How to Work with Graphic Libraries for Simple Visualizations
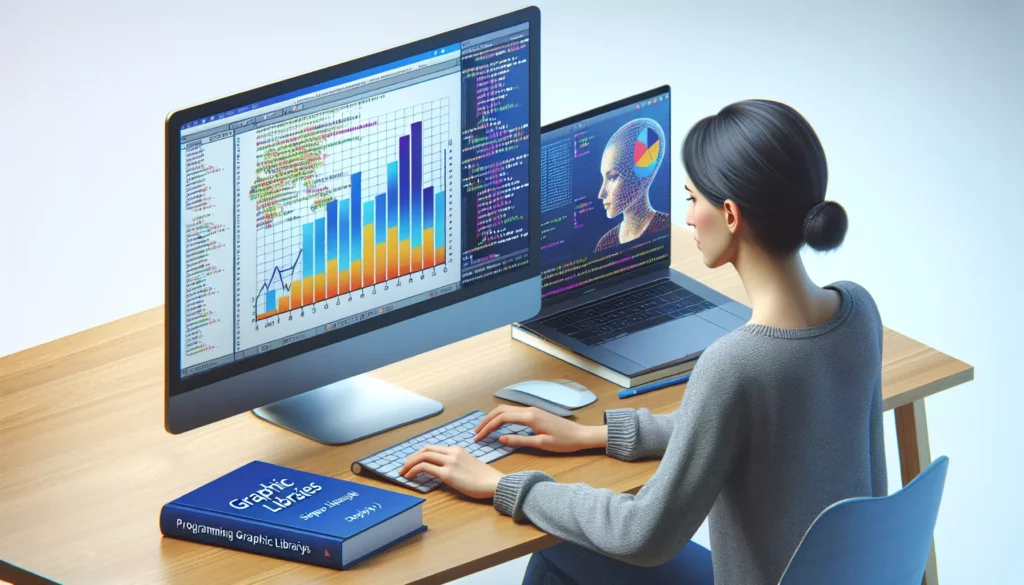
In the world of programming and data analysis, the ability to create visual representations of data is invaluable. Graphic libraries provide powerful tools for developers to transform raw data into meaningful, easy-to-understand visualizations. This skill is particularly crucial for aspiring programmers and those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll explore how to work with graphic libraries to create simple yet effective visualizations, a key component in the toolkit of any proficient coder.
Understanding Graphic Libraries
Graphic libraries are collections of pre-written code that simplify the process of creating visual elements in programming. They abstract away many of the complex calculations and rendering processes, allowing developers to focus on the data and design aspects of their visualizations. Some popular graphic libraries include:
- Matplotlib (Python)
- D3.js (JavaScript)
- ggplot2 (R)
- Chart.js (JavaScript)
- Plotly (Python, R, JavaScript)
Each library has its strengths and is suited for different types of visualizations and programming languages. In this article, we’ll primarily focus on Matplotlib for Python, as it’s widely used and versatile for beginners and advanced users alike.
Getting Started with Matplotlib
Matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. To begin using Matplotlib, you’ll need to install it first. You can do this using pip, Python’s package installer:
pip install matplotlib
Once installed, you can import Matplotlib in your Python script:
import matplotlib.pyplot as plt
This imports the pyplot module and aliases it as ‘plt’, which is a common convention in the Python community.
Creating Your First Plot
Let’s start with a simple line plot. This is often used to show trends over time or relationships between two variables. Here’s a basic example:
import matplotlib.pyplot as plt
import numpy as np
# Generate some data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create the plot
plt.plot(x, y)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
# Display the plot
plt.show()
This code creates a simple sine wave plot. Let’s break down what each part does:
- We import numpy (as np) to generate some sample data.
- We create two arrays: x (a range of values from 0 to 10) and y (the sine of x).
- plt.plot(x, y) creates the actual line plot.
- We add labels to the x and y axes and a title to the plot.
- Finally, plt.show() displays the plot.
Customizing Your Plots
Matplotlib offers extensive customization options. You can change colors, line styles, markers, and more. Here’s an example of a more customized plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
plt.plot(x, y1, color='blue', linestyle='--', label='Sine')
plt.plot(x, y2, color='red', linestyle='-.', label='Cosine')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sine and Cosine Waves')
plt.legend()
plt.grid(True)
plt.show()
In this example, we’ve added several customizations:
- We’re plotting two lines (sine and cosine) on the same graph.
- Each line has a different color and line style.
- We’ve added a legend to distinguish between the lines.
- We’ve added a grid to make it easier to read values off the plot.
Different Types of Plots
Matplotlib supports a wide variety of plot types. Let’s explore a few more common ones:
Scatter Plot
Scatter plots are useful for showing the relationship between two variables. Here’s how to create a simple scatter plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.random.rand(50)
y = np.random.rand(50)
plt.scatter(x, y, color='green', alpha=0.5)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot')
plt.show()
In this example, we’re using random data for both x and y. The alpha parameter sets the transparency of the points.
Bar Plot
Bar plots are great for comparing quantities across different categories. Here’s a simple bar plot:
import matplotlib.pyplot as plt
categories = ['A', 'B', 'C', 'D']
values = [3, 7, 2, 5]
plt.bar(categories, values)
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')
plt.show()
Histogram
Histograms are used to show the distribution of a dataset. Here’s how to create a histogram:
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(1000)
plt.hist(data, bins=30)
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
plt.show()
In this example, we’re using normally distributed random data. The ‘bins’ parameter determines how many bars the histogram will have.
Subplots: Combining Multiple Plots
Often, you’ll want to display multiple plots side by side for comparison. Matplotlib makes this easy with subplots:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 4))
ax1.plot(x, np.sin(x))
ax1.set_title('Sine Wave')
ax2.plot(x, np.cos(x))
ax2.set_title('Cosine Wave')
plt.tight_layout()
plt.show()
This code creates two side-by-side plots. The figsize parameter sets the overall size of the figure.
Saving Your Plots
Once you’ve created your visualization, you might want to save it for later use or to include in a report. Matplotlib makes this straightforward:
plt.savefig('my_plot.png', dpi=300, bbox_inches='tight')
This line should be added before plt.show(). The ‘dpi’ parameter sets the resolution, and ‘bbox_inches=’tight” ensures that the entire plot fits within the saved image.
Interactive Plotting with Matplotlib
While Matplotlib is primarily used for static plots, it also supports some level of interactivity. Here’s a simple example of how to create an interactive plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots()
line, = ax.plot(x, y)
def update(event):
if event.key == 'up':
line.set_ydata(np.sin(x) * 1.1)
elif event.key == 'down':
line.set_ydata(np.sin(x) * 0.9)
fig.canvas.draw()
fig.canvas.mpl_connect('key_press_event', update)
plt.show()
In this example, pressing the up arrow key will increase the amplitude of the sine wave, while pressing the down arrow key will decrease it.
Working with Real Data
While these examples use generated data, in real-world scenarios, you’ll often be working with data from external sources. Let’s look at how you might visualize some real data using Matplotlib:
import matplotlib.pyplot as plt
import pandas as pd
# Load data from a CSV file
data = pd.read_csv('sample_data.csv')
plt.figure(figsize=(10, 6))
plt.plot(data['Date'], data['Value'], marker='o')
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('Data Trend Over Time')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
This example assumes you have a CSV file named ‘sample_data.csv’ with ‘Date’ and ‘Value’ columns. It creates a line plot of the data over time, with markers for each data point and rotated x-axis labels for better readability.
Advanced Techniques
As you become more comfortable with Matplotlib, you can explore more advanced techniques:
3D Plotting
Matplotlib supports 3D plotting, which can be useful for visualizing multidimensional data:
import matplotlib.pyplot as plt
import numpy as np
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = np.random.standard_normal(100)
y = np.random.standard_normal(100)
z = np.random.standard_normal(100)
ax.scatter(x, y, z)
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
plt.show()
Animations
You can create animated plots using Matplotlib’s animation module:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def animate(i):
line.set_ydata(np.sin(x + i/10))
return line,
ani = animation.FuncAnimation(fig, animate, np.arange(1, 200), interval=25, blit=True)
plt.show()
This creates an animation of a moving sine wave.
Best Practices for Data Visualization
As you work with graphic libraries, keep these best practices in mind:
- Choose the right type of plot for your data and what you want to convey.
- Keep it simple – don’t overcrowd your visualizations with unnecessary information.
- Use color effectively – color can highlight important aspects of your data, but too much can be distracting.
- Label everything clearly – your axes, legend, and title should clearly explain what the visualization is showing.
- Consider your audience – tailor your visualization to who will be viewing it and what they need to understand from it.
- Be consistent – if you’re creating multiple plots, use consistent styles and colors for easier comparison.
- Tell a story – your visualization should help convey a clear message or insight about your data.
Conclusion
Working with graphic libraries like Matplotlib is an essential skill for any programmer, especially those preparing for technical interviews or looking to enter the data science field. The ability to create clear, informative visualizations can set you apart in coding challenges and real-world projects alike.
As you continue to practice and explore, you’ll find that mastering these tools opens up new ways of understanding and presenting data. Remember, the key to becoming proficient is practice and experimentation. Try recreating plots you see in articles or reports, work with different types of data, and don’t be afraid to dive into the documentation to discover new features and techniques.
Visualization is not just about making pretty pictures – it’s about turning data into insights, and insights into action. As you develop your skills in working with graphic libraries, you’re building a powerful tool for communication and analysis that will serve you well throughout your programming career.