Implementing Efficient File Compression Techniques: A Comprehensive Guide
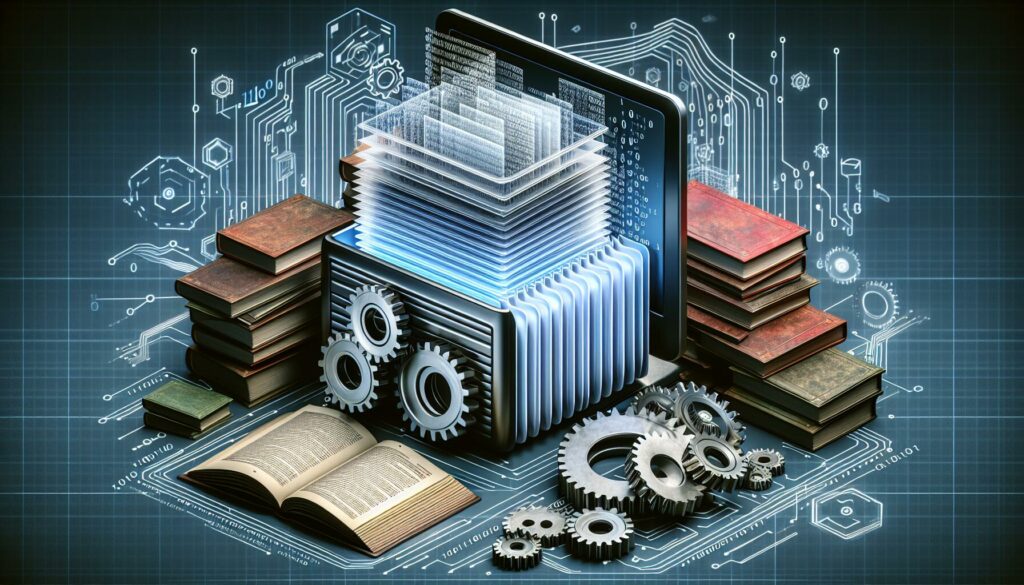
In the world of digital data and storage optimization, file compression techniques play a crucial role in reducing file sizes, saving storage space, and improving data transfer speeds. As aspiring software engineers and developers, understanding and implementing efficient file compression algorithms is an essential skill that can significantly enhance your programming toolkit. In this comprehensive guide, we’ll explore various file compression techniques, their underlying principles, and provide practical implementations to help you master this important aspect of computer science.
Table of Contents
- Introduction to File Compression
- Lossless Compression Techniques
- Lossy Compression Techniques
- Practical Implementations
- Performance Comparison
- Best Practices and Considerations
- Conclusion
1. Introduction to File Compression
File compression is the process of encoding information using fewer bits than the original representation. This technique is used to reduce the size of files, which in turn saves storage space and decreases data transmission time. Compression algorithms can be broadly categorized into two types: lossless and lossy compression.
Lossless compression allows the original data to be perfectly reconstructed from the compressed data. This type of compression is ideal for text files, executable programs, and other data where losing even a single bit of information could be catastrophic.
Lossy compression, on the other hand, reduces file size by permanently eliminating certain information, especially redundant information. This type of compression is commonly used for multimedia files like images, audio, and video, where some loss of quality is acceptable in exchange for significantly smaller file sizes.
2. Lossless Compression Techniques
Run-Length Encoding (RLE)
Run-Length Encoding is one of the simplest forms of data compression. It works by replacing sequences of identical data elements (runs) with a single data value and a count. This method is particularly effective for files with long runs of repeated data.
For example, the string “AABBBCCCC” would be encoded as “2A3B4C”.
Huffman Coding
Huffman coding is a popular algorithm for lossless data compression. It assigns variable-length codes to characters based on their frequencies of occurrence. More frequent characters are assigned shorter codes, while less frequent characters receive longer codes. This results in an overall reduction in the number of bits required to represent the data.
The algorithm works by building a binary tree (Huffman tree) based on the frequency of each character in the input. The path from the root to a leaf node represents the Huffman code for that character.
LZW Compression
Lempel-Ziv-Welch (LZW) compression is a dictionary-based algorithm that builds a dictionary of substrings dynamically as it compresses the data. It’s particularly effective for text and other data with repeated patterns.
The algorithm starts with a dictionary of single characters and progressively adds new substrings to the dictionary as it encounters them. It then replaces these substrings with their dictionary indices in the compressed output.
3. Lossy Compression Techniques
Transform Coding
Transform coding is a type of data compression for “lossy” compression. It’s commonly used in digital audio, images, and video compression. The process involves transforming the input data into a different domain (e.g., frequency domain) where it can be more efficiently represented and compressed.
A popular example of transform coding is the Discrete Cosine Transform (DCT) used in JPEG image compression. The DCT transforms image data from the spatial domain to the frequency domain, where less important high-frequency components can be discarded, resulting in smaller file sizes with minimal perceptible loss in image quality.
Vector Quantization
Vector quantization is a technique used to compress data by mapping a large set of points (vectors) to a smaller set of points. It’s often used in image and speech compression.
The algorithm works by dividing the input data into vectors and then mapping each vector to the nearest vector in a predefined codebook. Only the index of the codebook vector is stored, resulting in compression. During decompression, the index is used to look up the corresponding vector in the codebook.
4. Practical Implementations
Now that we’ve covered the theoretical aspects of various compression techniques, let’s dive into practical implementations of some of these algorithms.
Implementing Run-Length Encoding
Here’s a simple implementation of Run-Length Encoding in Python:
def run_length_encode(data):
encoded = []
count = 1
for i in range(1, len(data)):
if data[i] == data[i-1]:
count += 1
else:
encoded.append((data[i-1], count))
count = 1
encoded.append((data[-1], count))
return encoded
def run_length_decode(encoded):
return ''.join(char * count for char, count in encoded)
# Example usage
original = "AABBBCCCC"
encoded = run_length_encode(original)
decoded = run_length_decode(encoded)
print(f"Original: {original}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
This implementation encodes the input string into a list of tuples, where each tuple contains a character and its run length. The decoding function reconstructs the original string from this encoded format.
Implementing Huffman Coding
Implementing Huffman coding is more complex. Here’s a basic implementation in Python:
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
# Count frequency of each character
frequency = defaultdict(int)
for char in text:
frequency[char] += 1
# Create a priority queue to store nodes
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
# Build the Huffman tree
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
internal = Node(None, left.freq + right.freq)
internal.left = left
internal.right = right
heapq.heappush(heap, internal)
return heap[0]
def build_codes(root, current_code, codes):
if root is None:
return
if root.char is not None:
codes[root.char] = current_code
return
build_codes(root.left, current_code + "0", codes)
build_codes(root.right, current_code + "1", codes)
def huffman_encode(text):
root = build_huffman_tree(text)
codes = {}
build_codes(root, "", codes)
encoded = "".join(codes[char] for char in text)
return encoded, codes
def huffman_decode(encoded, codes):
reversed_codes = {code: char for char, code in codes.items()}
decoded = ""
current_code = ""
for bit in encoded:
current_code += bit
if current_code in reversed_codes:
decoded += reversed_codes[current_code]
current_code = ""
return decoded
# Example usage
text = "this is an example for huffman encoding"
encoded, codes = huffman_encode(text)
decoded = huffman_decode(encoded, codes)
print(f"Original: {text}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
print(f"Compression ratio: {len(encoded) / (len(text) * 8):.2f}")
This implementation builds a Huffman tree, generates Huffman codes for each character, and then uses these codes to encode and decode the input text.
Implementing LZW Compression
Here’s a basic implementation of LZW compression in Python:
def lzw_compress(text):
dictionary = {chr(i): i for i in range(256)}
next_code = 256
result = []
current_string = ""
for char in text:
current_string += char
if current_string not in dictionary:
result.append(dictionary[current_string[:-1]])
dictionary[current_string] = next_code
next_code += 1
current_string = char
if current_string:
result.append(dictionary[current_string])
return result
def lzw_decompress(compressed):
dictionary = {i: chr(i) for i in range(256)}
next_code = 256
result = ""
current_string = chr(compressed[0])
result += current_string
for code in compressed[1:]:
if code in dictionary:
entry = dictionary[code]
elif code == next_code:
entry = current_string + current_string[0]
else:
raise ValueError("Invalid compressed code")
result += entry
dictionary[next_code] = current_string + entry[0]
next_code += 1
current_string = entry
return result
# Example usage
text = "TOBEORNOTTOBEORTOBEORNOT"
compressed = lzw_compress(text)
decompressed = lzw_decompress(compressed)
print(f"Original: {text}")
print(f"Compressed: {compressed}")
print(f"Decompressed: {decompressed}")
print(f"Compression ratio: {len(compressed) * 16 / (len(text) * 8):.2f}")
This implementation builds a dictionary of substrings dynamically during compression and uses this dictionary to encode the input text. During decompression, it reconstructs the dictionary to recover the original text.
5. Performance Comparison
When it comes to choosing the right compression technique for your specific use case, it’s important to consider various factors such as compression ratio, encoding/decoding speed, and the nature of your data. Let’s compare the performance of the compression techniques we’ve implemented:
- Run-Length Encoding (RLE):
- Pros: Simple to implement, fast encoding and decoding.
- Cons: Only effective for data with long runs of repeated characters.
- Best for: Binary images, simple graphics with large uniform areas.
- Huffman Coding:
- Pros: Provides good compression for a wide range of data types.
- Cons: Requires two passes over the data (one to calculate frequencies, one to encode).
- Best for: Text files, especially those with varying character frequencies.
- LZW Compression:
- Pros: Adaptive algorithm that can handle a variety of data types well.
- Cons: Can be less effective for very short inputs or highly random data.
- Best for: Text files, especially those with repeated patterns or phrases.
To properly compare these algorithms, you should test them on various types and sizes of data relevant to your specific use case. Measure factors such as:
- Compression ratio (compressed size / original size)
- Encoding time
- Decoding time
- Memory usage during compression and decompression
Here’s a simple Python script to compare these metrics for our implemented algorithms:
import time
import sys
def compare_compression_algorithms(text):
print(f"Original text size: {sys.getsizeof(text)} bytes")
# RLE
start_time = time.time()
rle_encoded = run_length_encode(text)
rle_encode_time = time.time() - start_time
start_time = time.time()
rle_decoded = run_length_decode(rle_encoded)
rle_decode_time = time.time() - start_time
rle_size = sys.getsizeof(rle_encoded)
# Huffman
start_time = time.time()
huffman_encoded, huffman_codes = huffman_encode(text)
huffman_encode_time = time.time() - start_time
start_time = time.time()
huffman_decoded = huffman_decode(huffman_encoded, huffman_codes)
huffman_decode_time = time.time() - start_time
huffman_size = sys.getsizeof(huffman_encoded) + sys.getsizeof(huffman_codes)
# LZW
start_time = time.time()
lzw_compressed = lzw_compress(text)
lzw_encode_time = time.time() - start_time
start_time = time.time()
lzw_decompressed = lzw_decompress(lzw_compressed)
lzw_decode_time = time.time() - start_time
lzw_size = sys.getsizeof(lzw_compressed)
print("\nCompression Results:")
print(f"RLE - Size: {rle_size} bytes, Encode time: {rle_encode_time:.6f}s, Decode time: {rle_decode_time:.6f}s")
print(f"Huffman - Size: {huffman_size} bytes, Encode time: {huffman_encode_time:.6f}s, Decode time: {huffman_decode_time:.6f}s")
print(f"LZW - Size: {lzw_size} bytes, Encode time: {lzw_encode_time:.6f}s, Decode time: {lzw_decode_time:.6f}s")
# Example usage
sample_text = "This is a sample text to test our compression algorithms. " * 100
compare_compression_algorithms(sample_text)
Run this script with different types and sizes of input data to get a better understanding of how each algorithm performs in various scenarios.
6. Best Practices and Considerations
When implementing and using file compression techniques in your projects, consider the following best practices and considerations:
- Choose the right algorithm for your data: Different compression algorithms work better for different types of data. Analyze your data characteristics and choose accordingly.
- Balance compression ratio and speed: Higher compression ratios often come at the cost of longer encoding/decoding times. Choose a balance that suits your application’s needs.
- Consider memory usage: Some algorithms (like LZW) can consume significant memory for large inputs. Be mindful of memory constraints in your environment.
- Test with real-world data: Always test your compression implementation with realistic data sets to ensure it performs as expected in production scenarios.
- Error handling and data integrity: Implement proper error handling and checksums to ensure data integrity during compression and decompression.
- Parallel processing: For large data sets, consider implementing parallel compression/decompression to take advantage of multi-core processors.
- Streaming support: If your application deals with continuous data streams, adapt your implementation to support streaming compression and decompression.
- Compression levels: For some algorithms, you can implement different levels of compression, allowing users to choose between faster compression or better compression ratios.
- Security considerations: Be aware that compression can sometimes lead to security vulnerabilities (e.g., the CRIME attack). Take appropriate precautions when compressing sensitive data.
- Licensing and patents: Be aware of any licensing or patent issues related to the compression algorithms you use, especially in commercial applications.
7. Conclusion
File compression is a fundamental concept in computer science and software engineering. Understanding and implementing efficient file compression techniques can significantly improve your skills as a programmer and open up new possibilities in data storage and transmission optimization.
In this comprehensive guide, we’ve explored various lossless and lossy compression techniques, including Run-Length Encoding, Huffman Coding, and LZW Compression. We’ve provided practical implementations of these algorithms in Python and discussed their strengths, weaknesses, and suitable use cases.
As you continue to develop your skills, consider exploring more advanced compression algorithms like DEFLATE (used in ZIP files), LZMA (used in 7z files), or domain-specific compression techniques for images, audio, and video. Additionally, stay updated with the latest developments in compression technology, as new algorithms and techniques are continually being developed to meet the ever-growing demands of data storage and transmission.
Remember that mastering file compression techniques not only enhances your technical skills but also contributes to creating more efficient and optimized software solutions. As you prepare for technical interviews and real-world programming challenges, the knowledge and experience gained from implementing these algorithms will prove invaluable.
Keep practicing, experimenting with different data types and sizes, and always strive to understand the underlying principles behind these algorithms. With dedication and continuous learning, you’ll be well-equipped to tackle complex data management challenges and stand out as a skilled software engineer in the competitive tech industry.