Infrastructure as Code (IaC): Revolutionizing DevOps with Terraform and Ansible
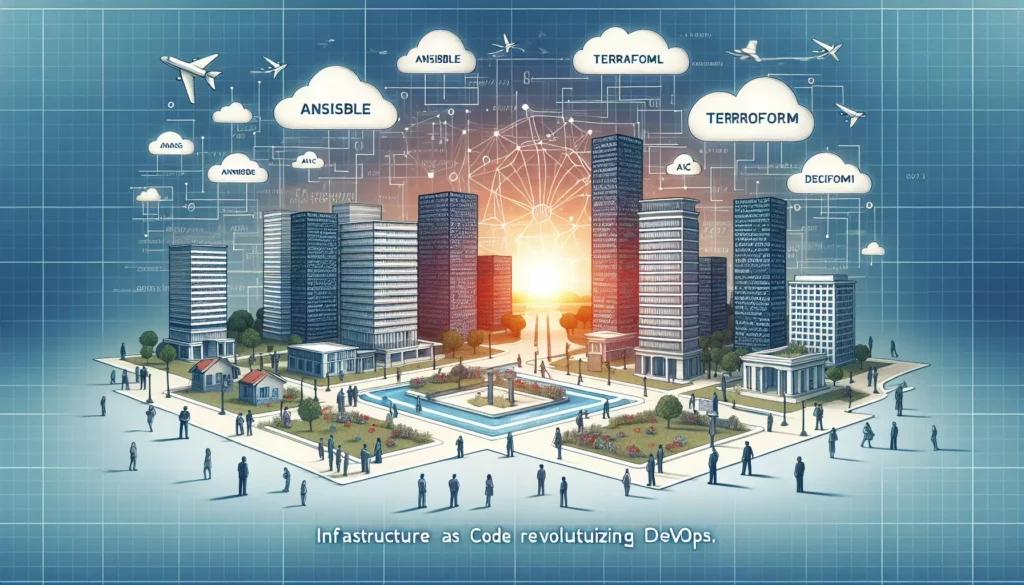
In the ever-evolving landscape of software development and IT operations, Infrastructure as Code (IaC) has emerged as a game-changing paradigm. As organizations strive for greater agility, scalability, and efficiency in their infrastructure management, IaC has become an indispensable tool in the DevOps toolkit. This comprehensive guide will delve into the world of Infrastructure as Code, with a particular focus on two powerful tools: Terraform and Ansible.
What is Infrastructure as Code (IaC)?
Infrastructure as Code is a practice in which infrastructure provisioning and management are handled through machine-readable definition files, rather than manual processes or interactive configuration tools. This approach allows developers and operations teams to manage and provision infrastructure using the same versioning, testing, and collaboration practices that they use for software code.
The key benefits of IaC include:
- Consistency and repeatability in infrastructure deployment
- Faster provisioning and scaling of resources
- Improved collaboration between development and operations teams
- Version control and change tracking for infrastructure
- Reduced human error and increased automation
- Cost savings through efficient resource utilization
Terraform: The Swiss Army Knife of Infrastructure Provisioning
Terraform, developed by HashiCorp, is an open-source IaC tool that has gained immense popularity in recent years. It allows users to define and provision infrastructure across multiple cloud providers and services using a declarative language.
Key Features of Terraform
- Cloud-agnostic: Works with various cloud providers (AWS, Azure, Google Cloud, etc.)
- Declarative syntax: Describe the desired end-state of your infrastructure
- Resource graph: Understands dependencies between resources
- Plan and apply workflow: Preview changes before applying them
- State management: Keeps track of the current state of your infrastructure
- Modular design: Reusable infrastructure components
Getting Started with Terraform
To begin using Terraform, you’ll need to install it on your local machine. Once installed, you can create your first Terraform configuration file, typically named main.tf
. Here’s a simple example that provisions an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
To apply this configuration, you would run the following commands:
terraform init
terraform plan
terraform apply
These commands initialize the Terraform working directory, create an execution plan, and apply the changes to create the specified infrastructure.
Advanced Terraform Concepts
As you become more comfortable with Terraform, you can explore more advanced concepts:
- Variables and outputs for flexible and reusable configurations
- Modules for encapsulating and sharing infrastructure components
- Workspaces for managing multiple environments
- Remote state storage for team collaboration
- Provisioners for executing scripts on resources after creation
Ansible: The Configuration Management Powerhouse
While Terraform excels at provisioning infrastructure, Ansible shines in the realm of configuration management and application deployment. Developed by Red Hat, Ansible is an open-source automation tool that uses a simple, human-readable language to describe system configurations and automate IT processes.
Key Features of Ansible
- Agentless architecture: No need to install software on managed nodes
- YAML-based playbooks: Easy-to-read and write configuration files
- Idempotent operations: Safe to run multiple times
- Extensive module library: Built-in support for various systems and services
- Dynamic inventory: Automatically discover and manage resources
- Role-based organization: Reusable and shareable configuration components
Getting Started with Ansible
To begin using Ansible, you’ll need to install it on your control node. Once installed, you can create your first Ansible playbook, typically named playbook.yml
. Here’s a simple example that installs and starts the Apache web server on a group of servers:
---
- name: Install and start Apache
hosts: webservers
become: yes
tasks:
- name: Install Apache
apt:
name: apache2
state: present
- name: Start Apache service
service:
name: apache2
state: started
enabled: yes
To run this playbook, you would use the following command:
ansible-playbook playbook.yml
This command executes the playbook, applying the specified configuration to the target hosts.
Advanced Ansible Concepts
As you become more proficient with Ansible, you can explore more advanced features:
- Roles for organizing and reusing playbooks
- Vault for encrypting sensitive data
- Dynamic inventories for cloud environments
- Handlers for triggering actions based on changes
- Templating with Jinja2 for dynamic configurations
Combining Terraform and Ansible: A Powerful IaC Duo
While Terraform and Ansible are powerful tools in their own right, combining them can create a comprehensive IaC solution that covers both infrastructure provisioning and configuration management.
Terraform for Infrastructure, Ansible for Configuration
A common pattern is to use Terraform to provision the underlying infrastructure (e.g., virtual machines, networks, storage) and then use Ansible to configure the provisioned resources (e.g., installing software, setting up services, deploying applications).
Here’s a high-level workflow:
- Use Terraform to create the necessary infrastructure resources
- Output the details of the created resources (e.g., IP addresses) from Terraform
- Use Ansible’s dynamic inventory to discover the newly created resources
- Run Ansible playbooks to configure the resources and deploy applications
Example: Provisioning and Configuring a Web Server
Let’s walk through a simple example that combines Terraform and Ansible to provision and configure a web server on AWS.
First, create a Terraform configuration file (main.tf
) to provision an EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "web_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
key_name = "your-key-pair"
tags = {
Name = "WebServer"
}
}
output "public_ip" {
value = aws_instance.web_server.public_ip
}
Apply this configuration using Terraform:
terraform init
terraform apply
Next, create an Ansible playbook (configure_webserver.yml
) to install and configure Apache:
---
- name: Configure Web Server
hosts: all
become: yes
tasks:
- name: Install Apache
apt:
name: apache2
state: present
- name: Start Apache service
service:
name: apache2
state: started
enabled: yes
- name: Create custom index.html
copy:
content: "<h1>Hello from Terraform and Ansible!</h1>"
dest: /var/www/html/index.html
Finally, run the Ansible playbook using the public IP output from Terraform:
ansible-playbook -i "$(terraform output -raw public_ip)," configure_webserver.yml -u ubuntu --private-key /path/to/your/key.pem
This command runs the Ansible playbook on the newly provisioned EC2 instance, configuring it as a web server.
Best Practices for Infrastructure as Code
To make the most of IaC tools like Terraform and Ansible, consider the following best practices:
- Version Control: Store your IaC configurations in a version control system like Git to track changes and collaborate with team members.
- Modularization: Break down your infrastructure into reusable modules or roles to promote code reuse and maintainability.
- Testing: Implement testing for your IaC configurations, including syntax checks, unit tests, and integration tests.
- CI/CD Integration: Incorporate your IaC workflows into your continuous integration and deployment pipelines.
- Security: Use tools like HashiCorp Vault or Ansible Vault to manage sensitive information securely.
- Documentation: Maintain clear and up-to-date documentation for your IaC configurations and processes.
- State Management: For Terraform, use remote state storage and state locking to enable team collaboration and prevent conflicts.
- Idempotency: Design your configurations to be idempotent, ensuring that repeated applications don’t cause unintended changes.
- Tagging and Naming Conventions: Implement consistent tagging and naming conventions for your resources to improve organization and cost management.
- Least Privilege: Follow the principle of least privilege when setting up permissions for your IaC tools and processes.
Challenges and Considerations
While Infrastructure as Code offers numerous benefits, it’s important to be aware of potential challenges:
- Learning Curve: IaC tools require time and effort to learn, especially for teams transitioning from manual processes.
- Tool Selection: Choosing the right tools for your specific needs and environment can be challenging given the variety of options available.
- State Management: Keeping infrastructure state in sync with the actual deployed resources can be complex, especially in large or rapidly changing environments.
- Scalability: As infrastructure grows, managing large IaC codebases can become challenging and require careful organization and modularization.
- Cultural Shift: Adopting IaC often requires a cultural shift within organizations, moving towards a more collaborative and automated approach to infrastructure management.
The Future of Infrastructure as Code
As technology continues to evolve, so too does the landscape of Infrastructure as Code. Some emerging trends and future directions include:
- GitOps: Tighter integration between IaC and Git workflows for continuous deployment and management of infrastructure.
- Policy as Code: Incorporating compliance and security policies directly into IaC workflows.
- AI and Machine Learning: Leveraging AI to optimize infrastructure configurations and predict potential issues.
- Multi-Cloud and Edge Computing: Enhanced support for managing infrastructure across diverse and distributed environments.
- Infrastructure as Software: Treating infrastructure more like software, with concepts like unit testing and continuous integration applied to infrastructure code.
Conclusion
Infrastructure as Code has revolutionized the way organizations manage and provision their IT resources. Tools like Terraform and Ansible have made it possible to treat infrastructure with the same rigor and best practices applied to software development. By embracing IaC, teams can achieve greater agility, consistency, and efficiency in their infrastructure management processes.
As you embark on your journey with Infrastructure as Code, remember that it’s not just about learning new tools, but about adopting a new mindset. Embrace the principles of automation, version control, and continuous improvement. Start small, experiment, and gradually expand your use of IaC as you become more comfortable with the concepts and tools.
Whether you’re a developer looking to better understand the infrastructure your applications run on, or an operations professional seeking to streamline your processes, mastering Infrastructure as Code is a valuable skill that will serve you well in the modern IT landscape. So dive in, start coding your infrastructure, and unlock the potential of this powerful approach to IT management.