Improv Coding: Mastering On-the-Spot Problem Solving Through Theater Techniques
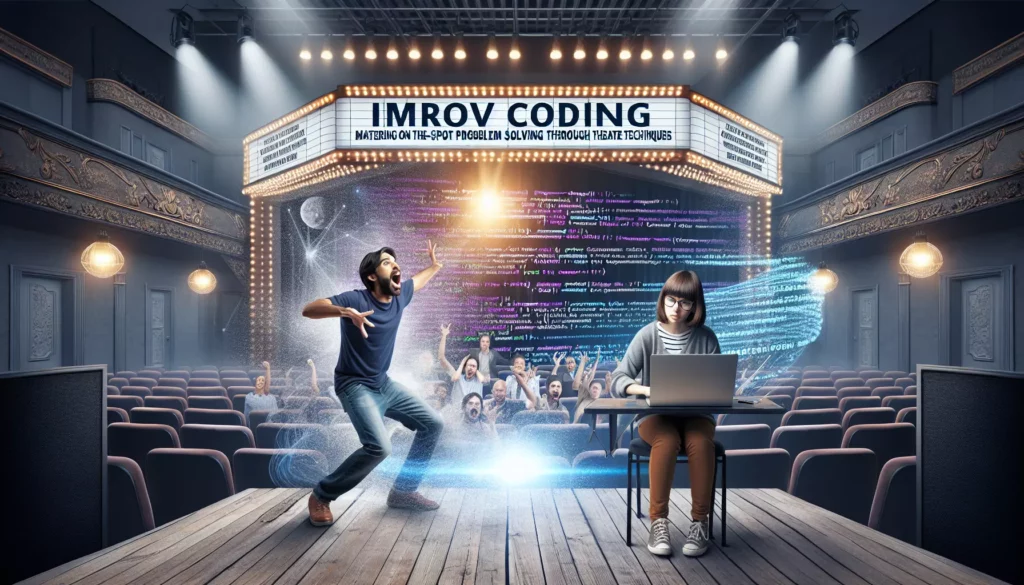
In the fast-paced world of software development, the ability to think on your feet and solve problems quickly is invaluable. While traditional coding education focuses on algorithms, data structures, and syntax, there’s a unique approach that can significantly enhance a programmer’s problem-solving skills: improv coding. By borrowing techniques from improvisational theater, developers can sharpen their ability to tackle unexpected challenges, collaborate effectively, and innovate in real-time. In this comprehensive guide, we’ll explore how improv techniques can revolutionize your coding practice and prepare you for the dynamic demands of technical interviews and real-world programming scenarios.
Understanding Improv Coding
Improv coding is the fusion of improvisational theater principles with software development practices. It’s about cultivating a mindset that embraces spontaneity, creativity, and adaptability in coding scenarios. Just as improv actors must think quickly and collaboratively on stage, programmers using improv techniques learn to approach coding challenges with flexibility and openness to new ideas.
Key Principles of Improv Coding:
- Embrace the unexpected
- Say “Yes, and…” to ideas
- Build on others’ contributions
- Stay present and focused
- Trust your instincts
These principles foster an environment where creativity flourishes, and problem-solving becomes a dynamic, collaborative process. By integrating these concepts into your coding practice, you can develop a more agile and responsive approach to software development.
The Benefits of Improv Coding
Incorporating improv techniques into your coding repertoire offers numerous advantages:
1. Enhanced Problem-Solving Skills
Improv coding sharpens your ability to think on your feet, allowing you to approach problems from multiple angles quickly. This skill is particularly valuable during technical interviews, where you may encounter unexpected challenges or need to pivot your approach mid-solution.
2. Improved Collaboration
The “Yes, and…” principle of improv encourages building on others’ ideas rather than dismissing them. This fosters a more collaborative coding environment, essential for pair programming and team projects.
3. Increased Creativity
By breaking away from rigid thinking patterns, improv coding helps you generate innovative solutions and think outside the box when tackling complex problems.
4. Better Adaptability
In the ever-evolving tech landscape, the ability to adapt quickly to new technologies and methodologies is crucial. Improv coding cultivates this adaptability, making you more resilient in the face of change.
5. Reduced Fear of Failure
Improv embraces mistakes as opportunities for creativity. This mindset can help programmers overcome the fear of writing imperfect code, encouraging experimentation and learning.
Improv Techniques for Coders
Let’s explore some specific improv techniques and how they can be applied to coding:
1. The “Yes, and…” Technique
In improv, actors are taught to accept and build upon their scene partners’ ideas using the phrase “Yes, and…”. In coding, this translates to:
- Embracing teammates’ suggestions during brainstorming sessions
- Building upon existing code rather than immediately rewriting it
- Exploring multiple solution paths before settling on one
Example scenario: During a code review, instead of immediately rejecting a colleague’s unconventional approach, consider how you might build upon or adapt their idea to improve the overall solution.
2. Character Development
Improv actors create characters on the spot. For coders, this translates to:
- Quickly understanding and embodying the perspective of different stakeholders (users, clients, team members)
- Adapting your coding style to match project requirements or team conventions
- Roleplaying different personas during user experience design
Example exercise: In your next project, try writing code from the perspective of a security-focused developer, then switch to a performance-oriented mindset. Notice how this shift in perspective influences your code design.
3. Scene Work
Improv scenes often start with a simple suggestion and evolve organically. In coding, this can be applied as:
- Starting with a basic code structure and iteratively adding complexity
- Pair programming sessions where developers take turns building on each other’s code
- Rapid prototyping sessions where ideas evolve quickly
Try this: Set a timer for 15 minutes and start with a basic function. Take turns with a partner adding features or optimizations, building on each other’s work without extensive planning.
4. Active Listening
Improv requires intense focus on scene partners. For coders, this means:
- Paying close attention during code reviews and team discussions
- Truly understanding user needs and client requirements
- Being receptive to feedback and new ideas
Practice active listening in your next team meeting by summarizing and building upon your colleagues’ ideas before presenting your own.
5. Embracing Failure
In improv, mistakes are often the source of the best material. Apply this to coding by:
- Viewing bugs as opportunities for learning and improvement
- Encouraging a culture where team members feel safe to take risks
- Using failed approaches as stepping stones to better solutions
Next time you encounter a bug, instead of getting frustrated, challenge yourself to find three potential learning opportunities from the experience.
Implementing Improv Coding in Your Practice
Now that we’ve explored the techniques, let’s look at how to incorporate improv coding into your daily practice:
1. Coding Warm-ups
Start your coding sessions with quick improv exercises to get your creative juices flowing:
- 60-second algorithm: Write a function to solve a simple problem in just one minute
- Random API challenge: Randomly select an API and create a mini-project around it in 15 minutes
- Code transformation: Take a piece of code and refactor it in three different ways in rapid succession
2. Pair Programming with a Twist
Enhance your pair programming sessions with improv elements:
- Role reversal: Swap roles (driver/navigator) every 5 minutes instead of at set intervals
- Constraint programming: Introduce random constraints (e.g., “no for loops allowed”) mid-session
- Storytelling code: Build a program where each function must continue a story started by the previous one
3. Improv Code Reviews
Transform code reviews into more dynamic, collaborative sessions:
- Yes, and… reviews: Reviewers must start each comment with “Yes, and…” to encourage building on ideas
- Live refactoring: Conduct reviews where the team improvises improvements in real-time
- Perspective shifts: Review code from different personas (e.g., a junior dev, a security expert, a performance guru)
4. Hackathons and Code Jams
Organize events that emphasize improvisation and rapid development:
- Theme roulette: Participants are given random themes to incorporate into their projects
- Tech stack shuffle: Teams must switch tech stacks midway through the event
- Collaborative chain coding: Each team member adds to the project for a set time before passing it on
5. Improv in Technical Interviews
Apply improv techniques to excel in technical interviews:
- Think aloud: Narrate your thought process as you work through problems
- Embrace constraints: If given limitations, use them as creative springboards
- Scenario adaptation: Practice solving problems with unexpected twists or changes in requirements
Improv Coding Exercises
To help you get started with improv coding, here are some exercises you can try:
1. The One-Line Wonder
Challenge: Write a complete, functional program in a single line of code. This exercise forces you to think creatively about syntax and program structure.
Example: Here’s a one-line Python program that generates a list of prime numbers up to 50:
print([x for x in range(2, 51) if all(x % y != 0 for y in range(2, int(x**0.5) + 1))])
2. The Language Leap
Challenge: Implement the same algorithm in three different programming languages within 30 minutes. This exercise improves your ability to think in multiple programming paradigms.
Example: Implement a binary search algorithm in Python, JavaScript, and Go:
# Python
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
// JavaScript
function binarySearch(arr, target) {
let left = 0, right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
else if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
// Go
func binarySearch(arr []int, target int) int {
left, right := 0, len(arr)-1
for left <= right {
mid := (left + right) / 2
if arr[mid] == target {
return mid
} else if arr[mid] < target {
left = mid + 1
} else {
right = mid - 1
}
}
return -1
}
3. The Refactor Race
Challenge: Take a poorly written piece of code and refactor it in as many ways as possible within 10 minutes. This exercise enhances your ability to quickly identify and implement improvements.
Example: Refactor this inefficient JavaScript function that finds the sum of even numbers in an array:
function sumEvenNumbers(numbers) {
var sum = 0;
for (var i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 == 0) {
sum = sum + numbers[i];
}
}
return sum;
}
Possible refactors:
// Using reduce
const sumEvenNumbers = numbers =>
numbers.reduce((sum, num) => num % 2 === 0 ? sum + num : sum, 0);
// Using filter and reduce
const sumEvenNumbers = numbers =>
numbers.filter(num => num % 2 === 0).reduce((sum, num) => sum + num, 0);
// Using for...of loop
function sumEvenNumbers(numbers) {
let sum = 0;
for (const num of numbers) {
if (num % 2 === 0) sum += num;
}
return sum;
}
4. The API Improvisation
Challenge: Given a random public API, create a small application that uses it in an unexpected way within 20 minutes. This exercise improves your ability to quickly understand and creatively use new tools.
Example: Use the Pokemon API to create a password generator:
async function generatePokemonPassword() {
const response = await fetch('https://pokeapi.co/api/v2/pokemon?limit=1000');
const data = await response.json();
const pokemon = data.results[Math.floor(Math.random() * data.results.length)];
const pokemonDetails = await fetch(pokemon.url).then(res => res.json());
const name = pokemon.name;
const id = pokemonDetails.id.toString().padStart(3, '0');
const type = pokemonDetails.types[0].type.name;
return `${name.charAt(0).toUpperCase() + name.slice(1)}${id}${type}!`;
}
generatePokemonPassword().then(password => console.log(password));
5. The Constraint Coding Challenge
Challenge: Solve a coding problem with a set of randomly assigned constraints. This exercise forces you to think creatively within limitations.
Example: Write a function to reverse a string with the following constraints:
- You can’t use any built-in reverse methods
- You can’t use a traditional for loop
- You must use at least one higher-order function
A possible solution:
const reverseString = str =>
str.split('').reduce((reversed, char) => char + reversed, '');
Overcoming Challenges in Improv Coding
While improv coding offers numerous benefits, it’s not without its challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Fear of Making Mistakes
Challenge: The spontaneity of improv can be intimidating, especially for perfectionists.
Solution: Embrace the improv mindset that mistakes are opportunities. Start with low-stakes exercises and gradually increase complexity. Celebrate creative solutions, even if they’re not perfect.
2. Balancing Speed and Quality
Challenge: The emphasis on quick thinking might lead to concerns about code quality.
Solution: Use improv coding for ideation and initial problem-solving, then follow up with structured refactoring. Implement code reviews to catch any issues that arise from rapid development.
3. Resistance from Traditional Developers
Challenge: Team members accustomed to more structured approaches might be skeptical of improv techniques.
Solution: Introduce improv coding gradually. Start with small exercises in team meetings or as optional activities. Demonstrate the benefits through improved problem-solving and creativity in your own work.
4. Maintaining Focus
Challenge: The free-flowing nature of improv can sometimes lead to tangents or loss of direction.
Solution: Set clear goals and time limits for improv sessions. Use techniques like timeboxing to maintain focus. Regularly regroup to ensure you’re still working towards the intended objective.
5. Integrating with Existing Methodologies
Challenge: Fitting improv coding into established development processes can be tricky.
Solution: Look for natural integration points, such as brainstorming sessions, hackathons, or exploratory coding phases. Use improv techniques to complement rather than replace existing methodologies.
The Future of Improv Coding
As the tech industry continues to evolve, the principles of improv coding are likely to become increasingly valuable. Here’s how improv coding might shape the future of software development:
1. Adaptive AI Pair Programming
Imagine AI coding assistants that can engage in improv-style pair programming, adapting their suggestions based on the developer’s coding style and thought process in real-time.
2. Dynamic Coding Interviews
Technical interviews may evolve to include more improv-style elements, assessing a candidate’s ability to adapt and innovate on the spot rather than just solving predefined problems.
3. Collaborative Coding Platforms
Future IDEs and coding platforms might incorporate features that facilitate improv coding techniques, such as real-time collaborative editing with built-in prompts for creative problem-solving.
4. Improv in Computer Science Education
CS curricula may start to incorporate improv techniques to develop students’ creativity and adaptability alongside traditional programming skills.
5. Cross-disciplinary Innovation
The principles of improv coding could inspire new approaches in other fields, leading to innovative problem-solving methods across various industries.
Conclusion
Improv coding is more than just a novel approach to software development; it’s a powerful tool for enhancing creativity, adaptability, and problem-solving skills in the ever-changing world of technology. By incorporating improv techniques into your coding practice, you can develop a more flexible and innovative approach to tackling complex problems, collaborating with team members, and navigating the challenges of technical interviews.
Remember, the goal of improv coding is not to replace traditional coding practices but to complement them. It’s about expanding your toolkit and developing a mindset that can help you thrive in unpredictable situations. As you integrate these techniques into your work, you’ll likely find that your ability to think on your feet, generate creative solutions, and adapt to new challenges improves significantly.
So, the next time you’re faced with a coding challenge, try channeling your inner improv artist. Embrace the unexpected, say “Yes, and…” to new ideas, and see where your creativity takes you. With practice, you’ll not only become a more versatile and resilient coder but also discover new joy and excitement in the art of programming.
Happy coding, and may your improv skills lead you to elegant solutions and breakthrough innovations!