How to Write Good Commit Messages in Git: A Comprehensive Guide
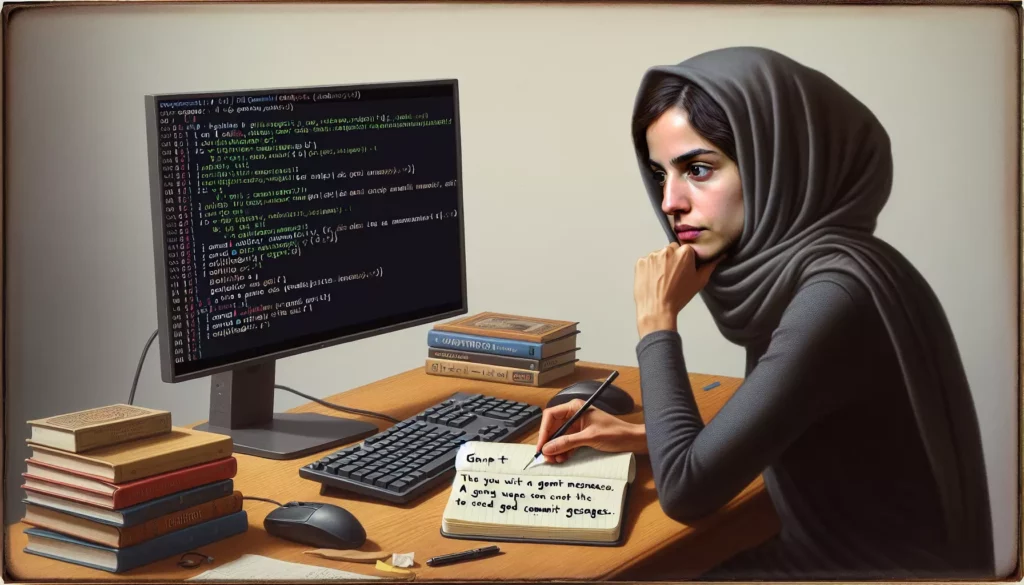
In the world of software development, version control is an essential practice, and Git has emerged as the industry standard. One crucial aspect of using Git effectively is writing clear, informative commit messages. Good commit messages not only help you and your team understand the history of your project but also make it easier to track changes, debug issues, and maintain your codebase. In this comprehensive guide, we’ll explore the art of crafting excellent commit messages in Git, providing you with best practices, tips, and examples to elevate your version control game.
Why Are Good Commit Messages Important?
Before diving into the specifics of writing good commit messages, let’s understand why they matter:
- Historical context: Well-written commit messages provide a clear history of your project’s evolution, making it easier to understand why certain changes were made.
- Collaboration: In team environments, good commit messages help other developers understand your changes without having to dig through the code.
- Debugging: When tracking down bugs, informative commit messages can help pinpoint when and why issues were introduced.
- Code reviews: Reviewers can better understand the purpose and context of your changes, leading to more effective code reviews.
- Automated tooling: Many tools, such as release note generators, rely on commit messages to create documentation automatically.
The Anatomy of a Good Commit Message
A well-structured commit message typically consists of three main parts:
- A short, concise subject line
- A blank line
- A more detailed description (body)
Here’s an example of a well-formatted commit message:
Fix login button not responding on mobile devices
- Add touch event listener to login button
- Adjust button size for better touch targets
- Update CSS media queries for mobile responsiveness
This commit addresses the issue where users on mobile devices
were unable to log in due to an unresponsive login button.
The changes improve the overall mobile user experience.
Now, let’s break down each component and discuss best practices for writing them effectively.
1. The Subject Line
The subject line is the most critical part of your commit message. It should be concise, descriptive, and to the point. Here are some guidelines for writing effective subject lines:
- Keep it short: Aim for 50 characters or less. This ensures that the subject line is fully visible in most Git interfaces without truncation.
- Use the imperative mood: Write as if you’re giving a command. For example, use “Fix” instead of “Fixed” or “Fixes.”
- Capitalize the first letter: Start your subject line with a capital letter.
- Don’t end with a period: Save punctuation for the commit message body.
- Be specific: Provide enough context to understand the change without looking at the code.
Examples of good subject lines:
- “Add user authentication feature”
- “Fix memory leak in image processing module”
- “Update dependencies to latest versions”
- “Refactor database connection handling”
2. The Blank Line
Always include a blank line between the subject line and the commit message body. This separation helps in parsing the commit message and improves readability.
3. The Commit Message Body
The body of your commit message is where you can provide more detailed information about the changes. Here are some tips for writing an effective commit message body:
- Explain the why, not just the what: While the code shows what changed, use the commit message to explain why the change was necessary.
- Use bullet points: For multiple related changes, use bullet points to improve readability.
- Keep lines short: Wrap lines at 72 characters to ensure proper formatting in various Git tools.
- Include relevant details: Mention related issue numbers, links to resources, or other pertinent information.
- Use proper grammar and punctuation: Write in complete sentences and use proper punctuation to enhance clarity.
Best Practices for Writing Commit Messages
Now that we’ve covered the structure of a good commit message, let’s dive into some best practices that will help you write more effective commit messages:
1. Be Consistent
Consistency is key when it comes to commit messages. Establish a standard format for your team or project and stick to it. This could include using specific prefixes for different types of changes, such as:
- “feat:” for new features
- “fix:” for bug fixes
- “docs:” for documentation changes
- “style:” for formatting changes
- “refactor:” for code refactoring
- “test:” for adding or modifying tests
- “chore:” for maintenance tasks
Example:
feat: Add user profile picture upload functionality
- Implement file upload component
- Add server-side image processing
- Update user model to include profile picture URL
This feature allows users to upload and display their profile
pictures, enhancing the personalization of user accounts.
2. Use the Present Tense
Write your commit messages in the present tense. This convention makes your commit history read like a series of actions being applied to the codebase. For example:
- Good: “Add feature X” or “Fix bug Y”
- Avoid: “Added feature X” or “Fixed bug Y”
3. Reference Issue Trackers
If your project uses an issue tracker, include relevant issue numbers in your commit messages. This helps link the code changes to specific issues or feature requests. For example:
Fix login page layout on small screens (Closes #123)
- Adjust container width for mobile devices
- Increase form input font size for better readability
- Center align submit button
This commit addresses the layout issues reported in issue #123,
improving the login experience on mobile devices.
4. Separate Concerns
Try to keep each commit focused on a single concern or change. If you’re working on multiple unrelated changes, consider splitting them into separate commits. This makes it easier to understand, review, and potentially revert changes if needed.
5. Avoid Redundancy
Don’t repeat information that’s already available in the diff. Instead, focus on explaining why the change was made and any non-obvious consequences of the change.
6. Use Conventional Commits
Consider adopting the Conventional Commits specification, which provides a standardized format for commit messages. This format is particularly useful for automated tooling and generating changelogs. The basic structure is:
<type>[optional scope]: <description>
[optional body]
[optional footer(s)]
Example:
feat(auth): implement two-factor authentication
- Add 2FA setup page
- Integrate with SMS service for OTP delivery
- Update login flow to include 2FA step
BREAKING CHANGE: Users will need to set up 2FA on their next login
Closes #456
Tools to Help Write Better Commit Messages
Several tools can assist you in writing and enforcing good commit messages:
1. Git Hooks
You can use Git hooks, particularly the commit-msg
hook, to enforce commit message standards. Here’s an example of a simple bash script that checks the commit message format:
#!/bin/bash
commit_msg_file=$1
commit_msg=$(cat "$commit_msg_file")
# Check if the commit message starts with a capital letter
if ! [[ $commit_msg =~ ^[A-Z] ]]; then
echo "Error: Commit message must start with a capital letter."
exit 1
fi
# Check if the subject line is under 50 characters
subject_line=$(echo "$commit_msg" | head -n1)
if [ ${#subject_line} -gt 50 ]; then
echo "Error: Subject line must be 50 characters or less."
exit 1
fi
# Additional checks can be added here
exit 0
Save this script as .git/hooks/commit-msg
and make it executable with chmod +x .git/hooks/commit-msg
.
2. Commitlint
Commitlint is a popular tool that checks if your commit messages meet the conventional commit format. You can integrate it with your CI/CD pipeline to enforce commit message standards across your team.
3. Commitizen
Commitizen is a command-line tool that prompts you to fill out required commit fields in a standardized way. It helps maintain consistency in commit messages across your project or team.
Examples of Good Commit Messages
Let’s look at some examples of good commit messages to inspire your own:
Refactor user authentication module
- Extract auth logic into separate service
- Implement JWT token-based authentication
- Add unit tests for new auth service
This refactoring improves the modularity of our authentication
system and paves the way for easier implementation of additional
auth methods in the future.
Fix race condition in concurrent file uploads (Fixes #789)
- Implement file locking mechanism
- Add retry logic for failed uploads
- Improve error handling and reporting
This fix addresses the intermittent file corruption issues
reported by users when uploading multiple files simultaneously.
Optimize database queries for product search
- Add indexes on frequently searched columns
- Rewrite JOIN queries to use subqueries
- Implement query result caching
These optimizations reduce the average search response time
from 2.5 seconds to 200ms, significantly improving user experience
for our e-commerce platform.
Common Mistakes to Avoid
When writing commit messages, be sure to avoid these common pitfalls:
- Vague messages: Avoid commit messages like “Fix bug” or “Update code” that don’t provide any meaningful information.
- Overly long subject lines: Keep your subject lines concise and to the point.
- Including redundant information: Don’t repeat what’s already visible in the diff, such as listing every file changed.
- Using passive voice: Stick to the active, imperative mood for clarity and consistency.
- Neglecting to explain why: Always strive to explain the reasoning behind your changes, not just what you changed.
Conclusion
Writing good commit messages is an essential skill for any developer working with Git. By following the guidelines and best practices outlined in this guide, you can significantly improve the quality of your commit history, making it easier for you and your team to understand, maintain, and collaborate on your codebase.
Remember, a well-crafted commit message is an investment in the future of your project. It may take a little extra time now, but it can save hours of confusion and debugging down the line. As you continue to work on your coding projects, whether it’s for personal growth, preparing for technical interviews, or collaborating on open-source initiatives, make it a habit to write clear, informative commit messages.
By mastering this skill, you’ll not only improve your own workflow but also contribute to a more efficient and collaborative development environment. So the next time you’re about to commit your changes, take a moment to craft a message that future you (and your teammates) will thank you for.