gradients = tape.gradient(loss, self.model.trainable_variables)
self.optimizer.apply_gradients(zip(gradients, self.model.trainable_variables))
return loss
def _discount_rewards(self, rewards, gamma=0.99):
discounted_r = np.zeros_like(rewards)
running_add = 0
for t in reversed(range(0, len(rewards))):
running_add = running_add * gamma + rewards[t]
discounted_r[t] = running_add
return discounted_r
Applications of Reinforcement Learning
Reinforcement Learning has found applications in various domains, including:
- Game Playing: RL has achieved superhuman performance in games like Go (AlphaGo), Chess, and various Atari games.
- Robotics: RL is used to teach robots complex tasks and motor skills.
- Autonomous Vehicles: RL algorithms help in developing self-driving cars and drones.
- Resource Management: RL can optimize resource allocation in data centers and power grids.
- Finance: RL is used for algorithmic trading and portfolio management.
- Healthcare: RL algorithms can assist in treatment planning and drug discovery.
- Natural Language Processing: RL is applied in dialogue systems and text summarization.
Challenges and Future Directions
While reinforcement learning has shown remarkable success in various domains, there are still several challenges and areas for improvement:
- Sample Efficiency: Many RL algorithms require a large number of interactions with the environment to learn effectively. Improving sample efficiency is crucial for real-world applications.
- Exploration in High-dimensional Spaces: Efficient exploration in complex, high-dimensional state and action spaces remains a challenge.
- Transfer Learning: Developing RL agents that can transfer knowledge between tasks and environments is an active area of research.
- Safe Exploration: Ensuring that RL agents explore safely, especially in real-world applications like robotics or autonomous vehicles, is critical.
- Interpretability: Making RL algorithms more interpretable and explainable is important for building trust and understanding their decision-making processes.
- Multi-agent RL: Developing efficient algorithms for scenarios involving multiple agents interacting with each other and the environment.
- Continuous Control: Improving performance in continuous action spaces, which are common in robotics and control systems.
Conclusion
Reinforcement Learning is a powerful paradigm in machine learning that enables agents to learn through interaction with their environment. From simple algorithms like Q-learning to more advanced approaches like Deep Q-Networks and Policy Gradient methods, RL has shown remarkable success in various domains.
As research in this field continues to advance, we can expect to see even more sophisticated algorithms and applications of reinforcement learning. The potential for RL to solve complex real-world problems is immense, and it remains an exciting area of study for researchers and practitioners alike.
By understanding the fundamentals of reinforcement learning algorithms and keeping up with the latest developments, you’ll be well-equipped to leverage this powerful approach in your own projects and contribute to the growing field of artificial intelligence.