Introduction to Data Encryption Standards and Practices
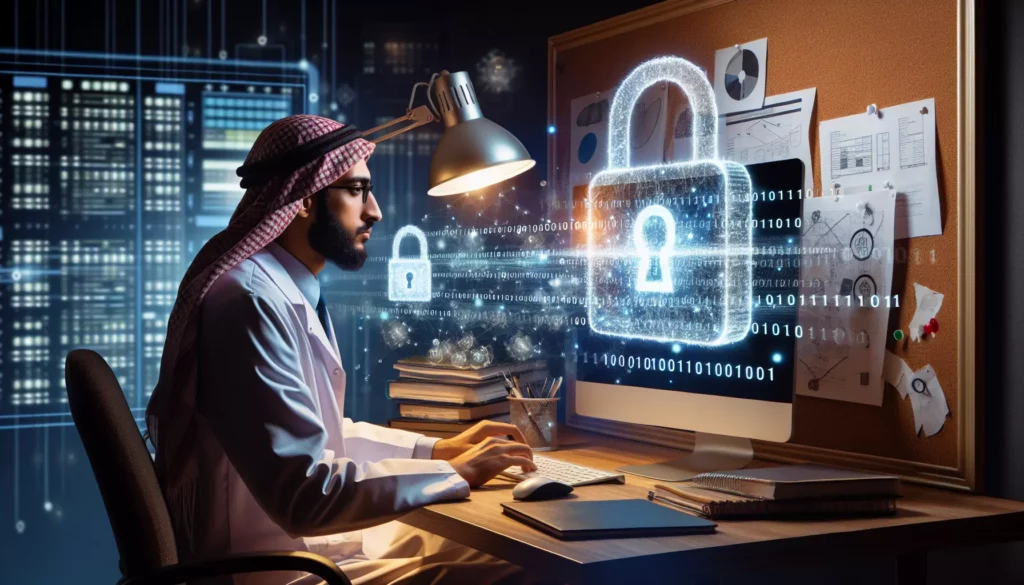
In today’s digital age, where data breaches and cyber threats are increasingly common, understanding data encryption has become crucial for programmers and tech professionals. This comprehensive guide will introduce you to the fundamental concepts of data encryption, explore various encryption standards, and discuss best practices for implementing secure encryption in your projects.
Table of Contents
- What is Encryption?
- The Importance of Encryption
- Types of Encryption
- Common Encryption Algorithms
- Encryption Standards and Protocols
- Best Practices for Implementing Encryption
- Challenges and Considerations in Encryption
- The Future of Encryption
- Conclusion
1. What is Encryption?
Encryption is the process of converting information or data into a code to prevent unauthorized access. It’s a fundamental aspect of information security that protects the confidentiality of digital data. When data is encrypted, it becomes scrambled and unreadable to anyone who doesn’t have the decryption key.
The basic workflow of encryption involves:
- Taking plaintext (the original, readable data)
- Applying an encryption algorithm along with an encryption key
- Producing ciphertext (the encrypted, unreadable data)
To retrieve the original information, the reverse process, known as decryption, is applied using the appropriate decryption key.
2. The Importance of Encryption
Encryption plays a vital role in protecting sensitive information in various contexts:
- Data Privacy: Encryption ensures that personal and sensitive data remains confidential, even if it falls into the wrong hands.
- Secure Communications: It enables secure transmission of data over networks, protecting against eavesdropping and man-in-the-middle attacks.
- Regulatory Compliance: Many industries require encryption to comply with data protection regulations like GDPR, HIPAA, or PCI-DSS.
- Intellectual Property Protection: Encryption helps safeguard valuable intellectual property and trade secrets.
- Authentication: It can be used to verify the identity of users and the integrity of data.
3. Types of Encryption
There are two main types of encryption:
3.1 Symmetric Encryption
Symmetric encryption, also known as secret-key encryption, uses the same key for both encryption and decryption. It’s fast and efficient, making it suitable for encrypting large amounts of data. However, the main challenge lies in securely sharing the secret key between parties.
Examples of symmetric encryption algorithms include:
- AES (Advanced Encryption Standard)
- DES (Data Encryption Standard)
- 3DES (Triple DES)
- Blowfish
3.2 Asymmetric Encryption
Asymmetric encryption, also called public-key cryptography, uses a pair of keys: a public key for encryption and a private key for decryption. The public key can be freely shared, while the private key must be kept secret. This approach solves the key distribution problem of symmetric encryption but is generally slower and more computationally intensive.
Examples of asymmetric encryption algorithms include:
- RSA (Rivest-Shamir-Adleman)
- ECC (Elliptic Curve Cryptography)
- Diffie-Hellman
- DSA (Digital Signature Algorithm)
4. Common Encryption Algorithms
Let’s dive deeper into some of the most widely used encryption algorithms:
4.1 AES (Advanced Encryption Standard)
AES is a symmetric block cipher that has become the de facto standard for encryption. It supports key sizes of 128, 192, and 256 bits, with AES-256 being the most secure. AES is widely used for securing sensitive data in various applications, from file encryption to secure communications.
Here’s a simple example of using AES encryption in Python with the PyCryptodome library:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_aes(plaintext, key):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext.encode())
return (cipher.nonce, tag, ciphertext)
# Generate a random 256-bit key
key = get_random_bytes(32)
# Encrypt a message
message = "Hello, World!"
encrypted = encrypt_aes(message, key)
print(f"Encrypted: {encrypted}")
4.2 RSA (Rivest-Shamir-Adleman)
RSA is one of the first practical asymmetric cryptosystems and is widely used for secure data transmission. It is based on the computational difficulty of factoring large integers. RSA is commonly used in digital signatures and key exchange protocols.
Here’s a basic example of RSA encryption using Python’s cryptography library:
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import hashes
def generate_rsa_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
return private_key, public_key
def encrypt_rsa(message, public_key):
ciphertext = public_key.encrypt(
message.encode(),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return ciphertext
# Generate RSA key pair
private_key, public_key = generate_rsa_keys()
# Encrypt a message
message = "Hello, RSA!"
encrypted = encrypt_rsa(message, public_key)
print(f"Encrypted: {encrypted}")
4.3 ECC (Elliptic Curve Cryptography)
ECC is an approach to asymmetric cryptography based on the algebraic structure of elliptic curves over finite fields. It offers equivalent security to RSA with smaller key sizes, making it more efficient for mobile and low-power devices.
5. Encryption Standards and Protocols
Several encryption standards and protocols have been developed to ensure secure communication and data protection:
5.1 SSL/TLS (Secure Sockets Layer/Transport Layer Security)
SSL and its successor TLS are cryptographic protocols designed to provide secure communication over a computer network. They are widely used for securing web browsing (HTTPS), email, instant messaging, and voice over IP (VoIP).
5.2 PGP (Pretty Good Privacy)
PGP is an encryption program that provides cryptographic privacy and authentication for data communication. It is often used for signing, encrypting, and decrypting texts, emails, files, directories, and whole disk partitions.
5.3 IPsec (Internet Protocol Security)
IPsec is a protocol suite for securing Internet Protocol (IP) communications by authenticating and encrypting each IP packet of a communication session. It is widely used in Virtual Private Networks (VPNs).
6. Best Practices for Implementing Encryption
When implementing encryption in your projects, consider the following best practices:
- Use Strong Algorithms: Always use well-established, publicly scrutinized encryption algorithms like AES, RSA, or ECC.
- Proper Key Management: Securely generate, store, and manage encryption keys. Never hardcode keys in your source code.
- Implement Perfect Forward Secrecy: Use techniques that ensure past communications cannot be decrypted if long-term keys are compromised.
- Keep Software Updated: Regularly update your encryption libraries and software to patch known vulnerabilities.
- Use Appropriate Key Sizes: Choose key sizes that provide adequate security. For example, use at least 2048-bit keys for RSA and 256-bit keys for AES.
- Secure Key Exchange: When using symmetric encryption, ensure that the initial key exchange is done securely, preferably using asymmetric encryption.
- Implement Proper Authentication: Combine encryption with strong authentication mechanisms to verify the identity of communicating parties.
- Use Secure Random Number Generators: Employ cryptographically secure random number generators for creating keys and initialization vectors.
Here’s an example of generating a secure random key in Python:
import secrets
def generate_secure_key(key_size=32):
return secrets.token_bytes(key_size)
# Generate a 256-bit (32-byte) key
secure_key = generate_secure_key()
print(f"Secure Key: {secure_key.hex()}")
7. Challenges and Considerations in Encryption
While encryption is crucial for data security, it also presents several challenges:
7.1 Performance Overhead
Encryption and decryption operations can introduce significant computational overhead, especially for large datasets or real-time applications. Balancing security with performance is often a key consideration.
7.2 Key Management
Proper key management is critical but challenging. It involves secure key generation, storage, distribution, and rotation. Poor key management can undermine even the strongest encryption algorithms.
7.3 Quantum Computing Threat
The advent of quantum computing poses a potential threat to many current encryption methods, particularly asymmetric algorithms like RSA. This has led to research in post-quantum cryptography.
7.4 Legal and Ethical Considerations
The use of encryption can sometimes conflict with legal requirements, such as lawful interception. There’s an ongoing debate about balancing privacy rights with national security concerns.
8. The Future of Encryption
As technology evolves, so does the field of encryption. Here are some trends shaping the future of encryption:
8.1 Homomorphic Encryption
Homomorphic encryption allows computations to be performed on encrypted data without decrypting it. This has significant implications for cloud computing and data privacy.
8.2 Quantum Cryptography
Quantum cryptography leverages the principles of quantum mechanics to create theoretically unbreakable encryption. Quantum Key Distribution (QKD) is one of the most promising applications in this field.
8.3 Blockchain and Decentralized Encryption
Blockchain technology is being explored for its potential in creating decentralized, tamper-resistant encryption systems.
8.4 AI and Machine Learning in Cryptography
AI and machine learning are being used to develop more robust encryption algorithms and to detect potential vulnerabilities in existing systems.
9. Conclusion
Data encryption is a cornerstone of modern information security. As a programmer or tech professional, understanding encryption standards and practices is crucial for developing secure applications and protecting sensitive data. By implementing strong encryption algorithms, following best practices, and staying informed about emerging trends, you can significantly enhance the security of your projects and contribute to a safer digital ecosystem.
Remember, encryption is an evolving field, and what’s considered secure today may not be in the future. Continuous learning and adaptation are key to staying ahead in the world of cryptography and data security.
As you continue your journey in coding and software development, consider how you can incorporate robust encryption practices into your projects. Whether you’re building a web application, a mobile app, or working with big data, the principles and techniques discussed in this guide will serve as a solid foundation for implementing secure, encrypted systems.