Implementing Common Sorting Algorithms Efficiently
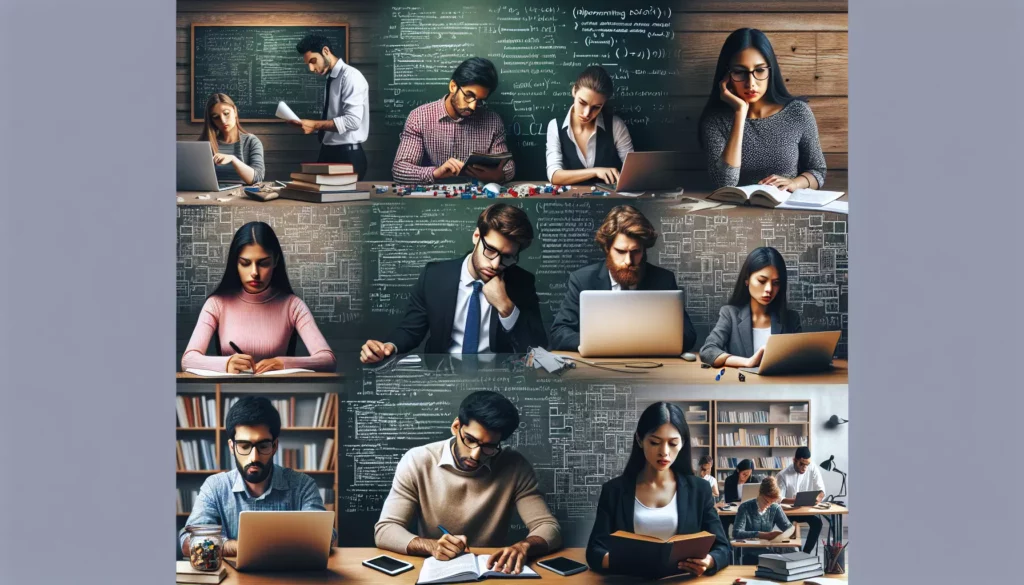
Sorting algorithms are fundamental components of computer science and play a crucial role in organizing data efficiently. As aspiring programmers and software engineers, it’s essential to understand and implement these algorithms effectively. In this comprehensive guide, we’ll explore several common sorting algorithms, discuss their implementations, analyze their time and space complexities, and provide practical examples to solidify your understanding.
1. Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order. While it’s not the most efficient algorithm for large datasets, it’s easy to understand and implement.
Implementation
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(unsorted_array)
print(sorted_array) # Output: [11, 12, 22, 25, 34, 64, 90]
Time and Space Complexity
- Time Complexity: O(n^2) in the worst and average cases
- Space Complexity: O(1)
Bubble Sort’s quadratic time complexity makes it inefficient for large datasets, but its simplicity and in-place sorting (constant space complexity) can be advantageous in certain scenarios with small arrays or nearly sorted data.
2. Selection Sort
Selection Sort divides the input list into two parts: a sorted portion at the left end and an unsorted portion at the right end. It repeatedly selects the smallest (or largest) element from the unsorted portion and moves it to the sorted portion.
Implementation
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i + 1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
unsorted_array = [64, 25, 12, 22, 11]
sorted_array = selection_sort(unsorted_array)
print(sorted_array) # Output: [11, 12, 22, 25, 64]
Time and Space Complexity
- Time Complexity: O(n^2) in all cases
- Space Complexity: O(1)
Like Bubble Sort, Selection Sort has a quadratic time complexity, making it inefficient for large datasets. However, it performs fewer swaps compared to Bubble Sort, which can be beneficial when memory writes are costly.
3. Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It iterates through the input elements, growing the sorted array with each iteration. It’s efficient for small datasets and nearly sorted arrays.
Implementation
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
unsorted_array = [12, 11, 13, 5, 6]
sorted_array = insertion_sort(unsorted_array)
print(sorted_array) # Output: [5, 6, 11, 12, 13]
Time and Space Complexity
- Time Complexity: O(n^2) in the worst and average cases, O(n) in the best case (nearly sorted array)
- Space Complexity: O(1)
Insertion Sort’s efficiency for small datasets and its ability to sort the array in-place make it a popular choice for certain scenarios, especially when dealing with nearly sorted data.
4. Merge Sort
Merge Sort is an efficient, stable, and comparison-based sorting algorithm that follows the divide-and-conquer paradigm. It divides the unsorted list into n sublists, each containing one element, then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining.
Implementation
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] <= R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
# Example usage
unsorted_array = [38, 27, 43, 3, 9, 82, 10]
sorted_array = merge_sort(unsorted_array)
print(sorted_array) # Output: [3, 9, 10, 27, 38, 43, 82]
Time and Space Complexity
- Time Complexity: O(n log n) in all cases
- Space Complexity: O(n)
Merge Sort’s consistent O(n log n) time complexity makes it efficient for large datasets. However, its space complexity of O(n) can be a drawback when memory is limited.
5. Quick Sort
Quick Sort is another efficient sorting algorithm that uses the divide-and-conquer strategy. It selects a ‘pivot’ element from the array and partitions the other elements into two sub-arrays, according to whether they are less than or greater than the pivot.
Implementation
def partition(arr, low, high):
i = low - 1
pivot = arr[high]
for j in range(low, high):
if arr[j] <= pivot:
i += 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return i + 1
def quick_sort(arr, low, high):
if low < high:
pi = partition(arr, low, high)
quick_sort(arr, low, pi - 1)
quick_sort(arr, pi + 1, high)
return arr
# Example usage
unsorted_array = [10, 7, 8, 9, 1, 5]
sorted_array = quick_sort(unsorted_array, 0, len(unsorted_array) - 1)
print(sorted_array) # Output: [1, 5, 7, 8, 9, 10]
Time and Space Complexity
- Time Complexity: O(n log n) on average, O(n^2) in the worst case
- Space Complexity: O(log n) due to the recursive call stack
Quick Sort’s average-case time complexity and in-place sorting make it one of the most widely used sorting algorithms. However, its worst-case time complexity can be a concern in certain scenarios.
6. Heap Sort
Heap Sort is a comparison-based sorting algorithm that uses a binary heap data structure. It divides its input into a sorted and an unsorted region, and it iteratively shrinks the unsorted region by extracting the largest element and moving that to the sorted region.
Implementation
def heapify(arr, n, i):
largest = i
l = 2 * i + 1
r = 2 * i + 2
if l < n and arr[largest] < arr[l]:
largest = l
if r < n and arr[largest] < arr[r]:
largest = r
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n - 1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
return arr
# Example usage
unsorted_array = [12, 11, 13, 5, 6, 7]
sorted_array = heap_sort(unsorted_array)
print(sorted_array) # Output: [5, 6, 7, 11, 12, 13]
Time and Space Complexity
- Time Complexity: O(n log n) in all cases
- Space Complexity: O(1)
Heap Sort’s consistent time complexity and in-place sorting make it an excellent choice for scenarios where stable sorting is not required and memory usage is a concern.
7. Radix Sort
Radix Sort is a non-comparative integer sorting algorithm that sorts data with integer keys by grouping keys by the individual digits which share the same significant position and value.
Implementation
def counting_sort(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_num = max(arr)
exp = 1
while max_num // exp > 0:
counting_sort(arr, exp)
exp *= 10
return arr
# Example usage
unsorted_array = [170, 45, 75, 90, 802, 24, 2, 66]
sorted_array = radix_sort(unsorted_array)
print(sorted_array) # Output: [2, 24, 45, 66, 75, 90, 170, 802]
Time and Space Complexity
- Time Complexity: O(d * (n + k)), where d is the number of digits, n is the number of elements, and k is the range of input
- Space Complexity: O(n + k)
Radix Sort can be faster than comparison-based sorting algorithms for integers or strings with fixed-length keys. However, it requires additional space and is limited to inputs with fixed-size keys.
Choosing the Right Sorting Algorithm
Selecting the appropriate sorting algorithm depends on various factors:
- Input size: For small datasets, simpler algorithms like Insertion Sort may be faster due to lower overhead. For larger datasets, more efficient algorithms like Merge Sort or Quick Sort are preferred.
- Data distribution: Some algorithms perform better with partially sorted data (e.g., Insertion Sort), while others are consistently efficient regardless of data distribution (e.g., Merge Sort).
- Memory constraints: In-place sorting algorithms like Quick Sort or Heap Sort are preferable when memory is limited.
- Stability requirement: If maintaining the relative order of equal elements is important, stable sorting algorithms like Merge Sort should be used.
- Time complexity consistency: Algorithms with consistent time complexity (e.g., Merge Sort) may be preferred in scenarios where predictable performance is crucial.
Optimizing Sorting Implementations
To implement sorting algorithms efficiently, consider the following optimization techniques:
- Hybrid algorithms: Combine multiple sorting algorithms to leverage their strengths. For example, using Insertion Sort for small subarrays within Merge Sort or Quick Sort.
- Pivot selection in Quick Sort: Use techniques like median-of-three to choose a better pivot and reduce the chance of worst-case scenarios.
- Tail recursion optimization: Implement tail call optimization in recursive algorithms to reduce stack space usage.
- Cache-friendly implementations: Design algorithms to maximize cache utilization, especially for large datasets.
- Parallel sorting: Utilize multi-threading or distributed computing for sorting very large datasets.
Conclusion
Understanding and implementing common sorting algorithms efficiently is crucial for any programmer or software engineer. Each algorithm has its strengths and weaknesses, and choosing the right one depends on the specific requirements of your application. By mastering these algorithms and their optimizations, you’ll be better equipped to handle a wide range of sorting problems and improve the overall performance of your software systems.
Remember that while theoretical knowledge is important, practical implementation and testing are equally crucial. Experiment with different algorithms, analyze their performance on various datasets, and continually refine your understanding of when and how to apply each sorting technique effectively.