HTML5 Features: Revolutionizing Web Development
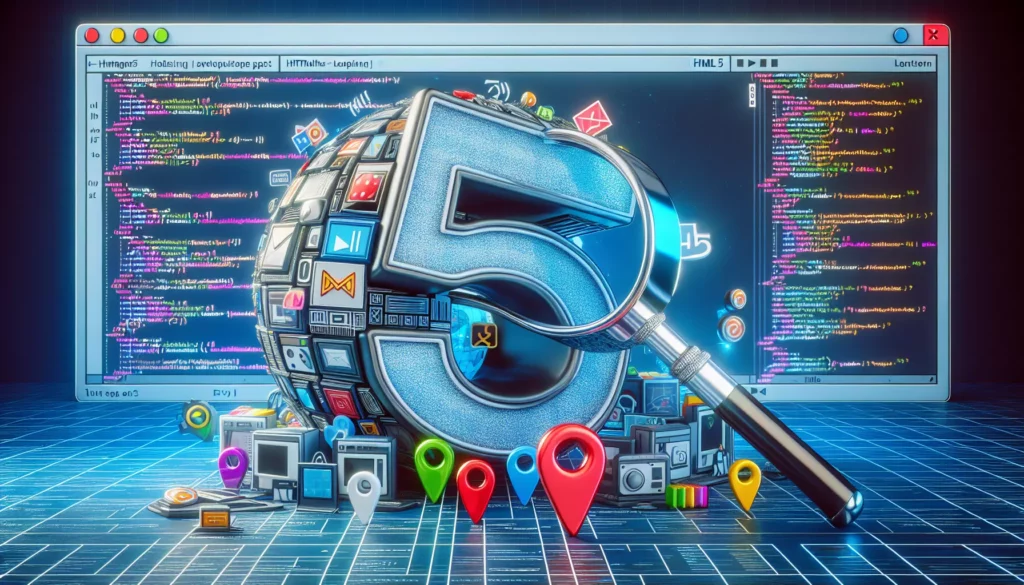
In the ever-evolving landscape of web development, HTML5 stands as a cornerstone technology that has revolutionized how we create and interact with web content. As part of our journey at AlgoCademy to empower aspiring developers with cutting-edge knowledge, we’re diving deep into the features of HTML5 that make it an indispensable tool in modern web development. Whether you’re a beginner taking your first steps in coding or an experienced developer preparing for technical interviews at major tech companies, understanding HTML5’s capabilities is crucial for your success.
What is HTML5?
HTML5 is the latest version of the Hypertext Markup Language, the standard language for structuring and presenting content on the World Wide Web. Released in 2014, HTML5 brought a plethora of new features and improvements that have significantly enhanced web development capabilities. It’s not just an upgrade; it’s a complete overhaul that addresses the needs of modern web applications.
Key Features of HTML5
Let’s explore the standout features that make HTML5 a game-changer in web development:
1. Semantic Elements
HTML5 introduced a set of semantic elements that provide meaning to the structure of web content. These elements make it easier for search engines to understand the content and improve accessibility for users with disabilities.
Some key semantic elements include:
- <header>
- <nav>
- <article>
- <section>
- <aside>
- <footer>
Here’s an example of how these semantic elements can be used:
<body>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<main>
<article>
<h2>Article Title</h2>
<p>Article content goes here...</p>
</article>
<aside>
<h3>Related Links</h3>
<ul>
<li><a href="#">Link 1</a></li>
<li><a href="#">Link 2</a></li>
</ul>
</aside>
</main>
<footer>
<p>© 2023 My Website. All rights reserved.</p>
</footer>
</body>
2. Multimedia Support
HTML5 provides native support for audio and video content without the need for plugins like Flash. This feature has significantly improved the user experience and reduced dependency on third-party software.
Here’s how you can embed video content using HTML5:
<video width="320" height="240" controls>
<source src="movie.mp4" type="video/mp4">
<source src="movie.ogg" type="video/ogg">
Your browser does not support the video tag.
</video>
And for audio:
<audio controls>
<source src="audio.mp3" type="audio/mpeg">
<source src="audio.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
3. Canvas and SVG
HTML5 introduced the <canvas> element, which allows for dynamic, scriptable rendering of 2D shapes and images. It’s widely used for creating games, data visualization, and other interactive features.
Here’s a simple example of drawing on a canvas:
<canvas id="myCanvas" width="200" height="100" style="border:1px solid #000000;">
</canvas>
<script>
var c = document.getElementById("myCanvas");
var ctx = c.getContext("2d");
ctx.moveTo(0, 0);
ctx.lineTo(200, 100);
ctx.stroke();
</script>
SVG (Scalable Vector Graphics) support was also improved in HTML5, allowing for resolution-independent graphics that can be styled with CSS and manipulated with JavaScript.
4. Offline Web Applications
HTML5 introduced the Application Cache (AppCache) feature, which allows web applications to work offline. This is particularly useful for creating progressive web apps (PWAs) that can function without an internet connection.
While AppCache is now deprecated, its successor, the Service Worker API, provides even more powerful offline capabilities:
// Register a service worker
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/sw.js').then(function(registration) {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(function(error) {
console.log('Service Worker registration failed:', error);
});
}
5. Geolocation
HTML5 provides a Geolocation API that allows web applications to access the user’s geographical location (with permission). This feature has enabled the development of location-based services directly within web browsers.
Here’s how you can use the Geolocation API:
if ("geolocation" in navigator) {
navigator.geolocation.getCurrentPosition(function(position) {
console.log("Latitude:", position.coords.latitude);
console.log("Longitude:", position.coords.longitude);
});
} else {
console.log("Geolocation is not supported by this browser.");
}
6. Web Storage
HTML5 introduced new methods for storing data on the client-side: localStorage and sessionStorage. These provide a more intuitive alternative to cookies for storing key-value pairs in the browser.
Using localStorage:
// Storing data
localStorage.setItem('username', 'John Doe');
// Retrieving data
let username = localStorage.getItem('username');
console.log(username); // Output: John Doe
// Removing data
localStorage.removeItem('username');
// Clearing all data
localStorage.clear();
7. Web Workers
Web Workers allow web applications to run scripts in background threads. This means you can perform time-consuming processing without affecting the performance of the page.
Here’s a simple example of using a Web Worker:
// In your main script
const worker = new Worker('worker.js');
worker.postMessage({num: 10});
worker.onmessage = function(e) {
console.log('Result: ' + e.data);
};
// In worker.js
self.onmessage = function(e) {
const result = e.data.num * 2;
self.postMessage(result);
};
8. Form Enhancements
HTML5 introduced several new input types and attributes that make form handling much easier and more user-friendly. These include:
- New input types: date, time, email, url, search, etc.
- Form validation attributes: required, pattern, min, max, etc.
- The <datalist> element for autocomplete functionality
Here’s an example showcasing some of these features:
<form>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="website">Website:</label>
<input type="url" id="website" name="website">
<label for="birthdate">Birthdate:</label>
<input type="date" id="birthdate" name="birthdate">
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="0" max="120">
<label for="favorite-color">Favorite Color:</label>
<input type="color" id="favorite-color" name="favorite-color">
<label for="browser">Choose your browser:</label>
<input list="browsers" name="browser" id="browser">
<datalist id="browsers">
<option value="Chrome">
<option value="Firefox">
<option value="Safari">
<option value="Edge">
</datalist>
<input type="submit" value="Submit">
</form>
9. Drag and Drop API
HTML5 introduced native drag and drop functionality, allowing users to click and hold on an element, drag it to a different location, and release the mouse button to drop it there.
Here’s a basic example of how to implement drag and drop:
<div id="div1" ondrop="drop(event)" ondragover="allowDrop(event)"></div>
<img id="drag1" src="img_logo.gif" draggable="true" ondragstart="drag(event)" width="336" height="69">
<script>
function allowDrop(ev) {
ev.preventDefault();
}
function drag(ev) {
ev.dataTransfer.setData("text", ev.target.id);
}
function drop(ev) {
ev.preventDefault();
var data = ev.dataTransfer.getData("text");
ev.target.appendChild(document.getElementById(data));
}
</script>
10. WebSockets
HTML5 introduced support for WebSockets, which provide a full-duplex, bidirectional communication channel between a client and a server. This allows for real-time data exchange, making it ideal for applications like chat systems, live sports updates, or collaborative tools.
Here’s a simple example of how to use WebSockets:
// Create WebSocket connection
const socket = new WebSocket('ws://example.com/socket');
// Connection opened
socket.addEventListener('open', function (event) {
socket.send('Hello Server!');
});
// Listen for messages
socket.addEventListener('message', function (event) {
console.log('Message from server ', event.data);
});
// Connection closed
socket.addEventListener('close', function (event) {
console.log('Server connection closed');
});
The Impact of HTML5 on Web Development
The introduction of HTML5 has had a profound impact on web development:
- Improved User Experience: Features like native video and audio support, offline capabilities, and improved forms have significantly enhanced the user experience on the web.
- Mobile-Friendly Development: HTML5’s features are designed with mobile devices in mind, making it easier to create responsive and mobile-friendly websites.
- Reduced Dependency on Plugins: With native support for multimedia and advanced graphics, HTML5 has reduced the need for plugins like Flash, improving security and performance.
- Better SEO: Semantic elements help search engines better understand the structure and content of web pages, potentially improving search rankings.
- Enhanced Performance: Features like Web Workers and efficient APIs have led to faster, more responsive web applications.
- Cross-Platform Compatibility: HTML5’s wide adoption means that web applications can run consistently across different platforms and devices.
HTML5 and Modern Web Development Frameworks
HTML5’s features have been embraced and extended by modern web development frameworks and libraries. For instance:
- React: Utilizes HTML5’s DOM manipulation capabilities to efficiently update the user interface.
- Angular: Leverages HTML5 features in its template syntax and form controls.
- Vue.js: Makes extensive use of HTML5’s custom data attributes for its directive system.
- Progressive Web Apps (PWAs): Built on HTML5 technologies like Service Workers for offline functionality and Web App Manifests for installability.
Preparing for Technical Interviews with HTML5 Knowledge
For those preparing for technical interviews, especially at major tech companies, a solid understanding of HTML5 is crucial. Here are some areas to focus on:
- Semantic HTML: Understand the importance and correct usage of semantic elements.
- Accessibility: Know how HTML5 features can be used to improve web accessibility.
- Performance Optimization: Understand how features like Web Workers and efficient APIs can be used to optimize web application performance.
- Browser Compatibility: Be aware of browser support for different HTML5 features and how to handle fallbacks.
- Security Considerations: Understand the security implications of features like local storage and how to use them safely.
- Integration with JavaScript: Know how to leverage HTML5 APIs in JavaScript for enhanced functionality.
Conclusion
HTML5 has revolutionized web development, providing powerful tools and APIs that enable developers to create rich, interactive web applications. Its features have become integral to modern web development practices and are essential knowledge for any aspiring web developer.
At AlgoCademy, we emphasize the importance of understanding these core web technologies. Whether you’re just starting your coding journey or preparing for technical interviews at top tech companies, a solid grasp of HTML5 and its capabilities will serve you well. As you continue to learn and grow, remember that HTML5 is just one piece of the puzzle. Combine it with CSS3 for styling, JavaScript for interactivity, and modern frameworks and libraries to create truly impressive web applications.
Keep exploring, keep coding, and keep pushing the boundaries of what’s possible on the web. The skills you develop here will be invaluable as you progress in your career as a web developer.