Introduction to Modular Programming: Breaking Code into Functions and Modules
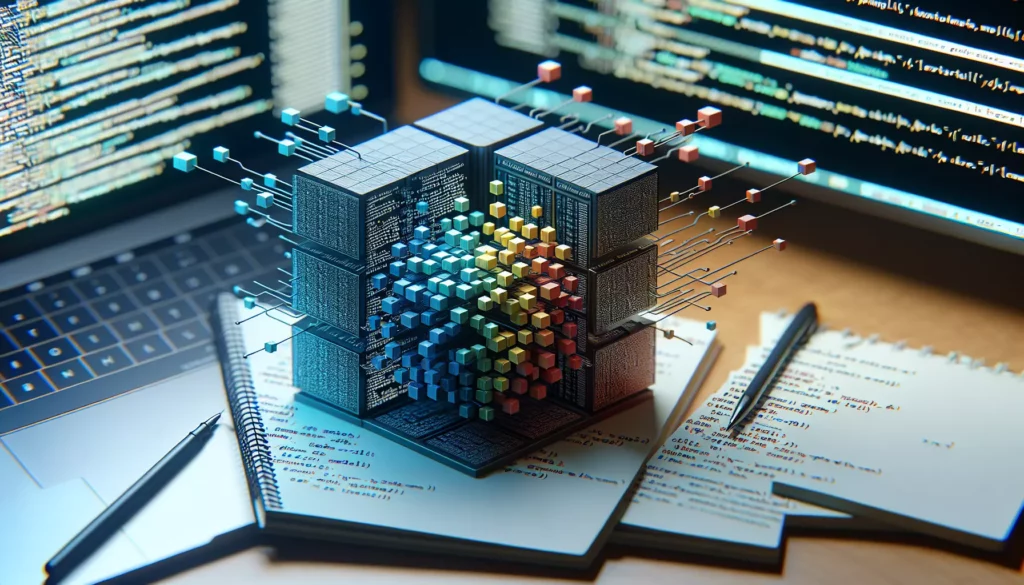
In the world of programming, writing clean, maintainable, and reusable code is a crucial skill that separates novice programmers from seasoned professionals. One of the fundamental techniques to achieve this is through modular programming. This approach involves breaking down complex programs into smaller, manageable pieces called functions and modules. In this comprehensive guide, we’ll explore the concept of modular programming, its benefits, and how to implement it effectively in your code.
What is Modular Programming?
Modular programming is a software design technique that emphasizes separating the functionality of a program into independent, interchangeable modules. Each module contains everything necessary to execute only one aspect of the desired functionality. This approach allows for better organization, easier maintenance, and improved reusability of code.
The two primary building blocks of modular programming are:
- Functions: Self-contained blocks of code that perform a specific task.
- Modules: Collections of related functions, classes, and variables that can be imported and used in other parts of a program.
The Benefits of Modular Programming
Adopting a modular approach to programming offers numerous advantages:
- Improved Readability: By breaking code into smaller, focused pieces, it becomes easier to understand and follow the logic of the program.
- Enhanced Maintainability: Modular code is easier to update and debug, as changes can be made to specific functions or modules without affecting the entire program.
- Increased Reusability: Well-designed modules can be reused in multiple projects, saving time and effort in future development.
- Better Collaboration: Modular code allows multiple developers to work on different parts of a project simultaneously without interfering with each other’s work.
- Easier Testing: Individual functions and modules can be tested in isolation, making it simpler to identify and fix bugs.
- Scalability: As projects grow, modular design makes it easier to add new features and functionality without compromising the existing codebase.
Breaking Code into Functions
Functions are the building blocks of modular programming. They encapsulate a specific task or calculation, making your code more organized and easier to understand. Let’s look at how to create and use functions effectively:
Anatomy of a Function
A typical function consists of the following components:
- Function name
- Parameters (optional)
- Function body
- Return statement (optional)
Here’s a basic example of a function in Python:
def greet(name):
return f"Hello, {name}!"
# Using the function
message = greet("Alice")
print(message) # Output: Hello, Alice!
Best Practices for Creating Functions
- Single Responsibility Principle: Each function should have a single, well-defined purpose. If a function is doing too many things, consider breaking it into smaller functions.
- Descriptive Names: Choose clear and descriptive names for your functions that indicate what they do. For example,
calculate_area()
is better thancalc_a()
. - Keep Functions Short: Aim to keep functions concise and focused. If a function becomes too long or complex, it might be a sign that it should be broken down further.
- Use Parameters Wisely: Pass necessary data as parameters rather than relying on global variables. This makes functions more flexible and reusable.
- Return Values: When appropriate, have functions return values instead of modifying global state. This promotes a more functional programming style and makes testing easier.
Example: Refactoring Code into Functions
Let’s look at an example of how we can refactor a piece of code to use functions:
Before refactoring:
# Calculate the area of a rectangle
length = 10
width = 5
area = length * width
print(f"The area of the rectangle is: {area}")
# Calculate the perimeter of a rectangle
perimeter = 2 * (length + width)
print(f"The perimeter of the rectangle is: {perimeter}")
# Calculate the volume of a rectangular prism
height = 3
volume = length * width * height
print(f"The volume of the rectangular prism is: {volume}")
After refactoring:
def calculate_rectangle_area(length, width):
return length * width
def calculate_rectangle_perimeter(length, width):
return 2 * (length + width)
def calculate_rectangular_prism_volume(length, width, height):
return length * width * height
def print_result(calculation_name, result):
print(f"The {calculation_name} is: {result}")
# Using the functions
length = 10
width = 5
height = 3
area = calculate_rectangle_area(length, width)
print_result("area of the rectangle", area)
perimeter = calculate_rectangle_perimeter(length, width)
print_result("perimeter of the rectangle", perimeter)
volume = calculate_rectangular_prism_volume(length, width, height)
print_result("volume of the rectangular prism", volume)
As you can see, the refactored code is more organized, easier to read, and more maintainable. Each calculation is now encapsulated in its own function, making it simpler to reuse these calculations elsewhere in the program if needed.
Creating and Using Modules
While functions help organize code within a single file, modules allow you to organize code across multiple files. A module is typically a Python file containing functions, classes, and variables that can be imported and used in other parts of your program.
Creating a Module
To create a module, simply save your Python code in a file with a .py
extension. For example, let’s create a module called geometry.py
that contains our rectangle and prism calculations:
# geometry.py
def calculate_rectangle_area(length, width):
return length * width
def calculate_rectangle_perimeter(length, width):
return 2 * (length + width)
def calculate_rectangular_prism_volume(length, width, height):
return length * width * height
Importing and Using Modules
Once you’ve created a module, you can import it into other Python scripts using the import
statement. There are several ways to import modules:
- Importing the entire module:
import geometry
area = geometry.calculate_rectangle_area(10, 5)
print(f"The area is: {area}")
- Importing specific functions from a module:
from geometry import calculate_rectangle_area, calculate_rectangle_perimeter
area = calculate_rectangle_area(10, 5)
perimeter = calculate_rectangle_perimeter(10, 5)
print(f"The area is: {area}")
print(f"The perimeter is: {perimeter}")
- Importing all functions from a module (use with caution):
from geometry import *
area = calculate_rectangle_area(10, 5)
perimeter = calculate_rectangle_perimeter(10, 5)
volume = calculate_rectangular_prism_volume(10, 5, 3)
print(f"The area is: {area}")
print(f"The perimeter is: {perimeter}")
print(f"The volume is: {volume}")
Note: While importing all functions using *
can be convenient, it’s generally not recommended for larger projects as it can lead to naming conflicts and make it harder to track where functions are coming from.
Best Practices for Creating and Using Modules
- Logical Organization: Group related functions and classes into modules based on their functionality or purpose.
- Clear Naming: Use descriptive names for your modules that reflect their contents. For example,
data_processing.py
oruser_authentication.py
. - Documentation: Include docstrings at the beginning of your module and for each function to explain their purpose and usage.
- Avoid Circular Imports: Be careful not to create circular dependencies between modules, as this can lead to import errors.
- Use Relative Imports: When importing from modules within the same package, use relative imports to make the code more portable.
Advanced Modular Programming Concepts
Packages
As your project grows, you may want to organize related modules into packages. A package is simply a directory containing a special file called __init__.py
(which can be empty) and one or more module files.
Here’s an example of a package structure:
my_package/
__init__.py
module1.py
module2.py
subpackage/
__init__.py
module3.py
You can then import modules from this package like this:
from my_package import module1
from my_package.subpackage import module3
Object-Oriented Programming and Modular Design
Object-Oriented Programming (OOP) complements modular programming by allowing you to create reusable and organized code through classes and objects. Classes can be thought of as blueprints for creating objects that encapsulate both data (attributes) and behavior (methods).
Here’s an example of how you might use OOP principles in our geometry module:
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
def perimeter(self):
return 2 * (self.length + self.width)
class RectangularPrism(Rectangle):
def __init__(self, length, width, height):
super().__init__(length, width)
self.height = height
def volume(self):
return self.area() * self.height
# Using the classes
rect = Rectangle(10, 5)
print(f"Rectangle area: {rect.area()}")
print(f"Rectangle perimeter: {rect.perimeter()}")
prism = RectangularPrism(10, 5, 3)
print(f"Prism volume: {prism.volume()}")
This approach allows for even more organized and reusable code, as the properties and behaviors of shapes are encapsulated within their respective classes.
Design Patterns
Design patterns are reusable solutions to common problems in software design. They can help you create more modular and flexible code. Some popular design patterns include:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable.
Understanding and applying these patterns can significantly improve the modularity and flexibility of your code.
Conclusion
Modular programming is a powerful technique that can greatly improve the quality, maintainability, and reusability of your code. By breaking your programs into functions and modules, you create more organized and manageable codebases that are easier to understand, test, and extend.
As you continue to develop your programming skills, keep these principles in mind:
- Strive to write functions that have a single, clear purpose.
- Organize related functions into modules.
- Use descriptive names for your functions and modules.
- Take advantage of object-oriented programming principles when appropriate.
- Learn and apply design patterns to solve common programming challenges.
Remember, becoming proficient in modular programming takes practice. As you work on various projects, continuously look for opportunities to refactor your code into more modular structures. Over time, you’ll develop an intuition for when and how to break down complex problems into smaller, more manageable pieces.
By mastering modular programming, you’ll not only write better code but also be better prepared for collaborative development environments and larger-scale projects. This skill is invaluable whether you’re working on personal projects, contributing to open-source software, or preparing for technical interviews at major tech companies.
Keep practicing, stay curious, and happy coding!