Introduction to Algorithms Complexity: Understanding Big O Notation
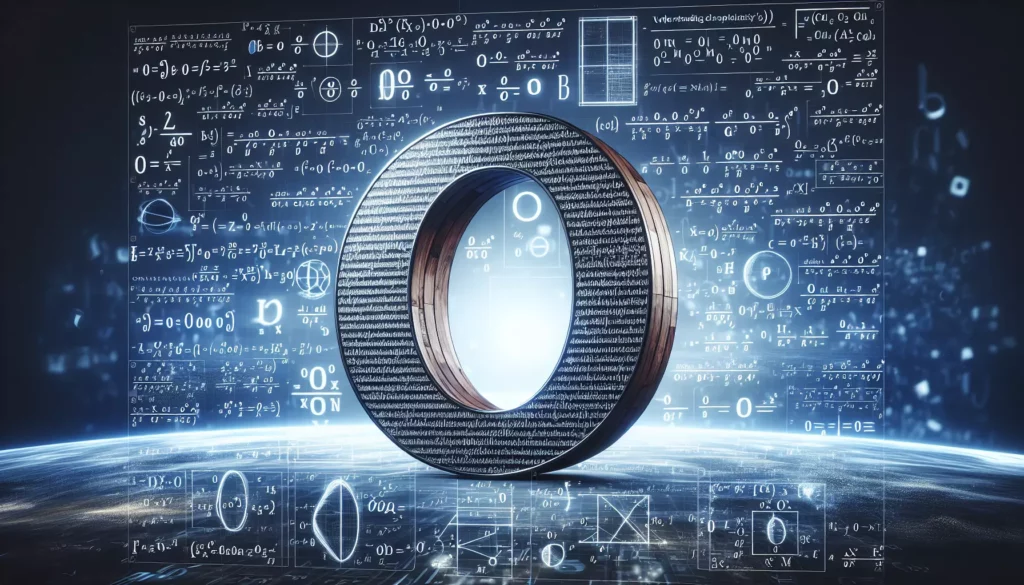
In the world of computer science and programming, efficiency is key. As software systems grow larger and more complex, the need for optimized algorithms becomes increasingly crucial. This is where the concept of algorithm complexity comes into play, and at the heart of this concept lies Big O Notation. In this comprehensive guide, we’ll dive deep into the world of algorithm complexity, exploring what Big O Notation is, why it matters, and how you can use it to analyze and improve your code.
What is Algorithm Complexity?
Before we delve into Big O Notation, let’s first understand what we mean by algorithm complexity. Algorithm complexity refers to the amount of resources (such as time and space) required by an algorithm to run as a function of the input size. There are two main types of complexity we consider:
- Time Complexity: This measures how long an algorithm takes to run as the input size increases.
- Space Complexity: This measures how much memory an algorithm uses as the input size increases.
While both are important, we’ll focus primarily on time complexity in this article, as it’s often the most critical factor in algorithm performance.
Introducing Big O Notation
Big O Notation is a mathematical notation used to describe the upper bound of an algorithm’s growth rate. In simpler terms, it tells us how the runtime of an algorithm grows as the input size increases. The “O” in Big O stands for “Order of,” which refers to the order of magnitude of the algorithm’s growth rate.
Big O Notation expresses the worst-case scenario for an algorithm’s time complexity. It provides a standardized way to compare the efficiency of different algorithms, regardless of the specific hardware or programming language used.
Why is Big O Notation Important?
Understanding Big O Notation is crucial for several reasons:
- Performance Prediction: It helps you predict how your algorithm will perform as the input size grows.
- Algorithm Comparison: It provides a standardized way to compare different algorithms solving the same problem.
- Optimization: It guides you in optimizing your code by identifying inefficient parts of your algorithm.
- Scalability: It helps you understand how well your algorithm will scale as data sizes increase.
- Interview Preparation: Big O analysis is a common topic in technical interviews, especially for roles at major tech companies.
Common Big O Notations
Let’s explore some of the most common Big O notations, from the most efficient to the least:
O(1) – Constant Time
An algorithm with O(1) complexity performs the same number of operations regardless of the input size. These are the most efficient algorithms.
Example: Accessing an array element by index.
function getArrayElement(arr, index) {
return arr[index];
}
O(log n) – Logarithmic Time
These algorithms reduce the problem size by a factor in each step. They are very efficient, especially for large inputs.
Example: Binary search in a sorted array.
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
O(n) – Linear Time
The runtime of these algorithms grows linearly with the input size. They are generally considered efficient for small to medium-sized inputs.
Example: Linear search in an unsorted array.
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
O(n log n) – Linearithmic Time
These algorithms are slightly less efficient than linear time but still perform well. Many efficient sorting algorithms fall into this category.
Example: Merge sort algorithm.
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
O(n^2) – Quadratic Time
These algorithms have a runtime that’s proportional to the square of the input size. They can become slow with larger inputs.
Example: Bubble sort algorithm.
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
O(2^n) – Exponential Time
These algorithms have a runtime that doubles with each addition to the input. They become very slow very quickly.
Example: Recursive calculation of Fibonacci numbers without memoization.
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
Analyzing Algorithms with Big O Notation
When analyzing an algorithm’s time complexity using Big O Notation, we follow these general steps:
- Identify the input: Determine what constitutes the input and how it affects the algorithm’s runtime.
- Count the operations: Identify the basic operations that contribute to the runtime.
- Express in terms of input size: Write an expression that relates the number of operations to the input size.
- Simplify: Remove constants and lower-order terms, keeping only the highest-order term.
- Express in Big O notation: Write the final expression using Big O notation.
Let’s analyze a simple example:
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
Analysis:
- The input is the array
arr
. Let’s say its length is n. - The basic operation is the addition inside the loop, which happens n times.
- The number of operations is directly proportional to n.
- There are no lower-order terms to remove.
- The time complexity is O(n).
Common Pitfalls in Big O Analysis
While Big O Notation is a powerful tool, there are some common misconceptions and pitfalls to avoid:
1. Ignoring Constants
Big O Notation ignores constants. For example, O(2n) is simplified to O(n). While this makes analysis simpler, it’s important to remember that constants can matter in practice, especially for smaller inputs.
2. Focusing Only on Worst-Case Scenarios
Big O represents the upper bound or worst-case scenario. Sometimes, average-case performance (often denoted as Θ (Theta) notation) might be more relevant in real-world scenarios.
3. Overlooking Space Complexity
While we often focus on time complexity, space complexity can be equally important, especially in memory-constrained environments.
4. Misunderstanding Nested Loops
Nested loops don’t always result in O(n^2) complexity. The complexity depends on how the loops relate to the input size.
Improving Algorithm Efficiency
Understanding Big O Notation is just the first step. The real power comes from using this knowledge to improve your algorithms. Here are some strategies:
1. Use Appropriate Data Structures
Different data structures have different time complexities for various operations. Choosing the right data structure can significantly improve your algorithm’s efficiency.
For example, using a hash table (O(1) average case for insertion and lookup) instead of an array (O(n) for search) can dramatically speed up certain operations.
2. Avoid Unnecessary Work
Look for opportunities to reduce the number of operations your algorithm performs. This might involve breaking out of loops early, using memoization, or avoiding redundant calculations.
3. Divide and Conquer
Many efficient algorithms use a divide-and-conquer approach, breaking down a problem into smaller subproblems. This often leads to logarithmic time complexities.
4. Use Dynamic Programming
For problems with overlapping subproblems, dynamic programming can often reduce time complexity from exponential to polynomial.
5. Amortized Analysis
Some data structures, like dynamic arrays, have operations that are occasionally costly but cheap on average. Understanding amortized analysis can help you make better decisions about when to use such structures.
Big O Notation in Practice
While understanding the theory of Big O Notation is crucial, it’s equally important to see how it applies in real-world scenarios. Let’s look at some practical examples:
1. API Design
When designing APIs, considering the time complexity of operations is crucial. For instance, if you’re creating a data structure to store user information:
- An array might be fine for small numbers of users (O(n) lookup)
- A hash table would be better for larger numbers (O(1) average case lookup)
- A balanced tree could be a good compromise if you need sorted data (O(log n) lookup)
2. Database Queries
Understanding time complexity helps in optimizing database queries:
- Full table scans are O(n) and should be avoided for large tables
- Indexed lookups are typically O(log n) or O(1), making them much faster for large datasets
- Joins can be expensive, often O(n * m) where n and m are the sizes of the joined tables
3. Web Application Scaling
As web applications grow, algorithmic efficiency becomes increasingly important:
- Caching frequently accessed data can reduce time complexity from O(n) to O(1)
- Pagination of large result sets can change the perceived complexity from O(n) to O(1) from the user’s perspective
- Using appropriate data structures in your backend can significantly impact response times as data grows
Advanced Topics in Algorithm Complexity
As you become more comfortable with basic Big O analysis, there are several advanced topics you might want to explore:
1. Amortized Analysis
This technique considers the average performance of a sequence of operations, rather than just the worst-case scenario for a single operation. It’s particularly useful for analyzing data structures like dynamic arrays.
2. Space-Time Tradeoffs
Often, you can trade space complexity for time complexity, or vice versa. Understanding these tradeoffs can help you make informed decisions about algorithm design.
3. Lower Bounds and Omega Notation
While Big O provides an upper bound, Omega (Ω) notation provides a lower bound on the growth rate of an algorithm. Understanding both can give a more complete picture of an algorithm’s performance.
4. NP-Completeness
Some problems are believed to have no polynomial-time solutions. Understanding NP-completeness can help you recognize when you’re dealing with such problems and adjust your approach accordingly.
Conclusion
Big O Notation is a fundamental concept in computer science and a crucial tool for any serious programmer. It provides a standardized way to analyze and compare algorithms, helping us make informed decisions about algorithm design and optimization.
Remember, the goal of understanding Big O Notation isn’t just to write faster code—it’s to write scalable, efficient solutions that can handle growing amounts of data and complexity. As you continue your journey in programming, you’ll find that a solid grasp of algorithmic complexity will serve you well in everything from day-to-day coding tasks to technical interviews at top tech companies.
Keep practicing, analyzing, and optimizing. The more you work with Big O Notation, the more intuitive it will become, and the better equipped you’ll be to tackle complex programming challenges. Happy coding!