Interview Inception: Preparing for Coding Interviews by Creating a Coding Interview Simulator
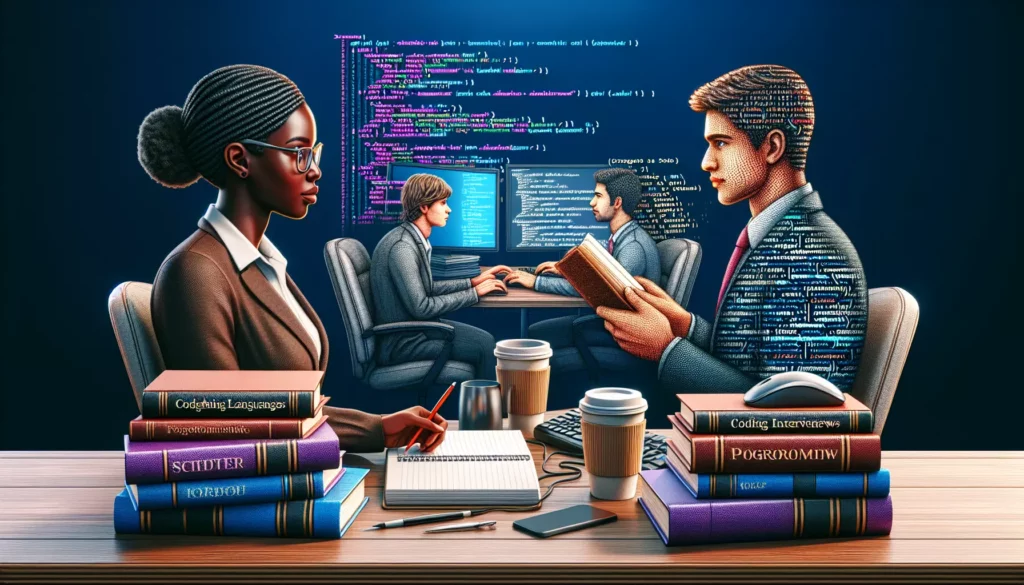
In the competitive world of software engineering, landing a job at a top tech company often requires passing rigorous coding interviews. These interviews test not only your coding skills but also your problem-solving abilities and algorithmic thinking. As the saying goes, “practice makes perfect,” and what better way to practice for coding interviews than by creating your own coding interview simulator? In this comprehensive guide, we’ll explore how building a coding interview simulator can significantly enhance your preparation for technical interviews, particularly those at major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
Why Create a Coding Interview Simulator?
Before we dive into the details of building a coding interview simulator, let’s understand why this approach is particularly effective:
- Deep Understanding: By creating a simulator, you’ll gain a deeper understanding of the interview process and the types of problems typically asked.
- Customization: You can tailor the simulator to focus on your weak areas or specific types of problems you want to practice.
- Active Learning: Building the simulator involves active engagement with coding concepts, reinforcing your learning.
- Problem Creation: Coming up with interview questions forces you to think from the interviewer’s perspective, enhancing your problem-solving skills.
- Technical Skills: Developing the simulator itself is a coding project that can improve your programming abilities.
Components of a Coding Interview Simulator
A comprehensive coding interview simulator should include the following components:
- Problem Bank: A collection of coding problems of varying difficulty levels.
- Code Editor: An interface where users can write and edit code.
- Test Cases: Pre-defined inputs and expected outputs to validate solutions.
- Timer: To simulate the time pressure of real interviews.
- Hint System: Provides incremental hints to users who are stuck.
- Solution Checker: Evaluates the correctness and efficiency of submitted code.
- Performance Metrics: Tracks and displays user progress over time.
Step 1: Setting Up the Development Environment
Before we start building our simulator, let’s set up a development environment. We’ll use Python for this project due to its simplicity and powerful libraries. Here’s what you’ll need:
- Python 3.x installed on your system
- A code editor (e.g., Visual Studio Code, PyCharm)
- Basic knowledge of web development (HTML, CSS, JavaScript)
- Familiarity with a web framework like Flask or Django
Let’s start by creating a new Python virtual environment and installing Flask:
python -m venv interview_simulator
source interview_simulator/bin/activate # On Windows, use `interview_simulator\Scripts\activate`
pip install flask
Step 2: Creating the Problem Bank
The heart of your simulator will be the problem bank. Start by creating a Python file called problems.py
to store your coding problems. Each problem should include a description, example inputs and outputs, and test cases.
problems = [
{
"id": 1,
"title": "Two Sum",
"description": "Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target.",
"example": "Input: nums = [2,7,11,15], target = 9\nOutput: [0,1]",
"test_cases": [
{"input": "[2,7,11,15], 9", "output": "[0,1]"},
{"input": "[3,2,4], 6", "output": "[1,2]"},
{"input": "[3,3], 6", "output": "[0,1]"}
],
"difficulty": "Easy"
},
# Add more problems here
]
Step 3: Building the Web Interface
Now, let’s create a simple web interface for our simulator using Flask. Create a new file called app.py
:
from flask import Flask, render_template, request, jsonify
from problems import problems
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html', problems=problems)
@app.route('/problem/<int:problem_id>')
def problem(problem_id):
problem = next((p for p in problems if p["id"] == problem_id), None)
if problem:
return render_template('problem.html', problem=problem)
return "Problem not found", 404
if __name__ == '__main__':
app.run(debug=True)
Create a templates folder and add two HTML files: index.html
for the problem list and problem.html
for the individual problem view.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Coding Interview Simulator</title>
</head>
<body>
<h1>Coding Interview Simulator</h1>
<ul>
{% for problem in problems %}
<li><a href="{{ url_for('problem', problem_id=problem.id) }}">{{ problem.title }} ({{ problem.difficulty }})</a></li>
{% endfor %}
</ul>
</body>
</html>
problem.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{ problem.title }} - Coding Interview Simulator</title>
</head>
<body>
<h1>{{ problem.title }}</h1>
<p>Difficulty: {{ problem.difficulty }}</p>
<h2>Description</h2>
<p>{{ problem.description }}</p>
<h2>Example</h2>
<pre>{{ problem.example }}</pre>
<h2>Your Solution</h2>
<textarea id="code-editor" rows="20" cols="80"></textarea>
<br>
<button id="submit-btn">Submit Solution</button>
<div id="result"></div>
</body>
</html>
Step 4: Implementing the Code Editor
For a more professional code editor experience, we can integrate a JavaScript-based code editor like Monaco Editor (the editor used in Visual Studio Code). Add the following to your problem.html
file:
<!-- Add this in the <head> section -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/monaco-editor/0.30.1/min/vs/loader.min.js"></script>
<!-- Replace the textarea with this div -->
<div id="code-editor" style="width:800px;height:400px;border:1px solid grey"></div>
<!-- Add this script at the end of the body -->
<script>
require.config({ paths: { 'vs': 'https://cdnjs.cloudflare.com/ajax/libs/monaco-editor/0.30.1/min/vs' }});
require(['vs/editor/editor.main'], function() {
var editor = monaco.editor.create(document.getElementById('code-editor'), {
value: [
'def solution(nums, target):',
' # Write your code here',
' pass',
'',
'# Example usage:',
'# result = solution([2,7,11,15], 9)',
'# print(result)'
].join('\n'),
language: 'python',
theme: 'vs-dark'
});
});
</script>
Step 5: Adding a Timer
To simulate the time pressure of real interviews, let’s add a timer to our problem page. Add the following HTML and JavaScript to your problem.html
file:
<!-- Add this below the problem description -->
<div id="timer">Time: 00:00</div>
<!-- Add this script at the end of the body -->
<script>
let seconds = 0;
let timerInterval;
function startTimer() {
timerInterval = setInterval(() => {
seconds++;
const minutes = Math.floor(seconds / 60);
const remainingSeconds = seconds % 60;
document.getElementById('timer').textContent =
`Time: ${minutes.toString().padStart(2, '0')}:${remainingSeconds.toString().padStart(2, '0')}`;
}, 1000);
}
function stopTimer() {
clearInterval(timerInterval);
}
// Start the timer when the page loads
window.onload = startTimer;
</script>
Step 6: Implementing the Solution Checker
Now, let’s create a backend route to check the submitted solutions. Add the following to your app.py
file:
import io
import sys
from contextlib import redirect_stdout
@app.route('/check_solution/<int:problem_id>', methods=['POST'])
def check_solution(problem_id):
problem = next((p for p in problems if p["id"] == problem_id), None)
if not problem:
return jsonify({"error": "Problem not found"}), 404
user_code = request.json['code']
results = []
for test_case in problem['test_cases']:
input_data = eval(test_case['input'])
expected_output = eval(test_case['output'])
# Capture stdout
captured_output = io.StringIO()
sys.stdout = captured_output
try:
# Execute user code
exec(user_code)
result = eval(f"solution(*{input_data})")
# Check if the result matches the expected output
if result == expected_output:
results.append({"status": "Pass", "input": test_case['input'], "expected": expected_output, "actual": result})
else:
results.append({"status": "Fail", "input": test_case['input'], "expected": expected_output, "actual": result})
except Exception as e:
results.append({"status": "Error", "input": test_case['input'], "error": str(e)})
finally:
sys.stdout = sys.__stdout__
return jsonify(results)
Now, update the JavaScript in problem.html
to send the code to the server for checking:
<script>
// ... (previous code)
document.getElementById('submit-btn').addEventListener('click', function() {
const code = editor.getValue();
fetch(`/check_solution/{{ problem.id }}`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({code: code}),
})
.then(response => response.json())
.then(data => {
let resultHtml = '<h3>Results:</h3><ul>';
data.forEach(result => {
resultHtml += `<li>${result.status}: Input ${result.input}, Expected ${result.expected}, Actual ${result.actual || result.error}</li>`;
});
resultHtml += '</ul>';
document.getElementById('result').innerHTML = resultHtml;
stopTimer();
})
.catch((error) => {
console.error('Error:', error);
});
});
</script>
Step 7: Adding a Hint System
To help users who are stuck, let’s implement a hint system. First, add hints to your problem data in problems.py
:
problems = [
{
# ... (previous problem data)
"hints": [
"Consider using a hash table to store the complement of each number.",
"As you iterate through the array, check if the current number's complement exists in the hash table.",
"The time complexity of this solution should be O(n)."
]
},
# ... (other problems)
]
Now, add a hint button and a container for hints in problem.html
:
<!-- Add this below the code editor -->
<button id="hint-btn">Get Hint</button>
<div id="hints"></div>
<!-- Add this script at the end of the body -->
<script>
let hintIndex = 0;
const hints = {{ problem.hints|tojson }};
document.getElementById('hint-btn').addEventListener('click', function() {
if (hintIndex < hints.length) {
const hintElement = document.createElement('p');
hintElement.textContent = `Hint ${hintIndex + 1}: ${hints[hintIndex]}`;
document.getElementById('hints').appendChild(hintElement);
hintIndex++;
} else {
alert('No more hints available!');
}
});
</script>
Step 8: Implementing Performance Metrics
To help users track their progress, let’s add some basic performance metrics. We’ll store the user’s attempts and completion times for each problem. First, add a new route to app.py
:
from flask import session
app.secret_key = 'your_secret_key_here' # Required for using sessions
@app.route('/save_attempt/<int:problem_id>', methods=['POST'])
def save_attempt(problem_id):
if 'attempts' not in session:
session['attempts'] = {}
if str(problem_id) not in session['attempts']:
session['attempts'][str(problem_id)] = []
attempt_data = request.json
session['attempts'][str(problem_id)].append(attempt_data)
session.modified = True
return jsonify({"message": "Attempt saved successfully"})
Now, update the JavaScript in problem.html
to save the attempt data:
<script>
// ... (previous code)
document.getElementById('submit-btn').addEventListener('click', function() {
const code = editor.getValue();
const endTime = new Date();
const timeSpent = (endTime - startTime) / 1000; // Time spent in seconds
fetch(`/check_solution/{{ problem.id }}`, {
// ... (previous fetch code)
})
.then(response => response.json())
.then(data => {
// ... (previous result handling code)
// Save attempt data
const allTestsPassed = data.every(result => result.status === 'Pass');
fetch(`/save_attempt/{{ problem.id }}`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
timeSpent: timeSpent,
passed: allTestsPassed,
timestamp: new Date().toISOString()
}),
});
})
.catch((error) => {
console.error('Error:', error);
});
});
const startTime = new Date(); // Record start time when the page loads
</script>
Step 9: Adding a Progress Dashboard
Finally, let’s create a dashboard where users can view their progress. Add a new route to app.py
:
@app.route('/dashboard')
def dashboard():
if 'attempts' not in session:
return render_template('dashboard.html', attempts={})
attempts = session['attempts']
problem_stats = {}
for problem_id, problem_attempts in attempts.items():
problem = next((p for p in problems if str(p["id"]) == problem_id), None)
if problem:
problem_stats[problem_id] = {
"title": problem["title"],
"attempts": len(problem_attempts),
"solved": any(attempt["passed"] for attempt in problem_attempts),
"best_time": min(attempt["timeSpent"] for attempt in problem_attempts) if problem_attempts else None
}
return render_template('dashboard.html', problem_stats=problem_stats)
Create a new template file dashboard.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Progress Dashboard - Coding Interview Simulator</title>
</head>
<body>
<h1>Your Progress</h1>
<table>
<tr>
<th>Problem</th>
<th>Attempts</th>
<th>Solved</th>
<th>Best Time (seconds)</th>
</tr>
{% for problem_id, stats in problem_stats.items() %}
<tr>
<td><a href="{{ url_for('problem', problem_id=problem_id) }}">{{ stats.title }}</a></td>
<td>{{ stats.attempts }}</td>
<td>{{ 'Yes' if stats.solved else 'No' }}</td>
<td>{{ stats.best_time|round(2) if stats.best_time else 'N/A' }}</td>
</tr>
{% endfor %}
</table>
<a href="{{ url_for('index') }}">Back to Problem List</a>
</body>
</html>
Finally, add a link to the dashboard in your index.html
file:
<!-- Add this below the problem list -->
<a href="{{ url_for('dashboard') }}">View Your Progress</a>
Conclusion
Congratulations! You’ve just built a basic coding interview simulator. This project not only helps you prepare for coding interviews but also enhances your web development skills. Here are some ways you can further improve your simulator:
- Add more problems: Expand your problem bank with a variety of questions covering different data structures and algorithms.
- Implement user authentication: Allow users to create accounts and save their progress across sessions.
- Improve code execution: Use a sandboxed environment for safer code execution.
- Add complexity analysis: Implement a system to analyze the time and space complexity of submitted solutions.
- Create a discussion forum: Allow users to discuss problems and share their approaches.
- Implement a rating system: Let users rate problems based on difficulty and quality.
- Add video explanations: Create or link to video explanations for each problem.
- Implement a recommendation system: Suggest problems based on the user’s performance and areas that need improvement.
Remember, the key to succeeding in coding interviews is consistent practice and a deep understanding of fundamental concepts. By creating and using this simulator, you’re not only preparing for interviews but also gaining valuable software development experience. Keep refining your skills, and you’ll be well-prepared for your next coding interview at a top tech company!