Implementing Algorithms for Automated Testing: A Comprehensive Guide
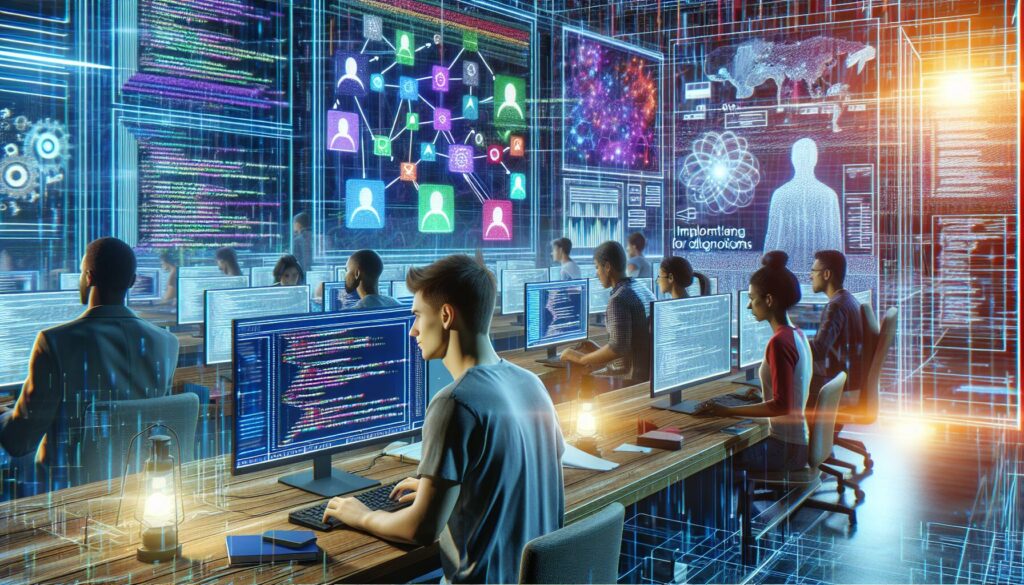
In the world of software development, automated testing has become an indispensable practice. It ensures code quality, reduces human error, and accelerates the development process. At the heart of effective automated testing lies the implementation of robust algorithms. This comprehensive guide will explore the intricacies of implementing algorithms for automated testing, providing you with the knowledge and tools to enhance your testing practices.
Table of Contents
- Introduction to Automated Testing
- The Importance of Algorithms in Automated Testing
- Common Algorithms Used in Automated Testing
- Implementing Algorithms for Test Case Generation
- Algorithms for Efficient Test Execution
- Algorithmic Approaches to Test Result Analysis
- Leveraging AI and Machine Learning in Automated Testing
- Best Practices for Algorithm Implementation in Testing
- Challenges and Solutions in Algorithmic Testing
- Future Trends in Algorithmic Automated Testing
- Conclusion
1. Introduction to Automated Testing
Automated testing is the practice of using software tools to execute pre-scripted tests on a software application. It involves the use of automated testing tools to write and run tests, compare actual outcomes with predicted outcomes, set up test preconditions, and other test control and test reporting functions. The primary goal of automated testing is to reduce the need for manual testing efforts, increase test coverage, and improve the overall quality of software products.
Key benefits of automated testing include:
- Increased efficiency and speed of testing processes
- Improved accuracy and consistency in test execution
- Enhanced test coverage across different scenarios
- Early detection of bugs and issues in the development cycle
- Cost reduction in the long run
- Ability to perform tests that are difficult or impossible to do manually
As we delve deeper into the world of automated testing, we’ll explore how algorithms play a crucial role in making these benefits a reality.
2. The Importance of Algorithms in Automated Testing
Algorithms are the backbone of automated testing. They provide the logical structure and decision-making processes that guide test case generation, execution, and result analysis. The importance of algorithms in automated testing cannot be overstated for several reasons:
Efficiency and Optimization
Well-designed algorithms can significantly improve the efficiency of test execution. They can optimize test case selection, reduce redundant tests, and minimize the time required for test runs.
Scalability
As software systems grow in complexity, the number of possible test scenarios increases exponentially. Algorithms allow for the creation and management of large-scale test suites that can adapt to growing system requirements.
Consistency and Reliability
Algorithms ensure that tests are executed consistently across different environments and iterations. This reliability is crucial for identifying regressions and maintaining software quality over time.
Intelligent Test Case Generation
Advanced algorithms can generate test cases based on various criteria, such as code coverage, risk assessment, or historical data. This leads to more comprehensive and targeted testing strategies.
Automated Decision Making
During test execution, algorithms can make real-time decisions on test flow, data selection, and error handling, mimicking complex user interactions and system behaviors.
Result Analysis and Reporting
Algorithmic approaches to result analysis can quickly process large volumes of test data, identify patterns, and generate insightful reports that help developers pinpoint issues more effectively.
3. Common Algorithms Used in Automated Testing
Several algorithms have proven particularly useful in the context of automated testing. Let’s explore some of the most common ones:
3.1 Boundary Value Analysis (BVA)
BVA is a technique used to test the boundaries of input domains. It’s based on the principle that errors often occur at the edges of input ranges.
function generateBoundaryValues(min, max) {
return [
min - 1, // Just below minimum
min, // Minimum
min + 1, // Just above minimum
max - 1, // Just below maximum
max, // Maximum
max + 1 // Just above maximum
];
}
3.2 Equivalence Partitioning
This technique divides the input domain into classes of data that should be processed similarly by the system.
function generateEquivalenceClasses(min, max) {
const range = max - min;
const partitionSize = range / 3;
return [
min + partitionSize / 2, // Low partition
min + partitionSize * 1.5, // Middle partition
max - partitionSize / 2 // High partition
];
}
3.3 Decision Table Testing
Decision tables are used to test system behavior for different input combinations, especially useful for complex business logic.
function createDecisionTable(conditions, actions) {
const combinations = 2 ** conditions.length;
let table = [];
for (let i = 0; i < combinations; i++) {
let row = {};
for (let j = 0; j < conditions.length; j++) {
row[conditions[j]] = !!(i & (1 << j));
}
for (let action of actions) {
row[action] = evaluateAction(row, action);
}
table.push(row);
}
return table;
}
3.4 State Transition Testing
This algorithm is used to test systems that can be in different states and transition between them based on inputs or events.
class StateMachine {
constructor(initialState) {
this.currentState = initialState;
this.transitions = new Map();
}
addTransition(fromState, event, toState) {
if (!this.transitions.has(fromState)) {
this.transitions.set(fromState, new Map());
}
this.transitions.get(fromState).set(event, toState);
}
processEvent(event) {
const stateTransitions = this.transitions.get(this.currentState);
if (stateTransitions && stateTransitions.has(event)) {
this.currentState = stateTransitions.get(event);
return true;
}
return false;
}
}
3.5 Path Coverage Algorithm
This algorithm aims to generate test cases that cover all possible paths through a program’s control flow graph.
function generateAllPaths(graph, start, end, path = []) {
path = path.concat(start);
if (start === end) {
return [path];
}
if (!graph[start]) {
return [];
}
let paths = [];
for (let node of graph[start]) {
if (!path.includes(node)) {
let newPaths = generateAllPaths(graph, node, end, path);
paths = paths.concat(newPaths);
}
}
return paths;
}
4. Implementing Algorithms for Test Case Generation
Implementing algorithms for test case generation is a critical aspect of automated testing. It involves creating a systematic approach to produce test cases that cover various scenarios and edge cases. Let’s explore some key strategies and implementations:
4.1 Combinatorial Testing
Combinatorial testing is an efficient technique for testing multiple input parameters. It generates test cases that cover all possible combinations of input values, typically using algorithms like All-Pairs or Orthogonal Array Testing.
function generatePairwiseCombinations(parameters) {
let combinations = [];
for (let i = 0; i < parameters.length - 1; i++) {
for (let j = i + 1; j < parameters.length; j++) {
for (let value1 of parameters[i]) {
for (let value2 of parameters[j]) {
combinations.push({[i]: value1, [j]: value2});
}
}
}
}
return combinations;
}
4.2 Data-Driven Test Generation
Data-driven testing involves creating test cases based on data sources. This approach is particularly useful for testing systems with large datasets or complex data relationships.
function generateDataDrivenTests(dataSource, testTemplate) {
return dataSource.map(data => {
let test = Object.assign({}, testTemplate);
for (let key in data) {
test.input[key] = data[key];
test.expectedOutput = calculateExpectedOutput(data);
}
return test;
});
}
4.3 Model-Based Test Generation
Model-based testing involves creating a model of the system under test and generating test cases based on this model. This approach is particularly effective for complex systems with many states and transitions.
class ModelBasedTestGenerator {
constructor(model) {
this.model = model;
}
generateTests() {
let tests = [];
for (let state of this.model.states) {
for (let transition of this.model.getTransitions(state)) {
tests.push({
initialState: state,
action: transition.action,
expectedFinalState: transition.targetState
});
}
}
return tests;
}
}
4.4 Mutation Testing
Mutation testing involves making small changes (mutations) to the source code and generating tests that can detect these changes. This helps in assessing the quality of existing tests and generating new ones.
function generateMutations(sourceCode) {
let mutations = [];
// Example: Replace arithmetic operators
const operators = ['+', '-', '*', '/'];
for (let i = 0; i < sourceCode.length; i++) {
if (operators.includes(sourceCode[i])) {
for (let op of operators) {
if (op !== sourceCode[i]) {
let mutatedCode = sourceCode.slice(0, i) + op + sourceCode.slice(i + 1);
mutations.push(mutatedCode);
}
}
}
}
return mutations;
}
4.5 Fuzz Testing
Fuzz testing involves generating random or semi-random input data to test the system’s robustness and error handling capabilities.
function generateFuzzTestCases(inputSchema, count) {
let testCases = [];
for (let i = 0; i < count; i++) {
let testCase = {};
for (let field in inputSchema) {
testCase[field] = generateRandomValue(inputSchema[field]);
}
testCases.push(testCase);
}
return testCases;
}
function generateRandomValue(schema) {
switch(schema.type) {
case 'string':
return Math.random().toString(36).substring(7);
case 'number':
return Math.random() * (schema.max - schema.min) + schema.min;
case 'boolean':
return Math.random() > 0.5;
// Add more types as needed
}
}
5. Algorithms for Efficient Test Execution
Efficient test execution is crucial for maintaining a fast and effective automated testing process. Here are some algorithmic approaches to optimize test execution:
5.1 Test Case Prioritization
This algorithm orders test cases based on criteria such as importance, historical failure rate, or code coverage to ensure that the most critical tests are run first.
function prioritizeTests(tests, criteria) {
return tests.sort((a, b) => {
let scoreA = calculateTestScore(a, criteria);
let scoreB = calculateTestScore(b, criteria);
return scoreB - scoreA; // Higher score first
});
}
function calculateTestScore(test, criteria) {
let score = 0;
if (criteria.importance) score += test.importance * criteria.importance;
if (criteria.failureRate) score += test.failureRate * criteria.failureRate;
if (criteria.coverage) score += test.coverage * criteria.coverage;
return score;
}
5.2 Parallel Test Execution
This algorithm distributes tests across multiple threads or machines to reduce overall execution time.
async function runTestsInParallel(tests, maxConcurrency) {
let results = [];
let running = 0;
let queue = [...tests];
while (queue.length > 0 || running > 0) {
while (running < maxConcurrency && queue.length > 0) {
let test = queue.shift();
running++;
runTest(test).then(result => {
results.push(result);
running--;
});
}
await new Promise(resolve => setTimeout(resolve, 100)); // Wait a bit
}
return results;
}
async function runTest(test) {
// Actual test execution logic here
return { test, result: 'pass' }; // Example result
}
5.3 Test Suite Reduction
This algorithm identifies and removes redundant or less valuable tests to minimize execution time without significantly impacting test coverage.
function reduceTestSuite(tests, coverageMap) {
let reducedSuite = [];
let coveredFeatures = new Set();
for (let test of tests) {
let newFeatures = test.coveredFeatures.filter(f => !coveredFeatures.has(f));
if (newFeatures.length > 0) {
reducedSuite.push(test);
newFeatures.forEach(f => coveredFeatures.add(f));
}
}
return reducedSuite;
}
5.4 Adaptive Test Selection
This algorithm dynamically selects which tests to run based on recent code changes or test history.
function selectTestsToRun(allTests, codeChanges, testHistory) {
return allTests.filter(test => {
// Run if affected by code changes
if (isTestAffectedByChanges(test, codeChanges)) return true;
// Run if recently failed
if (hasRecentlyFailed(test, testHistory)) return true;
// Run periodically even if not affected
if (shouldRunPeriodically(test, testHistory)) return true;
return false;
});
}
6. Algorithmic Approaches to Test Result Analysis
Analyzing test results effectively is crucial for identifying issues, understanding system behavior, and improving the testing process. Here are some algorithmic approaches to test result analysis:
6.1 Failure Clustering
This algorithm groups similar test failures together to help identify common issues or patterns.
function clusterFailures(failures, similarityThreshold) {
let clusters = [];
for (let failure of failures) {
let added = false;
for (let cluster of clusters) {
if (calculateSimilarity(failure, cluster[0]) > similarityThreshold) {
cluster.push(failure);
added = true;
break;
}
}
if (!added) {
clusters.push([failure]);
}
}
return clusters;
}
function calculateSimilarity(failure1, failure2) {
// Implement similarity calculation logic
// This could be based on error messages, stack traces, etc.
}
6.2 Regression Detection
This algorithm compares current test results with historical data to identify new failures or regressions.
function detectRegressions(currentResults, historicalResults) {
let regressions = [];
for (let test in currentResults) {
if (currentResults[test] === 'fail' && historicalResults[test] === 'pass') {
regressions.push(test);
}
}
return regressions;
}
6.3 Coverage Analysis
This algorithm analyzes which parts of the code were exercised by the tests and identifies areas with insufficient coverage.
function analyzeCoverage(coverageData, threshold) {
let insufficientCoverage = [];
for (let module in coverageData) {
if (coverageData[module] < threshold) {
insufficientCoverage.push(module);
}
}
return insufficientCoverage;
}
6.4 Performance Trend Analysis
This algorithm tracks performance metrics over time to identify trends or degradations.
function analyzePerformanceTrend(performanceData) {
let trends = {};
for (let metric in performanceData[0]) {
let values = performanceData.map(data => data[metric]);
trends[metric] = calculateTrend(values);
}
return trends;
}
function calculateTrend(values) {
// Implement trend calculation (e.g., linear regression)
// Return trend information (e.g., slope, correlation)
}
7. Leveraging AI and Machine Learning in Automated Testing
Artificial Intelligence (AI) and Machine Learning (ML) are revolutionizing automated testing by introducing more intelligent and adaptive testing strategies. Here are some ways AI and ML are being applied in testing:
7.1 Predictive Test Selection
ML models can predict which tests are most likely to fail based on code changes and historical data, allowing for more targeted testing.
class PredictiveTestSelector {
constructor(model) {
this.model = model; // Pretrained ML model
}
selectTests(allTests, codeChanges) {
return allTests.filter(test => {
let features = this.extractFeatures(test, codeChanges);
let failureProbability = this.model.predict(features);
return failureProbability > 0.5; // Threshold for selection
});
}
extractFeatures(test, codeChanges) {
// Extract relevant features for the ML model
// This could include test history, code change metrics, etc.
}
}
7.2 Automated Test Case Generation
AI can generate test cases by learning from existing code, documentation, and user behavior patterns.
class AITestCaseGenerator {
constructor(model) {
this.model = model; // AI model for test generation
}
generateTestCases(functionSignature, description) {
let prompt = `Generate test cases for:
Function: ${functionSignature}
Description: ${description}`;
return this.model.generateText(prompt);
}
parseGeneratedTests(generatedText) {
// Parse the AI-generated text into structured test cases
}
}
7.3 Intelligent Test Data Generation
ML models can generate realistic and diverse test data that covers a wide range of scenarios.
class IntelligentDataGenerator {
constructor(model) {
this.model = model; // Trained on real data patterns
}
generateTestData(schema, count) {
let generatedData = [];
for (let i = 0; i < count; i++) {
let dataPoint = {};
for (let field in schema) {
dataPoint[field] = this.model.generateValue(field, schema[field]);
}
generatedData.push(dataPoint);
}
return generatedData;
}
}
7.4 Anomaly Detection in Test Results
AI can identify unusual patterns or outliers in test results that might indicate bugs or system issues.
class AnomalyDetector {
constructor(model) {
this.model = model; // Trained anomaly detection model
}
detectAnomalies(testResults) {
let anomalies = [];
for (let result of testResults) {
let features = this.extractFeatures(result);
if (this.model.isAnomaly(features)) {
anomalies.push(result);
}
}
return anomalies;
}
extractFeatures(testResult) {
// Extract relevant features for anomaly detection
}
}
8. Best Practices for Algorithm Implementation in Testing
Implementing algorithms for automated testing requires careful consideration and adherence to best practices. Here are some key guidelines to follow:
8.1 Modularity and Reusability
Design your algorithms as modular components that can be easily reused across different testing scenarios.
class TestAlgorithm {
constructor(config) {
this.config = config;
}
execute(input) {
// Algorithm logic here
}
// Helper methods
preprocess(data) { /* ... */ }
postprocess(result) { /* ... */ }
}
// Usage
let algorithm = new TestAlgorithm({ /* config */ });
let result = algorithm.execute(testData);
8.2 Scalability
Ensure that your algorithms can handle increasing amounts of data and complexity as your test suite grows.
function scalableAlgorithm(data) {
const CHUNK_SIZE = 1000;
let results = [];
for (let i = 0; i < data.length; i += CHUNK_SIZE) {
let chunk = data.slice(i, i + CHUNK_SIZE);
results = results.concat(processChunk(chunk));
}
return results;
}
function processChunk(chunk) {
// Process a manageable chunk of data
}
8.3 Performance Optimization
Optimize your algorithms for performance to ensure fast execution of tests, especially for large test suites.
function optimizedSearch(array, target) {
let left = 0;
let right = array.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (array[mid] === target) return mid;
if (array[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
8.4 Error Handling and Logging
Implement robust error handling and logging mechanisms to facilitate debugging and maintenance.
function robustAlgorithm(input) {
try {
validateInput(input);
let result = processInput(input);
logSuccess(result);
return result;
} catch (error) {
logError(error);
throw new Error(`Algorithm failed: ${error.message}`);
}
}
function validateInput(input) { /* ... */ }
function processInput(input) { /* ... */ }
function logSuccess(result) { /* ... */ }
function logError(error) { /* ... */ }
8.5 Configurability
Make your algorithms configurable to adapt to different testing needs and environments.
class ConfigurableAlgorithm {
constructor(options) {
this.options = Object.assign({}, this.defaultOptions, options);
}
get defaultOptions() {
return {
threshold: 0.5,
maxIterations: 1000,
// other default options
};
}
execute(data) {
// Use this.options in the algorithm logic
}
}
// Usage
let algorithm = new ConfigurableAlgorithm({ threshold: 0.7, maxIterations: 500 });
algorithm.execute(testData);
9. Challenges and Solutions in Algorithmic Testing
Implementing algorithms for automated testing comes with its own set of challenges. Here are some common issues and potential solutions:
9.1 Challenge: Handling Complex Test Scenarios
Solution: Use composite design patterns to build complex test scenarios from simpler components.
class TestStep {
execute() { /* ... */ }
}
class TestScenario {
constructor() {
this.steps = [];
}
addStep(step) {
this.steps.push(step);
}
execute() {
for (let step of this.steps) {
step.execute();
}
}
}
// Usage
let scenario = new TestScenario();
scenario.addStep(new LoginStep());
scenario.addStep(new NavigateStep());
scenario.addStep(new VerificationStep());
scenario.execute();
9.2 Challenge: Maintaining Test Data
Solution: Implement a data management system that separates test data from test logic.
class TestDataManager {
constructor() {
this.data = new Map();
}
load(source) {
// Load data from file, database, etc.
}
get(key) {
return this.data.get(key);
}
set(key, value) {
this.data.set(key, value);
}
}
// Usage
let dataManager = new TestDataManager();
dataManager.load('test_data.json');
let userData = dataManager.get('user1');
9.3 Challenge: Dealing with Flaky Tests
Solution: Implement retry mechanisms and analyze patterns in flaky tests.
async function runWithRetry(testFunc, maxRetries = 3) {
for (let attempt = 1; attempt <= maxRetries; attempt++) {
try {
return await testFunc();
} catch (error) {
if (attempt === maxRetries) throw error;
console.log(`Attempt ${attempt} failed, retrying...`);
await new Promise(resolve => setTimeout(resolve, 1000)); // Wait before retry
}
}
}
// Usage
await runWithRetry(async () => {
// Your potentially flaky test here
});
9.4 Challenge: Maintaining Test Coverage as Code Evolves
Solution: Implement continuous integration with automated coverage analysis.
class CoverageAnalyzer {
analyzeCoverage(testResults) {
let coverage = this.calculateCoverage(testResults);
this.reportCoverage(coverage);
this.alertIfBelowThreshold(coverage);
}
calculateCoverage(testResults) { /* ... */ }
reportCoverage(coverage) { /* ... */ }
alertIfBelowThreshold(coverage) { /* ... */ }
}
// Usage in CI pipeline
let analyzer = new CoverageAnalyzer();
analyzer.analyzeCoverage(testResults);
9.5 Challenge: Balancing Test Depth and Execution Time
Solution: Implement smart test selection algorithms that prioritize critical tests.
class TestPrioritizer {
prioritizeTests(tests, timeConstraint) {
let prioritizedTests = tests.sort((a, b) =>
this.calculatePriority(b) - this.calculatePriority(a)
);
let selectedTests = [];
let totalTime = 0;
for (let test of prioritizedTests) {
if (totalTime + test.estimatedTime <= timeConstraint) {
selectedTests.push(test);
totalTime += test.estimatedTime;
} else {
break;
}
}
return selectedTests;
}
calculatePriority(test) {
// Calculate based on factors like importance, recent failures, code changes
}
}
// Usage
let prioritizer = new TestPrioritizer();
let testsToRun = prioritizer.prioritizeTests(allTests, 60 * 60); // 1 hour time constraint
10. Future Trends in Algorithmic Automated Testing
As technology continues to evolve, so do the trends in algorithmic automated testing. Here are some emerging trends and how they might shape the future of testing:
10.1 AI-Driven Test Generation and Execution
AI will play an increasingly significant role in generating and executing tests, potentially creating more comprehensive and efficient test suites than human testers.
class AITestGenerator {
constructor(aiModel) {
this.aiModel = aiModel;
}
generateTests(codebase) {
let codeAnalysis = this.analyzeCode(codebase);
return this.aiModel.generateTestSuite(codeAnalysis);
}
analyzeCode(codebase) {
// Perform static code analysis
}
}
// Future usage
let generator = new AITestGenerator(advancedAIModel);
let tests = generator.generateTests(projectCodebase);
10.2 Self-Healing Tests
Tests that can automatically adapt to minor UI changes or system updates, reducing maintenance overhead.
class SelfHealingTest {
constructor(testLogic, uiMap) {
this.testLogic = testLogic;
this.uiMap = uiMap;
}
async run() {
try {
await this.testLogic();
} catch (error) {
if (this.canHeal(error)) {
await this.healAndRetry();
} else {
throw error;
}
}
}
canHeal(error)