How to Work with JSON and XML Data Formats: A Comprehensive Guide
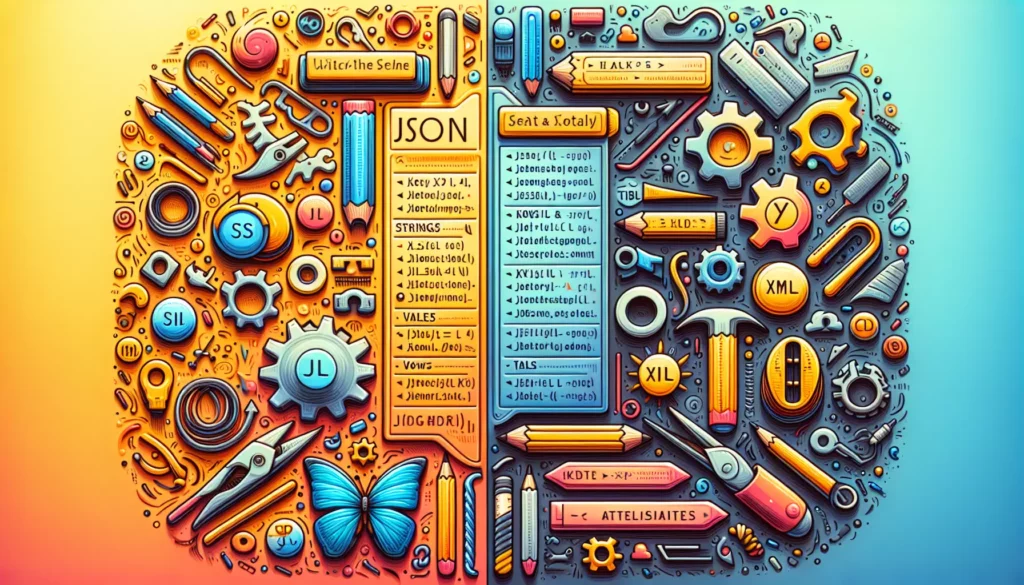
In today’s data-driven world, understanding how to work with different data formats is crucial for any programmer. Two of the most common data formats you’ll encounter are JSON (JavaScript Object Notation) and XML (eXtensible Markup Language). These formats are widely used for storing and exchanging data between systems, making them essential tools in a developer’s toolkit. In this comprehensive guide, we’ll explore how to work with JSON and XML data formats, providing you with the knowledge and skills to handle these formats effectively in your coding projects.
Table of Contents
- Introduction to JSON and XML
- JSON Basics
- XML Basics
- Working with JSON
- Working with XML
- Comparing JSON and XML
- Best Practices for JSON and XML
- Real-World Examples
- Conclusion
1. Introduction to JSON and XML
Before diving into the specifics of working with JSON and XML, let’s briefly introduce these two data formats and understand their significance in modern programming.
JSON (JavaScript Object Notation)
JSON is a lightweight, text-based data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript programming language but is language-independent, making it a popular choice for data exchange in various programming environments.
XML (eXtensible Markup Language)
XML is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable. It is designed to store and transport data, and it can be used to represent any kind of structured information.
2. JSON Basics
Let’s start by exploring the fundamental concepts of JSON.
JSON Structure
JSON data is built on two structures:
- A collection of name/value pairs (similar to an object in many programming languages)
- An ordered list of values (similar to an array in many programming languages)
JSON Data Types
JSON supports the following data types:
- String: A sequence of characters enclosed in double quotes
- Number: Integer or floating-point
- Boolean: true or false
- Array: An ordered list of values enclosed in square brackets
- Object: An unordered collection of name/value pairs enclosed in curly braces
- null: Represents a null value
JSON Example
Here’s a simple example of JSON data:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"isStudent": false,
"hobbies": ["reading", "swimming", "coding"],
"address": {
"street": "123 Main St",
"zipCode": "10001"
}
}
3. XML Basics
Now, let’s explore the fundamental concepts of XML.
XML Structure
XML documents are structured as a tree of elements, each element potentially containing attributes, other elements, or text content.
XML Components
- Elements: The basic building blocks of XML, defined by tags
- Attributes: Additional information about elements, included within the opening tag
- Comments: Used to include notes or explanations within the XML document
- Processing Instructions: Used to provide instructions to applications processing the XML
- CDATA Sections: Used to include text that contains characters that would otherwise be treated as markup
XML Example
Here’s a simple example of XML data:
<?xml version="1.0" encoding="UTF-8"?>
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
<isStudent>false</isStudent>
<hobbies>
<hobby>reading</hobby>
<hobby>swimming</hobby>
<hobby>coding</hobby>
</hobbies>
<address>
<street>123 Main St</street>
<zipCode>10001</zipCode>
</address>
</person>
4. Working with JSON
Now that we understand the basics of JSON, let’s explore how to work with it in various programming languages.
Parsing JSON
Parsing JSON involves converting a JSON string into a data structure that can be easily manipulated in your programming language of choice. Here are examples in different languages:
Python
import json
json_string = '{"name": "John Doe", "age": 30, "city": "New York"}'
parsed_data = json.loads(json_string)
print(parsed_data['name']) # Output: John Doe
JavaScript
const jsonString = '{"name": "John Doe", "age": 30, "city": "New York"}';
const parsedData = JSON.parse(jsonString);
console.log(parsedData.name); // Output: John Doe
Java
import org.json.JSONObject;
String jsonString = "{\"name\": \"John Doe\", \"age\": 30, \"city\": \"New York\"}";
JSONObject parsedData = new JSONObject(jsonString);
System.out.println(parsedData.getString("name")); // Output: John Doe
Creating JSON
Creating JSON involves converting data structures in your programming language into a JSON string. Here are examples:
Python
import json
data = {
"name": "Jane Smith",
"age": 28,
"city": "London"
}
json_string = json.dumps(data)
print(json_string) # Output: {"name": "Jane Smith", "age": 28, "city": "London"}
JavaScript
const data = {
name: "Jane Smith",
age: 28,
city: "London"
};
const jsonString = JSON.stringify(data);
console.log(jsonString); // Output: {"name":"Jane Smith","age":28,"city":"London"}
Java
import org.json.JSONObject;
JSONObject data = new JSONObject();
data.put("name", "Jane Smith");
data.put("age", 28);
data.put("city", "London");
String jsonString = data.toString();
System.out.println(jsonString); // Output: {"name":"Jane Smith","age":28,"city":"London"}
Manipulating JSON Data
Once you’ve parsed JSON data, you can easily manipulate it using your programming language’s native data structures. Here’s an example in Python:
import json
# Parse JSON string
json_string = '{"name": "John Doe", "age": 30, "hobbies": ["reading", "swimming"]}'
data = json.loads(json_string)
# Modify data
data['age'] = 31
data['hobbies'].append('coding')
# Convert back to JSON string
updated_json = json.dumps(data, indent=2)
print(updated_json)
This will output:
{
"name": "John Doe",
"age": 31,
"hobbies": [
"reading",
"swimming",
"coding"
]
}
5. Working with XML
Now let’s explore how to work with XML in various programming languages.
Parsing XML
Parsing XML involves converting an XML string into a data structure that can be easily manipulated in your programming language. Here are examples in different languages:
Python
import xml.etree.ElementTree as ET
xml_string = '''
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
</person>
'''
root = ET.fromstring(xml_string)
print(root.find('name').text) # Output: John Doe
JavaScript (using DOMParser)
const xmlString = `
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
</person>
`;
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlString, "text/xml");
console.log(xmlDoc.getElementsByTagName("name")[0].textContent); // Output: John Doe
Java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import java.io.ByteArrayInputStream;
String xmlString = "<person><name>John Doe</name><age>30</age><city>New York</city></person>";
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new ByteArrayInputStream(xmlString.getBytes()));
Element root = doc.getDocumentElement();
System.out.println(root.getElementsByTagName("name").item(0).getTextContent()); // Output: John Doe
Creating XML
Creating XML involves constructing an XML document programmatically. Here are examples:
Python
import xml.etree.ElementTree as ET
root = ET.Element("person")
ET.SubElement(root, "name").text = "Jane Smith"
ET.SubElement(root, "age").text = "28"
ET.SubElement(root, "city").text = "London"
xml_string = ET.tostring(root, encoding='unicode')
print(xml_string)
JavaScript
const xmlDoc = document.implementation.createDocument(null, "person");
const root = xmlDoc.documentElement;
const nameElement = xmlDoc.createElement("name");
nameElement.textContent = "Jane Smith";
root.appendChild(nameElement);
const ageElement = xmlDoc.createElement("age");
ageElement.textContent = "28";
root.appendChild(ageElement);
const cityElement = xmlDoc.createElement("city");
cityElement.textContent = "London";
root.appendChild(cityElement);
const serializer = new XMLSerializer();
const xmlString = serializer.serializeToString(xmlDoc);
console.log(xmlString);
Java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import java.io.StringWriter;
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.newDocument();
Element root = doc.createElement("person");
doc.appendChild(root);
Element name = doc.createElement("name");
name.appendChild(doc.createTextNode("Jane Smith"));
root.appendChild(name);
Element age = doc.createElement("age");
age.appendChild(doc.createTextNode("28"));
root.appendChild(age);
Element city = doc.createElement("city");
city.appendChild(doc.createTextNode("London"));
root.appendChild(city);
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
StringWriter writer = new StringWriter();
transformer.transform(new DOMSource(doc), new StreamResult(writer));
String xmlString = writer.getBuffer().toString();
System.out.println(xmlString);
Manipulating XML Data
Once you’ve parsed XML data, you can manipulate it using your programming language’s XML libraries. Here’s an example in Python:
import xml.etree.ElementTree as ET
# Parse XML string
xml_string = '''
<person>
<name>John Doe</name>
<age>30</age>
<hobbies>
<hobby>reading</hobby>
<hobby>swimming</hobby>
</hobbies>
</person>
'''
root = ET.fromstring(xml_string)
# Modify data
age_elem = root.find('age')
age_elem.text = '31'
hobbies_elem = root.find('hobbies')
new_hobby = ET.SubElement(hobbies_elem, 'hobby')
new_hobby.text = 'coding'
# Convert back to XML string
updated_xml = ET.tostring(root, encoding='unicode')
print(updated_xml)
This will output:
<person>
<name>John Doe</name>
<age>31</age>
<hobbies>
<hobby>reading</hobby>
<hobby>swimming</hobby>
<hobby>coding</hobby>
</hobbies>
</person>
6. Comparing JSON and XML
While both JSON and XML are used for data interchange, they have some key differences:
Advantages of JSON
- Lightweight and less verbose
- Easier to read and write for humans
- Faster to parse and generate
- Native support in JavaScript
- Better support for arrays
Advantages of XML
- More expressive and can represent complex data structures
- Supports metadata through attributes
- Has schema languages for validation (e.g., XSD)
- Better support for mixed content (text and elements)
- Widely used in enterprise systems and SOAP web services
When to Use JSON vs XML
Choose JSON when:
- You need a lightweight data format
- You’re working primarily with JavaScript or web applications
- You want faster parsing and generation
- Your data structure is relatively simple
Choose XML when:
- You need to represent complex data structures
- You require metadata or attributes for your data
- You need strong validation through schemas
- You’re working with systems that require XML (e.g., SOAP web services)
7. Best Practices for JSON and XML
When working with JSON and XML, consider the following best practices:
JSON Best Practices
- Use consistent naming conventions (e.g., camelCase or snake_case)
- Keep your JSON structure as flat as possible
- Use arrays for lists of similar items
- Validate JSON data before parsing to prevent errors
- Use appropriate data types (e.g., boolean instead of string for true/false values)
XML Best Practices
- Use meaningful and descriptive element names
- Use attributes for metadata, not for data that changes frequently
- Keep your XML structure logical and hierarchical
- Use namespaces to avoid conflicts in large XML documents
- Validate XML against a schema (XSD) when possible
General Best Practices
- Always handle potential errors when parsing or generating data
- Use appropriate character encoding (UTF-8 is recommended)
- Implement proper security measures to prevent injection attacks
- Optimize for performance when working with large datasets
- Document your data structures and any custom formats
8. Real-World Examples
Let’s look at some real-world examples of how JSON and XML are used in various applications:
JSON Examples
API Responses
Many web APIs return data in JSON format. Here’s an example of a response from a weather API:
{
"location": {
"name": "New York",
"country": "United States"
},
"current": {
"temp_c": 22,
"temp_f": 71.6,
"condition": {
"text": "Partly cloudy",
"icon": "//cdn.weatherapi.com/weather/64x64/day/116.png"
}
}
}
Configuration Files
JSON is often used for configuration files, such as package.json in Node.js projects:
{
"name": "my-project",
"version": "1.0.0",
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
},
"scripts": {
"start": "node index.js",
"test": "jest"
}
}
XML Examples
RSS Feeds
RSS feeds, used for syndicating web content, are typically in XML format:
<?xml version="1.0" encoding="UTF-8" ?>
<rss version="2.0">
<channel>
<title>My Blog</title>
<link>https://www.myblog.com</link>
<description>Latest posts from My Blog</description>
<item>
<title>New Post Title</title>
<link>https://www.myblog.com/new-post</link>
<description>This is a summary of the new post.</description>
<pubDate>Mon, 06 Sep 2021 12:00:00 GMT</pubDate>
</item>
</channel>
</rss>
SOAP Web Services
SOAP (Simple Object Access Protocol) web services use XML for message exchange:
<?xml version="1.0"?>
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope">
<soap:Header>
</soap:Header>
<soap:Body>
<m:GetStockPrice xmlns:m="http://www.example.org/stock">
<m:StockName>IBM</m:StockName>
</m:GetStockPrice>
</soap:Body>
</soap:Envelope>
9. Conclusion
Understanding how to work with JSON and XML data formats is crucial for any programmer in today’s data-driven world. Both formats have their strengths and are used extensively in various applications, from web development to enterprise systems.
JSON’s simplicity and lightweight nature make it ideal for web applications and APIs, while XML’s expressiveness and strong validation capabilities make it suitable for complex data structures and enterprise systems.
By mastering these formats, you’ll be better equipped to handle data interchange, configuration, and integration tasks in your programming projects. Remember to follow best practices, choose the right format for your specific use case, and always prioritize data security and efficiency in your implementations.
As you continue your journey in programming and data handling, keep exploring new tools and libraries that can help you work more effectively with JSON and XML. Stay updated with the latest developments in data formats and exchange protocols to ensure you’re always using the most efficient and appropriate solutions for your projects.