Implementing Algorithms for Data Compression: A Comprehensive Guide
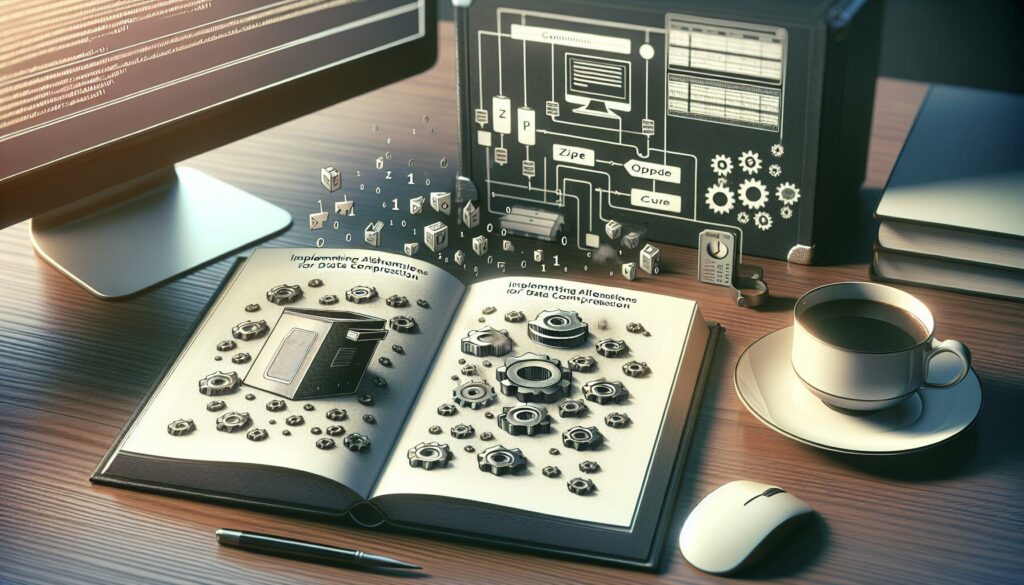
In the ever-expanding digital landscape, data compression plays a crucial role in optimizing storage and transmission of information. As aspiring programmers and software engineers, understanding and implementing data compression algorithms is an essential skill that can significantly enhance your coding prowess and prepare you for technical interviews at top tech companies. In this comprehensive guide, we’ll dive deep into the world of data compression algorithms, exploring their principles, implementations, and practical applications.
Table of Contents
- Introduction to Data Compression
- Lossless Compression Algorithms
- Lossy Compression Algorithms
- Implementing Compression Algorithms
- Performance Analysis and Optimization
- Real-World Applications
- Future Trends in Data Compression
- Conclusion
1. Introduction to Data Compression
Data compression is the process of encoding information using fewer bits than the original representation. This technique is essential for reducing storage requirements and improving transmission speeds across networks. Compression algorithms can be broadly categorized into two types:
- Lossless compression: Preserves all original data and allows for perfect reconstruction.
- Lossy compression: Achieves higher compression ratios by discarding some less critical information.
The choice between lossless and lossy compression depends on the specific application and the nature of the data being compressed. For instance, text documents and program files typically require lossless compression to maintain integrity, while images and audio files can often benefit from lossy compression techniques.
2. Lossless Compression Algorithms
Lossless compression algorithms ensure that the decompressed data is identical to the original. Let’s explore three popular lossless compression techniques:
2.1 Run-Length Encoding (RLE)
Run-Length Encoding is one of the simplest forms of data compression. It works by replacing sequences of identical data elements (runs) with a single data value and count.
Example:
Original data: WWWWWWWWWWWWBWWWWWWWWWWWWBBBWWWWWWWWWWWWWWWWWWB
Compressed data: 12W1B12W3B24W1B
RLE is particularly effective for data with long runs of repeated values, such as simple graphics or binary data.
2.2 Huffman Coding
Huffman coding is a variable-length prefix coding algorithm that assigns shorter codes to more frequent symbols and longer codes to less frequent ones. It builds a binary tree (Huffman tree) based on the frequency of each symbol in the input data.
The algorithm follows these steps:
- Calculate the frequency of each symbol in the input data.
- Create a leaf node for each symbol and add it to a priority queue.
- While there is more than one node in the queue:
- Remove the two nodes with the lowest frequency.
- Create a new internal node with these two nodes as children.
- Add the new node back to the queue.
- The remaining node is the root of the Huffman tree.
- Traverse the tree to assign binary codes to each symbol.
Huffman coding is widely used in various file compression formats and is particularly effective for text compression.
2.3 Lempel-Ziv-Welch (LZW)
LZW is a dictionary-based compression algorithm that builds a dictionary of substrings as it processes the input data. It replaces repeated occurrences of substrings with references to their earlier occurrences in the dictionary.
The LZW algorithm works as follows:
- Initialize the dictionary with single-character strings.
- Read input data character by character.
- Find the longest matching substring in the dictionary.
- Output the code for the matching substring.
- Add the matched substring plus the next character to the dictionary.
- Repeat steps 2-5 until the end of input.
LZW is particularly effective for compressing data with repeated patterns and is used in various file formats, including GIF and TIFF.
3. Lossy Compression Algorithms
Lossy compression algorithms achieve higher compression ratios by discarding some information, making them suitable for applications where perfect reconstruction is not necessary, such as image and audio compression.
3.1 Transform Coding
Transform coding is a lossy compression technique that involves transforming the input data into a different domain, typically using mathematical transforms like the Discrete Cosine Transform (DCT) or Wavelet Transform. The transformed data is then quantized and encoded.
The general steps in transform coding are:
- Divide the input data into blocks.
- Apply the mathematical transform to each block.
- Quantize the transformed coefficients.
- Encode the quantized coefficients.
Transform coding is widely used in image and video compression standards like JPEG and MPEG.
3.2 Vector Quantization
Vector Quantization (VQ) is a lossy compression technique that works by dividing the input data into vectors and mapping each vector to the nearest representative vector from a predefined codebook.
The VQ process involves:
- Dividing the input data into vectors.
- Creating a codebook of representative vectors.
- Mapping each input vector to the nearest codebook vector.
- Encoding the index of the matched codebook vector.
VQ is used in various applications, including image and speech compression.
4. Implementing Compression Algorithms
Now that we’ve covered the theoretical aspects of various compression algorithms, let’s dive into their implementations. We’ll focus on implementing the lossless compression algorithms discussed earlier: Run-Length Encoding, Huffman Coding, and LZW.
4.1 RLE Implementation
Here’s a simple implementation of Run-Length Encoding in Python:
def rle_encode(data):
encoding = []
count = 1
for i in range(1, len(data)):
if data[i] == data[i-1]:
count += 1
else:
encoding.append((count, data[i-1]))
count = 1
encoding.append((count, data[-1]))
return encoding
def rle_decode(encoding):
return ''.join(char * count for count, char in encoding)
# Example usage
original = "WWWWWWWWWWWWBWWWWWWWWWWWWBBBWWWWWWWWWWWWWWWWWWB"
encoded = rle_encode(original)
decoded = rle_decode(encoded)
print(f"Original: {original}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
print(f"Original == Decoded: {original == decoded}")
This implementation demonstrates both encoding and decoding functions for RLE. The rle_encode
function compresses the input string, while rle_decode
reconstructs the original data from the compressed format.
4.2 Huffman Coding Implementation
Implementing Huffman coding is more complex. Here’s a basic implementation in Python:
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(data):
frequency = defaultdict(int)
for char in data:
frequency[char] += 1
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
merged = Node(None, left.freq + right.freq)
merged.left = left
merged.right = right
heapq.heappush(heap, merged)
return heap[0]
def build_huffman_codes(root):
codes = {}
def dfs(node, code):
if node.char:
codes[node.char] = code
return
dfs(node.left, code + "0")
dfs(node.right, code + "1")
dfs(root, "")
return codes
def huffman_encode(data):
root = build_huffman_tree(data)
codes = build_huffman_codes(root)
encoded = "".join(codes[char] for char in data)
return encoded, codes
def huffman_decode(encoded, codes):
reversed_codes = {code: char for char, code in codes.items()}
decoded = ""
current_code = ""
for bit in encoded:
current_code += bit
if current_code in reversed_codes:
decoded += reversed_codes[current_code]
current_code = ""
return decoded
# Example usage
data = "this is an example for huffman encoding"
encoded, codes = huffman_encode(data)
decoded = huffman_decode(encoded, codes)
print(f"Original: {data}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
print(f"Original == Decoded: {data == decoded}")
This implementation includes functions for building the Huffman tree, generating Huffman codes, encoding, and decoding. The huffman_encode
function compresses the input string, while huffman_decode
reconstructs the original data from the compressed format and the Huffman codes.
4.3 LZW Implementation
Here’s a basic implementation of the LZW algorithm in Python:
def lzw_encode(data):
dictionary = {chr(i): i for i in range(256)}
result = []
w = ""
code = 256
for c in data:
wc = w + c
if wc in dictionary:
w = wc
else:
result.append(dictionary[w])
dictionary[wc] = code
code += 1
w = c
if w:
result.append(dictionary[w])
return result
def lzw_decode(encoded):
dictionary = {i: chr(i) for i in range(256)}
result = []
w = chr(encoded.pop(0))
result.append(w)
code = 256
for k in encoded:
if k in dictionary:
entry = dictionary[k]
elif k == code:
entry = w + w[0]
else:
raise ValueError("Invalid compressed code")
result.append(entry)
dictionary[code] = w + entry[0]
code += 1
w = entry
return "".join(result)
# Example usage
data = "TOBEORNOTTOBEORTOBEORNOT"
encoded = lzw_encode(data)
decoded = lzw_decode(encoded)
print(f"Original: {data}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
print(f"Original == Decoded: {data == decoded}")
This implementation includes both encoding and decoding functions for the LZW algorithm. The lzw_encode
function compresses the input string, while lzw_decode
reconstructs the original data from the compressed format.
5. Performance Analysis and Optimization
When implementing compression algorithms, it’s crucial to analyze their performance in terms of compression ratio, time complexity, and space complexity. Here are some key considerations:
- Compression ratio: Measure the ratio of compressed size to original size for different types of input data.
- Time complexity: Analyze the running time of encoding and decoding operations for various input sizes.
- Space complexity: Consider the memory usage of the algorithm, especially for large inputs.
- Trade-offs: Understand the trade-offs between compression ratio, speed, and memory usage.
To optimize the performance of compression algorithms, consider the following techniques:
- Use efficient data structures: Implement priority queues, hash tables, or trees to improve algorithm efficiency.
- Parallel processing: Utilize multi-threading or distributed computing for large-scale compression tasks.
- Adaptive compression: Dynamically adjust compression parameters based on input characteristics.
- Preprocessing: Apply techniques like sorting or filtering to improve compression effectiveness.
- Hardware acceleration: Leverage GPU or specialized hardware for compute-intensive operations.
6. Real-World Applications
Data compression algorithms find applications in various domains:
- File compression: ZIP, RAR, and other archive formats use combinations of compression algorithms.
- Image compression: JPEG, PNG, and WebP formats employ different compression techniques.
- Video compression: MPEG, H.264, and newer codecs use advanced compression algorithms.
- Audio compression: MP3, AAC, and other formats compress audio data for efficient storage and streaming.
- Network protocols: HTTP compression and VoIP codecs optimize data transmission over networks.
- Database systems: Compression techniques are used to reduce storage requirements and improve query performance.
- Backup and archiving: Efficient compression is crucial for long-term data storage and retrieval.
Understanding these applications can help you appreciate the importance of compression algorithms in modern computing and guide your focus when preparing for technical interviews.
7. Future Trends in Data Compression
As data volumes continue to grow exponentially, the field of data compression is evolving to meet new challenges. Some emerging trends include:
- Machine learning-based compression: Utilizing neural networks and other ML techniques to achieve better compression ratios.
- Context-aware compression: Adapting compression strategies based on the semantic content of the data.
- Quantum data compression: Exploring quantum algorithms for potentially exponential improvements in compression efficiency.
- Edge computing compression: Developing lightweight compression techniques suitable for IoT devices and edge nodes.
- DNA data storage: Investigating compression methods for storing digital information in DNA molecules.
Staying informed about these trends can give you an edge in technical interviews and help you contribute to cutting-edge projects in your future career.
8. Conclusion
Mastering data compression algorithms is an essential skill for aspiring software engineers and computer scientists. By understanding the principles behind various compression techniques and implementing them from scratch, you’ll develop a deeper appreciation for algorithmic thinking and problem-solving.
As you prepare for technical interviews at top tech companies, remember that compression algorithms often appear in coding challenges and system design questions. The knowledge and skills you’ve gained from this guide will serve you well in tackling such problems and demonstrating your expertise to potential employers.
Continue to practice implementing and optimizing these algorithms, and explore their applications in real-world scenarios. With dedication and hands-on experience, you’ll be well-equipped to excel in your coding interviews and contribute to the ever-evolving field of data compression in your future career.