In-Place Reversal of a Linked List: A Comprehensive Guide
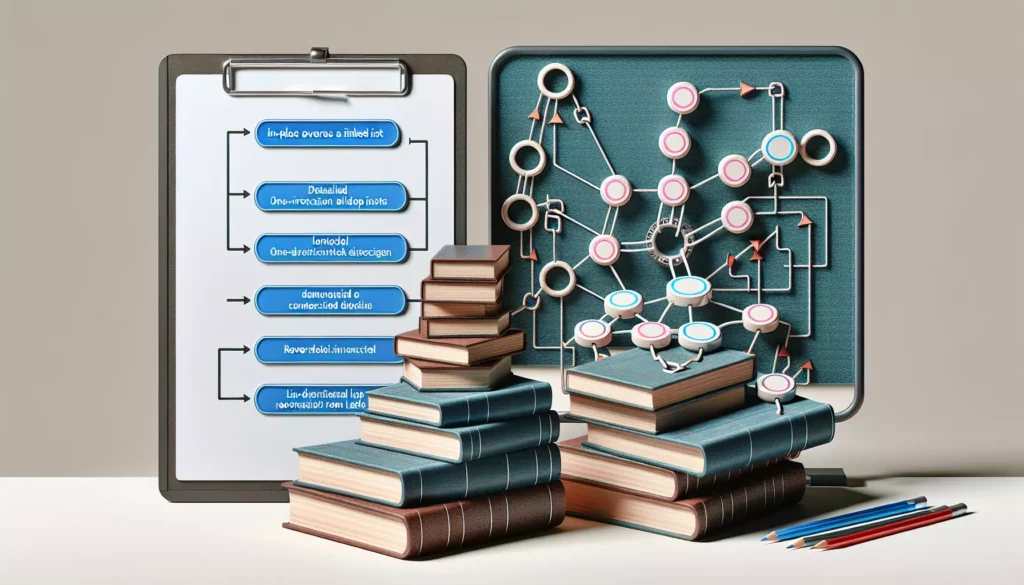
Linked lists are fundamental data structures in computer science, and understanding how to manipulate them efficiently is crucial for any programmer. One common operation on linked lists is reversing their order. In this comprehensive guide, we’ll explore the in-place reversal of a linked list, a technique that allows us to reverse the list without using additional data structures. This method is not only memory-efficient but also demonstrates a deep understanding of linked list operations.
Table of Contents
- Understanding Linked Lists
- Why In-Place Reversal?
- Algorithm Explanation
- Step-by-Step Walkthrough
- Code Implementation
- Time and Space Complexity
- Common Pitfalls and How to Avoid Them
- Variations and Related Problems
- Real-World Applications
- Interview Tips and Tricks
- Conclusion
1. Understanding Linked Lists
Before diving into the reversal process, let’s refresh our understanding of linked lists. A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence.
There are primarily two types of linked lists:
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has pointers to both the next and previous nodes.
For this tutorial, we’ll focus on singly linked lists, as the reversal process is more challenging and interesting for this type.
2. Why In-Place Reversal?
In-place reversal of a linked list is a technique where we reverse the direction of the links between nodes without using any additional data structure. This approach has several advantages:
- Space Efficiency: It doesn’t require extra space proportional to the size of the list.
- Time Efficiency: It can be done in a single pass through the list.
- Demonstrates Understanding: It shows a deep grasp of pointer manipulation and linked list concepts.
These qualities make in-place reversal a favorite topic in coding interviews, especially for companies like Google, Amazon, and Facebook.
3. Algorithm Explanation
The in-place reversal algorithm for a singly linked list follows these key steps:
- Initialize three pointers:
prev
as NULL,current
as the head of the list, andnext
as NULL. - Iterate through the list until
current
becomes NULL: - Store the next node:
next = current.next
- Reverse the current node’s pointer:
current.next = prev
- Move
prev
andcurrent
one step forward:prev = current
current = next
- Set the new head of the reversed list to
prev
.
This algorithm effectively reverses the direction of each link in the list, thereby reversing the entire list.
4. Step-by-Step Walkthrough
Let’s walk through the reversal process with a simple example. Consider a linked list: 1 → 2 → 3 → 4 → NULL
- Initial State:
prev = NULL
current = 1
(head of the list)next = NULL
(will be set in the loop)
- First Iteration:
- Set
next = current.next
(next = 2) - Reverse link:
current.next = prev
(1 → NULL) - Move
prev
tocurrent
(prev = 1) - Move
current
tonext
(current = 2)
Result: NULL ↠1 2 → 3 → 4 → NULL
- Set
- Second Iteration:
- Set
next = current.next
(next = 3) - Reverse link:
current.next = prev
(2 → 1) - Move
prev
tocurrent
(prev = 2) - Move
current
tonext
(current = 3)
Result: NULL ↠1 ↠2 3 → 4 → NULL
- Set
- Third Iteration:
- Set
next = current.next
(next = 4) - Reverse link:
current.next = prev
(3 → 2) - Move
prev
tocurrent
(prev = 3) - Move
current
tonext
(current = 4)
Result: NULL ↠1 ↠2 ↠3 4 → NULL
- Set
- Fourth Iteration:
- Set
next = current.next
(next = NULL) - Reverse link:
current.next = prev
(4 → 3) - Move
prev
tocurrent
(prev = 4) - Move
current
tonext
(current = NULL)
Result: NULL ↠1 ↠2 ↠3 ↠4
- Set
- Loop Ends:
current
is NULL, so we exit the loop. - Set New Head: The new head of the reversed list is
prev
, which is 4.
Final Result: 4 → 3 → 2 → 1 → NULL
5. Code Implementation
Now that we understand the algorithm, let’s implement it in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next = current.next
current.next = prev
prev = current
current = next
return prev # prev is now the new head
# Helper function to create a linked list from a list of values
def createLinkedList(values):
dummy = ListNode(0)
current = dummy
for val in values:
current.next = ListNode(val)
current = current.next
return dummy.next
# Helper function to convert a linked list to a list for easy printing
def linkedListToList(head):
result = []
current = head
while current:
result.append(current.val)
current = current.next
return result
# Test the reversal
original_list = createLinkedList([1, 2, 3, 4, 5])
reversed_list = reverseLinkedList(original_list)
print("Original list:", linkedListToList(original_list))
print("Reversed list:", linkedListToList(reversed_list))
This implementation includes helper functions to create a linked list from a list of values and to convert a linked list back to a list for easy printing. When you run this code, you should see the following output:
Original list: [1, 2, 3, 4, 5]
Reversed list: [5, 4, 3, 2, 1]
6. Time and Space Complexity
Understanding the time and space complexity of the in-place reversal algorithm is crucial for optimizing performance and resource usage:
Time Complexity: O(n)
The time complexity of this algorithm is O(n), where n is the number of nodes in the linked list. This is because we traverse the list exactly once, performing a constant number of operations for each node.
Space Complexity: O(1)
The space complexity is O(1), or constant space. We only use a fixed number of pointers (prev
, current
, and next
) regardless of the size of the input list. This is what makes the algorithm “in-place” – it doesn’t require additional space proportional to the input size.
This combination of linear time complexity and constant space complexity makes the in-place reversal algorithm highly efficient and desirable in many scenarios, especially when dealing with large lists or in memory-constrained environments.
7. Common Pitfalls and How to Avoid Them
When implementing the in-place reversal of a linked list, there are several common mistakes that programmers, especially beginners, might make. Being aware of these pitfalls can help you write more robust code and perform better in coding interviews:
1. Forgetting to Handle the Edge Cases
Pitfall: Not considering empty lists or lists with only one node.
Solution: Always check if the head is NULL or if there’s only one node before starting the main reversal logic.
def reverseLinkedList(head):
if not head or not head.next:
return head
# ... rest of the reversal logic
2. Losing the Reference to the Next Node
Pitfall: Changing current.next
before saving the reference to the next node.
Solution: Always store the next node in a variable before modifying current.next
.
next = current.next # Store next node
current.next = prev # Then modify current.next
3. Incorrect Loop Termination
Pitfall: Using the wrong condition to terminate the loop, which can lead to infinite loops or premature termination.
Solution: Ensure the loop continues as long as current
is not NULL.
while current is not None:
# Reversal logic
4. Forgetting to Update the Head
Pitfall: Not returning the new head of the reversed list.
Solution: Remember that after reversal, the last node becomes the new head. Return prev
at the end of the function.
return prev # prev is the new head after reversal
5. Modifying the Original List When It Shouldn’t Be Modified
Pitfall: In some cases, you might need to preserve the original list while returning a new reversed list.
Solution: If needed, create a deep copy of the list before reversing.
6. Not Handling Circular Lists
Pitfall: If there’s a possibility of circular lists, the basic algorithm might enter an infinite loop.
Solution: Implement a cycle detection mechanism if circular lists are a possibility in your use case.
8. Variations and Related Problems
The in-place reversal of a linked list is a fundamental problem that serves as a building block for many other linked list manipulations. Understanding this concept can help you solve various related problems. Here are some variations and related problems you might encounter:
1. Reverse a Linked List II
Problem: Reverse a linked list from position m to n. Do it in one-pass.
Variation: This problem requires you to reverse only a portion of the list, which adds complexity to the basic reversal algorithm.
2. Reverse Nodes in k-Group
Problem: Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.
Variation: This problem combines the concept of reversal with the idea of working on chunks of the list.
3. Palindrome Linked List
Problem: Determine if a linked list is a palindrome.
Application: You can use the reversal technique to solve this by reversing the second half of the list and comparing it with the first half.
4. Reorder List
Problem: Reorder a linked list in the pattern L0 → Ln → L1 → Ln-1 → L2 → Ln-2 → …
Application: This problem can be solved by finding the middle, reversing the second half, and then merging the two halves.
5. Swap Nodes in Pairs
Problem: Given a linked list, swap every two adjacent nodes and return its head.
Variation: This problem requires a similar thought process to reversal but with a different pattern.
6. Rotate List
Problem: Rotate a linked list to the right by k places.
Application: You can use the reversal technique as part of the solution to this problem.
Understanding how to reverse a linked list in-place provides you with a powerful tool to approach these and many other linked list problems. It’s often used as a subroutine in more complex list manipulations.
9. Real-World Applications
While reversing a linked list might seem like a purely academic exercise, it has several practical applications in real-world software development:
1. Undo Functionality
In applications that require an undo feature, like text editors or graphic design software, a linked list can be used to store the history of actions. Reversing part of this list can be used to implement the undo functionality.
2. Browser History
Web browsers use a similar concept to manage browsing history. The back and forward buttons essentially navigate a linked list of web pages, and reversing portions of this list can be useful in implementing certain navigation features.
3. Transaction Processing
In financial systems or databases, transactions are often stored in a linked list-like structure. Reversing these transactions can be part of a rollback mechanism in case of errors or system failures.
4. Network Packet Processing
In network programming, packets might need to be processed in reverse order of arrival for certain protocols or algorithms.
5. Memory Management
In some memory management systems, free memory blocks are maintained in a linked list. Reversing this list can be part of memory defragmentation or optimization processes.
6. Data Processing Pipelines
In data processing or ETL (Extract, Transform, Load) pipelines, reversing parts of the data flow can be necessary for certain transformations or to undo certain operations.
7. Blockchain Technology
While not a direct application of linked list reversal, the concept of linking blocks in a chain and the ability to traverse this chain in both directions is fundamental to blockchain technology.
8. Version Control Systems
Git and other version control systems use linked list-like structures to maintain the history of changes. The ability to reverse and manipulate these structures is crucial for features like reverting changes or creating branches.
Understanding and being able to implement linked list reversal is not just about solving a coding problem; it’s about grasping a fundamental concept that has wide-ranging applications in software development. This is why it’s such a popular topic in coding interviews, especially for companies that deal with large-scale systems and data processing.
10. Interview Tips and Tricks
When tackling the linked list reversal problem in a coding interview, keep these tips in mind to maximize your chances of success:
1. Clarify the Problem
- Ask if it’s a singly or doubly linked list.
- Confirm if in-place reversal is required or if you can use extra space.
- Check if there are any constraints on the values in the list.
2. Think Out Loud
Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
3. Start with the Brute Force Approach
If you’re stuck, start by explaining a simple solution, even if it’s not optimal. You can then work on optimizing it.
4. Draw It Out
Use diagrams to illustrate the reversal process. This can help both you and the interviewer visualize the solution.
5. Handle Edge Cases
Don’t forget to consider empty lists and lists with only one node. Mention these cases to the interviewer.
6. Optimize for Space
Emphasize that your solution uses O(1) space, which is often a key point of this problem.
7. Test Your Code
After implementing, walk through your code with a simple example to catch any bugs.
8. Discuss Time and Space Complexity
Be prepared to analyze and explain the time and space complexity of your solution.
9. Consider Follow-up Questions
Be ready for variations like reversing only a portion of the list or handling doubly linked lists.
10. Practice Implementation Without IDE Support
In interviews, you might not have access to an IDE. Practice implementing the solution on paper or a whiteboard.
11. Know Your Programming Language
Be familiar with the syntax for linked list operations in your chosen programming language.
12. Explain Real-World Applications
If asked, be ready to discuss where this algorithm might be used in real-world scenarios.
Remember, the goal in an interview is not just to solve the problem, but to demonstrate your problem-solving skills, coding ability, and communication skills. Stay calm, think logically, and don’t hesitate to ask for clarification if needed.
11. Conclusion
Mastering the in-place reversal of a linked list is a crucial skill for any programmer, particularly those aiming for positions at top tech companies. This problem encapsulates fundamental concepts of data structures, pointer manipulation, and algorithm optimization.
Throughout this guide, we’ve covered:
- The basics of linked lists and why in-place reversal is important
- A step-by-step explanation of the reversal algorithm
- Detailed code implementation in Python
- Analysis of time and space complexity
- Common pitfalls and how to avoid them
- Variations and related problems
- Real-world applications of this technique
- Interview tips for tackling this problem
Remember, the key to mastering this and similar problems is practice. Implement the solution multiple times, try the variations, and challenge yourself to optimize your code further. As you become more comfortable with linked list manipulations, you’ll find that many other data structure problems become more approachable.
In the world of software development, particularly in areas like systems programming, database management, and low-level networking, understanding how to efficiently manipulate data structures like linked lists is invaluable. The skills you develop solving problems like this will serve you well throughout your career, whether you’re optimizing critical systems or designing new algorithms.
Keep practicing, stay curious, and always look for opportunities to apply these concepts in real-world scenarios. With dedication and consistent effort, you’ll be well-prepared not just for coding interviews, but for the challenges of building complex, efficient software systems in your future career.