Introduction to Algorithms: Where to Begin Your Journey
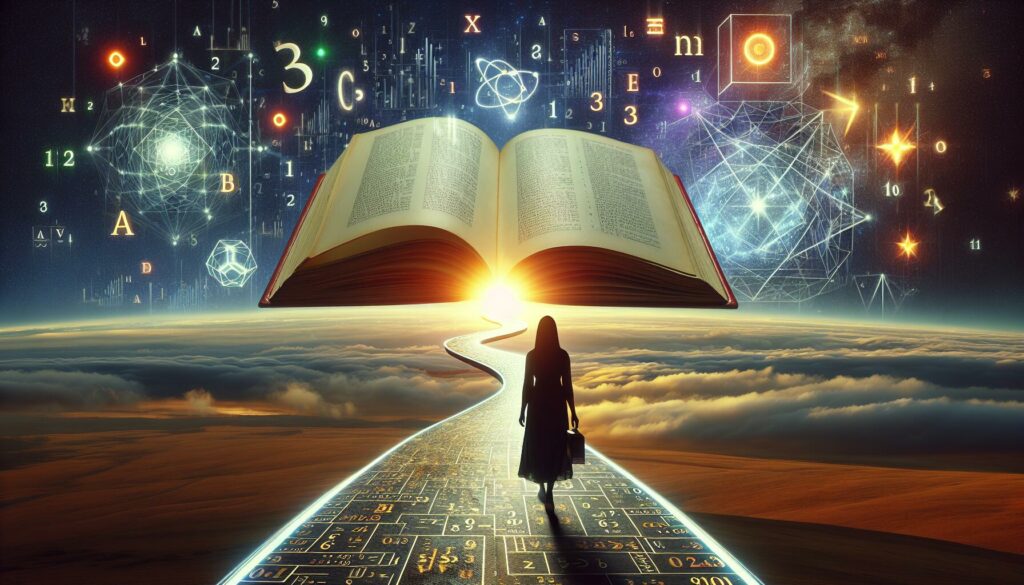
Welcome to the exciting world of algorithms! If you’re embarking on your coding journey or looking to level up your programming skills, understanding algorithms is a crucial step. In this comprehensive guide, we’ll explore the fundamentals of algorithms, why they’re important, and how you can start mastering them to become a more effective programmer.
What Are Algorithms?
At its core, an algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In the context of computer science, algorithms are the building blocks of all software applications. They’re the recipes that tell a computer how to process data, make decisions, and produce desired outcomes.
Think of algorithms like cooking recipes. Just as a recipe provides instructions to create a dish, an algorithm provides instructions for a computer to solve a problem. And just like there can be multiple recipes for the same dish, there can be multiple algorithms to solve the same problem – some more efficient than others.
Why Are Algorithms Important?
Understanding algorithms is crucial for several reasons:
- Problem-solving skills: Learning algorithms enhances your ability to break down complex problems into manageable steps.
- Efficiency: Good algorithms can significantly improve the speed and performance of your programs.
- Scalability: Efficient algorithms allow your solutions to handle larger amounts of data without slowing down.
- Interview preparation: Many technical interviews, especially for major tech companies, focus heavily on algorithmic problem-solving.
- Foundation for advanced topics: A solid grasp of algorithms is essential for understanding more complex computer science concepts.
Getting Started with Algorithms
Now that we understand the importance of algorithms, let’s discuss how you can begin your journey into this fascinating field.
1. Master the Basics of Programming
Before diving deep into algorithms, ensure you have a strong foundation in at least one programming language. Python is often recommended for beginners due to its readability and extensive libraries, but languages like Java, C++, or JavaScript are also excellent choices.
Here’s a simple example of an algorithm implemented in Python – the classic “Hello, World!” program:
def greet():
print("Hello, World!")
greet()
This simple function demonstrates the basic structure of an algorithm: a defined set of steps (in this case, just one step) to accomplish a task (printing a greeting).
2. Understand Basic Data Structures
Data structures are the building blocks of algorithms. Familiarize yourself with fundamental data structures such as:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
Understanding these structures will help you choose the right tool for each algorithmic task. For instance, here’s a simple implementation of a stack in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Outputs: 3
3. Learn Basic Algorithm Design Techniques
As you progress, you’ll want to familiarize yourself with common algorithm design techniques. Some fundamental ones include:
- Brute Force: Trying all possible solutions to find the correct one.
- Divide and Conquer: Breaking a problem into smaller subproblems, solving them, and combining the results.
- Greedy Algorithms: Making the locally optimal choice at each step.
- Dynamic Programming: Solving complex problems by breaking them down into simpler subproblems.
Here’s a simple example of a divide-and-conquer algorithm – the binary search:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(result) # Outputs: 3 (index of 7 in the array)
4. Practice, Practice, Practice
The key to mastering algorithms is consistent practice. Here are some ways to hone your skills:
- Coding challenges: Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of algorithmic problems to solve.
- Implement classic algorithms: Try coding well-known algorithms like bubble sort, merge sort, or Dijkstra’s algorithm from scratch.
- Participate in coding competitions: Sites like Codeforces and TopCoder host regular competitions that can test and improve your skills.
- Contribute to open-source projects: This can give you real-world experience in applying algorithmic thinking to solve practical problems.
5. Study Time and Space Complexity
As you become more comfortable with algorithms, it’s crucial to understand how to analyze their efficiency. This is where the concepts of time complexity and space complexity come in.
Time complexity refers to how the runtime of an algorithm grows as the input size increases. It’s typically expressed using Big O notation. For example, an algorithm with O(n) time complexity will take twice as long to run if you double the input size.
Space complexity refers to how much additional memory an algorithm needs as the input size grows. This is also typically expressed using Big O notation.
Here’s a simple example to illustrate different time complexities:
# O(1) - Constant time
def get_first_element(arr):
return arr[0] if arr else None
# O(n) - Linear time
def find_max(arr):
if not arr:
return None
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
# O(n^2) - Quadratic time
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
Common Algorithms to Study
As you progress in your algorithmic journey, there are several classic algorithms you should become familiar with. These form the foundation of many more complex algorithms and are frequently used in technical interviews.
1. Sorting Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
Here’s a simple implementation of the Merge Sort algorithm in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = merge_sort(unsorted_array)
print(sorted_array) # Outputs: [11, 12, 22, 25, 34, 64, 90]
2. Searching Algorithms
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
3. Graph Algorithms
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Kruskal’s Algorithm
- Prim’s Algorithm
4. Dynamic Programming
Dynamic Programming (DP) is a powerful technique for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems. Here’s a classic DP problem – the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Usage
print(fibonacci(10)) # Outputs: 55
Tools and Resources for Learning Algorithms
As you embark on your journey to master algorithms, there are numerous resources available to support your learning:
1. Online Platforms
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and preparing for technical interviews.
- LeetCode: Provides a vast collection of coding challenges, many focused on algorithmic problem-solving.
- HackerRank: Offers coding challenges and competitions in various domains, including algorithms.
- Coursera: Hosts several high-quality algorithm courses from top universities.
2. Books
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Grokking Algorithms” by Aditya Bhargava
- “The Algorithm Design Manual” by Steven S. Skiena
3. Visualization Tools
- VisuAlgo: Offers interactive visualizations of various data structures and algorithms.
- Algorithm Visualizer: An interactive platform where you can visualize algorithms as they run.
Applying Algorithmic Thinking in Real-World Scenarios
While learning algorithms is crucial for passing technical interviews, their real value lies in applying algorithmic thinking to solve real-world problems. Here are some ways algorithms are used in various industries:
1. Finance
- High-frequency trading algorithms
- Risk assessment models
- Fraud detection systems
2. Healthcare
- Disease prediction models
- Medical image processing
- Drug discovery algorithms
3. Transportation
- Route optimization for delivery services
- Traffic flow prediction
- Autonomous vehicle navigation
4. E-commerce
- Recommendation systems
- Pricing optimization algorithms
- Supply chain management
By understanding algorithms, you’re not just preparing for interviews – you’re developing a skill set that can be applied to solve complex problems across various domains.
Conclusion: Your Algorithmic Journey Begins
Embarking on the path to mastering algorithms can seem daunting at first, but remember that every expert was once a beginner. The key is to start with the basics, practice consistently, and gradually tackle more complex problems.
As you progress in your journey, you’ll find that understanding algorithms not only makes you a better programmer but also enhances your problem-solving skills in general. Whether you’re aiming to ace technical interviews at top tech companies or simply want to improve your coding skills, a strong foundation in algorithms will serve you well.
Remember, the world of algorithms is vast and constantly evolving. Stay curious, keep learning, and don’t be afraid to tackle challenging problems. With persistence and practice, you’ll be solving complex algorithmic problems in no time.
Happy coding, and may your algorithmic journey be filled with exciting discoveries and satisfying “Aha!” moments!