Identifying Problems That Need Binary Search Optimization
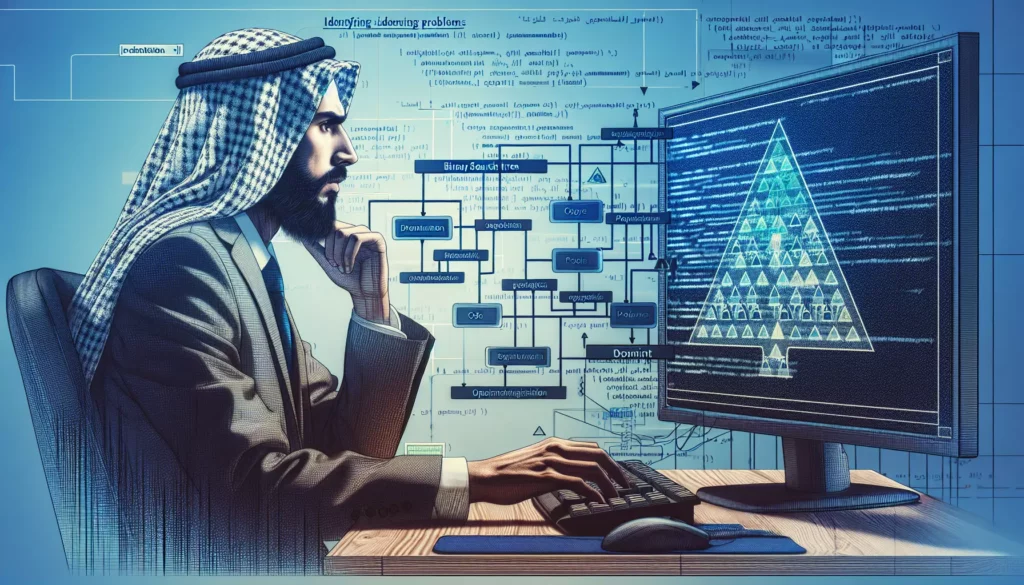
In the world of coding and algorithmic problem-solving, efficiency is key. As aspiring developers and seasoned programmers alike strive to optimize their code, one powerful tool stands out: binary search. This elegant algorithm can dramatically reduce search times in certain scenarios, but knowing when to apply it is crucial. In this comprehensive guide, we’ll explore how to identify problems that can benefit from binary search optimization, understand its applications, and learn to recognize scenarios where it shines.
Understanding Binary Search
Before we dive into identifying problems suitable for binary search, let’s briefly recap what binary search is and how it works.
Binary search is a highly efficient algorithm used to locate a specific element within a sorted array. Unlike linear search, which checks each element sequentially, binary search repeatedly divides the search interval in half. This divide-and-conquer approach allows it to achieve a time complexity of O(log n), making it significantly faster than linear search for large datasets.
Here’s a simple implementation of binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Key Characteristics of Binary Search Problems
To effectively identify problems that can benefit from binary search optimization, it’s essential to recognize the key characteristics that make a problem suitable for this algorithm. Here are the primary factors to consider:
1. Sorted Data
The most fundamental requirement for applying binary search is that the data must be sorted. This sorting can be in ascending or descending order, but it must be consistent throughout the dataset. If the data isn’t sorted, you’ll need to sort it first, which may negate the performance benefits of binary search for small datasets.
2. Random Access
Binary search requires the ability to access elements at any index in constant time. This is typically satisfied by data structures like arrays or array-like structures that support indexing.
3. Comparison-Based Problem
The problem should involve comparing elements to determine if you’ve found the target or which direction to search next. This comparison should yield a clear “less than,” “greater than,” or “equal to” result.
4. Search Space Reduction
Effective binary search problems allow you to eliminate half of the remaining search space with each comparison. This is what gives binary search its logarithmic time complexity.
5. Well-Defined Search Boundaries
You should be able to clearly define the initial search boundaries (e.g., the start and end of an array) and update these boundaries as the search progresses.
Common Problem Types Suitable for Binary Search
Now that we understand the key characteristics, let’s explore some common types of problems where binary search can be effectively applied:
1. Finding a Specific Element in a Sorted Array
This is the classic application of binary search. When you need to find a particular value in a sorted array, binary search is often the go-to solution.
Example problem: Given a sorted array of integers and a target value, determine if the target exists in the array and return its index if found.
2. Finding the Insertion Point for a New Element
Binary search can be modified to find the correct position to insert a new element into a sorted array while maintaining its order.
Example problem: Given a sorted array and a target value, return the index where the target should be inserted to maintain the array’s sorted order.
3. Finding the First or Last Occurrence of an Element
When dealing with sorted arrays that may contain duplicate elements, binary search can be adapted to find the first or last occurrence of a specific value.
Example problem: In a sorted array with duplicate elements, find the index of the first (or last) occurrence of a given target value.
4. Search in Rotated Sorted Array
A variation of binary search can be used to search in a sorted array that has been rotated around a pivot point.
Example problem: Given a sorted array that has been rotated an unknown number of times, find a target element in the array.
5. Finding a Peak Element
Binary search can be applied to find a peak element in an array, where a peak is defined as an element greater than its neighbors.
Example problem: Find any peak element in an array where an element is greater than its adjacent elements.
6. Square Root Calculation
Binary search can be used to efficiently calculate the square root of a number to a specified precision.
Example problem: Implement a function to calculate the square root of a given number with a specified precision using binary search.
7. Minimizing Maximum or Maximizing Minimum
Many optimization problems involve finding a value that minimizes the maximum or maximizes the minimum of some quantity. Binary search on the answer space can often be applied in these scenarios.
Example problem: Given an array of integers representing the difficulty of tasks and a number of workers, find the minimum difficulty such that each worker can be assigned a contiguous subarray of tasks with total difficulty not exceeding this minimum.
Identifying Binary Search Opportunities in Complex Problems
While some problems explicitly call for binary search, others may require more analysis to recognize the opportunity for optimization. Here are some strategies to help you identify less obvious binary search applications:
1. Look for Monotonic Properties
If you can identify a monotonic relationship in the problem space (i.e., a property that is consistently increasing or decreasing), there might be an opportunity to apply binary search.
2. Consider the Search Space
Even if the problem doesn’t involve a sorted array, consider whether the solution space itself can be treated as a sorted range. This is often the case in optimization problems where you’re searching for a specific value that satisfies certain conditions.
3. Analyze the Decision Problem
Many optimization problems can be converted into decision problems. If you can efficiently answer “Is there a solution better than X?”, you might be able to use binary search to find the optimal solution.
4. Look for Logarithmic Time Complexity Requirements
If a problem specifically asks for a solution with O(log n) time complexity, it’s a strong hint that binary search might be applicable.
5. Consider Preprocessing
Some problems may become suitable for binary search after preprocessing the input data, such as sorting an unsorted array.
Examples of Non-Obvious Binary Search Applications
Let’s examine some problems where the application of binary search might not be immediately apparent:
1. Median of Two Sorted Arrays
Problem: Given two sorted arrays of integers, find the median of the combined array without actually merging them.
Binary search application: You can use binary search to partition the arrays in a way that allows you to find the median element(s) efficiently.
2. Capacity To Ship Packages Within D Days
Problem: Given an array of package weights and a number of days, find the minimum capacity of the ship that can ship all packages within the given number of days.
Binary search application: You can binary search on the possible capacities and check for each capacity if it’s feasible to ship all packages within the given days.
3. Koko Eating Bananas
Problem: Koko loves to eat bananas. There are N piles of bananas, the i-th pile has piles[i] bananas. The guards have gone and will come back in H hours. Koko can decide her bananas-per-hour eating speed of K. Each hour, she chooses some pile of bananas, and eats K bananas from that pile. If the pile has less than K bananas, she eats all of them instead, and won’t eat any more bananas during this hour. Koko likes to eat slowly, but still wants to finish eating all the bananas before the guards come back. Return the minimum integer K such that she can eat all the bananas within H hours.
Binary search application: You can binary search on the possible eating speeds and check for each speed if Koko can finish all bananas within H hours.
4. Split Array Largest Sum
Problem: Given an array nums which consists of non-negative integers and an integer m, you can split the array into m non-empty continuous subarrays. Write an algorithm to minimize the largest sum among these m subarrays.
Binary search application: You can binary search on the possible largest sums and check for each sum if it’s possible to split the array into m subarrays with maximum subarray sum not exceeding this value.
Implementing Binary Search in Different Scenarios
Now that we’ve identified various scenarios where binary search can be applied, let’s look at how to implement binary search for some of these less obvious applications:
1. Square Root Calculation
def square_root(n, precision):
left, right = 0, n
while right - left > precision:
mid = (left + right) / 2
if mid * mid > n:
right = mid
else:
left = mid
return left
2. Capacity To Ship Packages Within D Days
def shipWithinDays(weights, D):
left, right = max(weights), sum(weights)
def canShip(capacity):
days = 1
current_load = 0
for weight in weights:
if current_load + weight > capacity:
days += 1
current_load = weight
else:
current_load += weight
if days > D:
return False
return True
while left < right:
mid = (left + right) // 2
if canShip(mid):
right = mid
else:
left = mid + 1
return left
3. Koko Eating Bananas
def minEatingSpeed(piles, H):
left, right = 1, max(piles)
def canEatAll(speed):
return sum((pile - 1) // speed + 1 for pile in piles) <= H
while left < right:
mid = (left + right) // 2
if canEatAll(mid):
right = mid
else:
left = mid + 1
return left
Common Pitfalls and How to Avoid Them
While binary search is a powerful tool, there are several common pitfalls that programmers should be aware of:
1. Off-by-One Errors
Binary search is notorious for off-by-one errors. Be careful when updating the left and right pointers, and make sure your termination condition is correct.
2. Integer Overflow
When calculating the middle index, using (left + right) // 2 can lead to integer overflow for large arrays. A safer alternative is left + (right – left) // 2.
3. Infinite Loops
Ensure that your search space is actually reducing in each iteration. If not, you may end up in an infinite loop.
4. Handling Duplicates
When dealing with arrays that may contain duplicates, standard binary search might not behave as expected. You may need to modify the algorithm to handle these cases correctly.
5. Floating-Point Precision
When applying binary search to floating-point numbers, be aware of precision issues. You may need to use a small epsilon value for comparisons.
Conclusion
Binary search is a versatile and powerful algorithm that can significantly optimize search operations and solve a wide range of problems efficiently. By understanding its key characteristics and recognizing the types of problems it can solve, you can leverage binary search to create more efficient solutions.
Remember, the key to mastering binary search lies not just in implementing the algorithm correctly, but in identifying when and how to apply it creatively to solve complex problems. As you encounter new coding challenges, always consider whether binary search might be applicable, even if it’s not immediately obvious.
Practice implementing binary search in various scenarios, and you’ll find that it becomes an invaluable tool in your problem-solving toolkit. Whether you’re preparing for coding interviews, optimizing existing systems, or tackling algorithmic challenges, the ability to identify and apply binary search optimization will serve you well in your journey as a programmer.