Introduction to Handling Dates and Times in Code
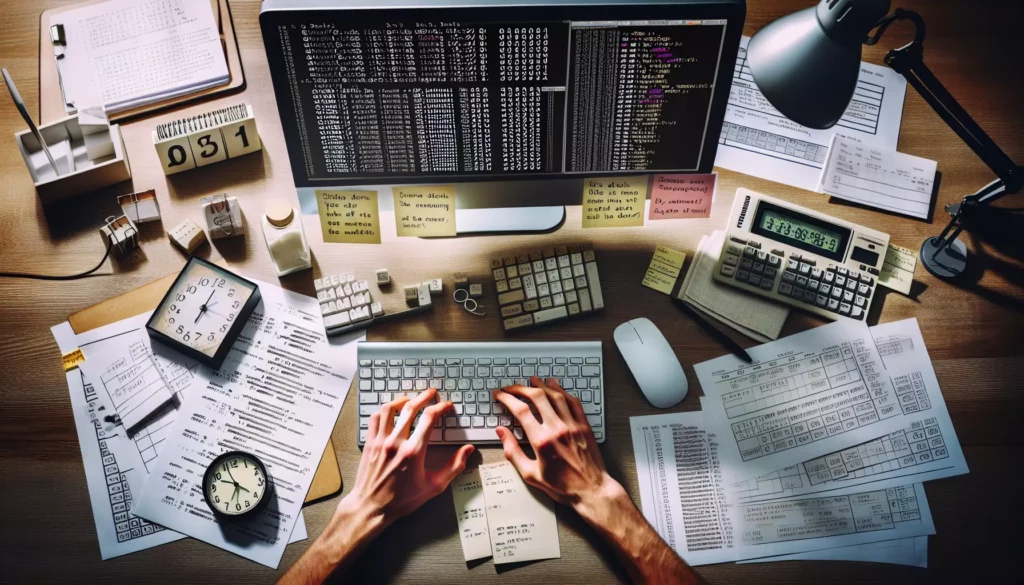
In the world of programming, dealing with dates and times is a common and crucial task. Whether you’re building a scheduling application, analyzing time-series data, or simply need to display the current date on a website, understanding how to work with dates and times is essential. This blog post will introduce you to the fundamentals of handling dates and times in code, covering various programming languages and best practices.
Why Date and Time Handling is Important
Before we dive into the specifics, let’s consider why mastering date and time manipulation is crucial for developers:
- Accuracy: Many applications rely on precise timing for critical operations.
- User Experience: Displaying dates and times in a user-friendly format enhances the overall user experience.
- Data Analysis: Time-based data is crucial in fields like finance, science, and business intelligence.
- Scheduling: Applications that involve scheduling or time-based events require robust date and time handling.
- Internationalization: Different regions have varying date and time formats, making proper handling essential for global applications.
Basic Concepts
1. Timestamps
A timestamp is a sequence of characters or encoded information identifying when a certain event occurred, usually giving date and time of day, sometimes accurate to a small fraction of a second. In most programming languages, timestamps are represented as the number of seconds (or milliseconds) that have elapsed since the Unix Epoch (January 1, 1970, at 00:00:00 UTC).
2. Date Objects
Many programming languages provide built-in date objects or classes that encapsulate date and time information. These objects often come with methods to perform various operations like formatting, arithmetic, and comparisons.
3. Time Zones
Time zones add complexity to date and time handling. It’s crucial to consider time zones when working with dates, especially in applications that serve users across different regions.
4. Formatting
Date and time formatting refers to how dates and times are displayed or parsed. Different regions have different conventions for representing dates (e.g., MM/DD/YYYY in the US, DD/MM/YYYY in many European countries).
Handling Dates and Times in Different Programming Languages
Python
Python provides the datetime
module for working with dates and times. Here’s a basic example:
from datetime import datetime, timedelta
# Get current date and time
now = datetime.now()
print(f"Current date and time: {now}")
# Format date
formatted_date = now.strftime("%Y-%m-%d %H:%M:%S")
print(f"Formatted date: {formatted_date}")
# Date arithmetic
future_date = now + timedelta(days=7)
print(f"Date after 7 days: {future_date}")
# Parsing date string
date_string = "2023-06-15 14:30:00"
parsed_date = datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S")
print(f"Parsed date: {parsed_date}")
JavaScript
JavaScript has a built-in Date
object for working with dates and times:
// Get current date and time
const now = new Date();
console.log("Current date and time:", now);
// Format date
const options = { year: 'numeric', month: 'long', day: 'numeric', hour: '2-digit', minute: '2-digit' };
console.log("Formatted date:", now.toLocaleString('en-US', options));
// Date arithmetic
const futureDate = new Date(now.getTime() + 7 * 24 * 60 * 60 * 1000);
console.log("Date after 7 days:", futureDate);
// Parsing date string
const dateString = "2023-06-15T14:30:00";
const parsedDate = new Date(dateString);
console.log("Parsed date:", parsedDate);
Java
Java 8 introduced the java.time
package, which provides a comprehensive API for date and time handling:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeExample {
public static void main(String[] args) {
// Get current date and time
LocalDateTime now = LocalDateTime.now();
System.out.println("Current date and time: " + now);
// Format date
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDate = now.format(formatter);
System.out.println("Formatted date: " + formattedDate);
// Date arithmetic
LocalDateTime futureDate = now.plusDays(7);
System.out.println("Date after 7 days: " + futureDate);
// Parsing date string
String dateString = "2023-06-15 14:30:00";
LocalDateTime parsedDate = LocalDateTime.parse(dateString, formatter);
System.out.println("Parsed date: " + parsedDate);
}
}
Common Challenges and Best Practices
1. Time Zone Handling
When working with dates and times, it’s crucial to consider time zones, especially in applications that serve users across different regions. Here are some best practices:
- Store dates in UTC: When storing dates in a database, it’s generally recommended to use UTC (Coordinated Universal Time) to avoid ambiguity.
- Convert to local time for display: When displaying dates to users, convert them to the user’s local time zone.
- Use libraries for time zone conversions: Time zone rules are complex and change frequently. Use reliable libraries like
pytz
for Python ormoment-timezone
for JavaScript to handle time zone conversions.
Here’s an example of time zone handling in Python:
from datetime import datetime
from pytz import timezone
# Create a datetime in UTC
utc_time = datetime.now(timezone('UTC'))
print(f"UTC time: {utc_time}")
# Convert to a different time zone
ny_time = utc_time.astimezone(timezone('America/New_York'))
print(f"New York time: {ny_time}")
2. Daylight Saving Time (DST)
Daylight Saving Time adds another layer of complexity to date and time handling. When working with dates that might fall during DST transitions, be extra cautious. Most modern date and time libraries handle DST automatically, but it’s important to be aware of potential issues.
3. Date Arithmetic
Performing arithmetic operations on dates (like adding days or calculating the difference between two dates) can be tricky. Be aware of edge cases like leap years and month boundaries. Most date and time libraries provide methods for safe date arithmetic.
4. Formatting and Parsing
When formatting dates for display or parsing date strings, always use standard format specifiers or parsing patterns. This ensures consistency and reduces the risk of errors. Additionally, consider internationalization requirements when formatting dates.
5. Testing
Thoroughly test your date and time handling code, especially around edge cases like:
- DST transitions
- Leap years
- Date/time rollovers (e.g., year, month, day changes)
- Different time zones
Advanced Topics
1. Working with Time Series Data
In data analysis and scientific computing, working with time series data is common. Libraries like Pandas in Python provide powerful tools for handling time series:
import pandas as pd
# Create a date range
date_range = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
# Create a time series
ts = pd.Series(range(len(date_range)), index=date_range)
# Resample to monthly frequency
monthly_ts = ts.resample('M').mean()
print(monthly_ts)
2. Handling Recurring Events
For applications that deal with recurring events (like calendar applications), you might need to work with more complex date patterns. Libraries like rrule
in Python can be helpful:
from dateutil.rrule import rrule, DAILY, MO, WE, FR
# Create a rule for every Monday, Wednesday, and Friday
rule = rrule(DAILY, byweekday=(MO, WE, FR), dtstart=datetime(2023, 1, 1), until=datetime(2023, 12, 31))
# Get all occurrences
occurrences = list(rule)
for date in occurrences[:5]:
print(date)
3. Performance Considerations
When working with large amounts of date and time data, performance can become a concern. Here are some tips:
- Use appropriate data types: For example, if you only need date information without time, use a date-only type to save memory.
- Batch operations: When performing operations on many dates, try to batch them for better performance.
- Consider using specialized libraries: For high-performance date and time operations, consider using libraries optimized for such tasks.
Conclusion
Handling dates and times is a fundamental skill for any programmer. While it may seem straightforward at first, it can quickly become complex when dealing with time zones, different formats, and various edge cases. By understanding the basics, following best practices, and leveraging the right tools and libraries, you can effectively manage date and time operations in your code.
Remember, the key to mastering date and time handling is practice. Try working on projects that involve different aspects of date and time manipulation, such as building a calendar application, analyzing time series data, or creating a scheduling system. As you gain more experience, you’ll become more comfortable with the intricacies of working with dates and times in various programming contexts.
Lastly, always stay updated with the latest best practices and tools in your programming language of choice. Date and time handling libraries are continually evolving, often introducing new features and improvements that can make your code more efficient and robust.
Further Resources
To deepen your understanding of date and time handling in code, consider exploring these resources:
- Python datetime documentation
- JavaScript Date object documentation
- Java 8 java.time package documentation
- Pandas Time Series documentation
- Python dateutil library
By mastering date and time handling, you’ll be better equipped to tackle a wide range of programming challenges and build more robust, user-friendly applications. Happy coding!