Implementing Kruskal’s and Prim’s Algorithms: A Comprehensive Guide
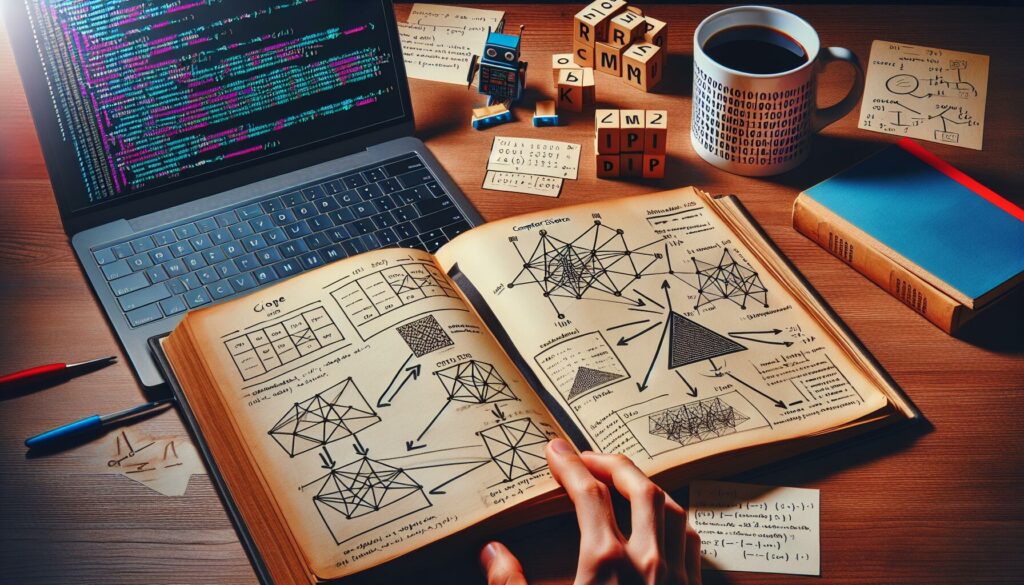
In the world of computer science and algorithm design, finding efficient ways to solve complex problems is paramount. Two such problems that often arise in various applications are finding the minimum spanning tree (MST) of a graph. Two popular algorithms for solving this problem are Kruskal’s algorithm and Prim’s algorithm. In this comprehensive guide, we’ll dive deep into these algorithms, understand their implementations, and explore their applications in real-world scenarios.
Understanding Minimum Spanning Trees
Before we delve into the algorithms, let’s first understand what a minimum spanning tree is. A minimum spanning tree of a weighted, undirected graph is a subset of the edges that connects all the vertices together, without any cycles, and with the minimum possible total edge weight. In simpler terms, it’s the cheapest way to connect all nodes in a graph.
MSTs have numerous practical applications, including:
- Network design (e.g., laying out electrical wiring or computer networks)
- Approximation algorithms for NP-hard problems like the traveling salesman problem
- Cluster analysis in data mining
- Image segmentation in computer vision
Kruskal’s Algorithm
Kruskal’s algorithm is a greedy algorithm that finds a minimum spanning tree for a weighted undirected graph. It works by sorting all the edges from lowest weight to highest weight and then adding the edges one by one to the MST, skipping any edge that would create a cycle.
Algorithm Steps:
- Sort all edges in non-decreasing order of their weight.
- Pick the smallest edge. Check if it forms a cycle with the spanning tree formed so far. If not, include this edge. Else, discard it.
- Repeat step 2 until there are (V-1) edges in the spanning tree, where V is the number of vertices.
Implementation in Python
Let’s implement Kruskal’s algorithm in Python:
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal_mst(graph):
edges = [(w, u, v) for u, edges in graph.items() for v, w in edges.items()]
edges.sort()
vertices = list(graph.keys())
ds = DisjointSet(vertices)
mst = []
for w, u, v in edges:
if ds.find(u) != ds.find(v):
ds.union(u, v)
mst.append((u, v, w))
return mst
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
mst = kruskal_mst(graph)
print("Minimum Spanning Tree:", mst)
In this implementation, we use a Disjoint Set data structure to efficiently check for cycles and perform unions. The time complexity of Kruskal’s algorithm is O(E log E) or O(E log V), where E is the number of edges and V is the number of vertices.
Prim’s Algorithm
Prim’s algorithm is another greedy algorithm for finding the minimum spanning tree of a weighted undirected graph. Unlike Kruskal’s algorithm, which builds the MST by adding edges, Prim’s algorithm builds the MST by adding vertices.
Algorithm Steps:
- Initialize a tree with a single vertex, chosen arbitrarily from the graph.
- Grow the tree by one edge: of the edges that connect the tree to vertices not yet in the tree, find the minimum-weight edge, and transfer it to the tree.
- Repeat step 2 until all vertices are in the tree.
Implementation in Python
Here’s an implementation of Prim’s algorithm in Python:
import heapq
def prim_mst(graph):
start_vertex = next(iter(graph))
mst = []
visited = set([start_vertex])
edges = [(w, start_vertex, v) for v, w in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
w, u, v = heapq.heappop(edges)
if v not in visited:
visited.add(v)
mst.append((u, v, w))
for next_vertex, next_weight in graph[v].items():
if next_vertex not in visited:
heapq.heappush(edges, (next_weight, v, next_vertex))
return mst
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
mst = prim_mst(graph)
print("Minimum Spanning Tree:", mst)
In this implementation, we use a priority queue (implemented with a heap) to efficiently select the minimum-weight edge at each step. The time complexity of Prim’s algorithm is O((V + E) log V) when using a binary heap, where V is the number of vertices and E is the number of edges.
Comparison of Kruskal’s and Prim’s Algorithms
Both Kruskal’s and Prim’s algorithms are efficient methods for finding the minimum spanning tree of a graph. However, they have some differences that make each more suitable for certain types of graphs:
Aspect | Kruskal’s Algorithm | Prim’s Algorithm |
---|---|---|
Approach | Edge-based | Vertex-based |
Time Complexity | O(E log E) or O(E log V) | O((V + E) log V) with binary heap |
Better for | Sparse graphs | Dense graphs |
Data Structure | Disjoint Set | Priority Queue |
Applications and Real-World Examples
Minimum Spanning Tree algorithms have numerous practical applications across various domains. Let’s explore some real-world examples:
1. Network Design
In telecommunications and computer networking, MST algorithms are used to design efficient network topologies. For example, when laying out cables for a new office building, using an MST algorithm can help minimize the total length of cable required while ensuring all computers are connected.
2. Transportation Systems
In transportation planning, MST algorithms can be used to design efficient road networks or public transit systems. By modeling intersections as vertices and roads as edges (with weights representing distances or construction costs), planners can use these algorithms to find the most cost-effective way to connect all points of interest.
3. Image Segmentation
In computer vision and image processing, MST algorithms can be used for image segmentation. By representing pixels as vertices and the differences between neighboring pixels as edge weights, an MST can help identify distinct regions or objects within an image.
4. Clustering
In data mining and machine learning, MST algorithms can be used for clustering. By constructing an MST of data points and then removing the longest edges, we can create clusters of similar data points.
5. Circuit Design
In electronic circuit design, MST algorithms can help optimize the layout of components and connections on a printed circuit board (PCB), minimizing the total length of connections while ensuring all components are connected.
Advanced Considerations
While Kruskal’s and Prim’s algorithms are powerful tools for finding minimum spanning trees, there are some advanced considerations and variations to keep in mind:
1. Handling Disconnected Graphs
The basic implementations we’ve discussed assume that the input graph is connected. For disconnected graphs, you might need to modify the algorithms to handle multiple components. This could involve running the algorithm multiple times, once for each connected component.
2. Parallel and Distributed Implementations
For very large graphs, parallelized versions of these algorithms can be implemented to improve performance. For example, Borůvka’s algorithm, another MST algorithm, is more amenable to parallelization than Kruskal’s or Prim’s.
3. Dynamic Graphs
In some applications, the graph structure may change over time. There are variations of MST algorithms designed to efficiently update the minimum spanning tree as edges are added or removed from the graph, rather than recomputing the entire MST from scratch.
4. Approximate MSTs
For extremely large graphs where even O(E log V) complexity is too slow, there are approximate algorithms that can find a spanning tree that is close to minimum in less time.
Conclusion
Kruskal’s and Prim’s algorithms are fundamental tools in the field of graph theory and have wide-ranging applications in computer science and beyond. Understanding these algorithms and their implementations is crucial for any programmer or computer scientist dealing with network optimization problems.
As we’ve seen, each algorithm has its strengths and is better suited to different types of graphs. Kruskal’s algorithm, with its edge-based approach, is often preferred for sparse graphs, while Prim’s algorithm, with its vertex-based approach, can be more efficient for dense graphs.
By mastering these algorithms, you’ll be well-equipped to tackle a variety of real-world problems, from network design and transportation planning to image processing and data clustering. As you continue to explore the world of algorithms and data structures, remember that these fundamental concepts often serve as building blocks for more complex and specialized algorithms.
Keep practicing, experimenting with different graph structures, and considering how these algorithms might be applied to problems in your own field of interest. With time and experience, you’ll develop the intuition to choose the right algorithm for each unique problem you encounter.