Implementing Password Hashing Algorithms: A Comprehensive Guide
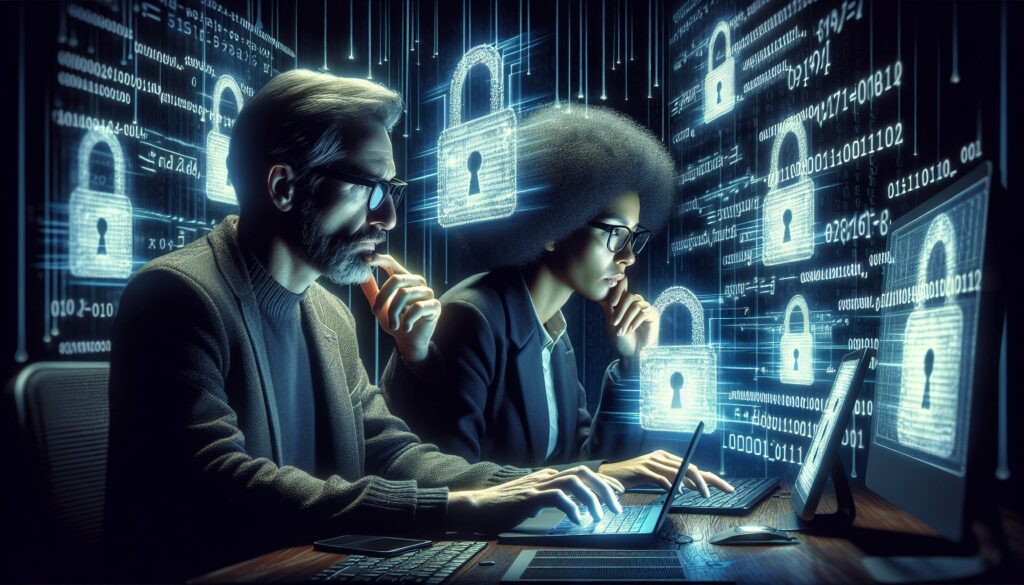
In the world of cybersecurity and user authentication, password hashing is a critical concept that every developer should understand and implement correctly. This article will dive deep into the importance of password hashing, explore various hashing algorithms, and provide practical examples of how to implement them in your applications.
Table of Contents
- Introduction to Password Hashing
- The Importance of Password Hashing
- Common Password Hashing Algorithms
- Implementing BCrypt
- Implementing Scrypt
- Implementing Argon2
- Best Practices for Password Hashing
- Comparing Hashing Algorithms
- Real-World Applications
- Conclusion
1. Introduction to Password Hashing
Password hashing is a fundamental security technique used to protect user credentials in databases. Instead of storing passwords in plain text, which would be a severe security risk, we use hashing algorithms to convert passwords into fixed-length strings of characters that are extremely difficult to reverse.
The basic process of password hashing works as follows:
- A user creates an account and provides a password.
- The password is passed through a hashing algorithm.
- The resulting hash is stored in the database instead of the original password.
- When the user attempts to log in, the provided password is hashed and compared to the stored hash.
- If the hashes match, the user is authenticated.
2. The Importance of Password Hashing
Password hashing is crucial for several reasons:
- Data Protection: In case of a data breach, hashed passwords are much harder for attackers to exploit than plain text passwords.
- Non-Reversibility: Good hashing algorithms are designed to be one-way functions, making it computationally infeasible to reverse the hash and obtain the original password.
- Compliance: Many data protection regulations and security standards require proper password storage techniques.
- User Privacy: Hashing helps protect user privacy by ensuring that even system administrators cannot see users’ actual passwords.
3. Common Password Hashing Algorithms
Several hashing algorithms have been developed over the years, each with its own strengths and weaknesses. Here are some of the most common ones:
MD5 (Message Digest algorithm 5)
MD5 is an older algorithm that produces a 128-bit hash value. However, it is no longer considered cryptographically secure due to vulnerabilities and should not be used for password hashing.
SHA (Secure Hash Algorithm) Family
The SHA family includes SHA-1, SHA-256, SHA-384, and SHA-512. While stronger than MD5, they are still vulnerable to certain types of attacks when used for password hashing without additional security measures.
BCrypt
BCrypt is a popular and secure password hashing algorithm. It incorporates a salt to protect against rainbow table attacks and is deliberately slow to compute, making brute-force attacks more difficult.
Scrypt
Scrypt is a password-based key derivation function that is designed to be computationally intensive, making it resistant to hardware-based attacks.
Argon2
Argon2 is a relatively new algorithm that won the Password Hashing Competition in 2015. It’s designed to be resistant to both CPU-intensive and memory-hard attacks.
4. Implementing BCrypt
BCrypt is widely used and supported in many programming languages. Let’s look at how to implement it in Python using the bcrypt
library.
Installation
First, install the bcrypt library:
pip install bcrypt
Hashing a Password
import bcrypt
def hash_password(password):
# Generate a salt
salt = bcrypt.gensalt()
# Hash the password
hashed = bcrypt.hashpw(password.encode('utf-8'), salt)
return hashed
# Example usage
password = "mysecretpassword"
hashed_password = hash_password(password)
print(f"Hashed password: {hashed_password}")
Verifying a Password
def verify_password(password, hashed):
return bcrypt.checkpw(password.encode('utf-8'), hashed)
# Example usage
password_to_check = "mysecretpassword"
is_correct = verify_password(password_to_check, hashed_password)
print(f"Password is correct: {is_correct}")
BCrypt automatically handles salting for you, which is one of its advantages. The salt is stored as part of the hashed password, so you don’t need to manage it separately.
5. Implementing Scrypt
Scrypt is another strong password hashing algorithm. Here’s how to implement it in Python using the scrypt
library.
Installation
pip install scrypt
Hashing a Password
import os
import scrypt
def hash_password_scrypt(password, salt=None):
if salt is None:
salt = os.urandom(32) # Generate a 32-byte random salt
hashed = scrypt.hash(password, salt, N=2**14, r=8, p=1, buflen=32)
return salt + hashed # Concatenate salt and hash
# Example usage
password = "mysecretpassword"
hashed_password = hash_password_scrypt(password)
print(f"Hashed password: {hashed_password.hex()}")
Verifying a Password
def verify_password_scrypt(password, stored_password):
salt = stored_password[:32] # Extract the salt
hashed = stored_password[32:] # Extract the hash
calculated_hash = scrypt.hash(password, salt, N=2**14, r=8, p=1, buflen=32)
return hashed == calculated_hash
# Example usage
password_to_check = "mysecretpassword"
is_correct = verify_password_scrypt(password_to_check, hashed_password)
print(f"Password is correct: {is_correct}")
Note that with Scrypt, we need to manage the salt ourselves. We generate a random salt and prepend it to the hash when storing, then extract it when verifying.
6. Implementing Argon2
Argon2 is the winner of the Password Hashing Competition and is considered one of the most secure algorithms available. Let’s implement it using the argon2-cffi
library in Python.
Installation
pip install argon2-cffi
Hashing a Password
from argon2 import PasswordHasher
def hash_password_argon2(password):
ph = PasswordHasher()
hash = ph.hash(password)
return hash
# Example usage
password = "mysecretpassword"
hashed_password = hash_password_argon2(password)
print(f"Hashed password: {hashed_password}")
Verifying a Password
from argon2 import PasswordHasher
from argon2.exceptions import VerifyMismatchError
def verify_password_argon2(password, hash):
ph = PasswordHasher()
try:
ph.verify(hash, password)
return True
except VerifyMismatchError:
return False
# Example usage
password_to_check = "mysecretpassword"
is_correct = verify_password_argon2(password_to_check, hashed_password)
print(f"Password is correct: {is_correct}")
Argon2 handles salt generation and storage automatically, similar to BCrypt. It also provides additional parameters to fine-tune memory usage, parallelism, and time cost.
7. Best Practices for Password Hashing
When implementing password hashing in your applications, consider the following best practices:
- Use Strong Algorithms: Stick to well-vetted algorithms like BCrypt, Scrypt, or Argon2. Avoid outdated algorithms like MD5 or SHA-1.
- Salt Your Hashes: Always use unique salts for each password. This prevents rainbow table attacks and ensures that identical passwords don’t produce the same hash.
- Tune Performance Parameters: Adjust the work factor or cost parameter to make the hashing process as slow as is acceptable for your application. This makes brute-force attacks more time-consuming.
- Keep Hashing Server-Side: Never hash passwords on the client-side (e.g., in JavaScript). Always perform hashing on the server.
- Use Secure Communications: Ensure that passwords are transmitted over secure, encrypted connections (HTTPS) to prevent interception.
- Implement Rate Limiting: Limit the number of login attempts to prevent brute-force attacks.
- Consider Key Stretching: Use key derivation functions that allow for multiple iterations to increase the time needed to compute a single hash.
- Keep Your Hashing Library Updated: Regularly update your hashing libraries to ensure you have the latest security patches.
8. Comparing Hashing Algorithms
Let’s compare the three algorithms we’ve implemented:
Algorithm | Pros | Cons |
---|---|---|
BCrypt |
|
|
Scrypt |
|
|
Argon2 |
|
|
The choice between these algorithms often depends on your specific requirements, the level of security needed, and the resources available on your system.
9. Real-World Applications
Password hashing is used in various real-world scenarios:
User Authentication Systems
Any application that requires user login functionality should implement password hashing. This includes social media platforms, e-commerce websites, and enterprise applications.
Password Managers
Password manager applications use strong hashing algorithms to secure the master password that unlocks all other stored passwords.
Cryptocurrency Wallets
Many cryptocurrency wallets use password hashing as part of their key derivation process to secure users’ private keys.
File Encryption Tools
Tools that encrypt files often use password hashing to derive encryption keys from user-provided passwords.
Database Security
Database management systems often use password hashing to secure administrative accounts and user credentials.
10. Conclusion
Implementing secure password hashing is a crucial aspect of building secure applications. By using modern algorithms like BCrypt, Scrypt, or Argon2, and following best practices, you can significantly enhance the security of your users’ credentials.
Remember that security is an ongoing process. Stay informed about the latest developments in cryptography and be prepared to upgrade your hashing methods as new vulnerabilities are discovered and more secure algorithms are developed.
As you continue to develop your programming skills, particularly in preparation for technical interviews at major tech companies, understanding these security concepts will be invaluable. Not only will it make you a more well-rounded developer, but it will also demonstrate your commitment to building secure and robust applications.
Practice implementing these hashing algorithms in your projects, and consider how you might explain the importance of password security in a technical interview setting. With a solid understanding of password hashing, you’ll be well-equipped to tackle security-related coding challenges and contribute to building safer digital ecosystems.