Introduction to GraphQL APIs: Basics for Beginners
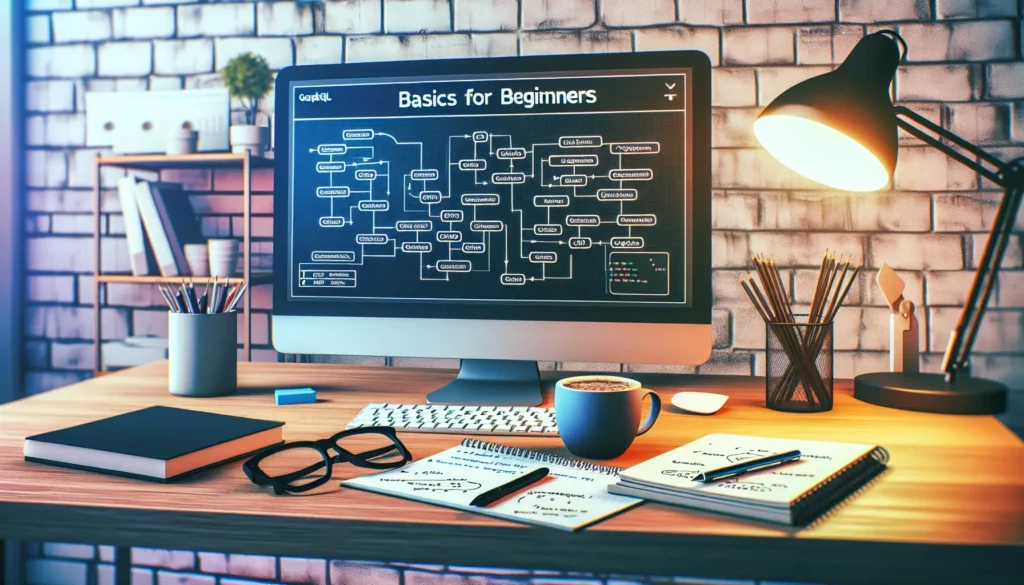
In the ever-evolving world of web development, efficient data fetching and manipulation have become crucial. As applications grow more complex, the need for flexible and powerful APIs has never been greater. Enter GraphQL, a revolutionary query language for APIs that has taken the developer community by storm. In this comprehensive guide, we’ll dive deep into the basics of GraphQL APIs, exploring why they’ve become so popular and how you can start using them in your projects.
What is GraphQL?
GraphQL is a query language and runtime for APIs that was developed by Facebook in 2012 and open-sourced in 2015. Unlike traditional REST APIs, GraphQL allows clients to request exactly the data they need, nothing more and nothing less. This flexibility makes it an excellent choice for modern web and mobile applications where efficiency and speed are paramount.
The name “GraphQL” comes from “Graph Query Language,” which reflects its ability to traverse relational data as if it were a graph. This approach enables developers to think about their data in terms of a schema with fields that are connected, rather than as separate API endpoints.
Key Concepts in GraphQL
Before we dive into the practical aspects of using GraphQL, let’s familiarize ourselves with some key concepts:
1. Schema
The schema is the heart of any GraphQL API. It defines the structure of your data and the operations that can be performed. A schema consists of object types, which represent the kinds of objects you can query, and fields on those types.
2. Queries
Queries in GraphQL are used to request data from the server. They allow clients to specify exactly what data they need, including nested relationships between objects.
3. Mutations
Mutations are used to modify data on the server. They allow clients to create, update, or delete data.
4. Resolvers
Resolvers are functions that determine how to fetch the data for each field in your schema. They define the logic for retrieving or modifying data from your data source.
Why Use GraphQL?
GraphQL offers several advantages over traditional REST APIs:
- Precise Data Fetching: Clients can request exactly the data they need, reducing over-fetching and under-fetching of data.
- Single Request, Multiple Resources: GraphQL allows you to fetch related data in a single request, even if it spans multiple resources.
- Strong Typing: GraphQL uses a strong type system to define the API’s capabilities, making it easier to develop robust and maintainable applications.
- Introspection: GraphQL APIs are self-documenting, allowing clients to query the schema for information about what queries are available.
- Versioning: GraphQL makes it easier to evolve your API over time without breaking existing queries.
Getting Started with GraphQL
Now that we understand the basics, let’s walk through setting up a simple GraphQL server using Node.js and the Apollo Server library.
Step 1: Setting Up the Project
First, create a new directory for your project and initialize a new Node.js project:
mkdir graphql-demo
cd graphql-demo
npm init -y
Next, install the necessary dependencies:
npm install apollo-server graphql
Step 2: Defining the Schema
Create a new file called schema.js
and define your GraphQL schema:
const { gql } = require('apollo-server');
const typeDefs = gql`
type Book {
id: ID!
title: String!
author: String!
}
type Query {
books: [Book]
book(id: ID!): Book
}
`;
module.exports = typeDefs;
This schema defines a Book
type and two queries: one to fetch all books and another to fetch a specific book by ID.
Step 3: Implementing Resolvers
Create a file called resolvers.js
to implement the logic for fetching data:
const books = [
{ id: '1', title: 'The Great Gatsby', author: 'F. Scott Fitzgerald' },
{ id: '2', title: 'To Kill a Mockingbird', author: 'Harper Lee' },
];
const resolvers = {
Query: {
books: () => books,
book: (_, { id }) => books.find(book => book.id === id),
},
};
module.exports = resolvers;
Step 4: Setting Up the Server
Create a file called server.js
to set up and start the Apollo Server:
const { ApolloServer } = require('apollo-server');
const typeDefs = require('./schema');
const resolvers = require('./resolvers');
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
Step 5: Running the Server
Start your GraphQL server by running:
node server.js
You should see a message indicating that the server is running, typically at http://localhost:4000
.
Querying Your GraphQL API
With your server up and running, you can now query your GraphQL API. Open your browser and navigate to the URL provided (usually http://localhost:4000
). You’ll see the Apollo Server Playground, which allows you to interact with your API.
Try running the following query to fetch all books:
{
books {
id
title
author
}
}
You should see a response like this:
{
"data": {
"books": [
{
"id": "1",
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald"
},
{
"id": "2",
"title": "To Kill a Mockingbird",
"author": "Harper Lee"
}
]
}
}
Now, let’s fetch a specific book by ID:
{
book(id: "1") {
title
author
}
}
The response should be:
{
"data": {
"book": {
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald"
}
}
}
Advanced GraphQL Concepts
While we’ve covered the basics, GraphQL offers many more advanced features that make it a powerful tool for building APIs. Let’s explore some of these concepts:
1. Mutations
Mutations allow you to modify data on the server. Let’s add a mutation to our schema to create a new book:
const { gql } = require('apollo-server');
const typeDefs = gql`
type Book {
id: ID!
title: String!
author: String!
}
type Query {
books: [Book]
book(id: ID!): Book
}
type Mutation {
addBook(title: String!, author: String!): Book
}
`;
module.exports = typeDefs;
Now, update your resolvers to handle this mutation:
const books = [
{ id: '1', title: 'The Great Gatsby', author: 'F. Scott Fitzgerald' },
{ id: '2', title: 'To Kill a Mockingbird', author: 'Harper Lee' },
];
const resolvers = {
Query: {
books: () => books,
book: (_, { id }) => books.find(book => book.id === id),
},
Mutation: {
addBook: (_, { title, author }) => {
const newBook = { id: String(books.length + 1), title, author };
books.push(newBook);
return newBook;
},
},
};
module.exports = resolvers;
You can now add a new book using a mutation:
mutation {
addBook(title: "1984", author: "George Orwell") {
id
title
author
}
}
2. Fragments
Fragments are reusable units of a query. They’re useful when you have repetitive fields in multiple queries. For example:
fragment BookDetails on Book {
id
title
author
}
query {
books {
...BookDetails
}
book(id: "1") {
...BookDetails
}
}
3. Interfaces and Union Types
Interfaces allow you to define a set of fields that multiple types can implement. Union types, on the other hand, let you return one of several possible object types from a field.
interface Media {
id: ID!
title: String!
}
type Book implements Media {
id: ID!
title: String!
author: String!
}
type Movie implements Media {
id: ID!
title: String!
director: String!
}
union SearchResult = Book | Movie
type Query {
search(term: String!): [SearchResult]
}
4. Subscriptions
Subscriptions allow clients to receive real-time updates when data changes on the server. They’re particularly useful for building real-time features like chat applications or live updates.
type Subscription {
bookAdded: Book
}
Best Practices for GraphQL APIs
As you begin working with GraphQL, keep these best practices in mind:
- Design Your Schema Carefully: Your schema is the contract between your server and clients. Take time to design it thoughtfully.
- Use Descriptive Names: Choose clear and descriptive names for types, fields, and operations.
- Implement Pagination: For large datasets, implement pagination to improve performance and user experience.
- Handle Errors Gracefully: Use the built-in error handling features of GraphQL to provide meaningful error messages to clients.
- Optimize Performance: Use techniques like batching and caching to improve the performance of your API.
- Secure Your API: Implement authentication and authorization to protect sensitive data.
- Version Your Schema: Use deprecation and schema evolution techniques to manage changes to your API over time.
Tools and Libraries for GraphQL Development
The GraphQL ecosystem is rich with tools and libraries to help you build and interact with GraphQL APIs:
- Apollo Client: A comprehensive state management library for JavaScript that integrates with GraphQL.
- GraphQL Playground: An interactive, in-browser GraphQL IDE for exploring and testing GraphQL APIs.
- GraphQL Code Generator: A tool that generates code from your GraphQL schema for various languages and frameworks.
- DataLoader: A utility to batch and cache database queries to solve the N+1 problem in GraphQL resolvers.
- GraphQL Voyager: A tool to visualize your GraphQL schema as an interactive graph.
Conclusion
GraphQL represents a significant shift in how we think about and implement APIs. Its flexibility, efficiency, and developer-friendly features make it an excellent choice for modern web and mobile applications. As you continue your journey with GraphQL, you’ll discover even more powerful features and patterns that can help you build robust and scalable APIs.
Remember, the key to mastering GraphQL is practice. Start by building small projects, experiment with different features, and gradually incorporate more advanced concepts as you become comfortable with the basics. With its growing ecosystem and supportive community, GraphQL is well-positioned to be a crucial part of the web development landscape for years to come.
As you progress in your GraphQL journey, don’t forget to explore how it can be integrated with other technologies and frameworks you’re familiar with. Whether you’re working with React, Node.js, or any other modern web technology, GraphQL can likely enhance your development workflow and improve the performance of your applications.
Happy coding, and may your queries be ever efficient!