How to Write Clean and Maintainable Code Under Pressure: A Comprehensive Guide
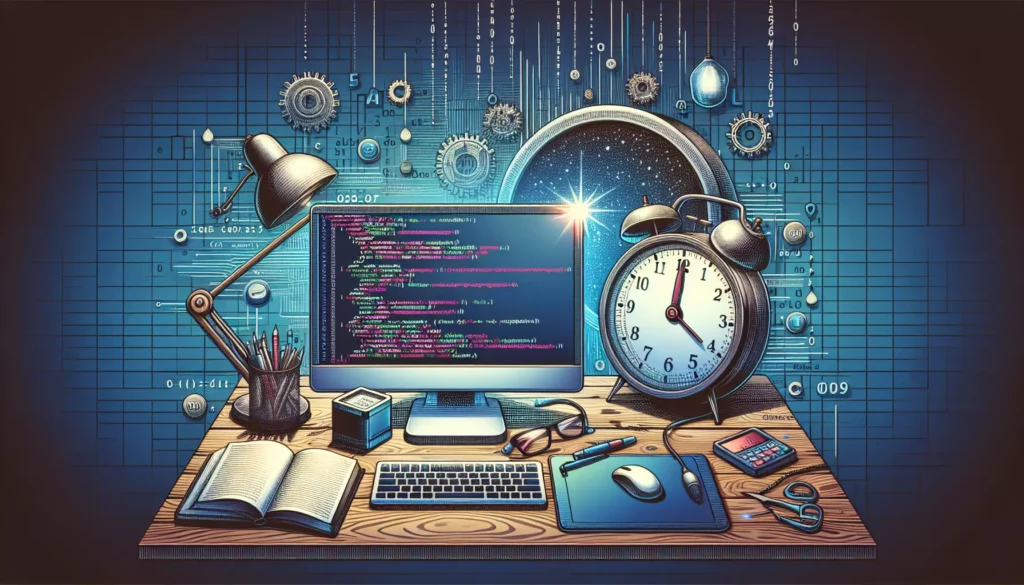
In the fast-paced world of software development, writing clean and maintainable code is a constant challenge. This challenge becomes even more daunting when you’re working under pressure, whether it’s due to tight deadlines, complex project requirements, or high-stakes situations. However, the ability to produce high-quality code even in stressful circumstances is a hallmark of a skilled programmer. In this comprehensive guide, we’ll explore strategies and best practices for writing clean and maintainable code under pressure, helping you become a more effective and efficient developer.
Understanding the Importance of Clean Code
Before we dive into specific techniques, it’s crucial to understand why clean code matters, especially when working under pressure. Clean code is:
- Easier to read and understand
- Simpler to maintain and update
- Less prone to bugs and errors
- More efficient in the long run
- Easier for team collaboration
When you’re under pressure, it might be tempting to cut corners and produce “quick and dirty” code. However, this approach often leads to technical debt, which can slow down development and create more problems in the future. By prioritizing clean code even under pressure, you’re investing in the long-term success of your project.
Strategies for Writing Clean Code Under Pressure
1. Prioritize Planning and Design
Even when time is tight, resist the urge to dive straight into coding. Spend a few minutes planning your approach:
- Outline the main components and their interactions
- Identify potential challenges and edge cases
- Consider the overall architecture and how your code fits into it
This initial investment in planning can save you significant time and headaches later on.
2. Follow Consistent Coding Standards
Adhering to a consistent coding style is crucial for maintaining readability and consistency. If your team has established coding standards, stick to them. If not, consider following widely accepted standards for your programming language. For example, in Python, you might follow the PEP 8 style guide:
# Good: Following PEP 8 naming conventions
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
return total / count if count > 0 else 0
# Bad: Inconsistent naming and spacing
def CalculateAverage( numbers ):
Total = sum(numbers)
Count = len(numbers)
return Total/Count if Count>0 else 0
3. Write Self-Documenting Code
When you’re pressed for time, detailed documentation might seem like a luxury. However, you can make your code more self-explanatory by using descriptive variable and function names, and by structuring your code logically. This approach reduces the need for extensive comments while still maintaining clarity.
# Self-documenting code
def process_user_input(user_input):
sanitized_input = remove_special_characters(user_input)
validated_input = validate_input_length(sanitized_input)
return format_input_for_database(validated_input)
# Less clear code
def process(input):
s = r(input)
v = vl(s)
return f(v)
4. Embrace Modular Design
Breaking your code into smaller, focused functions or modules can make it easier to manage and understand, even when you’re working quickly. Each function should have a single responsibility, making it easier to test, debug, and maintain.
# Modular design
def validate_email(email):
# Email validation logic here
pass
def create_user(username, email):
if not validate_email(email):
raise ValueError("Invalid email address")
# User creation logic here
pass
def send_welcome_email(email):
# Email sending logic here
pass
def register_new_user(username, email):
create_user(username, email)
send_welcome_email(email)
# Less modular approach
def register_new_user(username, email):
# Email validation logic here
# User creation logic here
# Email sending logic here
5. Use Version Control Effectively
Even when working under pressure, make frequent, small commits to your version control system. This practice allows you to:
- Track changes more easily
- Revert to previous versions if needed
- Collaborate more effectively with team members
Write clear, concise commit messages that explain the purpose of each change.
6. Leverage IDE Features and Tools
Modern Integrated Development Environments (IDEs) offer numerous features that can help you write cleaner code more quickly:
- Auto-formatting tools to maintain consistent style
- Code completion and suggestions
- Refactoring tools for easy code restructuring
- Static code analysis to identify potential issues
Familiarize yourself with these features and use them to your advantage when working under pressure.
7. Implement Error Handling
Proper error handling is crucial for creating robust code, even when time is tight. Use try-except blocks (or their equivalent in your language) to catch and handle potential errors gracefully:
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Cannot divide by zero")
result = None
except TypeError:
print("Error: Invalid input types")
result = None
return result
8. Write Tests (Even Simple Ones)
While comprehensive test coverage might not be feasible under tight deadlines, writing even basic tests can help catch obvious errors and provide a safety net for future changes. Focus on testing critical functionality and edge cases:
import unittest
class TestDivideNumbers(unittest.TestCase):
def test_valid_division(self):
self.assertEqual(divide_numbers(10, 2), 5)
def test_division_by_zero(self):
self.assertIsNone(divide_numbers(10, 0))
def test_invalid_input_types(self):
self.assertIsNone(divide_numbers("10", "2"))
if __name__ == '__main__':
unittest.main()
9. Use Meaningful Comments
While self-documenting code is ideal, sometimes complex algorithms or business logic require additional explanation. Use comments to clarify the “why” behind your code, rather than the “what” (which should be evident from the code itself):
def calculate_discount(total_purchase, customer_type):
# Apply progressive discount based on purchase amount and customer type
if customer_type == "premium":
# Premium customers get an additional 5% off
base_discount = 0.1
else:
base_discount = 0.05
# Increase discount for larger purchases
if total_purchase > 1000:
additional_discount = 0.05
elif total_purchase > 500:
additional_discount = 0.02
else:
additional_discount = 0
return base_discount + additional_discount
10. Avoid Premature Optimization
When working under pressure, focus on writing clear, correct code first. Premature optimization can lead to overly complex solutions and introduce bugs. Once your code is working correctly, you can profile it to identify genuine performance bottlenecks and optimize where necessary.
Maintaining Code Quality in High-Pressure Situations
1. Communicate with Your Team
If you’re working as part of a team, clear communication is essential, especially under pressure. Keep your team members informed about your progress, any challenges you’re facing, and any decisions that might affect the overall project. This can help prevent misunderstandings and ensure that everyone is aligned on priorities and approaches.
2. Take Regular Breaks
It might seem counterintuitive when you’re under pressure, but taking short, regular breaks can actually improve your productivity and code quality. Stepping away from your code for even a few minutes can help you return with a fresh perspective, often leading to better problem-solving and cleaner code.
3. Use Code Reviews
Even in high-pressure situations, try to incorporate some form of code review into your process. This could be a formal review by a team member or a quick pair programming session. Another set of eyes can catch issues you might have missed and provide valuable feedback on your code’s clarity and structure.
4. Refactor as You Go
While major refactoring might not be feasible under tight deadlines, small, incremental improvements can still be made. As you work, look for opportunities to improve your code’s structure or readability without introducing significant changes:
# Before refactoring
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
else:
result.append(item * 3)
return result
# After refactoring
def process_data(data):
def transform_item(item):
return item * 2 if item % 2 == 0 else item * 3
return [transform_item(item) for item in data]
5. Prioritize Critical Functionality
When time is limited, focus on implementing and thoroughly testing the most critical parts of your code first. This ensures that the core functionality is solid, even if you don’t have time to perfect every aspect of the system.
6. Document Important Decisions
If you make significant design decisions or compromises due to time constraints, document them briefly. This can be as simple as a comment in the code or a quick note in your project documentation. This information can be invaluable for future maintenance or when revisiting the code later.
7. Use Design Patterns and Best Practices
Familiarity with common design patterns and best practices can help you make better decisions quickly. For example, using the Strategy pattern can make it easier to swap out algorithms or behaviors without major code changes:
from abc import ABC, abstractmethod
class SortStrategy(ABC):
@abstractmethod
def sort(self, data):
pass
class QuickSort(SortStrategy):
def sort(self, data):
# QuickSort implementation
pass
class MergeSort(SortStrategy):
def sort(self, data):
# MergeSort implementation
pass
class Sorter:
def __init__(self, strategy: SortStrategy):
self.strategy = strategy
def perform_sort(self, data):
return self.strategy.sort(data)
# Usage
sorter = Sorter(QuickSort())
sorted_data = sorter.perform_sort(data)
8. Leverage Libraries and Frameworks
Don’t reinvent the wheel, especially when time is tight. Use well-established libraries and frameworks for common tasks. This not only saves time but often results in more reliable and efficient code. Just be sure to choose libraries that are well-maintained and compatible with your project.
9. Keep Your Development Environment Organized
A clean and organized development environment can significantly improve your efficiency when working under pressure. This includes:
- Keeping your project files well-structured
- Using meaningful file and directory names
- Maintaining a clear separation of concerns in your codebase
- Setting up your IDE with useful shortcuts and configurations
10. Learn from the Experience
After the pressure has subsided, take some time to reflect on the experience. Identify what worked well and what could be improved in future high-pressure situations. This continuous learning process will help you become more adept at writing clean code under any circumstances.
Conclusion
Writing clean and maintainable code under pressure is a skill that improves with practice and experience. By following the strategies and best practices outlined in this guide, you can significantly enhance your ability to produce high-quality code even in challenging circumstances. Remember that clean code is an investment in the future of your project and your growth as a developer.
As you continue to develop your skills, consider leveraging resources like AlgoCademy to further enhance your coding abilities. AlgoCademy offers interactive coding tutorials, problem-solving exercises, and AI-powered assistance that can help you refine your algorithmic thinking and coding practices. By combining these learning resources with real-world experience, you’ll be well-equipped to handle any coding challenge, no matter the pressure.
Remember, the goal is not perfection, but continuous improvement. Each time you face a high-pressure coding situation, you’ll have the opportunity to apply these principles and refine your approach. With time, writing clean and maintainable code will become second nature, allowing you to produce high-quality work consistently, regardless of the circumstances.