Introduction to Arrays and Lists: Storing and Accessing Data
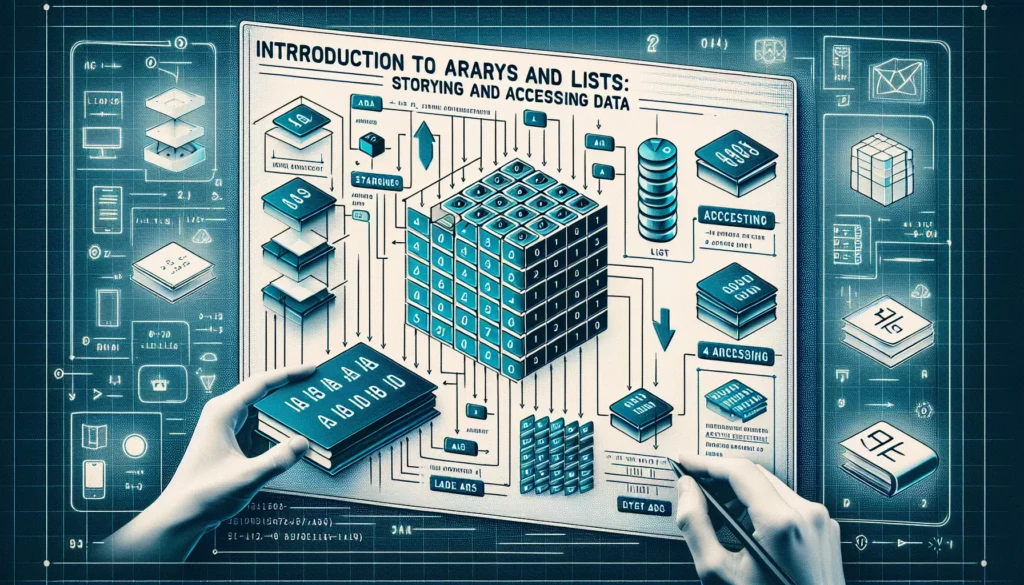
Welcome to AlgoCademy’s comprehensive guide on arrays and lists! As you embark on your journey to become a proficient programmer, understanding these fundamental data structures is crucial. Whether you’re a beginner just starting out or preparing for technical interviews at major tech companies, mastering arrays and lists will significantly boost your coding skills and problem-solving abilities.
What Are Arrays and Lists?
Arrays and lists are both data structures used to store collections of elements. While they serve similar purposes, there are some key differences between them:
Arrays
An array is a fixed-size, contiguous block of memory that stores elements of the same data type. Arrays are indexed, meaning each element can be accessed directly using its position (index) in the array. The main characteristics of arrays include:
- Fixed size (in most programming languages)
- Contiguous memory allocation
- Fast access time (O(1) for retrieving elements by index)
- Efficient memory usage
Lists
Lists, on the other hand, are more flexible data structures that can grow or shrink in size dynamically. They are typically implemented as linked lists or dynamic arrays. The main characteristics of lists include:
- Dynamic size
- Non-contiguous memory allocation (for linked lists)
- Slower access time for arbitrary elements (O(n) for linked lists, O(1) for dynamic arrays)
- More memory overhead due to additional pointers or capacity management
Creating Arrays and Lists
Let’s explore how to create arrays and lists in different programming languages:
Creating Arrays
Java
// Declaring and initializing an array of integers
int[] numbers = {1, 2, 3, 4, 5};
// Declaring an array of strings with a specific size
String[] names = new String[10];
// Multidimensional array
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Python
# In Python, lists are used instead of arrays
numbers = [1, 2, 3, 4, 5]
# Creating a list of strings
names = ["Alice", "Bob", "Charlie"]
# Multidimensional list
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
JavaScript
// Creating an array of numbers
let numbers = [1, 2, 3, 4, 5];
// Creating an array of strings
let names = ["Alice", "Bob", "Charlie"];
// Multidimensional array
let matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
Creating Lists
Java (ArrayList)
import java.util.ArrayList;
// Creating an ArrayList of integers
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
// Creating an ArrayList of strings
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Python
# In Python, lists are used for both arrays and dynamic lists
numbers = [1, 2, 3, 4, 5]
# Creating a list of strings
names = ["Alice", "Bob", "Charlie"]
# Adding elements to a list
numbers.append(6)
names.append("David")
JavaScript
// In JavaScript, arrays are used for both fixed-size and dynamic lists
let numbers = [1, 2, 3, 4, 5];
// Adding elements to an array
numbers.push(6);
let names = ["Alice", "Bob", "Charlie"];
names.push("David");
Accessing Elements in Arrays and Lists
Accessing elements in arrays and lists is typically done using index notation. Remember that in most programming languages, array indices start at 0.
Accessing Array Elements
Java
int[] numbers = {10, 20, 30, 40, 50};
// Accessing elements
int firstElement = numbers[0]; // 10
int thirdElement = numbers[2]; // 30
// Modifying elements
numbers[1] = 25; // Array is now {10, 25, 30, 40, 50}
Python
numbers = [10, 20, 30, 40, 50]
# Accessing elements
first_element = numbers[0] # 10
third_element = numbers[2] # 30
# Modifying elements
numbers[1] = 25 # List is now [10, 25, 30, 40, 50]
JavaScript
let numbers = [10, 20, 30, 40, 50];
// Accessing elements
let firstElement = numbers[0]; // 10
let thirdElement = numbers[2]; // 30
// Modifying elements
numbers[1] = 25; // Array is now [10, 25, 30, 40, 50]
Accessing List Elements
Accessing elements in lists is similar to arrays in most languages:
Java (ArrayList)
import java.util.ArrayList;
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
// Accessing elements
int firstElement = numbers.get(0); // 10
int secondElement = numbers.get(1); // 20
// Modifying elements
numbers.set(1, 25); // List is now [10, 25, 30]
Python
numbers = [10, 20, 30, 40, 50]
# Accessing elements (same as arrays)
first_element = numbers[0] # 10
third_element = numbers[2] # 30
# Modifying elements
numbers[1] = 25 # List is now [10, 25, 30, 40, 50]
JavaScript
let numbers = [10, 20, 30, 40, 50];
// Accessing elements (same as arrays)
let firstElement = numbers[0]; // 10
let thirdElement = numbers[2]; // 30
// Modifying elements
numbers[1] = 25; // Array is now [10, 25, 30, 40, 50]
Common Operations on Arrays and Lists
Let’s explore some common operations you can perform on arrays and lists:
1. Finding the Length
Java
int[] array = {1, 2, 3, 4, 5};
int length = array.length; // 5
ArrayList<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(3);
int size = list.size(); // 3
Python
numbers = [1, 2, 3, 4, 5]
length = len(numbers) # 5
JavaScript
let numbers = [1, 2, 3, 4, 5];
let length = numbers.length; // 5
2. Adding Elements
Java
// For arrays, you can't add elements directly (fixed size)
// For ArrayList
ArrayList<Integer> list = new ArrayList<>();
list.add(1); // Adds to the end
list.add(0, 2); // Adds at index 0
Python
numbers = [1, 2, 3]
numbers.append(4) # Adds to the end: [1, 2, 3, 4]
numbers.insert(0, 0) # Adds at index 0: [0, 1, 2, 3, 4]
JavaScript
let numbers = [1, 2, 3];
numbers.push(4); // Adds to the end: [1, 2, 3, 4]
numbers.unshift(0); // Adds at the beginning: [0, 1, 2, 3, 4]
3. Removing Elements
Java
// For arrays, you can't remove elements directly (fixed size)
// For ArrayList
ArrayList<Integer> list = new ArrayList<>(Arrays.asList(1, 2, 3, 4));
list.remove(1); // Removes element at index 1
list.remove(Integer.valueOf(3)); // Removes the first occurrence of 3
Python
numbers = [1, 2, 3, 4]
numbers.pop() # Removes and returns the last element: 4
numbers.pop(0) # Removes and returns the element at index 0: 1
numbers.remove(3) # Removes the first occurrence of 3
JavaScript
let numbers = [1, 2, 3, 4];
numbers.pop(); // Removes and returns the last element: 4
numbers.shift(); // Removes and returns the first element: 1
numbers.splice(1, 1); // Removes 1 element starting at index 1
4. Searching for Elements
Java
int[] array = {1, 2, 3, 4, 5};
int index = Arrays.binarySearch(array, 3); // Returns 2
ArrayList<Integer> list = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
int listIndex = list.indexOf(3); // Returns 2
Python
numbers = [1, 2, 3, 4, 5]
index = numbers.index(3) # Returns 2
JavaScript
let numbers = [1, 2, 3, 4, 5];
let index = numbers.indexOf(3); // Returns 2
Iterating Through Arrays and Lists
Iterating through arrays and lists is a common operation in programming. Here are some ways to do it in different languages:
Java
// For arrays
int[] numbers = {1, 2, 3, 4, 5};
// Using a for loop
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Using an enhanced for loop (for-each)
for (int num : numbers) {
System.out.println(num);
}
// For ArrayList
ArrayList<Integer> list = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
// Using an enhanced for loop
for (int num : list) {
System.out.println(num);
}
// Using an iterator
Iterator<Integer> it = list.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
// Using Java 8 streams
list.forEach(System.out::println);
Python
numbers = [1, 2, 3, 4, 5]
# Using a for loop
for num in numbers:
print(num)
# Using enumerate if you need the index
for index, num in enumerate(numbers):
print(f"Index {index}: {num}")
# List comprehension (for creating a new list)
squared = [num ** 2 for num in numbers]
# Using while loop with an index
i = 0
while i < len(numbers):
print(numbers[i])
i += 1
JavaScript
let numbers = [1, 2, 3, 4, 5];
// Using a for loop
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
// Using for...of loop
for (let num of numbers) {
console.log(num);
}
// Using forEach
numbers.forEach(num => console.log(num));
// Using map (for creating a new array)
let squared = numbers.map(num => num ** 2);
// Using a while loop
let i = 0;
while (i < numbers.length) {
console.log(numbers[i]);
i++;
}
Multidimensional Arrays and Lists
Multidimensional arrays and lists are used to represent tables, matrices, or more complex data structures. Here’s how to work with them:
Java
// 2D array
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// Accessing elements
int element = matrix[1][2]; // 6
// Iterating through a 2D array
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
// 2D ArrayList
ArrayList<ArrayList<Integer>> list2D = new ArrayList<>();
list2D.add(new ArrayList<>(Arrays.asList(1, 2, 3)));
list2D.add(new ArrayList<>(Arrays.asList(4, 5, 6)));
list2D.add(new ArrayList<>(Arrays.asList(7, 8, 9)));
Python
# 2D list
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
# Accessing elements
element = matrix[1][2] # 6
# Iterating through a 2D list
for row in matrix:
for element in row:
print(element, end=" ")
print()
# List comprehension for 2D list
flattened = [num for row in matrix for num in row]
JavaScript
// 2D array
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
// Accessing elements
let element = matrix[1][2]; // 6
// Iterating through a 2D array
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j]);
}
}
// Using forEach for 2D array
matrix.forEach(row => row.forEach(element => console.log(element)));
Time Complexity and Performance Considerations
Understanding the time complexity of operations on arrays and lists is crucial for writing efficient code, especially when preparing for technical interviews. Here’s a quick overview:
Arrays
- Access by index: O(1)
- Search: O(n) for unsorted, O(log n) for sorted (using binary search)
- Insertion at the end (for dynamic arrays): O(1) amortized
- Insertion at the beginning or middle: O(n)
- Deletion at the end: O(1)
- Deletion at the beginning or middle: O(n)
Lists (assuming dynamic arrays or linked lists)
- Access by index: O(1) for dynamic arrays, O(n) for linked lists
- Search: O(n)
- Insertion at the end: O(1) amortized for dynamic arrays, O(1) for linked lists
- Insertion at the beginning: O(n) for dynamic arrays, O(1) for linked lists
- Deletion at the end: O(1) amortized for dynamic arrays, O(n) for singly linked lists (O(1) for doubly linked lists)
- Deletion at the beginning: O(n) for dynamic arrays, O(1) for linked lists
Common Pitfalls and Best Practices
When working with arrays and lists, keep these tips in mind:
- Always check array bounds to avoid IndexOutOfBoundsException or similar errors.
- Be cautious with multidimensional arrays, as they can be memory-intensive.
- When dealing with large datasets, consider using more efficient data structures like HashSet or HashMap for faster lookups.
- In Java, prefer ArrayList over raw arrays for most use cases due to its flexibility.
- In Python, use list comprehensions for concise and readable code when creating new lists based on existing ones.
- In JavaScript, be aware of the differences between shallow and deep copying when working with nested arrays.
- When performance is critical, consider using typed arrays in JavaScript for numerical computations.
Conclusion
Arrays and lists are fundamental data structures in programming, and mastering them is essential for any aspiring software developer. They form the backbone of many algorithms and are often used in technical interviews to assess a candidate’s problem-solving skills.
As you continue your journey with AlgoCademy, practice implementing various algorithms using arrays and lists. Challenge yourself with problems that require efficient use of these data structures, such as two-pointer techniques, sliding window algorithms, and matrix manipulations.
Remember, the key to mastering these concepts is consistent practice and application. Use AlgoCademy’s interactive coding tutorials and AI-powered assistance to refine your skills and prepare for technical interviews at top tech companies.
Happy coding, and may your arrays always be in bounds and your lists ever-growing in your programming repertoire!