Introduction to Data Structures: Linked Lists, Stacks, and Queues
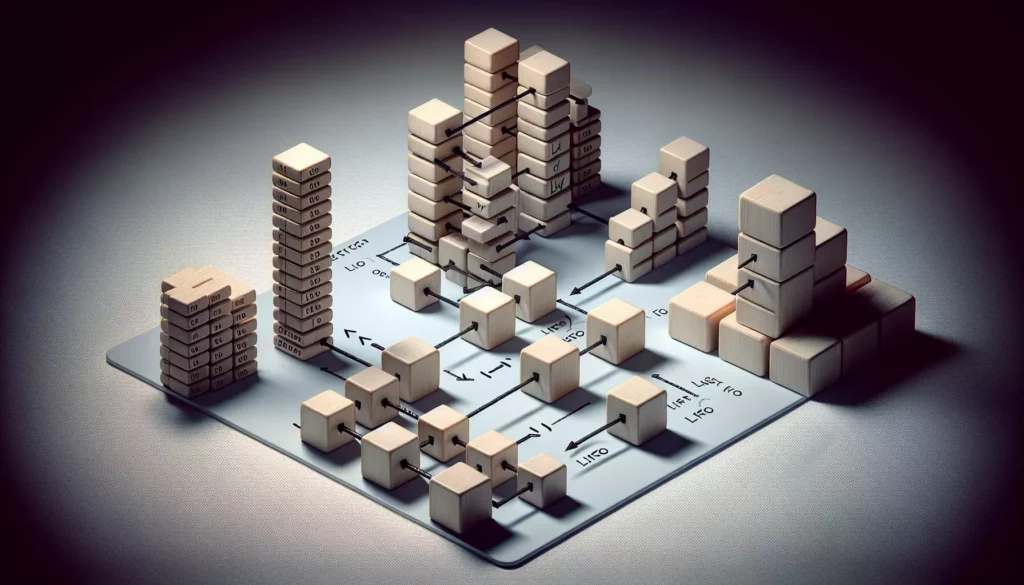
Data structures are fundamental concepts in computer science and programming that allow us to organize and store data efficiently. They play a crucial role in solving complex problems and optimizing algorithms. In this comprehensive guide, we’ll explore three essential data structures: linked lists, stacks, and queues. Understanding these structures is vital for anyone looking to enhance their coding skills, tackle technical interviews, or build efficient software systems.
1. Linked Lists
A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence.
1.1 Types of Linked Lists
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has pointers to both the next and previous nodes.
- Circular Linked List: The last node points back to the first node, creating a circle.
1.2 Implementing a Singly Linked List in Python
Let’s implement a basic singly linked list in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def display(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.display() # Output: 1 -> 2 -> 3 -> None
1.3 Advantages and Disadvantages of Linked Lists
Advantages:
- Dynamic size (can grow or shrink easily)
- Efficient insertion and deletion at any position
- No memory wastage (allocates memory as needed)
Disadvantages:
- Random access is not allowed (must traverse from the beginning)
- Extra memory for storing pointers
- Not cache-friendly due to non-contiguous memory allocation
2. Stacks
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. Think of it as a stack of plates – you can only add or remove plates from the top.
2.1 Key Operations
- Push: Add an element to the top of the stack
- Pop: Remove the top element from the stack
- Peek or Top: View the top element without removing it
- IsEmpty: Check if the stack is empty
2.2 Implementing a Stack in Python
We can implement a stack using a Python list:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
2.3 Applications of Stacks
- Function call management (call stack)
- Undo mechanisms in text editors
- Expression evaluation and syntax parsing
- Backtracking algorithms
3. Queues
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. It’s similar to a line of people waiting for a service – the first person to join the line is the first to be served.
3.1 Key Operations
- Enqueue: Add an element to the rear of the queue
- Dequeue: Remove an element from the front of the queue
- Front: Get the front element without removing it
- IsEmpty: Check if the queue is empty
3.2 Implementing a Queue in Python
Let’s implement a simple queue using Python’s collections.deque for efficient operations:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
print(queue.size()) # Output: 2
3.3 Applications of Queues
- Task scheduling in operating systems
- Breadth-First Search algorithm
- Print job scheduling
- Handling of requests on a single shared resource (e.g., printer, CPU)
4. Comparing Linked Lists, Stacks, and Queues
Now that we’ve explored these three data structures, let’s compare their characteristics and use cases:
Feature | Linked List | Stack | Queue |
---|---|---|---|
Access Pattern | Sequential | LIFO | FIFO |
Insertion | O(1) at head, O(n) elsewhere | O(1) at top | O(1) at rear |
Deletion | O(1) at head, O(n) elsewhere | O(1) from top | O(1) from front |
Search | O(n) | O(n) | O(n) |
Memory Usage | Dynamic | Can be fixed or dynamic | Can be fixed or dynamic |
5. Real-world Applications and Interview Questions
Understanding these data structures is crucial for solving various programming problems and excelling in technical interviews. Here are some common applications and interview questions related to linked lists, stacks, and queues:
5.1 Linked List Applications and Questions
- Implementing polynomial arithmetic
- Music player playlists
- Hash tables (for handling collisions)
Common interview questions:
- Reverse a linked list
- Detect a cycle in a linked list
- Find the middle element of a linked list
- Merge two sorted linked lists
5.2 Stack Applications and Questions
- Browser history (back button functionality)
- Undo/Redo operations in text editors
- Expression evaluation (e.g., postfix notation)
Common interview questions:
- Implement a min stack (a stack that supports push, pop, top, and retrieving the minimum element in constant time)
- Evaluate postfix expressions
- Check for balanced parentheses in an expression
- Implement a queue using two stacks
5.3 Queue Applications and Questions
- Job scheduling in operating systems
- Buffering for devices like keyboards
- Breadth-First Search in graphs
Common interview questions:
- Implement a stack using two queues
- Reverse the first K elements of a queue
- Generate binary numbers from 1 to n using a queue
- Implement a circular queue
6. Advanced Concepts and Variations
As you progress in your understanding of these data structures, you’ll encounter more advanced concepts and variations:
6.1 Advanced Linked List Concepts
- Skip Lists: A probabilistic data structure that allows for faster search within an ordered sequence of elements.
- XOR Linked Lists: A memory-efficient variation where each node contains the XOR of the addresses of previous and next nodes.
- Self-organizing lists: Lists that reorder themselves based on access frequency to improve average access time.
6.2 Advanced Stack Concepts
- Monotonic Stack: A stack that maintains elements in a strictly increasing or decreasing order.
- Stack with increment operation: A stack that supports an operation to increment elements in a range.
- Stack using heap: Implementing a stack with additional operations like finding the maximum element efficiently.
6.3 Advanced Queue Concepts
- Priority Queue: A queue where elements have associated priorities and are dequeued based on these priorities.
- Double-ended Queue (Deque): A queue that allows insertion and deletion at both ends.
- Circular Buffer: A fixed-size queue implemented in a circular array, useful for streaming data processing.
7. Implementing These Data Structures in Different Programming Languages
While we’ve focused on Python implementations, it’s valuable to understand how these data structures can be implemented in other popular programming languages. Let’s look at brief examples in Java and C++:
7.1 Linked List in Java
public class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
public class LinkedList {
Node head;
public void append(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
public void display() {
Node current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
}
7.2 Stack in C++
#include <vector>
class Stack {
private:
std::vector<int> items;
public:
void push(int item) {
items.push_back(item);
}
int pop() {
if (!isEmpty()) {
int top = items.back();
items.pop_back();
return top;
}
return -1; // Or throw an exception
}
int peek() {
if (!isEmpty()) {
return items.back();
}
return -1; // Or throw an exception
}
bool isEmpty() {
return items.empty();
}
int size() {
return items.size();
}
};
7.3 Queue in Java
import java.util.LinkedList;
public class Queue<T> {
private LinkedList<T> list = new LinkedList<>();
public void enqueue(T item) {
list.addLast(item);
}
public T dequeue() {
if (!isEmpty()) {
return list.removeFirst();
}
return null; // Or throw an exception
}
public T front() {
if (!isEmpty()) {
return list.getFirst();
}
return null; // Or throw an exception
}
public boolean isEmpty() {
return list.isEmpty();
}
public int size() {
return list.size();
}
}
8. Time and Space Complexity Analysis
Understanding the time and space complexity of operations on these data structures is crucial for efficient algorithm design and optimization. Let’s analyze the complexity of common operations:
8.1 Linked List
- Access: O(n) – We may need to traverse the entire list to find an element
- Insertion at head: O(1)
- Insertion at tail: O(n) for singly linked list, O(1) for doubly linked list with tail pointer
- Deletion at head: O(1)
- Deletion at tail: O(n) for singly linked list, O(1) for doubly linked list
- Search: O(n)
- Space complexity: O(n)
8.2 Stack
- Push: O(1)
- Pop: O(1)
- Peek: O(1)
- Search: O(n)
- Space complexity: O(n)
8.3 Queue
- Enqueue: O(1)
- Dequeue: O(1)
- Front: O(1)
- Search: O(n)
- Space complexity: O(n)
9. Best Practices and Common Pitfalls
When working with these data structures, keep the following best practices and common pitfalls in mind:
9.1 Best Practices
- Always check for empty structures before performing operations
- Use appropriate data structures for the problem at hand
- Consider using built-in implementations when available for better performance and reliability
- Implement proper error handling and boundary checks
- Document your code and include comments for complex operations
9.2 Common Pitfalls
- Forgetting to update pointers correctly in linked lists, leading to memory leaks or incorrect connections
- Neglecting to handle edge cases, such as empty structures or single-element structures
- Incorrect implementation of circular structures, causing infinite loops
- Misusing stacks or queues in situations where the other would be more appropriate
- Inefficient implementations that don’t take advantage of the data structure’s properties
10. Conclusion
Linked lists, stacks, and queues are fundamental data structures that form the building blocks of many complex algorithms and systems. Mastering these concepts is essential for any aspiring programmer or computer scientist. They not only help in solving a wide range of problems efficiently but also provide a strong foundation for understanding more advanced data structures and algorithms.
As you continue your journey in programming and computer science, remember that practice is key. Implement these data structures from scratch, solve problems using them, and explore their variations and applications. This hands-on experience will deepen your understanding and prepare you for technical interviews and real-world programming challenges.
Keep exploring, keep coding, and don’t hesitate to dive into more advanced topics as you grow more comfortable with these foundational concepts. Happy coding!