Introduction to Event-Driven Programming: A Comprehensive Guide
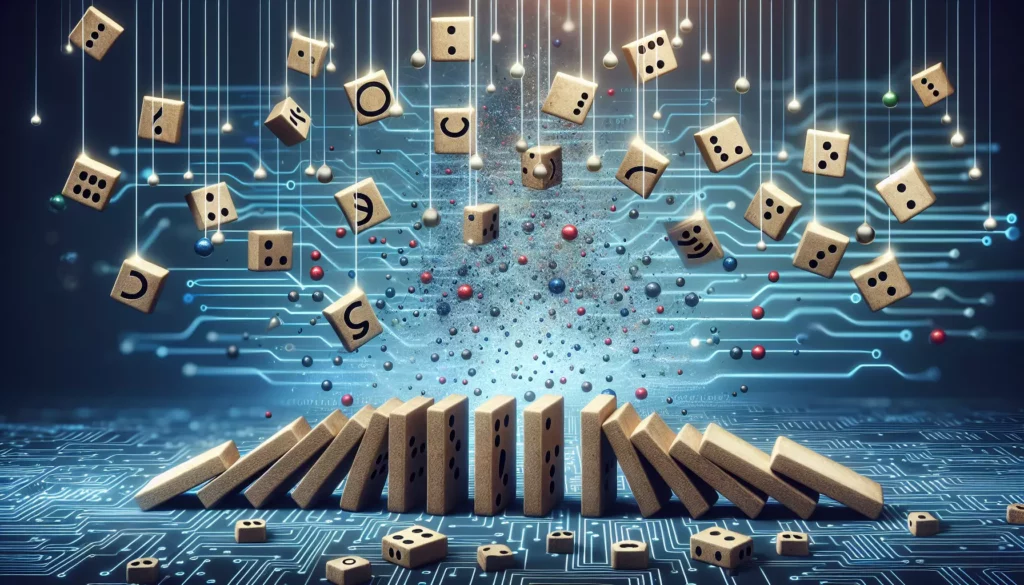
In the ever-evolving landscape of software development, event-driven programming has emerged as a powerful paradigm that revolutionizes how we design and build applications. This approach, centered around the concept of events and their handlers, offers a flexible and efficient way to create responsive and scalable systems. In this comprehensive guide, we’ll dive deep into the world of event-driven programming, exploring its core principles, benefits, and practical applications.
What is Event-Driven Programming?
Event-driven programming is a programming paradigm in which the flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs or threads. In this model, the program responds to events as they occur, rather than following a predetermined sequence of instructions.
At its core, event-driven programming revolves around two main components:
- Events: These are occurrences or changes in the system state that require some action or response.
- Event Handlers: These are functions or methods that are executed in response to specific events.
The event-driven approach is particularly well-suited for applications with graphical user interfaces, web applications, and systems that need to handle asynchronous operations efficiently.
Key Concepts in Event-Driven Programming
1. Event Loop
The event loop is a central concept in event-driven programming. It’s a continuous process that waits for and dispatches events or messages in a program. The event loop constantly checks for new events and calls the appropriate event handlers when events occur.
Here’s a simplified representation of an event loop in pseudocode:
while (program_is_running) {
event = wait_for_event()
if (event != null) {
process_event(event)
}
}
2. Event Emitters and Listeners
In many event-driven systems, there are objects or components that emit events (event emitters) and others that listen for and respond to these events (event listeners). This pattern allows for loose coupling between different parts of an application, as the emitter doesn’t need to know about the listeners, and vice versa.
3. Asynchronous Programming
Event-driven programming often goes hand in hand with asynchronous programming. This allows the program to continue executing other tasks while waiting for long-running operations (like I/O operations) to complete, rather than blocking the entire program.
4. Callback Functions
Callback functions are a fundamental concept in event-driven programming. These are functions passed as arguments to other functions and are executed when a specific event occurs or when an asynchronous operation completes.
Benefits of Event-Driven Programming
Event-driven programming offers several advantages that make it an attractive choice for many types of applications:
- Improved Responsiveness: By responding to events as they occur, applications can provide a more responsive user experience, particularly in GUI applications.
- Scalability: Event-driven architectures can handle a large number of concurrent operations efficiently, making them well-suited for scalable systems.
- Loose Coupling: Components in an event-driven system are often loosely coupled, which can lead to more modular and maintainable code.
- Flexibility: It’s easier to add new features or modify existing ones in an event-driven system without affecting other parts of the application.
- Asynchronous Processing: Event-driven programming naturally supports asynchronous operations, which can improve performance in I/O-bound applications.
Event-Driven Programming in Different Languages
Many modern programming languages and frameworks support event-driven programming. Let’s look at how event-driven concepts are implemented in some popular languages:
JavaScript
JavaScript, particularly in the context of web development, is inherently event-driven. The DOM (Document Object Model) in web browsers provides a rich set of events that developers can listen for and respond to.
Here’s a simple example of event handling in JavaScript:
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
In this example, we’re adding an event listener to a button element. When the button is clicked, the provided callback function is executed, displaying an alert.
Python
While Python is not inherently event-driven, it provides libraries and frameworks that support event-driven programming. For instance, the `asyncio` module in Python 3 provides tools for writing concurrent code using the async/await syntax.
Here’s a simple example using `asyncio`:
import asyncio
async def main():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(main())
In this example, the `asyncio.sleep()` function simulates an asynchronous operation. The `await` keyword allows the program to continue executing other tasks while waiting for the sleep operation to complete.
Java
Java provides robust support for event-driven programming, particularly through its Swing and JavaFX libraries for building graphical user interfaces.
Here’s a simple example using Swing:
import javax.swing.*;
import java.awt.event.*;
public class ButtonExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Example");
JButton button = new JButton("Click Me");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
});
frame.add(button);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
In this example, we create a button and add an action listener to it. When the button is clicked, the `actionPerformed` method is called, printing a message to the console.
Event-Driven Architectures
Event-driven programming principles can be extended to entire system architectures, leading to what’s known as Event-Driven Architecture (EDA). In an EDA, the flow of the system is determined by events moving through an event-driven pipeline.
Key components of an Event-Driven Architecture include:
- Event Producers: Components that generate events.
- Event Consumers: Components that receive and process events.
- Event Channels: The medium through which events are transmitted from producers to consumers.
- Event Processing Engine: A system that manages the flow of events and invokes the appropriate handlers.
Event-Driven Architectures are particularly useful in scenarios such as:
- Microservices architectures
- Real-time data processing systems
- Internet of Things (IoT) applications
- Complex event processing systems
Challenges and Considerations
While event-driven programming offers many benefits, it also comes with its own set of challenges:
- Complexity: Event-driven systems can become complex, especially when dealing with many interconnected events and handlers.
- Debugging: Debugging event-driven code can be more challenging than traditional procedural code, as the flow of execution is not always linear.
- Race Conditions: In concurrent systems, event-driven programming can lead to race conditions if not carefully managed.
- Testing: Testing event-driven systems can be more complex, as it often requires simulating various event scenarios.
- Error Handling: Proper error handling in event-driven systems requires careful consideration to ensure that errors in one event handler don’t negatively impact the entire system.
Best Practices for Event-Driven Programming
To make the most of event-driven programming and mitigate its challenges, consider the following best practices:
- Keep Event Handlers Small and Focused: Each event handler should have a single responsibility. This makes the code easier to understand and maintain.
- Use Event Delegation: Instead of attaching event listeners to multiple elements, use event delegation to attach a single listener to a parent element.
- Avoid Tight Coupling: Design your system so that event producers and consumers are loosely coupled. This improves flexibility and maintainability.
- Implement Proper Error Handling: Ensure that errors in event handlers are caught and handled appropriately to prevent system-wide failures.
- Use Asynchronous Operations Wisely: Leverage asynchronous operations for long-running tasks to keep your application responsive.
- Document Event Flows: Clearly document the events in your system, including their producers, consumers, and expected behaviors.
- Implement Logging and Monitoring: Robust logging and monitoring are crucial for debugging and maintaining event-driven systems.
Tools and Frameworks for Event-Driven Programming
Several tools and frameworks can help you implement event-driven systems more effectively:
- RxJS: A library for reactive programming using Observables, making it easier to compose asynchronous or callback-based code.
- Node.js: A JavaScript runtime built on Chrome’s V8 JavaScript engine, designed for building scalable network applications.
- Apache Kafka: A distributed streaming platform that allows you to build real-time streaming data pipelines and applications.
- Spring Framework: Provides comprehensive infrastructure support for developing Java applications, including support for event-driven programming.
- Akka: A toolkit for building highly concurrent, distributed, and resilient message-driven applications for Java and Scala.
Real-World Applications of Event-Driven Programming
Event-driven programming finds applications in various domains:
- Graphical User Interfaces: Most modern GUI frameworks use event-driven programming to handle user interactions.
- Web Applications: Event-driven programming is fundamental to creating interactive web applications, especially with frameworks like React and Vue.js.
- Real-time Data Processing: Systems that need to process large volumes of data in real-time often use event-driven architectures.
- Internet of Things (IoT): IoT devices typically operate on an event-driven model, responding to sensor data and user interactions.
- Game Development: Many game engines use event-driven programming to handle user inputs, collisions, and other game events.
- Financial Systems: Trading systems and other financial applications often use event-driven architectures to respond quickly to market changes.
Future Trends in Event-Driven Programming
As technology continues to evolve, we can expect to see several trends in event-driven programming:
- Serverless Computing: Event-driven programming is a natural fit for serverless architectures, where functions are triggered by events.
- Edge Computing: As computing moves closer to the data source, event-driven systems will play a crucial role in processing data at the edge.
- AI and Machine Learning Integration: Event-driven systems will increasingly incorporate AI and ML models to process and respond to events intelligently.
- Blockchain and Distributed Ledger Technologies: These technologies often use event-driven models to manage transactions and state changes.
- 5G and Beyond: The increased connectivity and low latency of 5G networks will enable more sophisticated real-time, event-driven applications.
Conclusion
Event-driven programming has become an essential paradigm in modern software development, offering a powerful approach to building responsive, scalable, and maintainable applications. By understanding the core principles of event-driven programming and following best practices, developers can harness its full potential to create robust and efficient systems.
As we’ve explored in this guide, event-driven programming is not just a theoretical concept but a practical approach with wide-ranging applications across various domains. From web development to IoT, from financial systems to game development, event-driven programming continues to shape the way we design and build software.
As you continue your journey in software development, mastering event-driven programming will undoubtedly be a valuable skill. It will enable you to create more responsive applications, handle complex asynchronous operations with ease, and build systems that can scale to meet the demands of modern computing environments.
Remember, like any programming paradigm, event-driven programming comes with its own set of challenges. But with careful design, proper error handling, and a solid understanding of the underlying principles, you can leverage its power to create truly impressive and efficient applications.
As technology continues to evolve, event-driven programming will likely play an even more significant role in shaping the future of software development. By staying informed about the latest trends and continuously honing your skills in this area, you’ll be well-prepared to tackle the exciting challenges that lie ahead in the world of programming.