Implementing Countdown Timers with Algorithms: A Comprehensive Guide
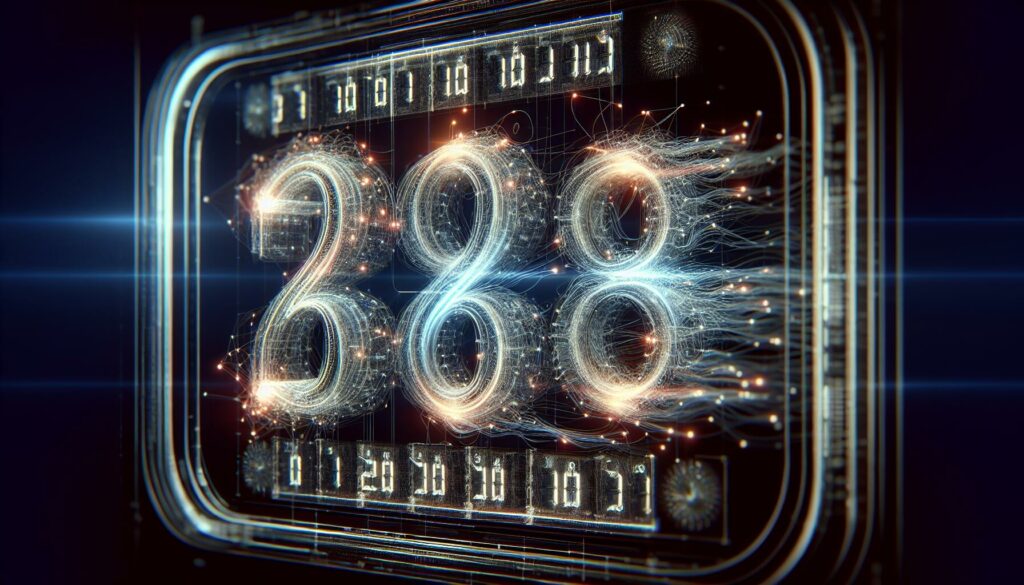
Countdown timers are ubiquitous in modern web applications and software. From e-commerce sites creating urgency for limited-time offers to productivity apps helping users manage their time effectively, countdown timers serve various purposes. In this comprehensive guide, we’ll explore the intricacies of implementing countdown timers using algorithms, covering everything from basic concepts to advanced techniques and optimizations.
Table of Contents
- Understanding Countdown Timers
- Basic Countdown Algorithm
- Implementing a Countdown Timer in JavaScript
- Advanced Techniques and Optimizations
- Real-World Applications of Countdown Timers
- Common Challenges and Solutions
- Testing and Debugging Countdown Timers
- Future Trends in Countdown Timer Implementations
- Conclusion
1. Understanding Countdown Timers
Before diving into the implementation details, it’s crucial to understand what countdown timers are and how they function. A countdown timer is a digital or analog device that counts down from a specified time interval to zero. In programming, it’s typically implemented as a function or class that keeps track of the remaining time and updates the display at regular intervals.
Key components of a countdown timer include:
- Start time: The initial time from which the countdown begins
- End time: The target time when the countdown should reach zero
- Current time: The continuously updating time as the countdown progresses
- Display: The visual representation of the remaining time
- Update mechanism: The method by which the timer refreshes and recalculates the remaining time
2. Basic Countdown Algorithm
The fundamental algorithm for a countdown timer is relatively straightforward. Here’s a high-level overview of the steps involved:
- Set the target end time
- Get the current time
- Calculate the time difference between the current time and the end time
- Convert the time difference into the desired format (e.g., days, hours, minutes, seconds)
- Display the formatted time
- Wait for a short interval (e.g., 1 second)
- Repeat steps 2-6 until the current time reaches or exceeds the end time
This basic algorithm forms the foundation for most countdown timer implementations. However, real-world applications often require more sophisticated approaches to handle various edge cases and optimize performance.
3. Implementing a Countdown Timer in JavaScript
Let’s implement a simple countdown timer in JavaScript to illustrate the basic concept:
function countdownTimer(endTime) {
const timer = setInterval(function() {
const now = new Date().getTime();
const timeLeft = endTime - now;
if (timeLeft < 0) {
clearInterval(timer);
console.log("Countdown finished!");
return;
}
const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24));
const hours = Math.floor((timeLeft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((timeLeft % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((timeLeft % (1000 * 60)) / 1000);
console.log(`${days}d ${hours}h ${minutes}m ${seconds}s`);
}, 1000);
}
// Usage: Set the end time 24 hours from now
const endTime = new Date().getTime() + 24 * 60 * 60 * 1000;
countdownTimer(endTime);
This implementation uses setInterval()
to update the countdown every second. It calculates the time difference between the current time and the end time, then breaks it down into days, hours, minutes, and seconds for display.
4. Advanced Techniques and Optimizations
While the basic implementation works for simple use cases, more complex applications may require advanced techniques and optimizations. Here are some areas to consider:
4.1. Performance Optimization
For better performance, especially when dealing with multiple timers or long-running countdowns, consider the following optimizations:
- Use
requestAnimationFrame()
instead ofsetInterval()
for smoother animations and better performance - Implement a single timer that manages multiple countdown instances
- Use Web Workers for background processing of time calculations
4.2. Precision and Drift Correction
JavaScript’s timing functions are not always precise, which can lead to drift over time. To improve accuracy:
- Use high-resolution time APIs like
performance.now()
- Implement drift correction by periodically synchronizing with a server time
- Use a cumulative error approach to adjust for small discrepancies over time
4.3. Time Zone Handling
When dealing with countdowns across different time zones:
- Use UTC timestamps for consistency
- Implement server-side time calculations to ensure accuracy
- Consider using libraries like Moment.js or date-fns for robust time zone handling
4.4. Pause and Resume Functionality
For more interactive countdown timers, implement pause and resume functionality:
class AdvancedCountdownTimer {
constructor(endTime) {
this.endTime = endTime;
this.pausedTime = null;
this.timer = null;
}
start() {
if (this.pausedTime) {
this.endTime += (Date.now() - this.pausedTime);
this.pausedTime = null;
}
this.update();
}
pause() {
if (this.timer) {
clearTimeout(this.timer);
this.pausedTime = Date.now();
}
}
resume() {
if (this.pausedTime) {
this.start();
}
}
update() {
const now = Date.now();
const timeLeft = this.endTime - now;
if (timeLeft <= 0) {
console.log("Countdown finished!");
return;
}
console.log(`Time left: ${this.formatTime(timeLeft)}`);
this.timer = setTimeout(() => this.update(), 1000);
}
formatTime(ms) {
const seconds = Math.floor(ms / 1000) % 60;
const minutes = Math.floor(ms / (1000 * 60)) % 60;
const hours = Math.floor(ms / (1000 * 60 * 60)) % 24;
const days = Math.floor(ms / (1000 * 60 * 60 * 24));
return `${days}d ${hours}h ${minutes}m ${seconds}s`;
}
}
// Usage
const timer = new AdvancedCountdownTimer(Date.now() + 24 * 60 * 60 * 1000);
timer.start();
// Pause after 5 seconds
setTimeout(() => {
timer.pause();
console.log("Timer paused");
// Resume after 3 more seconds
setTimeout(() => {
timer.resume();
console.log("Timer resumed");
}, 3000);
}, 5000);
5. Real-World Applications of Countdown Timers
Countdown timers have numerous practical applications across various domains. Here are some common use cases:
5.1. E-commerce and Marketing
- Limited-time offers and flash sales
- Product launches and pre-orders
- Auction end times
5.2. Event Management
- Countdown to event start times
- Session timers for conferences or workshops
- Registration or ticket sale deadlines
5.3. Productivity and Time Management
- Pomodoro technique timers
- Task duration tracking
- Break reminders
5.4. Gaming and Entertainment
- Countdown to game releases
- In-game timers for challenges or events
- Streaming countdown for live shows
5.5. Education and Assessment
- Exam timers
- Presentation timers
- Course enrollment deadlines
6. Common Challenges and Solutions
Implementing countdown timers can present several challenges. Here are some common issues and their solutions:
6.1. Browser Tab Inactivity
Challenge: When a browser tab is inactive, some browsers may throttle JavaScript timers, causing inaccuracies.
Solution: Use the Page Visibility API to detect when a tab becomes active and recalculate the countdown based on the actual elapsed time.
document.addEventListener("visibilitychange", function() {
if (!document.hidden) {
// Recalculate and update the timer
updateCountdown();
}
});
6.2. Network Latency and Server Time Discrepancies
Challenge: Client-side timers may become inaccurate due to network latency or differences between client and server time.
Solution: Implement server-side time calculations and periodically synchronize the client-side timer with the server.
function syncWithServer() {
fetch('/api/server-time')
.then(response => response.json())
.then(data => {
const serverTime = new Date(data.serverTime);
const clientTime = new Date();
const timeDiff = serverTime - clientTime;
// Adjust the countdown based on the time difference
adjustCountdown(timeDiff);
});
}
// Call syncWithServer periodically
setInterval(syncWithServer, 60000); // Sync every minute
6.3. Memory Leaks in Long-Running Timers
Challenge: Long-running timers or improper cleanup can lead to memory leaks, especially in single-page applications.
Solution: Ensure proper cleanup of timers when components are unmounted or the countdown is complete.
class CountdownComponent extends React.Component {
componentDidMount() {
this.timer = setInterval(() => this.tick(), 1000);
}
componentWillUnmount() {
clearInterval(this.timer);
}
tick() {
// Update countdown logic
}
render() {
// Render countdown display
}
}
6.4. Handling Time Zone Changes
Challenge: Users changing time zones or daylight saving time transitions can affect countdown accuracy.
Solution: Use UTC timestamps for calculations and convert to local time only for display purposes.
function getCountdown(endTimeUTC) {
const now = new Date();
const timeLeftUTC = endTimeUTC - now.getTime();
// Convert UTC time difference to local time components
const localOffset = now.getTimezoneOffset() * 60000;
const localTimeLeft = timeLeftUTC + localOffset;
// Calculate days, hours, minutes, seconds
// ...
return { days, hours, minutes, seconds };
}
7. Testing and Debugging Countdown Timers
Thorough testing and debugging are crucial for ensuring the reliability and accuracy of countdown timers. Here are some strategies and tools to help with this process:
7.1. Unit Testing
Write comprehensive unit tests to verify the correctness of your countdown logic. Use testing frameworks like Jest for JavaScript to automate these tests.
describe('Countdown Timer', () => {
test('calculates correct time difference', () => {
const now = new Date('2023-05-01T00:00:00Z');
const end = new Date('2023-05-02T00:00:00Z');
const diff = calculateTimeDifference(now, end);
expect(diff).toEqual({
days: 1,
hours: 0,
minutes: 0,
seconds: 0
});
});
// More test cases...
});
7.2. Time Manipulation
Use time manipulation libraries like Sinon.js to control the flow of time in your tests. This allows you to simulate long countdowns without actually waiting for the full duration.
describe('Countdown Timer', () => {
let clock;
beforeEach(() => {
clock = sinon.useFakeTimers();
});
afterEach(() => {
clock.restore();
});
test('updates every second', () => {
const timer = new CountdownTimer(Date.now() + 10000);
timer.start();
clock.tick(1000);
expect(timer.getTimeLeft()).toBe(9);
clock.tick(1000);
expect(timer.getTimeLeft()).toBe(8);
});
});
7.3. Browser DevTools
Utilize browser developer tools to debug and profile your countdown timer implementation:
- Use the Performance tab to identify any performance bottlenecks
- Set breakpoints in the Sources tab to step through your code
- Monitor memory usage in the Memory tab to detect potential leaks
7.4. Logging and Monitoring
Implement robust logging in your countdown timer to track its behavior over time. This can help identify issues that may only occur in specific scenarios or after extended periods.
class CountdownTimer {
constructor(endTime) {
this.endTime = endTime;
this.logger = new Logger();
}
update() {
const now = Date.now();
const timeLeft = this.endTime - now;
this.logger.log({
event: 'timer_update',
timeLeft,
currentTime: now,
endTime: this.endTime
});
// Rest of the update logic...
}
}
8. Future Trends in Countdown Timer Implementations
As web technologies continue to evolve, we can expect to see new trends and improvements in countdown timer implementations:
8.1. Web Workers for Improved Performance
Utilizing Web Workers for time calculations can offload processing from the main thread, leading to smoother user interfaces, especially for applications with multiple timers.
// main.js
const worker = new Worker('timer-worker.js');
worker.onmessage = function(e) {
updateTimerDisplay(e.data);
};
worker.postMessage({ command: 'start', endTime: Date.now() + 60000 });
// timer-worker.js
self.onmessage = function(e) {
if (e.data.command === 'start') {
startTimer(e.data.endTime);
}
};
function startTimer(endTime) {
const timer = setInterval(() => {
const timeLeft = endTime - Date.now();
if (timeLeft <= 0) {
clearInterval(timer);
self.postMessage({ timeLeft: 0, status: 'completed' });
} else {
self.postMessage({ timeLeft, status: 'running' });
}
}, 1000);
}
8.2. WebAssembly for High-Performance Timers
For applications requiring extremely precise timing or handling a large number of simultaneous countdowns, WebAssembly can provide near-native performance for time calculations.
8.3. Progressive Web Apps (PWAs) and Offline Functionality
Implementing countdown timers in PWAs will require handling offline scenarios and synchronization when the connection is restored.
8.4. AI-Powered Smart Timers
Future countdown timers might incorporate AI to adjust countdown behavior based on user patterns or external factors, providing more personalized and context-aware timing experiences.
8.5. Integration with Web Components
As Web Components gain wider adoption, we may see more reusable and customizable countdown timer components that can be easily integrated into various web applications.
class CountdownTimerComponent extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
}
connectedCallback() {
const endTime = parseInt(this.getAttribute('end-time'));
this.startTimer(endTime);
}
startTimer(endTime) {
// Timer logic here
}
// Other methods...
}
customElements.define('countdown-timer', CountdownTimerComponent);
// Usage in HTML
<countdown-timer end-time="1640995200000"></countdown-timer>
9. Conclusion
Implementing countdown timers with algorithms is a fascinating blend of mathematical precision and practical programming. From basic implementations to advanced techniques handling real-world challenges, countdown timers showcase the importance of time management in software development.
As we’ve explored in this comprehensive guide, creating effective countdown timers involves more than just simple time calculations. It requires careful consideration of performance optimization, accuracy, user experience, and cross-platform compatibility. By understanding the underlying algorithms and implementing best practices, developers can create robust and efficient countdown timers that enhance a wide range of applications.
The future of countdown timer implementations looks promising, with emerging technologies like Web Workers, WebAssembly, and AI opening up new possibilities for even more sophisticated and performant timers. As you continue to work with countdown timers, remember that the key to success lies in balancing technical precision with user-centric design, ensuring that your timers not only count down accurately but also provide meaningful and engaging experiences for your users.
Whether you’re building e-commerce platforms, productivity tools, or interactive web applications, mastering the art of countdown timer implementation will undoubtedly be a valuable skill in your developer toolkit. Keep experimenting, testing, and refining your approaches to create countdown timers that not only meet technical requirements but also delight and engage users across various digital experiences.