Insertion Sort: A Comprehensive Guide to Understanding and Implementing This Efficient Sorting Algorithm
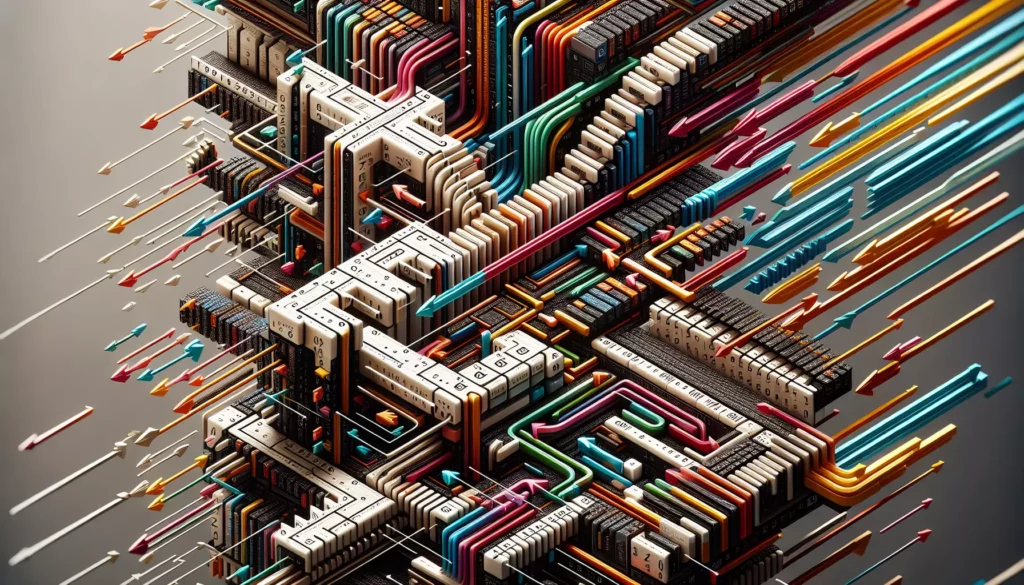
Sorting algorithms are fundamental in computer science and play a crucial role in organizing data efficiently. Among the various sorting techniques, insertion sort stands out as a simple yet effective method for arranging elements in a specific order. In this comprehensive guide, we’ll dive deep into the world of insertion sort, exploring its mechanics, implementation, time complexity, and real-world applications.
What is Insertion Sort?
Insertion sort is a comparison-based sorting algorithm that builds the final sorted array one element at a time. It iterates through an input array, removing one element at each iteration, and inserts it into its correct position in the sorted portion of the array. This process continues until all elements are sorted.
The algorithm is named “insertion sort” because it repeatedly takes an element from the unsorted portion and inserts it into its correct position in the sorted portion of the array.
How Insertion Sort Works
To understand the insertion sort algorithm, let’s break it down into steps:
- Start with the second element (index 1) of the array.
- Compare this element with the one before it.
- If the current element is smaller than the previous one, swap them.
- Continue comparing and swapping the current element with the previous elements until it’s in the correct position.
- Move to the next unsorted element and repeat steps 2-4 until the entire array is sorted.
Let’s visualize this process with an example:
Initial array: [5, 2, 4, 6, 1, 3]
Pass 1: [2, 5, 4, 6, 1, 3] (2 is inserted into its correct position)
Pass 2: [2, 4, 5, 6, 1, 3] (4 is inserted into its correct position)
Pass 3: [2, 4, 5, 6, 1, 3] (6 is already in its correct position)
Pass 4: [1, 2, 4, 5, 6, 3] (1 is inserted into its correct position)
Pass 5: [1, 2, 3, 4, 5, 6] (3 is inserted into its correct position)
Final sorted array: [1, 2, 3, 4, 5, 6]
Implementing Insertion Sort
Now that we understand how insertion sort works, let’s implement it in various programming languages.
Python Implementation
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and arr[j] > key:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
arr = [5, 2, 4, 6, 1, 3]
sorted_arr = insertion_sort(arr)
print(sorted_arr) # Output: [1, 2, 3, 4, 5, 6]
Java Implementation
public class InsertionSort {
public static void insertionSort(int[] arr) {
int n = arr.length;
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
public static void main(String[] args) {
int[] arr = {5, 2, 4, 6, 1, 3};
insertionSort(arr);
for (int num : arr) {
System.out.print(num + " ");
}
// Output: 1 2 3 4 5 6
}
}
JavaScript Implementation
function insertionSort(arr) {
for (let i = 1; i < arr.length; i++) {
let key = arr[i];
let j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
return arr;
}
// Example usage
const arr = [5, 2, 4, 6, 1, 3];
const sortedArr = insertionSort(arr);
console.log(sortedArr); // Output: [1, 2, 3, 4, 5, 6]
Time Complexity Analysis
Understanding the time complexity of insertion sort is crucial for determining its efficiency and suitability for different scenarios. Let’s break down the time complexity for different cases:
Best Case Scenario
The best case occurs when the input array is already sorted. In this scenario, the inner while loop doesn’t execute at all, and the algorithm only performs n-1 comparisons, where n is the number of elements in the array.
Time Complexity: O(n)
Average Case Scenario
In the average case, where the elements are randomly distributed, insertion sort makes approximately n²/4 comparisons and n²/4 swaps.
Time Complexity: O(n²)
Worst Case Scenario
The worst case occurs when the input array is sorted in reverse order. In this case, each element must be compared with every other element before it, resulting in the maximum number of comparisons and swaps.
Time Complexity: O(n²)
Space Complexity
Insertion sort is an in-place sorting algorithm, meaning it doesn’t require any extra space proportional to the input size. It only uses a constant amount of extra memory for variables.
Space Complexity: O(1)
Advantages of Insertion Sort
Despite its quadratic time complexity in the average and worst cases, insertion sort has several advantages that make it useful in certain scenarios:
- Simple implementation: Insertion sort is easy to understand and implement, making it a good choice for small projects or educational purposes.
- Efficient for small datasets: For small arrays or lists, insertion sort can outperform more complex algorithms due to its low overhead.
- Adaptive: Insertion sort performs well on partially sorted arrays, making it suitable for situations where the data is already nearly sorted.
- Stable sorting: Insertion sort maintains the relative order of equal elements, which is important in some applications.
- In-place sorting: It requires only a constant amount O(1) of additional memory space.
- Online algorithm: Insertion sort can sort a list as it receives it, making it useful for streaming data scenarios.
Disadvantages of Insertion Sort
While insertion sort has its merits, it also has some limitations:
- Inefficient for large datasets: Due to its quadratic time complexity, insertion sort becomes slow for large arrays or lists.
- Not suitable for reverse-sorted data: Insertion sort performs poorly when the input is sorted in reverse order, which is its worst-case scenario.
- Comparison-based: Like many simple sorting algorithms, insertion sort is comparison-based, which limits its lower bound to O(n log n) for the average and worst cases.
Variations and Optimizations
Several variations and optimizations of insertion sort have been developed to improve its performance or adapt it to specific scenarios:
1. Binary Insertion Sort
This variation uses binary search to find the correct position for inserting an element, reducing the number of comparisons needed. While it doesn’t improve the overall time complexity, it can be more efficient in practice, especially for larger datasets.
def binary_search(arr, val, start, end):
while start <= end:
mid = (start + end) // 2
if arr[mid] < val:
start = mid + 1
elif arr[mid] > val:
end = mid - 1
else:
return mid
return start
def binary_insertion_sort(arr):
for i in range(1, len(arr)):
val = arr[i]
j = binary_search(arr, val, 0, i - 1)
arr = arr[:j] + [val] + arr[j:i] + arr[i+1:]
return arr
# Example usage
arr = [5, 2, 4, 6, 1, 3]
sorted_arr = binary_insertion_sort(arr)
print(sorted_arr) # Output: [1, 2, 3, 4, 5, 6]
2. Shell Sort
Shell sort is a generalization of insertion sort that allows the exchange of items that are far apart. It starts by sorting pairs of elements far apart from each other, then progressively reducing the gap between elements to be compared. The last step is a simple insertion sort, but by then, the array is guaranteed to be almost sorted.
def shell_sort(arr):
n = len(arr)
gap = n // 2
while gap > 0:
for i in range(gap, n):
temp = arr[i]
j = i
while j >= gap and arr[j - gap] > temp:
arr[j] = arr[j - gap]
j -= gap
arr[j] = temp
gap //= 2
return arr
# Example usage
arr = [5, 2, 4, 6, 1, 3]
sorted_arr = shell_sort(arr)
print(sorted_arr) # Output: [1, 2, 3, 4, 5, 6]
3. Insertion Sort with Sentinel
This optimization eliminates the need for the boundary check in the inner loop by using a sentinel value. It can slightly improve performance, especially for larger arrays.
def insertion_sort_sentinel(arr):
n = len(arr)
sentinel = min(arr) - 1
arr = [sentinel] + arr
for i in range(2, n + 1):
j = i - 1
while arr[j] > arr[i]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = arr[i]
return arr[1:] # Remove the sentinel
# Example usage
arr = [5, 2, 4, 6, 1, 3]
sorted_arr = insertion_sort_sentinel(arr)
print(sorted_arr) # Output: [1, 2, 3, 4, 5, 6]
Real-world Applications of Insertion Sort
Despite its limitations for large datasets, insertion sort finds practical applications in various scenarios:
- Small dataset sorting: Insertion sort is often used as the sorting algorithm of choice for small arrays (typically less than 10-20 elements) in many programming language standard libraries.
- Partial sorting: When only a small portion of a large dataset needs to be sorted, insertion sort can be more efficient than more complex algorithms.
- Online sorting: In scenarios where data is received in real-time and needs to be sorted on-the-fly, insertion sort’s ability to handle streaming data makes it useful.
- Nearly sorted data: For datasets that are already almost sorted, insertion sort can be faster than more complex algorithms.
- Hybrid sorting algorithms: Insertion sort is often used as a subroutine in more advanced sorting algorithms, such as Introsort (used in many C++ standard library implementations).
- Educational purposes: Due to its simplicity, insertion sort is frequently taught in computer science courses to introduce sorting algorithms and algorithm analysis.
Comparison with Other Sorting Algorithms
To better understand insertion sort’s place among sorting algorithms, let’s compare it with some other popular sorting methods:
Insertion Sort vs. Bubble Sort
Both insertion sort and bubble sort have a worst-case and average time complexity of O(n²). However, insertion sort generally performs better in practice because:
- It makes fewer comparisons and swaps on average.
- It stops shifting elements as soon as it finds the right position, whereas bubble sort continues to the end of the array in each pass.
Insertion Sort vs. Selection Sort
Insertion sort and selection sort both have O(n²) time complexity, but insertion sort is usually preferred because:
- It performs better on partially sorted arrays.
- It’s adaptive, meaning its performance improves as the input array becomes more sorted.
- It’s stable, maintaining the relative order of equal elements.
Insertion Sort vs. Quicksort
Quicksort is generally faster than insertion sort, with an average-case time complexity of O(n log n). However, insertion sort can be preferable in certain situations:
- For very small arrays, insertion sort’s simplicity and low overhead can make it faster.
- Insertion sort is stable, while standard quicksort implementations are not.
- Insertion sort performs better on nearly sorted data.
Insertion Sort vs. Mergesort
Mergesort has a consistent O(n log n) time complexity, making it faster than insertion sort for larger datasets. However, insertion sort may be preferred when:
- Memory usage is a concern, as mergesort requires O(n) additional space.
- Dealing with small datasets or partially sorted arrays.
Best Practices and Tips for Using Insertion Sort
To make the most of insertion sort in your projects, consider the following best practices and tips:
- Use for small datasets: Insertion sort is most effective for arrays with fewer than 10-20 elements.
- Consider hybrid approaches: For larger datasets, consider using insertion sort as part of a hybrid algorithm, such as Introsort, which combines quicksort with insertion sort for small subarrays.
- Optimize for your specific use case: If you know your data is likely to be partially sorted, insertion sort can be a good choice.
- Implement binary insertion sort for larger arrays: If you need to use insertion sort on slightly larger arrays, consider implementing the binary insertion sort variation to reduce the number of comparisons.
- Use as a building block: Insertion sort can be an effective building block for more complex algorithms or data structures, such as maintaining sorted linked lists.
- Profile your code: When choosing between sorting algorithms, always profile your code with realistic data to ensure you’re making the best choice for your specific scenario.
Conclusion
Insertion sort is a simple yet powerful sorting algorithm that has stood the test of time. While it may not be the fastest option for large datasets, its simplicity, adaptability, and efficiency for small arrays make it a valuable tool in any programmer’s toolkit.
By understanding the mechanics, implementation, and characteristics of insertion sort, you can make informed decisions about when and how to use it in your projects. Whether you’re working on small-scale applications, dealing with nearly sorted data, or implementing more complex sorting algorithms, the principles of insertion sort provide a solid foundation for understanding and optimizing sorting processes.
As you continue to explore the world of algorithms and data structures, remember that each sorting method has its strengths and weaknesses. The key to becoming a proficient programmer is knowing when to apply each algorithm and how to optimize it for your specific needs. With insertion sort in your arsenal, you’re well-equipped to tackle a wide range of sorting challenges efficiently and effectively.