Introduction to Multithreading and Concurrency: Unleashing the Power of Parallel Programming
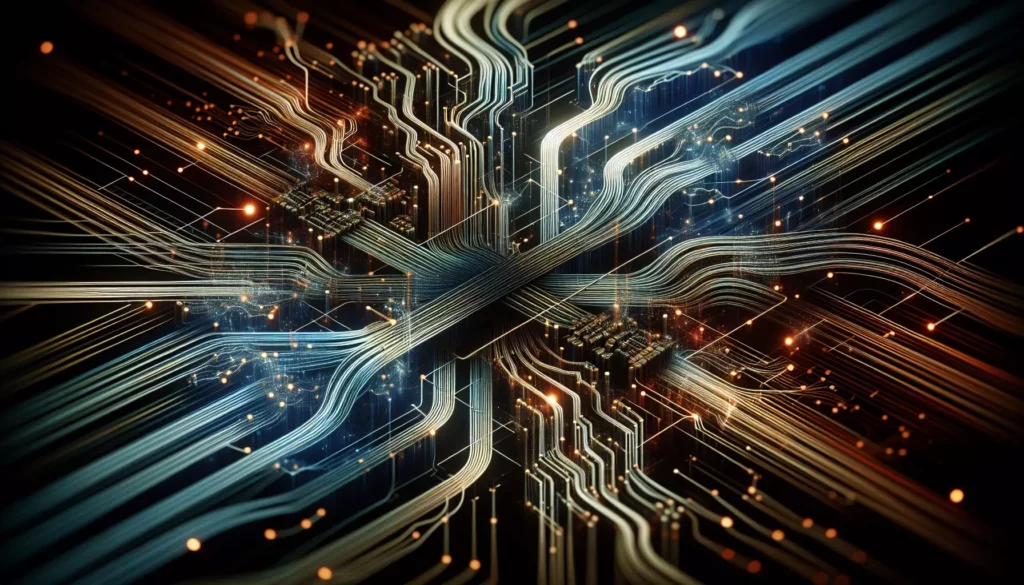
In today’s world of multi-core processors and complex software systems, understanding multithreading and concurrency is crucial for developers aiming to create efficient and responsive applications. This comprehensive guide will introduce you to the concepts of multithreading and concurrency, explore their benefits and challenges, and provide practical examples to help you grasp these essential programming paradigms.
Table of Contents
- What is Multithreading?
- Benefits of Multithreading
- Concurrency vs. Parallelism
- Threads vs. Processes
- Creating and Managing Threads
- Thread Synchronization
- Race Conditions and Deadlocks
- Thread Safety and Atomic Operations
- Common Concurrency Patterns
- Best Practices for Multithreaded Programming
- Debugging Multithreaded Applications
- Performance Considerations
- Real-world Examples of Multithreading
- The Future of Concurrency
- Conclusion
1. What is Multithreading?
Multithreading is a programming concept that allows a single program to execute multiple threads concurrently. A thread is the smallest unit of execution within a process, and it represents an independent path of execution through program code. By utilizing multiple threads, a program can perform multiple tasks simultaneously, improving overall performance and responsiveness.
In a single-threaded application, tasks are executed sequentially, one after another. This can lead to inefficient use of system resources, especially on modern multi-core processors. Multithreading enables programs to take full advantage of available hardware by distributing work across multiple cores, allowing for parallel execution of tasks.
2. Benefits of Multithreading
Implementing multithreading in your applications can offer several advantages:
- Improved Performance: By utilizing multiple CPU cores, multithreaded applications can execute tasks in parallel, significantly reducing overall execution time.
- Enhanced Responsiveness: Multithreading allows for better user interface responsiveness by preventing long-running tasks from blocking the main thread.
- Resource Sharing: Threads within the same process can easily share resources, making it efficient for tasks that require access to common data.
- Simplified Program Structure: Complex programs can be broken down into simpler, more manageable threads, improving code organization and maintainability.
- Scalability: Multithreaded applications can easily scale to take advantage of additional CPU cores as hardware improves.
3. Concurrency vs. Parallelism
While often used interchangeably, concurrency and parallelism are distinct concepts:
- Concurrency: Refers to the ability of a program to handle multiple tasks by switching between them, giving the illusion of simultaneous execution. Concurrent execution can occur on a single-core processor through time-slicing.
- Parallelism: Involves the actual simultaneous execution of multiple tasks on different CPU cores. True parallelism requires multi-core processors.
Understanding the difference between concurrency and parallelism is crucial for designing efficient multithreaded applications. While concurrency focuses on managing multiple tasks, parallelism aims to improve performance by executing tasks simultaneously.
4. Threads vs. Processes
To fully grasp multithreading, it’s essential to understand the difference between threads and processes:
- Process: A process is an instance of a program in execution. It has its own memory space, system resources, and at least one thread of execution (the main thread).
- Thread: A thread is a lightweight unit of execution within a process. Multiple threads in a process share the same memory space and system resources.
Key differences between threads and processes include:
- Threads within a process share memory, while processes have separate memory spaces.
- Thread creation and context switching are generally faster and less resource-intensive than process creation and switching.
- Threads can communicate more easily within a process, while inter-process communication is more complex.
5. Creating and Managing Threads
The exact method for creating and managing threads varies depending on the programming language and framework you’re using. Here’s a general overview of thread creation in a few popular languages:
Java
In Java, you can create threads by either extending the Thread
class or implementing the Runnable
interface. Here’s an example using the Runnable
interface:
public class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running");
}
}
// Create and start the thread
Thread thread = new Thread(new MyRunnable());
thread.start();
Python
Python provides the threading
module for working with threads. Here’s a simple example:
import threading
def print_message():
print("Thread is running")
# Create and start the thread
thread = threading.Thread(target=print_message)
thread.start()
C++
C++11 introduced a standardized way to work with threads using the <thread>
library:
#include <iostream>
#include <thread>
void print_message() {
std::cout << "Thread is running" << std::endl;
}
int main() {
std::thread t(print_message);
t.join();
return 0;
}
6. Thread Synchronization
When multiple threads access shared resources, synchronization becomes crucial to prevent data corruption and ensure thread safety. Common synchronization mechanisms include:
- Mutex (Mutual Exclusion): A lock that ensures only one thread can access a shared resource at a time.
- Semaphore: A synchronization primitive that can allow a specified number of threads to access a resource simultaneously.
- Monitor: A high-level synchronization construct that combines mutex and condition variables.
- Atomic Operations: Operations that are guaranteed to be executed as a single, uninterruptible unit.
Here’s an example of using a mutex in C++:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
int shared_variable = 0;
void increment() {
mtx.lock();
shared_variable++;
mtx.unlock();
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Shared variable: " << shared_variable << std::endl;
return 0;
}
7. Race Conditions and Deadlocks
Two common problems in multithreaded programming are race conditions and deadlocks:
Race Conditions
A race condition occurs when multiple threads access shared data concurrently, and the final result depends on the timing of thread execution. To prevent race conditions, proper synchronization mechanisms should be used.
Deadlocks
A deadlock is a situation where two or more threads are unable to proceed because each is waiting for the other to release a resource. Deadlocks can be prevented by careful resource allocation and by using techniques like lock ordering.
Here’s a simple example of a potential deadlock situation:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mutex1, mutex2;
void thread1_function() {
mutex1.lock();
std::this_thread::sleep_for(std::chrono::milliseconds(1000));
mutex2.lock();
// Critical section
mutex2.unlock();
mutex1.unlock();
}
void thread2_function() {
mutex2.lock();
std::this_thread::sleep_for(std::chrono::milliseconds(1000));
mutex1.lock();
// Critical section
mutex1.unlock();
mutex2.unlock();
}
int main() {
std::thread t1(thread1_function);
std::thread t2(thread2_function);
t1.join();
t2.join();
return 0;
}
In this example, if both threads acquire their first mutex and then attempt to acquire the second, a deadlock can occur. To prevent this, you could implement a consistent lock ordering strategy or use std::lock to acquire multiple locks atomically.
8. Thread Safety and Atomic Operations
Thread safety refers to the property of code that can be safely executed by multiple threads concurrently without causing race conditions or other synchronization issues. Achieving thread safety often involves using synchronization primitives or designing data structures and algorithms that are inherently thread-safe.
Atomic operations are indivisible operations that appear to the rest of the system to occur instantaneously. They are useful for implementing lock-free algorithms and can often provide better performance than traditional locking mechanisms.
Here’s an example of using atomic operations in C++:
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> counter(0);
void increment() {
for (int i = 0; i < 1000000; ++i) {
counter++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Counter value: " << counter << std::endl;
return 0;
}
9. Common Concurrency Patterns
Several design patterns can help manage concurrency in your applications:
- Producer-Consumer: One or more threads produce data, while others consume it, often using a shared buffer.
- Reader-Writer: Multiple threads can read shared data simultaneously, but writes require exclusive access.
- Thread Pool: A fixed number of worker threads are created to process tasks from a queue, improving performance and resource management.
- Barrier: A synchronization primitive that allows multiple threads to wait until all have reached a certain point before proceeding.
Here’s a simple example of a producer-consumer pattern using Java’s BlockingQueue:
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
class Producer implements Runnable {
private final BlockingQueue<Integer> queue;
Producer(BlockingQueue<Integer> q) { queue = q; }
public void run() {
try {
for (int i = 0; i < 10; i++) {
queue.put(i);
System.out.println("Produced: " + i);
Thread.sleep(100);
}
} catch (InterruptedException ex) {
Thread.currentThread().interrupt();
}
}
}
class Consumer implements Runnable {
private final BlockingQueue<Integer> queue;
Consumer(BlockingQueue<Integer> q) { queue = q; }
public void run() {
try {
while (true) {
Integer item = queue.take();
System.out.println("Consumed: " + item);
}
} catch (InterruptedException ex) {
Thread.currentThread().interrupt();
}
}
}
public class ProducerConsumerExample {
public static void main(String[] args) {
BlockingQueue<Integer> queue = new LinkedBlockingQueue<>(5);
new Thread(new Producer(queue)).start();
new Thread(new Consumer(queue)).start();
}
}
10. Best Practices for Multithreaded Programming
When working with multithreaded applications, consider the following best practices:
- Minimize shared mutable state to reduce the need for synchronization.
- Use higher-level concurrency constructs (e.g., java.util.concurrent in Java) when possible.
- Prefer immutable objects and thread-local variables where appropriate.
- Be cautious with nested locks to avoid deadlocks.
- Use timeouts when acquiring locks to prevent indefinite waiting.
- Document thread safety guarantees for your classes and methods.
- Use thread-safe data structures when sharing data between threads.
- Implement proper error handling and logging in multithreaded code.
11. Debugging Multithreaded Applications
Debugging multithreaded applications can be challenging due to the non-deterministic nature of thread execution. Some strategies for effective debugging include:
- Use thread-aware debuggers that can display and control individual threads.
- Implement comprehensive logging to track thread activities and state changes.
- Utilize thread dumps and stack traces to analyze thread states and detect deadlocks.
- Consider using specialized tools for detecting race conditions and other concurrency issues (e.g., ThreadSanitizer, Helgrind).
- Simplify your code by isolating multithreaded sections for easier debugging.
12. Performance Considerations
While multithreading can significantly improve performance, it’s essential to consider the following factors:
- Thread Creation Overhead: Creating and destroying threads has a cost. Consider using thread pools for better performance in scenarios with many short-lived tasks.
- Context Switching: Excessive thread switching can lead to performance degradation. Balance the number of threads with the available CPU cores.
- Synchronization Overhead: Heavy use of locks and other synchronization primitives can impact performance. Use lock-free algorithms and fine-grained locking where possible.
- False Sharing: Occurs when threads on different CPU cores access data that resides on the same cache line, leading to unnecessary cache invalidations. Be mindful of data layout to avoid false sharing.
- Load Balancing: Ensure work is evenly distributed among threads to maximize parallelism and avoid bottlenecks.
13. Real-world Examples of Multithreading
Multithreading is widely used in various applications and systems:
- Web Servers: Handle multiple client requests concurrently.
- Database Management Systems: Process multiple queries simultaneously.
- Video Games: Manage graphics rendering, physics simulations, and AI in parallel.
- Image and Video Processing: Distribute pixel or frame processing across multiple threads.
- Scientific Simulations: Parallelize complex calculations for faster results.
- Operating Systems: Manage multiple processes and system tasks concurrently.
14. The Future of Concurrency
As hardware continues to evolve and software complexity increases, the future of concurrency looks promising:
- Increased Core Counts: With CPUs offering more cores, efficient multithreading will become even more critical.
- Heterogeneous Computing: Utilizing specialized processors (e.g., GPUs, TPUs) alongside CPUs for parallel processing.
- New Programming Models: Development of more intuitive and safer concurrency models and languages.
- Quantum Computing: Potential for massive parallelism in certain problem domains.
- AI and Machine Learning: Continued focus on parallelizing AI algorithms and models.
15. Conclusion
Multithreading and concurrency are fundamental concepts in modern software development, enabling developers to create efficient, responsive, and scalable applications. By understanding these principles and applying best practices, you can harness the full power of modern hardware and create sophisticated, high-performance software systems.
As you continue your journey in software development, remember that mastering multithreading and concurrency is an ongoing process. Stay curious, keep practicing, and always be on the lookout for new techniques and technologies in this exciting field.
Happy coding, and may your threads always run smoothly!