Implementing Convex Optimization Algorithms: A Comprehensive Guide
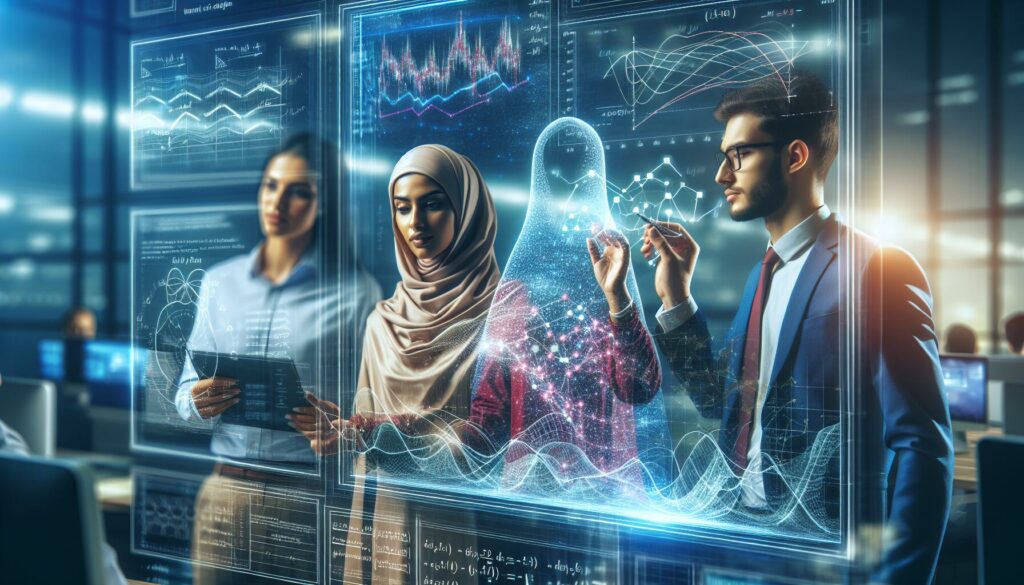
In the world of computer science and mathematical optimization, convex optimization algorithms play a crucial role in solving a wide range of problems efficiently. These algorithms are essential for various applications, including machine learning, signal processing, and control systems. In this comprehensive guide, we’ll explore the fundamentals of convex optimization, discuss popular algorithms, and provide practical implementations to help you master this important topic.
Table of Contents
- Introduction to Convex Optimization
- Gradient Descent Algorithm
- Newton’s Method
- Interior Point Methods
- Proximal Gradient Methods
- Stochastic Gradient Descent
- Conjugate Gradient Method
- Quasi-Newton Methods
- Applications of Convex Optimization
- Challenges and Future Directions
1. Introduction to Convex Optimization
Convex optimization is a subfield of mathematical optimization that deals with minimizing convex functions over convex sets. The primary goal is to find the global minimum of a convex function efficiently. Convex optimization problems have several desirable properties:
- They have a unique global minimum (or a convex set of global minima).
- Local minima are also global minima.
- They can be solved efficiently using various algorithms.
A typical convex optimization problem can be formulated as follows:
minimize f(x)
subject to g_i(x) <= 0, i = 1, ..., m
h_j(x) = 0, j = 1, ..., p
Where:
- f(x) is the convex objective function to be minimized
- g_i(x) are convex inequality constraints
- h_j(x) are affine equality constraints
Now, let’s dive into some of the most popular convex optimization algorithms and their implementations.
2. Gradient Descent Algorithm
Gradient Descent is one of the simplest and most widely used optimization algorithms. It iteratively moves towards the minimum of a function by taking steps proportional to the negative of the gradient at the current point.
Algorithm:
- Initialize the starting point x_0
- Repeat until convergence:
- Compute the gradient of the objective function at the current point
- Update the current point by moving in the opposite direction of the gradient
Python Implementation:
import numpy as np
def gradient_descent(f, grad_f, x0, learning_rate=0.01, max_iterations=1000, tolerance=1e-6):
x = x0
for i in range(max_iterations):
grad = grad_f(x)
x_new = x - learning_rate * grad
if np.linalg.norm(x_new - x) < tolerance:
break
x = x_new
return x
# Example usage
def f(x):
return x**2
def grad_f(x):
return 2*x
x0 = np.array([5.0])
result = gradient_descent(f, grad_f, x0)
print(f"Minimum found at: {result}")
Gradient descent is simple to implement and works well for many problems. However, it can be slow to converge for ill-conditioned problems and may struggle with saddle points in high-dimensional spaces.
3. Newton’s Method
Newton’s Method is a second-order optimization algorithm that uses both the gradient and the Hessian of the objective function. It converges faster than gradient descent for well-behaved functions but requires more computation per iteration.
Algorithm:
- Initialize the starting point x_0
- Repeat until convergence:
- Compute the gradient and Hessian of the objective function at the current point
- Solve the Newton system: H * Δx = -g
- Update the current point: x = x + Δx
Python Implementation:
import numpy as np
def newtons_method(f, grad_f, hessian_f, x0, max_iterations=100, tolerance=1e-6):
x = x0
for i in range(max_iterations):
grad = grad_f(x)
hess = hessian_f(x)
delta_x = np.linalg.solve(hess, -grad)
x_new = x + delta_x
if np.linalg.norm(x_new - x) < tolerance:
break
x = x_new
return x
# Example usage
def f(x):
return x[0]**2 + x[1]**2
def grad_f(x):
return np.array([2*x[0], 2*x[1]])
def hessian_f(x):
return np.array([[2, 0], [0, 2]])
x0 = np.array([5.0, 5.0])
result = newtons_method(f, grad_f, hessian_f, x0)
print(f"Minimum found at: {result}")
Newton’s Method converges quadratically for well-behaved functions, making it very efficient. However, it requires computing and inverting the Hessian matrix, which can be computationally expensive for high-dimensional problems.
4. Interior Point Methods
Interior Point Methods are a class of algorithms used for solving constrained optimization problems. They work by transforming the constrained problem into a sequence of unconstrained problems using barrier functions.
Algorithm (Primal-Dual Interior Point Method):
- Initialize primal and dual variables
- Repeat until convergence:
- Compute the barrier function and its derivatives
- Solve the Newton system for the search direction
- Perform a line search to determine the step size
- Update primal and dual variables
- Update the barrier parameter
Python Implementation (using cvxpy library):
import cvxpy as cp
import numpy as np
def interior_point_method(c, A, b):
m, n = A.shape
x = cp.Variable(n)
objective = cp.Minimize(c.T @ x)
constraints = [A @ x <= b]
problem = cp.Problem(objective, constraints)
result = problem.solve(solver=cp.ECOS)
return x.value, result
# Example usage
c = np.array([-1, -1])
A = np.array([[1, 1], [1, 0], [0, 1]])
b = np.array([1, 0.7, 0.7])
x_opt, opt_value = interior_point_method(c, A, b)
print(f"Optimal solution: {x_opt}")
print(f"Optimal value: {opt_value}")
Interior Point Methods are particularly useful for large-scale linear and quadratic programming problems. They can handle inequality constraints efficiently and have polynomial-time complexity for linear programming problems.
5. Proximal Gradient Methods
Proximal Gradient Methods are a class of first-order optimization algorithms that are particularly useful for solving composite optimization problems. These problems consist of a smooth convex function and a non-smooth convex function.
Algorithm (Proximal Gradient Descent):
- Initialize the starting point x_0
- Repeat until convergence:
- Compute the gradient of the smooth part of the objective function
- Take a gradient step
- Apply the proximal operator of the non-smooth part
Python Implementation:
import numpy as np
def proximal_gradient_descent(f, grad_f, prox_g, x0, learning_rate=0.01, max_iterations=1000, tolerance=1e-6):
x = x0
for i in range(max_iterations):
grad = grad_f(x)
x_half = x - learning_rate * grad
x_new = prox_g(x_half, learning_rate)
if np.linalg.norm(x_new - x) < tolerance:
break
x = x_new
return x
# Example usage: LASSO regression
def f(x, A, b):
return 0.5 * np.sum((A @ x - b) ** 2)
def grad_f(x, A, b):
return A.T @ (A @ x - b)
def prox_g(x, t, lambda_):
return np.sign(x) * np.maximum(np.abs(x) - t * lambda_, 0)
# Generate sample data
np.random.seed(42)
n, p = 100, 20
A = np.random.randn(n, p)
x_true = np.random.randn(p)
x_true[np.abs(x_true) < 0.5] = 0
b = A @ x_true + 0.1 * np.random.randn(n)
# Solve LASSO problem
lambda_ = 0.1
x0 = np.zeros(p)
result = proximal_gradient_descent(
lambda x: f(x, A, b),
lambda x: grad_f(x, A, b),
lambda x, t: prox_g(x, t, lambda_),
x0
)
print(f"LASSO solution: {result}")
Proximal Gradient Methods are particularly useful for problems with non-smooth regularization terms, such as L1 regularization in LASSO regression. They can handle non-differentiable functions and have good convergence properties for composite optimization problems.
6. Stochastic Gradient Descent
Stochastic Gradient Descent (SGD) is a variant of the gradient descent algorithm that is particularly useful for large-scale machine learning problems. Instead of computing the gradient using the entire dataset, SGD estimates the gradient using a small subset of the data (mini-batch) at each iteration.
Algorithm:
- Initialize the starting point w_0
- Repeat until convergence:
- Randomly select a mini-batch of samples from the dataset
- Compute the gradient estimate using the mini-batch
- Update the parameters using the estimated gradient
Python Implementation:
import numpy as np
def stochastic_gradient_descent(X, y, learning_rate=0.01, batch_size=32, epochs=100):
n_samples, n_features = X.shape
w = np.zeros(n_features)
b = 0
for epoch in range(epochs):
for i in range(0, n_samples, batch_size):
X_batch = X[i:i+batch_size]
y_batch = y[i:i+batch_size]
# Compute predictions
y_pred = np.dot(X_batch, w) + b
# Compute gradients
dw = (1/batch_size) * np.dot(X_batch.T, (y_pred - y_batch))
db = (1/batch_size) * np.sum(y_pred - y_batch)
# Update parameters
w -= learning_rate * dw
b -= learning_rate * db
return w, b
# Example usage: Linear Regression
np.random.seed(42)
n_samples, n_features = 1000, 5
X = np.random.randn(n_samples, n_features)
true_w = np.random.randn(n_features)
y = np.dot(X, true_w) + 0.1 * np.random.randn(n_samples)
w_sgd, b_sgd = stochastic_gradient_descent(X, y)
print(f"SGD solution - w: {w_sgd}, b: {b_sgd}")
Stochastic Gradient Descent is widely used in training deep neural networks and other large-scale machine learning models. It offers several advantages:
- Reduced memory requirements, as it processes data in small batches
- Faster iterations, allowing for quicker convergence on large datasets
- Ability to escape local minima due to the noise in gradient estimates
7. Conjugate Gradient Method
The Conjugate Gradient Method is an algorithm for solving large systems of linear equations and optimizing quadratic functions. It is particularly effective for sparse systems and can be adapted for non-linear optimization problems.
Algorithm (for quadratic optimization):
- Initialize x_0, compute r_0 = b – Ax_0, and set p_0 = r_0
- For k = 0, 1, 2, … until convergence:
- Compute α_k = (r_k^T r_k) / (p_k^T A p_k)
- Update x_k+1 = x_k + α_k p_k
- Compute r_k+1 = r_k – α_k A p_k
- Compute β_k = (r_k+1^T r_k+1) / (r_k^T r_k)
- Update p_k+1 = r_k+1 + β_k p_k
Python Implementation:
import numpy as np
def conjugate_gradient(A, b, x0=None, max_iterations=1000, tolerance=1e-6):
n = len(b)
if x0 is None:
x = np.zeros(n)
else:
x = x0
r = b - A @ x
p = r.copy()
r_norm_sq = np.dot(r, r)
for i in range(max_iterations):
Ap = A @ p
alpha = r_norm_sq / np.dot(p, Ap)
x += alpha * p
r -= alpha * Ap
r_norm_sq_new = np.dot(r, r)
if np.sqrt(r_norm_sq_new) < tolerance:
break
beta = r_norm_sq_new / r_norm_sq
p = r + beta * p
r_norm_sq = r_norm_sq_new
return x
# Example usage
A = np.array([[4, 1], [1, 3]])
b = np.array([1, 2])
x_cg = conjugate_gradient(A, b)
print(f"Conjugate Gradient solution: {x_cg}")
# Verify the solution
print(f"Ax - b: {A @ x_cg - b}")
The Conjugate Gradient Method has several advantages:
- It converges in at most n iterations for an n-dimensional problem (in exact arithmetic)
- It requires only matrix-vector products, making it suitable for large, sparse systems
- It can be adapted for non-linear optimization problems using nonlinear conjugate gradient methods
8. Quasi-Newton Methods
Quasi-Newton methods are optimization algorithms that approximate the Hessian matrix or its inverse using gradient information. These methods aim to achieve faster convergence than first-order methods while avoiding the computational cost of computing the exact Hessian.
BFGS Algorithm:
- Initialize x_0 and an initial approximation of the inverse Hessian H_0
- For k = 0, 1, 2, … until convergence:
- Compute the search direction: p_k = -H_k ∇f(x_k)
- Perform a line search to find an appropriate step size α_k
- Update x_k+1 = x_k + α_k p_k
- Compute s_k = x_k+1 – x_k and y_k = ∇f(x_k+1) – ∇f(x_k)
- Update the approximation of the inverse Hessian H_k+1 using the BFGS formula
Python Implementation (L-BFGS):
import numpy as np
from scipy.optimize import minimize
def rosenbrock(x):
return (1 - x[0])**2 + 100 * (x[1] - x[0]**2)**2
def rosenbrock_grad(x):
return np.array([
-2 * (1 - x[0]) - 400 * x[0] * (x[1] - x[0]**2),
200 * (x[1] - x[0]**2)
])
# Initial guess
x0 = np.array([-1.2, 1.0])
# Optimize using L-BFGS-B
result = minimize(rosenbrock, x0, method='L-BFGS-B', jac=rosenbrock_grad, options={'disp': True})
print(f"Optimal solution: {result.x}")
print(f"Optimal value: {result.fun}")
print(f"Number of iterations: {result.nit}")
Quasi-Newton methods, such as BFGS and L-BFGS, offer several advantages:
- Faster convergence than first-order methods like gradient descent
- No need to compute the exact Hessian matrix
- Suitable for large-scale optimization problems
- Adaptable to various problem structures
9. Applications of Convex Optimization
Convex optimization algorithms find applications in numerous fields, including:
- Machine Learning:
- Support Vector Machines (SVMs) for classification
- Logistic Regression for binary classification
- LASSO and Ridge Regression for feature selection and regularization
- Signal Processing:
- Compressed Sensing for signal reconstruction
- Image denoising and restoration
- Filter design and spectral estimation
- Control Systems:
- Model Predictive Control (MPC) for optimal control
- Robust control design
- Trajectory optimization for robotics
- Finance:
- Portfolio optimization
- Risk management
- Option pricing
- Operations Research:
- Resource allocation problems
- Network flow optimization
- Supply chain management
10. Challenges and Future Directions
While convex optimization has made significant progress, there are still challenges and areas for future research:
- Non-convex Optimization:
- Developing algorithms for efficiently solving non-convex problems
- Understanding the landscape of non-convex optimization in deep learning
- Large-scale and Distributed Optimization:
- Designing algorithms for extremely large-scale problems
- Developing efficient distributed optimization techniques
- Robustness and Uncertainty:
- Incorporating robustness to model uncertainties and data noise
- Developing algorithms for stochastic and online optimization
- Interpretability and Explainability:
- Developing optimization techniques that produce interpretable models
- Incorporating explainability constraints in optimization problems
- Integration with Machine Learning:
- Combining convex optimization with deep learning techniques
- Developing optimization-based approaches for model compression and quantization
As the field of convex optimization continues to evolve, these challenges present exciting opportunities for researchers and practitioners to develop new algorithms and applications.
Conclusion
Convex optimization algorithms play a crucial role in solving a wide range of problems efficiently. From the simple gradient descent to more advanced methods like interior point and quasi-Newton algorithms, each technique offers unique advantages for different problem structures. By understanding and implementing these algorithms, you can tackle complex optimization challenges in various domains, including machine learning, signal processing, and control systems.
As you continue to explore and implement convex optimization algorithms, remember that the choice of algorithm depends on the specific problem at hand, the scale of the data, and the desired trade-offs between computational complexity and convergence speed. Experiment with different methods and leverage available software libraries to find the most suitable approach for your optimization tasks.