How to Work with Strings: Manipulation and Formatting
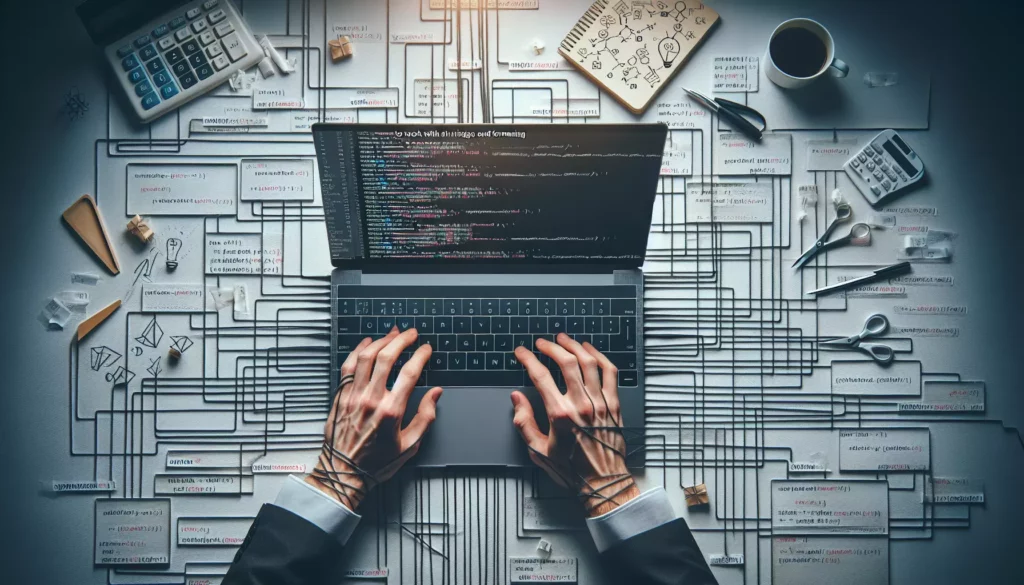
Strings are one of the most fundamental and widely used data types in programming. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mastering string manipulation and formatting is crucial. In this comprehensive guide, we’ll explore various techniques and best practices for working with strings, providing you with the skills you need to excel in your coding endeavors.
Understanding Strings
Before we dive into manipulation and formatting, let’s briefly review what strings are and how they’re represented in most programming languages.
A string is a sequence of characters, typically used to represent text. In most programming languages, strings are enclosed in either single quotes (”) or double quotes (“”). For example:
my_string = "Hello, World!"
another_string = 'AlgoCademy is awesome!'
Strings are immutable in many languages, meaning that once a string is created, it cannot be changed. However, we can create new strings based on existing ones through various manipulation techniques.
String Manipulation Techniques
1. Concatenation
Concatenation is the process of combining two or more strings to create a new string. Most languages use the ‘+’ operator for concatenation:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(full_name) # Output: John Doe
Some languages, like Python, also support string interpolation or f-strings for more readable concatenation:
age = 30
message = f"{first_name} {last_name} is {age} years old."
print(message) # Output: John Doe is 30 years old.
2. Slicing
Slicing allows you to extract a portion of a string based on index positions. The general syntax is string[start:end:step]
, where start
is inclusive and end
is exclusive:
my_string = "AlgoCademy"
print(my_string[0:4]) # Output: Algo
print(my_string[4:]) # Output: Cademy
print(my_string[::-1]) # Output: ymedaCoglA (reverses the string)
3. String Methods
Most programming languages provide built-in methods for string manipulation. Here are some common ones:
upper()
andlower()
: Convert a string to uppercase or lowercasestrip()
: Remove leading and trailing whitespacereplace()
: Replace occurrences of a substringsplit()
: Split a string into a list of substringsjoin()
: Join a list of strings into a single string
Example usage:
text = " Hello, World! "
print(text.upper()) # Output: " HELLO, WORLD! "
print(text.strip()) # Output: "Hello, World!"
print(text.replace(",", "")) # Output: " Hello World! "
words = "apple,banana,cherry"
fruit_list = words.split(",")
print(fruit_list) # Output: ['apple', 'banana', 'cherry']
joined = "-".join(fruit_list)
print(joined) # Output: "apple-banana-cherry"
4. String Formatting
String formatting allows you to create formatted strings with placeholders for variables. Different languages have various methods for string formatting:
Python:
# Using .format()
name = "Alice"
age = 25
print("My name is {} and I'm {} years old.".format(name, age))
# Using f-strings (Python 3.6+)
print(f"My name is {name} and I'm {age} years old.")
# Using % operator (older style)
print("My name is %s and I'm %d years old." % (name, age))
JavaScript:
// Using template literals
const name = "Bob";
const age = 30;
console.log(`My name is ${name} and I'm ${age} years old.`);
// Using concatenation
console.log("My name is " + name + " and I'm " + age + " years old.");
Advanced String Manipulation Techniques
1. Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. They allow you to search, extract, and replace text based on complex patterns. Here’s a simple example in Python:
import re
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\b\w{5}\b" # Matches 5-letter words
matches = re.findall(pattern, text)
print(matches) # Output: ['quick', 'brown', 'jumps']
Regular expressions can be complex but are incredibly useful for tasks like data validation, parsing, and text processing.
2. String Algorithms
Understanding and implementing string algorithms is crucial for technical interviews and efficient code. Some important string algorithms include:
- String searching (e.g., KMP algorithm, Boyer-Moore algorithm)
- String matching (e.g., Rabin-Karp algorithm)
- Longest common subsequence
- Palindrome checking
Here’s an example of a simple palindrome checking function:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
3. Unicode and Encoding
When working with strings, it’s important to understand Unicode and character encoding, especially when dealing with international text or special characters. Most modern programming languages use Unicode by default, but you may encounter situations where you need to handle different encodings.
In Python, you can work with different encodings using the encode()
and decode()
methods:
text = "Hello, 世界"
utf8_bytes = text.encode('utf-8')
print(utf8_bytes) # Output: b'Hello, \xe4\xb8\x96\xe7\x95\x8c'
decoded_text = utf8_bytes.decode('utf-8')
print(decoded_text) # Output: Hello, 世界
Best Practices for Working with Strings
As you develop your string manipulation skills, keep these best practices in mind:
- Use built-in methods: Leverage the built-in string methods provided by your programming language. They are often optimized for performance and readability.
- Be mindful of immutability: Remember that strings are immutable in many languages. Instead of modifying strings in place, create new strings with the desired changes.
- Consider performance: For large-scale string operations, be aware of the performance implications. For example, using
join()
to concatenate a list of strings is generally more efficient than using the ‘+’ operator in a loop. - Handle edge cases: When working with user input or external data, always consider edge cases like empty strings, null values, or unexpected characters.
- Use appropriate string formatting: Choose the most readable and maintainable string formatting method for your use case. For example, f-strings in Python are often more readable than older formatting methods.
- Validate input: When working with user-provided strings, validate and sanitize the input to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS).
- Consider internationalization: If your application might be used internationally, use Unicode and be prepared to handle different character encodings.
Practical Examples and Coding Challenges
To solidify your understanding of string manipulation and formatting, try solving these coding challenges:
1. Reverse Words in a String
Write a function that reverses the order of words in a given string. For example, “Hello World” should become “World Hello”.
def reverse_words(s):
# Split the string into words
words = s.split()
# Reverse the list of words and join them back into a string
return ' '.join(words[::-1])
print(reverse_words("Hello World")) # Output: "World Hello"
print(reverse_words("The quick brown fox")) # Output: "fox brown quick The"
2. String Compression
Implement a method to perform basic string compression using the counts of repeated characters. For example, the string “aabcccccaaa” would become “a2b1c5a3”. If the “compressed” string would not become smaller than the original string, your method should return the original string.
def compress_string(s):
if not s:
return s
compressed = []
count = 1
current_char = s[0]
for char in s[1:]:
if char == current_char:
count += 1
else:
compressed.append(current_char + str(count))
current_char = char
count = 1
compressed.append(current_char + str(count))
compressed_str = ''.join(compressed)
return compressed_str if len(compressed_str) < len(s) else s
print(compress_string("aabcccccaaa")) # Output: "a2b1c5a3"
print(compress_string("abcdef")) # Output: "abcdef" (original string)
3. Longest Palindromic Substring
Write a function to find the longest palindromic substring in a given string. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
def longest_palindrome(s):
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
length1 = expand_around_center(i, i)
length2 = expand_around_center(i, i + 1)
length = max(length1, length2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
print(longest_palindrome("babad")) # Output: "bab" or "aba"
print(longest_palindrome("cbbd")) # Output: "bb"
Conclusion
Mastering string manipulation and formatting is essential for any programmer, from beginners to those preparing for technical interviews at top tech companies. By understanding the fundamentals of strings, learning various manipulation techniques, and practicing with real-world problems, you’ll develop the skills needed to handle complex string operations efficiently.
Remember that working with strings often involves trade-offs between readability, performance, and maintainability. As you continue to develop your skills, focus on writing clean, efficient code that solves the problem at hand while considering potential edge cases and scalability issues.
Keep practicing with different string manipulation challenges, and don’t hesitate to explore more advanced topics like regular expressions and complex string algorithms. With dedication and consistent practice, you’ll be well-prepared to tackle any string-related problem in your coding journey or during technical interviews.
Happy coding, and may your strings always be perfectly manipulated and beautifully formatted!