Introduction to Conditional Statements: The Ifs, Elses, and Elses
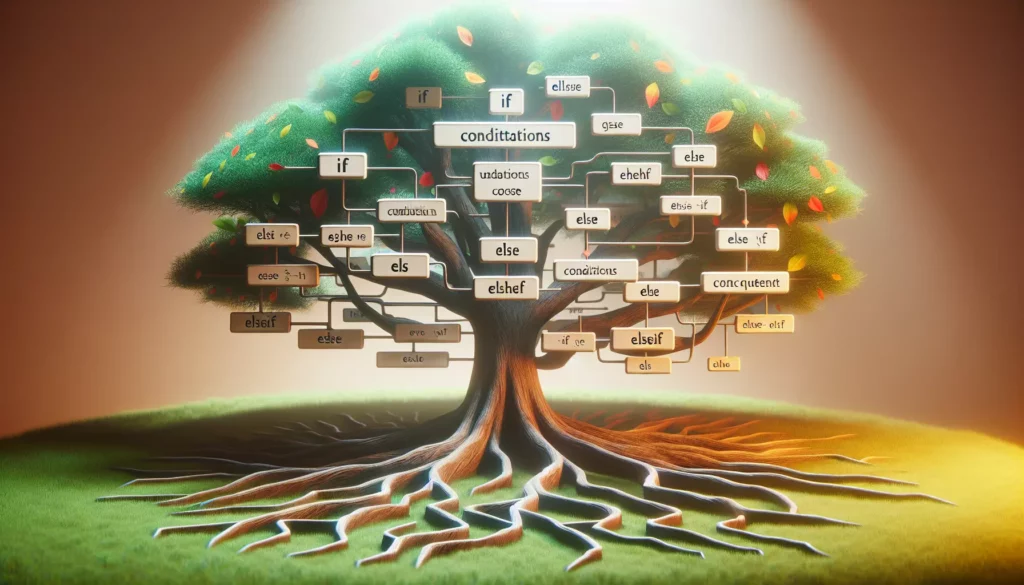
Conditional statements are the backbone of decision-making in programming. They allow your code to make choices based on specific conditions, enabling your programs to be dynamic and responsive. In this comprehensive guide, we’ll dive deep into the world of conditional statements, focusing on the fundamental constructs: if, else, and else if. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refresh your knowledge, this article will provide valuable insights into mastering conditional logic.
What Are Conditional Statements?
Conditional statements are programming constructs that allow a program to execute different code blocks based on whether a specified condition evaluates to true or false. They are essential for creating flexible and intelligent programs that can respond to various scenarios and user inputs.
The basic structure of a conditional statement typically includes:
- A condition to be evaluated
- A block of code to execute if the condition is true
- Optionally, a block of code to execute if the condition is false
The ‘if’ Statement
The ‘if’ statement is the most basic form of a conditional statement. It allows you to execute a block of code only if a specified condition is true.
Syntax:
if (condition) {
// Code to execute if the condition is true
}
Example:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
}
In this example, the message “You are eligible to vote” will be printed to the console only if the ‘age’ variable is 18 or greater.
Multiple Conditions with ‘if’
You can use logical operators to combine multiple conditions within a single ‘if’ statement:
let age = 25;
let hasID = true;
if (age >= 18 && hasID) {
console.log("You can enter the venue.");
}
This code will only execute if both conditions (age is 18 or older AND the person has an ID) are true.
The ‘else’ Statement
The ‘else’ statement is used in conjunction with ‘if’ to specify a block of code that should be executed when the ‘if’ condition is false.
Syntax:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Example:
let age = 15;
if (age >= 18) {
console.log("You are eligible to vote.");
} else {
console.log("You are not eligible to vote yet.");
}
In this case, since the age is less than 18, the second message will be printed.
The ‘else if’ Statement
The ‘else if’ statement allows you to check multiple conditions in sequence. It’s used when you have more than two possible outcomes.
Syntax:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if all conditions are false
}
Example:
let score = 75;
if (score >= 90) {
console.log("Grade: A");
} else if (score >= 80) {
console.log("Grade: B");
} else if (score >= 70) {
console.log("Grade: C");
} else {
console.log("Grade: F");
}
In this example, the program will output “Grade: C” because the score is 75, which falls into the third condition.
Nested Conditional Statements
Conditional statements can be nested within each other, allowing for more complex decision-making processes.
Example:
let age = 20;
let hasLicense = true;
if (age >= 18) {
if (hasLicense) {
console.log("You can drive a car.");
} else {
console.log("You need to get a license first.");
}
} else {
console.log("You are too young to drive.");
}
This nested structure allows for more granular control over the program’s flow based on multiple conditions.
Switch Statements: An Alternative to Multiple ‘if-else’
While not strictly part of the if-else family, switch statements provide an alternative way to handle multiple conditions, especially when comparing a single variable against multiple possible values.
Syntax:
switch (expression) {
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
// More cases...
default:
// Code to execute if no case matches
}
Example:
let day = 3;
let dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
// ... more cases ...
default:
dayName = "Invalid day";
}
console.log(dayName); // Outputs: "Wednesday"
Best Practices for Using Conditional Statements
- Keep it Simple: Try to avoid overly complex nested conditions. If your conditional logic becomes too complicated, consider breaking it down into separate functions or using switch statements.
- Use Meaningful Variable Names: Choose clear and descriptive names for your variables and conditions to make your code more readable.
- Consider the Order of Conditions: In ‘else if’ chains, put the most likely or most important conditions first for better performance and readability.
- Use Ternary Operators for Simple Conditions: For very simple if-else statements, consider using the ternary operator for more concise code.
- Avoid Redundant Conditions: If you find yourself repeating the same condition in multiple places, consider restructuring your code.
Common Pitfalls and How to Avoid Them
1. Forgetting Curly Braces
While it’s syntactically correct to omit curly braces for single-line if statements, it’s generally better to always use them to prevent errors and improve readability.
Avoid:
if (condition)
doSomething();
doSomethingElse(); // This will always execute!
Prefer:
if (condition) {
doSomething();
doSomethingElse(); // Now this only executes if the condition is true
}
2. Using ‘=’ Instead of ‘==’ or ‘===’
A common mistake is using the assignment operator (=) instead of the equality (==) or strict equality (===) operators in conditions.
Incorrect:
if (x = 5) {
// This will always be true and assign 5 to x
}
Correct:
if (x === 5) {
// This checks if x is equal to 5
}
3. Not Considering All Possible Cases
Ensure that your conditional statements account for all possible scenarios, including edge cases.
Example:
function getLetterGrade(score) {
if (score >= 90) {
return "A";
} else if (score >= 80) {
return "B";
} else if (score >= 70) {
return "C";
} else if (score >= 60) {
return "D";
} else {
return "F";
}
}
This function handles all possible score ranges, including scores below 60.
Advanced Techniques and Patterns
1. Short-Circuit Evaluation
Logical operators in JavaScript use short-circuit evaluation, which can be leveraged for concise conditional statements.
function greet(name) {
name && console.log(`Hello, ${name}!`);
}
greet("Alice"); // Outputs: Hello, Alice!
greet(); // Outputs nothing
2. Nullish Coalescing Operator (??)
The nullish coalescing operator provides a way to handle null or undefined values elegantly.
let user = {
name: "Alice",
age: null
};
let userAge = user.age ?? "Age not provided";
console.log(userAge); // Outputs: "Age not provided"
3. Object Literal Pattern for Multiple Conditions
When dealing with multiple conditions that map to specific actions, consider using an object literal instead of a long if-else chain.
function getColorName(code) {
const colorMap = {
"R": "Red",
"G": "Green",
"B": "Blue",
"Y": "Yellow"
};
return colorMap[code] || "Unknown Color";
}
console.log(getColorName("R")); // Outputs: "Red"
console.log(getColorName("X")); // Outputs: "Unknown Color"
Conditional Statements in Different Programming Languages
While the concepts of conditional statements are universal, the syntax can vary across different programming languages. Let’s look at how if-else statements are implemented in a few popular languages:
Python
age = 20
if age >= 18:
print("You are an adult")
elif age >= 13:
print("You are a teenager")
else:
print("You are a child")
Java
int age = 20;
if (age >= 18) {
System.out.println("You are an adult");
} else if (age >= 13) {
System.out.println("You are a teenager");
} else {
System.out.println("You are a child");
}
C++
#include <iostream>
using namespace std;
int main() {
int age = 20;
if (age >= 18) {
cout << "You are an adult" << endl;
} else if (age >= 13) {
cout << "You are a teenager" << endl;
} else {
cout << "You are a child" << endl;
}
return 0;
}
Real-World Applications of Conditional Statements
Conditional statements are ubiquitous in programming and are used in countless real-world applications. Here are a few examples:
1. User Authentication
Conditional statements are crucial in verifying user credentials and granting or denying access to specific features or areas of an application.
function authenticateUser(username, password) {
if (username === "admin" && password === "secretpassword") {
return "Access granted";
} else {
return "Access denied";
}
}
2. Form Validation
When processing user input, conditional statements help ensure that the data meets specific criteria before it’s submitted or processed.
function validateEmail(email) {
if (email.includes("@") && email.includes(".")) {
return "Valid email format";
} else {
return "Invalid email format";
}
}
3. Game Logic
In game development, conditional statements control various aspects of gameplay, such as determining win conditions or managing player interactions.
function checkGameOver(playerHealth, enemiesDefeated) {
if (playerHealth <= 0) {
return "Game Over: You lost";
} else if (enemiesDefeated >= 10) {
return "Game Over: You won!";
} else {
return "Game continues";
}
}
4. Data Analysis and Reporting
Conditional statements are essential in data processing, helping to categorize, filter, and analyze large datasets.
function categorizeTemperature(temp) {
if (temp < 0) {
return "Freezing";
} else if (temp < 10) {
return "Cold";
} else if (temp < 20) {
return "Cool";
} else if (temp < 30) {
return "Warm";
} else {
return "Hot";
}
}
Conclusion
Conditional statements are a fundamental concept in programming, allowing developers to create dynamic and responsive applications. By mastering the use of if, else, and else if statements, you’ll be able to write more efficient and intelligent code. Remember to keep your conditions clear and concise, consider all possible scenarios, and choose the most appropriate conditional structure for each situation.
As you continue your journey in programming, you’ll find that conditional statements are an essential tool in your problem-solving toolkit. They form the basis of decision-making in code and are crucial for creating interactive and intelligent applications. Practice using these statements in various scenarios to become more proficient, and don’t hesitate to explore more advanced conditional patterns as you grow in your coding skills.
Whether you’re building a simple script or a complex application, the principles of conditional logic will always be relevant. By understanding and effectively implementing conditional statements, you’re taking a significant step towards becoming a skilled and versatile programmer.