Introduction to Databases: SQL Basics for Programmers
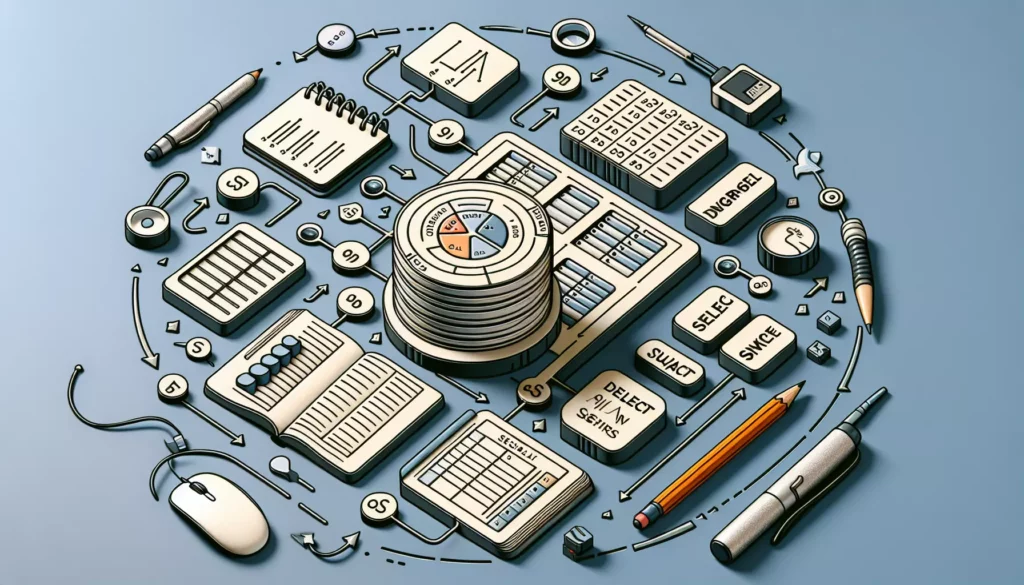
In today’s data-driven world, understanding databases and how to interact with them is an essential skill for programmers. Whether you’re building web applications, mobile apps, or working on data analysis projects, you’ll likely encounter databases at some point in your career. This comprehensive guide will introduce you to the fundamentals of databases, with a focus on SQL (Structured Query Language), the most widely used language for managing relational databases.
What is a Database?
A database is an organized collection of data stored and accessed electronically. It allows for efficient storage, retrieval, and manipulation of data. Databases come in various types, but the most common for application development are relational databases, which organize data into tables with rows and columns.
Key Database Concepts
- Table: A collection of related data organized in rows and columns.
- Row: Also known as a record or tuple, represents a single, implicitly structured data item in a table.
- Column: Also called a field or attribute, defines the data type and constraints for a specific piece of information in each row.
- Primary Key: A unique identifier for each row in a table.
- Foreign Key: A field in one table that refers to the primary key in another table, establishing a relationship between tables.
Introduction to SQL
SQL (Structured Query Language) is the standard language for interacting with relational databases. It allows you to create, read, update, and delete data in a database. SQL is used in various database management systems (DBMS) such as MySQL, PostgreSQL, SQLite, Oracle, and Microsoft SQL Server.
Basic SQL Commands
Let’s dive into some fundamental SQL commands that every programmer should know:
1. CREATE TABLE
The CREATE TABLE command is used to create a new table in the database. Here’s an example:
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
This command creates a table named “users” with four columns: id, username, email, and created_at. The PRIMARY KEY, NOT NULL, UNIQUE, and DEFAULT constraints are used to enforce data integrity.
2. INSERT INTO
The INSERT INTO command is used to add new rows of data to a table:
INSERT INTO users (id, username, email)
VALUES (1, 'johndoe', 'john@example.com');
This command inserts a new user into the “users” table with the specified values.
3. SELECT
The SELECT command is used to retrieve data from one or more tables:
SELECT * FROM users;
This command retrieves all columns and rows from the “users” table. You can also specify particular columns:
SELECT username, email FROM users;
4. WHERE Clause
The WHERE clause is used to filter results based on specific conditions:
SELECT * FROM users
WHERE username = 'johndoe';
This command retrieves all columns for the user with the username ‘johndoe’.
5. UPDATE
The UPDATE command is used to modify existing data in a table:
UPDATE users
SET email = 'newemail@example.com'
WHERE id = 1;
This command updates the email address for the user with id 1.
6. DELETE
The DELETE command is used to remove rows from a table:
DELETE FROM users
WHERE id = 1;
This command deletes the user with id 1 from the “users” table.
Advanced SQL Concepts
As you become more comfortable with basic SQL commands, you’ll want to explore more advanced concepts to enhance your database skills:
1. Joins
Joins are used to combine rows from two or more tables based on a related column between them. There are several types of joins:
- INNER JOIN
- LEFT JOIN (or LEFT OUTER JOIN)
- RIGHT JOIN (or RIGHT OUTER JOIN)
- FULL JOIN (or FULL OUTER JOIN)
Here’s an example of an INNER JOIN:
SELECT users.username, orders.order_date
FROM users
INNER JOIN orders ON users.id = orders.user_id;
This query retrieves the username and order date for all users who have placed orders.
2. Aggregate Functions
Aggregate functions perform calculations on a set of values and return a single result. Common aggregate functions include:
- COUNT()
- SUM()
- AVG()
- MAX()
- MIN()
Example usage:
SELECT COUNT(*) AS total_users FROM users;
This query returns the total number of users in the “users” table.
3. GROUP BY and HAVING
The GROUP BY clause is used to group rows that have the same values in specified columns. It’s often used with aggregate functions. The HAVING clause is used to specify conditions for the grouped results.
SELECT country, COUNT(*) AS user_count
FROM users
GROUP BY country
HAVING user_count > 100;
This query groups users by country and returns only the countries with more than 100 users.
4. Subqueries
A subquery is a query nested inside another query. It can be used in various parts of an SQL statement, such as the SELECT, FROM, or WHERE clauses.
SELECT username
FROM users
WHERE id IN (SELECT user_id FROM orders WHERE total > 1000);
This query selects usernames of users who have placed orders with a total value greater than 1000.
Database Design Principles
Understanding SQL is crucial, but it’s equally important to design your database properly. Here are some key principles to keep in mind:
1. Normalization
Normalization is the process of organizing data to reduce redundancy and improve data integrity. The most common forms of normalization are:
- First Normal Form (1NF)
- Second Normal Form (2NF)
- Third Normal Form (3NF)
Each form has specific rules that help structure your data efficiently.
2. Indexing
Indexes are used to speed up data retrieval operations on a database table. While they can significantly improve query performance, they also have overhead for insert, update, and delete operations. Use indexes judiciously on columns that are frequently used in WHERE clauses or JOIN conditions.
CREATE INDEX idx_username ON users(username);
This command creates an index on the username column of the users table.
3. Transactions
Transactions ensure that a series of SQL statements are executed as a single unit of work. They adhere to the ACID properties:
- Atomicity: All operations in a transaction succeed or they all fail.
- Consistency: The database remains in a consistent state before and after the transaction.
- Isolation: Concurrent transactions do not interfere with each other.
- Durability: Once a transaction is committed, its effects are permanent.
Here’s an example of a transaction in SQL:
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - 100 WHERE id = 1;
UPDATE accounts SET balance = balance + 100 WHERE id = 2;
COMMIT;
This transaction transfers 100 units from account 1 to account 2. If any part of the transaction fails, the entire operation is rolled back.
Database Management Systems (DBMS)
While SQL is a standard language, different database management systems may have slight variations in syntax and features. Here are some popular DBMS you might encounter:
1. MySQL
MySQL is an open-source relational database management system. It’s widely used for web applications and is a component of the popular LAMP (Linux, Apache, MySQL, PHP/Python/Perl) stack.
2. PostgreSQL
PostgreSQL, often called Postgres, is an advanced, open-source object-relational database system. It’s known for its robust feature set, including support for JSON, full-text search, and geospatial data.
3. SQLite
SQLite is a lightweight, serverless, and self-contained relational database engine. It’s often used in mobile apps and desktop applications where a full-fledged database server isn’t necessary.
4. Microsoft SQL Server
SQL Server is a relational database management system developed by Microsoft. It’s commonly used in enterprise environments and integrates well with other Microsoft technologies.
5. Oracle Database
Oracle Database is a multi-model database management system produced and marketed by Oracle Corporation. It’s known for its reliability and scalability, often used in large enterprise applications.
Best Practices for Working with Databases
As you start working with databases in your projects, keep these best practices in mind:
1. Use Prepared Statements
Prepared statements help prevent SQL injection attacks by separating SQL logic from data. Most programming languages and database libraries support prepared statements. Here’s an example in Python using the sqlite3 module:
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
username = 'johndoe'
cursor.execute('SELECT * FROM users WHERE username = ?', (username,))
result = cursor.fetchone()
print(result)
conn.close()
2. Implement Proper Error Handling
Always implement error handling when working with databases. This includes catching and logging database exceptions, and gracefully handling connection issues.
3. Use Connection Pooling
For applications that require frequent database connections, use connection pooling to improve performance. Connection pooling maintains a cache of database connections that can be reused, reducing the overhead of creating new connections.
4. Regularly Backup Your Database
Implement a robust backup strategy to protect against data loss. This may include regular full backups and incremental backups, depending on your needs.
5. Monitor Database Performance
Use database monitoring tools to keep track of query performance, resource usage, and potential bottlenecks. This can help you optimize your database and queries over time.
Conclusion
Understanding databases and SQL is a fundamental skill for any programmer. As you’ve seen in this introduction, SQL provides powerful tools for managing and querying data. From basic CRUD (Create, Read, Update, Delete) operations to more advanced concepts like joins and transactions, mastering SQL will enable you to build more efficient and robust applications.
Remember, this guide is just the beginning. As you continue your journey in software development, you’ll encounter more complex database scenarios and may need to dive deeper into specific database technologies. Keep practicing, explore real-world projects, and don’t hesitate to consult official documentation for the database system you’re using.
Databases are at the heart of most modern applications, and your proficiency in working with them will be a valuable asset throughout your programming career. Whether you’re preparing for technical interviews at top tech companies or building your own projects, a solid understanding of databases and SQL will serve you well.
Happy coding, and may your queries always return the results you expect!