How to Work with APIs: Making and Handling Requests
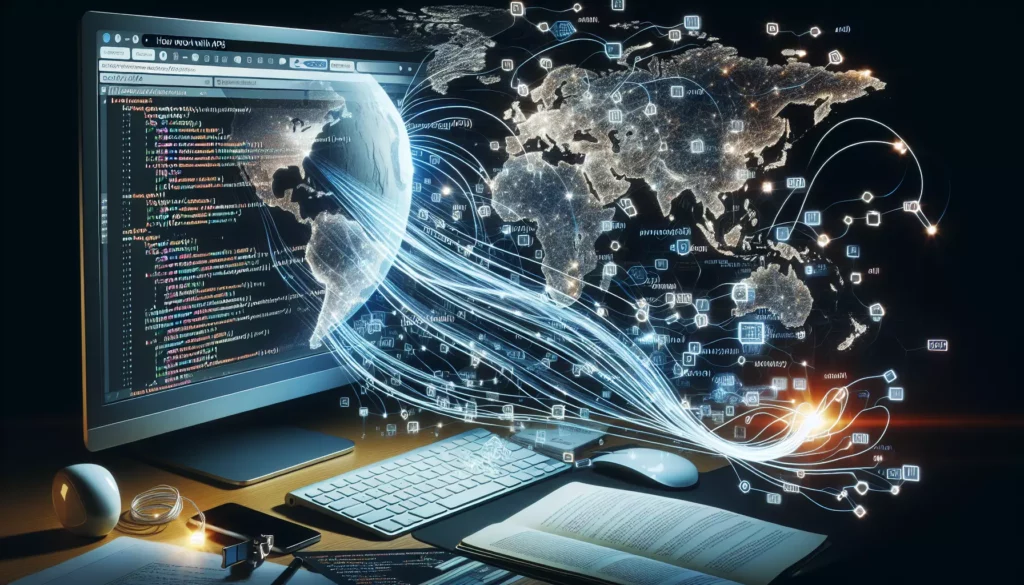
In today’s interconnected digital world, Application Programming Interfaces (APIs) play a crucial role in allowing different software systems to communicate and share data. Whether you’re building a web application, mobile app, or any other software project, understanding how to work with APIs is an essential skill for any developer. In this comprehensive guide, we’ll explore the ins and outs of making and handling API requests, providing you with the knowledge and tools you need to effectively integrate APIs into your projects.
Table of Contents
- Understanding APIs
- Types of API Requests
- Making API Requests
- Handling API Responses
- Error Handling and Debugging
- API Authentication and Security
- Best Practices for Working with APIs
- Tools and Libraries for API Development
- Conclusion
Understanding APIs
Before diving into the technical details of working with APIs, it’s essential to understand what they are and why they’re important. An API is a set of protocols, routines, and tools that specify how software components should interact. It acts as a bridge between different applications, allowing them to communicate and share data seamlessly.
APIs can be thought of as a contract between two applications, defining the types of requests and data that can be sent and received. They abstract the underlying complexity of a system, providing a simpler interface for developers to work with.
Key Benefits of APIs:
- Facilitating integration between different systems
- Enabling the creation of more powerful and feature-rich applications
- Improving efficiency by allowing developers to leverage existing services
- Promoting standardization and consistency in software development
Types of API Requests
When working with APIs, you’ll encounter different types of requests, each serving a specific purpose. The most common types of API requests are:
1. GET
Used to retrieve data from a specified resource. GET requests should only retrieve data and not modify it.
2. POST
Used to submit data to be processed to a specified resource. Often used when creating new records or sending data that needs to be added to a database.
3. PUT
Used to update existing data at a specified resource. PUT requests typically replace the entire resource with the new data provided.
4. PATCH
Similar to PUT, but used for partial updates to a resource. PATCH requests modify only specific fields of the existing data.
5. DELETE
Used to delete a specified resource.
6. HEAD
Similar to GET, but retrieves only the headers of the response, not the body.
7. OPTIONS
Used to describe the communication options for the target resource.
Understanding these different request types is crucial for effectively interacting with APIs and designing your own API endpoints.
Making API Requests
Now that we understand the types of API requests, let’s explore how to actually make these requests in various programming languages.
Using JavaScript (with Fetch API)
The Fetch API provides a powerful and flexible way to make HTTP requests in JavaScript. Here’s an example of a GET request:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
For a POST request, you might do something like this:
fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
key1: 'value1',
key2: 'value2'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Using Python (with requests library)
In Python, the requests library is a popular choice for making HTTP requests. Here’s how you might make a GET request:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Error:', response.status_code)
And here’s an example of a POST request:
import requests
payload = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post('https://api.example.com/data', json=payload)
if response.status_code == 201:
data = response.json()
print(data)
else:
print('Error:', response.status_code)
Using Java (with HttpClient)
In Java 11 and later, you can use the HttpClient class to make HTTP requests. Here’s an example of a GET request:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
And here’s how you might make a POST request:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
String jsonBody = "{\"key1\":\"value1\",\"key2\":\"value2\"}";
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.header("Content-Type", "application/json")
.POST(HttpRequest.BodyPublishers.ofString(jsonBody))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
Handling API Responses
Once you’ve made an API request, you need to know how to handle the response. API responses typically come in a structured format, most commonly JSON (JavaScript Object Notation) or XML (eXtensible Markup Language).
Parsing JSON Responses
JSON is the most widely used format for API responses due to its simplicity and ease of use. Here’s how you might parse a JSON response in different languages:
JavaScript
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data.key1);
console.log(data.key2);
})
.catch(error => console.error('Error:', error));
Python
import requests
import json
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
print(data['key1'])
print(data['key2'])
else:
print('Error:', response.status_code)
Java
import com.fasterxml.jackson.databind.ObjectMapper;
// Assuming you've already made the request and got the response as a String
String jsonResponse = response.body();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonResponse);
System.out.println(jsonNode.get("key1").asText());
System.out.println(jsonNode.get("key2").asText());
Handling Different Status Codes
API responses come with HTTP status codes that indicate the result of the request. It’s important to handle these appropriately:
- 2xx (Success): The request was successfully received, understood, and accepted.
- 3xx (Redirection): Further action needs to be taken to complete the request.
- 4xx (Client Error): The request contains bad syntax or cannot be fulfilled.
- 5xx (Server Error): The server failed to fulfill a valid request.
Here’s an example of how you might handle different status codes:
fetch('https://api.example.com/data')
.then(response => {
if (response.status === 200) {
return response.json();
} else if (response.status === 404) {
throw new Error('Data not found');
} else if (response.status === 500) {
throw new Error('Server error');
} else {
throw new Error('Unexpected error');
}
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Error Handling and Debugging
When working with APIs, it’s crucial to implement robust error handling to ensure your application can gracefully handle unexpected situations. Here are some tips for effective error handling and debugging:
1. Use Try-Catch Blocks
Wrap your API calls in try-catch blocks to catch and handle any exceptions that might occur:
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('An error occurred:', error);
}
2. Check Response Status
Always check the status of the API response before processing the data:
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
3. Implement Retry Logic
For transient errors, implement a retry mechanism with exponential backoff:
async function fetchWithRetry(url, maxRetries = 3) {
for (let i = 0; i < maxRetries; i++) {
try {
const response = await fetch(url);
if (response.ok) return response.json();
} catch (error) {
if (i === maxRetries - 1) throw error;
await new Promise(resolve => setTimeout(resolve, 2 ** i * 1000));
}
}
}
4. Use Logging
Implement comprehensive logging to track API calls and responses:
const response = await fetch('https://api.example.com/data');
console.log(`API Response Status: ${response.status}`);
const data = await response.json();
console.log('API Response Data:', data);
5. Validate Input Data
Before sending data to an API, validate it to prevent errors:
function validateUserData(userData) {
if (!userData.name || typeof userData.name !== 'string') {
throw new Error('Invalid name');
}
if (!userData.email || !userData.email.includes('@')) {
throw new Error('Invalid email');
}
// Add more validations as needed
}
API Authentication and Security
Many APIs require authentication to ensure that only authorized users can access the data. Here are some common authentication methods:
1. API Keys
API keys are simple tokens that you include with each request, usually in the header or as a query parameter:
const apiKey = 'your-api-key';
fetch('https://api.example.com/data', {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data));
2. OAuth 2.0
OAuth 2.0 is a more complex but secure authentication protocol. Here’s a simplified example:
const accessToken = 'your-access-token';
fetch('https://api.example.com/data', {
headers: {
'Authorization': `Bearer ${accessToken}`
}
})
.then(response => response.json())
.then(data => console.log(data));
3. JWT (JSON Web Tokens)
JWTs are another popular method for API authentication:
const jwt = 'your-jwt-token';
fetch('https://api.example.com/data', {
headers: {
'Authorization': `Bearer ${jwt}`
}
})
.then(response => response.json())
.then(data => console.log(data));
Security Best Practices
- Always use HTTPS for API requests to encrypt data in transit.
- Never expose API keys or tokens in client-side code.
- Implement rate limiting to prevent abuse of your API.
- Regularly rotate API keys and tokens.
- Use the principle of least privilege when granting API access.
Best Practices for Working with APIs
To ensure efficient and effective use of APIs, consider the following best practices:
1. Read the Documentation
Always start by thoroughly reading the API documentation. It will provide valuable information about endpoints, request formats, authentication methods, and any limitations or quotas.
2. Use Version Control
When working with APIs, especially if you’re building your own, use versioning to maintain backwards compatibility:
fetch('https://api.example.com/v1/data')
.then(response => response.json())
.then(data => console.log(data));
3. Implement Caching
To reduce the number of API calls and improve performance, implement caching where appropriate:
const cache = new Map();
async function fetchWithCache(url) {
if (cache.has(url)) {
return cache.get(url);
}
const response = await fetch(url);
const data = await response.json();
cache.set(url, data);
return data;
}
4. Handle Rate Limiting
Many APIs have rate limits. Implement logic to handle these limits gracefully:
async function fetchWithRateLimit(url) {
const response = await fetch(url);
if (response.status === 429) {
const retryAfter = response.headers.get('Retry-After');
await new Promise(resolve => setTimeout(resolve, retryAfter * 1000));
return fetchWithRateLimit(url);
}
return response.json();
}
5. Use Pagination
When dealing with large datasets, use pagination to fetch data in smaller chunks:
async function fetchAllData(baseUrl) {
let page = 1;
let allData = [];
while (true) {
const response = await fetch(`${baseUrl}?page=${page}`);
const data = await response.json();
if (data.length === 0) break;
allData = allData.concat(data);
page++;
}
return allData;
}
Tools and Libraries for API Development
There are numerous tools and libraries available to simplify API development and testing. Here are some popular ones:
1. Postman
Postman is a powerful tool for API development and testing. It allows you to send requests, inspect responses, and automate API tests.
2. Swagger/OpenAPI
Swagger (now known as OpenAPI) is a set of tools for designing, building, and documenting RESTful APIs.
3. Axios
Axios is a popular JavaScript library for making HTTP requests. It provides a simple and consistent API across different environments:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error:', error);
});
4. GraphQL
While not a tool per se, GraphQL is a query language for APIs that provides a more efficient, powerful and flexible alternative to REST:
const query = `
query {
user(id: "123") {
name
email
posts {
title
}
}
}
`;
fetch('https://api.example.com/graphql', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ query })
})
.then(res => res.json())
.then(result => console.log(result));
5. Insomnia
Similar to Postman, Insomnia is another popular API client that helps in designing, debugging, and testing APIs.
Conclusion
Working with APIs is a fundamental skill for modern software development. By understanding how to make and handle API requests, implement proper error handling and security measures, and follow best practices, you’ll be well-equipped to build robust and efficient applications that leverage the power of APIs.
Remember, the world of APIs is vast and constantly evolving. Stay curious, keep learning, and don’t hesitate to explore new APIs and tools as they become available. With practice and experience, you’ll become proficient in working with APIs, opening up endless possibilities for creating innovative and interconnected software solutions.
As you continue your journey in software development, consider exploring more advanced topics related to APIs, such as creating your own APIs, implementing real-time APIs with WebSockets, or diving deeper into API design principles. The skills you’ve learned here will serve as a solid foundation for these more advanced concepts.
Happy coding, and may your API requests always return 200 OK!