How to Write Clean and Readable Code Under Pressure: A Comprehensive Guide
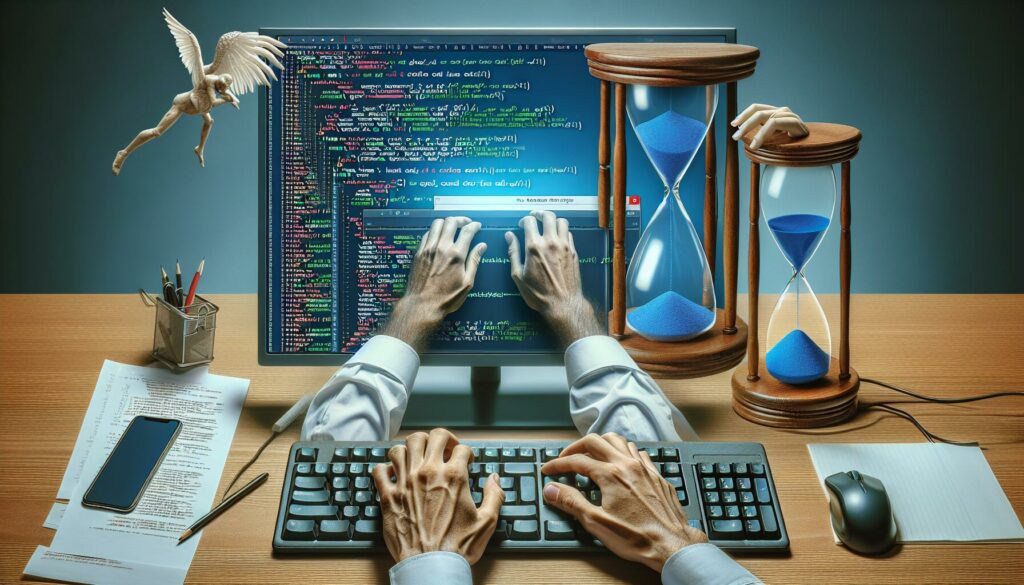
In the fast-paced world of software development, writing clean and readable code is a crucial skill that sets apart great programmers from the rest. This becomes even more critical when you’re under pressure, such as during a high-stakes technical interview or a tight project deadline. In this comprehensive guide, we’ll explore the importance of clean code and provide you with practical tips to maintain code quality even when the heat is on.
Table of Contents
- Why Clean Code Matters
- Mastering Naming Conventions
- The Art of Effective Commenting
- Embracing Simplicity
- Structuring Your Code for Readability
- Maintaining Consistency
- Refactoring on the Fly
- Writing Testable Code
- Leveraging Tools and IDE Features
- Practice Makes Perfect
- Conclusion
1. Why Clean Code Matters
Before diving into the specifics of writing clean code under pressure, it’s essential to understand why it matters in the first place. Clean code is not just about aesthetics; it’s about creating software that is maintainable, scalable, and less prone to bugs.
Benefits of Clean Code:
- Improved Readability: Clean code is easier to read and understand, not just for others but also for your future self.
- Easier Maintenance: When code is clean and well-organized, making changes or fixing bugs becomes much simpler.
- Reduced Cognitive Load: Clean code allows developers to focus on solving problems rather than deciphering complex structures.
- Better Collaboration: Team members can more easily work together when the codebase is clean and consistent.
- Faster Onboarding: New team members can get up to speed more quickly with a clean codebase.
- Improved Performance: Often, clean code leads to better performance as it’s easier to optimize and refactor.
In a high-pressure situation like a technical interview, writing clean code demonstrates your professionalism and attention to detail. It shows that you can produce high-quality work even under stress, which is a valuable skill in any development environment.
2. Mastering Naming Conventions
One of the most critical aspects of writing clean code is choosing appropriate names for variables, functions, and classes. Good naming can make your code self-documenting and reduce the need for excessive comments.
Tips for Effective Naming:
- Be Descriptive: Use names that clearly describe the purpose or content of the variable, function, or class.
- Use Intention-Revealing Names: The name should answer the question “Why does this exist?” or “What does it do?”
- Avoid Abbreviations: Unless they’re widely understood (e.g., ‘HTTP’), spell out words fully to avoid confusion.
- Use Consistent Conventions: Stick to a naming convention throughout your code (e.g., camelCase for variables, PascalCase for classes).
- Avoid Redundancy: Don’t repeat information that’s already clear from the context.
Here’s an example of how proper naming can improve code readability:
// Poor naming
function calc(a, b) {
return a * b;
}
// Better naming
function calculateRectangleArea(width, height) {
return width * height;
}
In the second example, it’s immediately clear what the function does and what the parameters represent, without needing any additional comments or context.
3. The Art of Effective Commenting
While clean code should be largely self-explanatory, comments still play a crucial role in explaining complex logic, documenting APIs, or providing context that isn’t immediately obvious from the code itself.
Guidelines for Effective Commenting:
- Comment on the Why, Not the What: The code itself should explain what it does. Use comments to explain why certain decisions were made.
- Keep Comments Updated: Outdated comments are worse than no comments at all. Always update comments when you change the corresponding code.
- Use Comments to Clarify Complex Logic: If a piece of code is necessarily complex, a brief comment can help other developers (or your future self) understand it quickly.
- Avoid Redundant Comments: Don’t state the obvious. If the code is self-explanatory, additional comments can clutter rather than clarify.
- Use Documentation Comments for Public APIs: For functions or classes that will be used by others, provide clear documentation on how to use them.
Here’s an example of effective commenting:
// Poor commenting
// This function calculates the factorial
function factorial(n) {
if (n === 0 || n === 1) return 1;
return n * factorial(n - 1);
}
// Better commenting
/**
* Calculates the factorial of a non-negative integer.
* @param {number} n - The number to calculate the factorial of.
* @returns {number} The factorial of n.
* @throws {Error} If n is negative or not an integer.
*/
function factorial(n) {
if (n < 0 || !Number.isInteger(n)) {
throw new Error("Input must be a non-negative integer");
}
if (n === 0 || n === 1) return 1;
return n * factorial(n - 1);
}
The second example provides useful information about the function’s behavior, parameters, return value, and potential errors, which is especially helpful for other developers who might use this function.
4. Embracing Simplicity
When under pressure, it’s tempting to come up with complex, clever solutions to problems. However, simplicity should be your goal. Simple code is easier to understand, debug, and maintain.
Strategies for Keeping Code Simple:
- KISS (Keep It Simple, Stupid): Always look for the simplest solution that solves the problem at hand.
- DRY (Don’t Repeat Yourself): Avoid duplicating code. If you find yourself writing similar code in multiple places, consider refactoring it into a reusable function.
- Single Responsibility Principle: Each function or class should have one, and only one, reason to change.
- Avoid Premature Optimization: Write clear, correct code first. Optimize only when necessary and after profiling.
- Use Built-in Functions and Libraries: Don’t reinvent the wheel. Utilize standard libraries and built-in functions when possible.
Here’s an example of simplifying code:
// Overly complex
function isEven(num) {
if (num % 2 === 0) {
return true;
} else {
return false;
}
}
// Simplified
function isEven(num) {
return num % 2 === 0;
}
The simplified version is more concise and easier to read, while still accomplishing the same task.
5. Structuring Your Code for Readability
The way you structure your code can greatly impact its readability. Good structure makes the flow of the program easier to follow and understand.
Tips for Better Code Structure:
- Use Meaningful Indentation: Consistent indentation helps visualize the code’s structure and hierarchy.
- Keep Functions Short: Aim for functions that do one thing and do it well. If a function gets too long, consider breaking it into smaller, more focused functions.
- Group Related Code: Keep related pieces of code close together. This could mean grouping related functions or keeping variable declarations close to where they’re used.
- Use Whitespace Effectively: Add blank lines between logical sections of code to improve readability.
- Follow a Logical Flow: Structure your code in a way that follows a natural, logical progression.
Here’s an example of improving code structure:
// Poor structure
function processOrder(order) {
let total = 0;
for (let item of order.items) {
total += item.price * item.quantity;
}
let tax = total * 0.1;
let shipping = total > 100 ? 0 : 10;
let grandTotal = total + tax + shipping;
console.log(`Order total: $${total}`);
console.log(`Tax: $${tax}`);
console.log(`Shipping: $${shipping}`);
console.log(`Grand total: $${grandTotal}`);
return grandTotal;
}
// Improved structure
function processOrder(order) {
const total = calculateTotal(order.items);
const tax = calculateTax(total);
const shipping = calculateShipping(total);
const grandTotal = total + tax + shipping;
logOrderDetails(total, tax, shipping, grandTotal);
return grandTotal;
}
function calculateTotal(items) {
return items.reduce((sum, item) => sum + item.price * item.quantity, 0);
}
function calculateTax(total) {
return total * 0.1;
}
function calculateShipping(total) {
return total > 100 ? 0 : 10;
}
function logOrderDetails(total, tax, shipping, grandTotal) {
console.log(`Order total: $${total}`);
console.log(`Tax: $${tax}`);
console.log(`Shipping: $${shipping}`);
console.log(`Grand total: $${grandTotal}`);
}
The improved structure breaks down the process into smaller, focused functions, making the code easier to read and maintain.
6. Maintaining Consistency
Consistency in your code style makes it easier to read and understand. When your code follows a consistent pattern, other developers (or your future self) can more quickly grasp its structure and intent.
Areas to Maintain Consistency:
- Naming Conventions: Use the same conventions for naming variables, functions, and classes throughout your code.
- Indentation and Formatting: Stick to a consistent style for indentation, bracket placement, and other formatting choices.
- File Organization: Organize your files and folders in a consistent manner across your project.
- Commenting Style: Use a consistent style for comments, including how you format function documentation.
- Error Handling: Handle errors consistently throughout your codebase.
Many development teams use style guides or linting tools to enforce consistency. Even if you’re working alone, it’s a good practice to establish and follow your own set of coding standards.
7. Refactoring on the Fly
Refactoring is the process of restructuring existing code without changing its external behavior. Even under pressure, it’s important to refactor as you go to keep your code clean and maintainable.
Tips for Quick Refactoring:
- Extract Method: If a piece of code is becoming too long or complex, consider extracting it into a separate method.
- Rename Variables: If you realize a variable name isn’t clear, don’t hesitate to rename it for better clarity.
- Remove Duplication: If you notice you’re writing similar code in multiple places, refactor it into a reusable function.
- Simplify Complex Conditions: If a conditional statement is becoming hard to read, consider breaking it down or extracting it into a well-named function.
Here’s an example of refactoring on the fly:
// Before refactoring
function processPayment(amount, cardNumber, expiryDate, cvv) {
// Validate input
if (amount <= 0) {
throw new Error("Invalid amount");
}
if (cardNumber.length !== 16) {
throw new Error("Invalid card number");
}
if (!expiryDate.match(/^\d{2}\/\d{2}$/)) {
throw new Error("Invalid expiry date");
}
if (cvv.length !== 3) {
throw new Error("Invalid CVV");
}
// Process payment
// ... payment processing logic ...
}
// After refactoring
function processPayment(amount, cardNumber, expiryDate, cvv) {
validatePaymentDetails(amount, cardNumber, expiryDate, cvv);
// Process payment
// ... payment processing logic ...
}
function validatePaymentDetails(amount, cardNumber, expiryDate, cvv) {
validateAmount(amount);
validateCardNumber(cardNumber);
validateExpiryDate(expiryDate);
validateCVV(cvv);
}
function validateAmount(amount) {
if (amount <= 0) {
throw new Error("Invalid amount");
}
}
function validateCardNumber(cardNumber) {
if (cardNumber.length !== 16) {
throw new Error("Invalid card number");
}
}
function validateExpiryDate(expiryDate) {
if (!expiryDate.match(/^\d{2}\/\d{2}$/)) {
throw new Error("Invalid expiry date");
}
}
function validateCVV(cvv) {
if (cvv.length !== 3) {
throw new Error("Invalid CVV");
}
}
This refactoring improves readability by separating concerns and making each function responsible for a single task.
8. Writing Testable Code
Even when under pressure, it’s crucial to write code that can be easily tested. Testable code is often cleaner and more modular by nature.
Principles for Writing Testable Code:
- Single Responsibility: Functions that do one thing are easier to test.
- Dependency Injection: Pass dependencies into functions rather than creating them inside the function.
- Pure Functions: Functions that always produce the same output for a given input are easier to test.
- Avoid Global State: Minimize the use of global variables as they can make testing unpredictable.
- Use Interfaces: In object-oriented programming, coding to interfaces makes it easier to mock dependencies in tests.
Here’s an example of making code more testable:
// Less testable
function getUserData() {
const userId = getCurrentUserId(); // Global function
const response = fetch(`https://api.example.com/users/${userId}`);
return response.json();
}
// More testable
function getUserData(getUserId, fetchData) {
const userId = getUserId();
return fetchData(`https://api.example.com/users/${userId}`);
}
// Usage
const userData = getUserData(
() => getCurrentUserId(),
(url) => fetch(url).then(response => response.json())
);
// Test
function testGetUserData() {
const mockGetUserId = () => "123";
const mockFetchData = (url) => Promise.resolve({ id: "123", name: "John Doe" });
const result = getUserData(mockGetUserId, mockFetchData);
// Assert result...
}
The more testable version allows for easier mocking of dependencies, making it simpler to write unit tests.
9. Leveraging Tools and IDE Features
Modern Integrated Development Environments (IDEs) and coding tools offer numerous features that can help you write cleaner code more efficiently, even under pressure.
Useful IDE Features and Tools:
- Auto-formatting: Many IDEs can automatically format your code to adhere to a specific style guide.
- Linters: Tools like ESLint for JavaScript can catch potential errors and style violations in real-time.
- Code Completion: Autocomplete features can help you write code faster and avoid typos.
- Refactoring Tools: IDEs often have built-in tools for common refactoring operations like renaming variables or extracting methods.
- Version Control Integration: Easy access to version control can help you make frequent, small commits.
- Snippets: Create and use code snippets for common patterns to save time and ensure consistency.
Take the time to familiarize yourself with these tools and customize them to your needs. They can significantly speed up your coding process and help maintain code quality even when you’re working quickly.
10. Practice Makes Perfect
Like any skill, writing clean code under pressure improves with practice. The more you do it, the more it becomes second nature.
Ways to Practice:
- Coding Challenges: Platforms like LeetCode, HackerRank, or AlgoCademy offer timed coding challenges that simulate pressure situations.
- Code Reviews: Regularly review others’ code and have your code reviewed. This helps you develop a critical eye for code quality.
- Refactoring Exercises: Take existing code and practice refactoring it to be cleaner and more efficient.
- Mock Interviews: Practice technical interviews with friends or through online platforms.
- Personal Projects: Work on personal coding projects with self-imposed time constraints.
Remember, the goal is not just to solve the problem, but to solve it with clean, readable code. Make this a habit in all your coding practices.
Conclusion
Writing clean and readable code under pressure is a valuable skill that can set you apart in technical interviews and real-world development scenarios. By focusing on clear naming conventions, effective commenting, code simplicity, and consistent structure, you can produce high-quality code even when time is tight.
Remember that clean code is not about perfection, but about continuous improvement. Every time you write code, try to make it a little cleaner, a little more readable than the last time. With practice and persistence, writing clean code will become second nature, allowing you to produce high-quality work even in high-pressure situations.
As you continue your journey in software development, whether you’re preparing for technical interviews or working on complex projects, always strive to write code that not only works but is also a pleasure for others (and your future self) to read and maintain. Clean code is a mark of a true professional in the field of software development.