Introduction to Functional Programming Principles
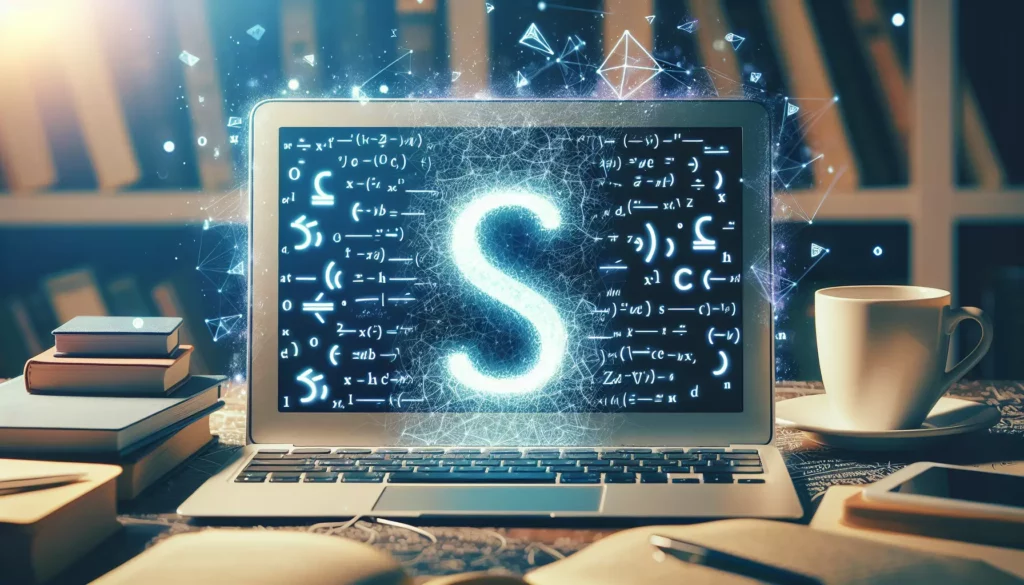
In the ever-evolving landscape of software development, functional programming has gained significant traction in recent years. This paradigm, which emphasizes the use of pure functions and immutable data, offers a different approach to solving complex problems and building robust applications. In this comprehensive guide, we’ll explore the core principles of functional programming, its benefits, and how it compares to other programming paradigms.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It’s a declarative style of programming, which means that the program logic is expressed without explicitly describing the control flow.
The key idea behind functional programming is to break down a problem into a set of functions. Ideally, functions only take inputs and produce outputs, and don’t have any internal state or side effects that affect the outside world.
Core Principles of Functional Programming
1. Pure Functions
A pure function is a function that:
- Always produces the same output for the same input
- Has no side effects (doesn’t modify any external state)
Here’s an example of a pure function in JavaScript:
function add(a, b) {
return a + b;
}
console.log(add(2, 3)); // Always outputs 5
This function is pure because it always returns the same result for the same inputs and doesn’t modify any external state.
2. Immutability
Immutability is the practice of not changing data once it’s created. Instead of modifying existing data, functional programming creates new data structures. This leads to more predictable code and makes it easier to track changes over time.
Here’s an example of working with immutable data in JavaScript:
const originalArray = [1, 2, 3];
const newArray = [...originalArray, 4];
console.log(originalArray); // [1, 2, 3]
console.log(newArray); // [1, 2, 3, 4]
In this example, instead of modifying the original array, we create a new array with the additional element.
3. First-Class and Higher-Order Functions
In functional programming, functions are treated as first-class citizens. This means they can be:
- Assigned to variables
- Passed as arguments to other functions
- Returned from functions
Higher-order functions are functions that can take other functions as arguments or return them. This enables powerful abstractions and code reuse.
Here’s an example of a higher-order function in JavaScript:
function multiplyBy(factor) {
return function(number) {
return number * factor;
}
}
const double = multiplyBy(2);
console.log(double(5)); // Outputs 10
4. Recursion
Recursion is a technique where a function calls itself to solve a problem. It’s often used in functional programming as an alternative to imperative loops. Recursive functions can lead to elegant solutions for complex problems.
Here’s an example of a recursive function to calculate factorial:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
console.log(factorial(5)); // Outputs 120
5. Function Composition
Function composition is the process of combining two or more functions to produce a new function. It’s a fundamental concept in functional programming that allows for building complex operations from simpler ones.
Here’s an example of function composition in JavaScript:
const add = x => x + 2;
const multiply = x => x * 3;
const addAndMultiply = x => multiply(add(x));
console.log(addAndMultiply(5)); // Outputs 21
Benefits of Functional Programming
1. Predictability
Because functional programs are composed of pure functions and immutable data, they are more predictable and easier to understand. The output of a function depends only on its inputs, making it easier to reason about the code’s behavior.
2. Testability
Pure functions are inherently easier to test because they always produce the same output for a given input. This eliminates the need for complex setup and teardown procedures in unit tests.
3. Parallel Processing
The absence of side effects and mutable state makes functional programs well-suited for parallel processing. Different functions can be executed simultaneously without worrying about race conditions or shared state.
4. Modularity
Functional programming encourages breaking down complex problems into smaller, reusable functions. This leads to more modular code that’s easier to maintain and refactor.
5. Debugging
With pure functions and immutable data, bugs are often easier to locate and fix. The flow of data is more transparent, making it easier to trace the source of errors.
Functional Programming vs. Other Paradigms
Functional vs. Imperative Programming
Imperative programming focuses on describing how a program operates, step by step. It relies heavily on statements that change program state. In contrast, functional programming describes what the program should accomplish without explicitly stating how to do it.
Here’s a comparison of imperative and functional approaches to summing an array:
// Imperative approach
function sumArrayImperative(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// Functional approach
function sumArrayFunctional(arr) {
return arr.reduce((sum, num) => sum + num, 0);
}
const numbers = [1, 2, 3, 4, 5];
console.log(sumArrayImperative(numbers)); // Outputs 15
console.log(sumArrayFunctional(numbers)); // Outputs 15
Functional vs. Object-Oriented Programming
Object-Oriented Programming (OOP) organizes code into objects that contain both data and behavior. Functional programming, on the other hand, separates data and behavior, focusing on functions that operate on data.
Here’s a simple example comparing OOP and functional approaches:
// Object-Oriented approach
class Circle {
constructor(radius) {
this.radius = radius;
}
area() {
return Math.PI * this.radius * this.radius;
}
}
const circle = new Circle(5);
console.log(circle.area()); // Outputs approximately 78.54
// Functional approach
function circleArea(radius) {
return Math.PI * radius * radius;
}
console.log(circleArea(5)); // Outputs approximately 78.54
Functional Programming Languages and Tools
While functional programming principles can be applied in many languages, some languages are specifically designed with functional programming in mind:
- Haskell: A purely functional programming language
- Scala: Combines object-oriented and functional programming paradigms
- Clojure: A modern Lisp dialect that runs on the Java Virtual Machine
- F#: Microsoft’s functional programming language for the .NET ecosystem
- Erlang: Known for its support for concurrency and distributed systems
Many mainstream languages also support functional programming concepts:
- JavaScript: With features like arrow functions, map, reduce, and filter
- Python: Supports functional programming with functions like map, filter, and lambda expressions
- Java: Introduced functional interfaces and the Stream API in Java 8
- C#: Supports LINQ and lambda expressions for functional-style programming
Getting Started with Functional Programming
If you’re new to functional programming, here are some steps to get started:
- Learn the basics: Start by understanding the core principles of functional programming.
- Practice with pure functions: Try to write functions that don’t have side effects and always return the same output for the same input.
- Embrace immutability: Get comfortable with creating new data structures instead of modifying existing ones.
- Explore higher-order functions: Learn to use functions like map, filter, and reduce.
- Study recursion: Practice solving problems recursively instead of using loops.
- Experiment with a functional language: Try out a language designed for functional programming, like Haskell or Clojure.
Challenges and Considerations
While functional programming offers many benefits, it also comes with some challenges:
- Learning curve: The paradigm shift can be challenging for developers accustomed to imperative or object-oriented programming.
- Performance overhead: Creating new data structures instead of modifying existing ones can lead to increased memory usage and potential performance impacts.
- Limited libraries: Some functional programming languages have smaller ecosystems compared to mainstream languages.
- Integration with existing systems: It can be challenging to integrate functional programming concepts into existing codebases or systems built with different paradigms.
Real-World Applications of Functional Programming
Functional programming is used in various domains and industries:
- Financial systems: For modeling complex financial instruments and risk analysis
- Big Data processing: Frameworks like Apache Spark use functional concepts for distributed data processing
- Web development: React, a popular JavaScript library, incorporates functional programming principles
- Artificial Intelligence: Functional programming is used in some AI and machine learning applications
- Telecommunications: Erlang, a functional language, is widely used in telecom systems
Conclusion
Functional programming offers a powerful and elegant approach to solving complex programming problems. Its emphasis on pure functions, immutability, and declarative code leads to more predictable, testable, and maintainable software. While it may require a shift in thinking for developers accustomed to other paradigms, the benefits of functional programming make it a valuable addition to any programmer’s toolkit.
As you continue your journey in software development, consider exploring functional programming concepts and how they can be applied to your projects. Whether you’re working on web applications, data processing systems, or complex algorithms, functional programming principles can help you write cleaner, more robust code.
Remember, the goal is not necessarily to replace other programming paradigms entirely, but to understand when and how to apply functional concepts to solve problems more effectively. By adding functional programming to your skill set, you’ll be better equipped to choose the right tool for each programming challenge you encounter.