How to Visualize Problems Using Diagrams and Charts: A Comprehensive Guide
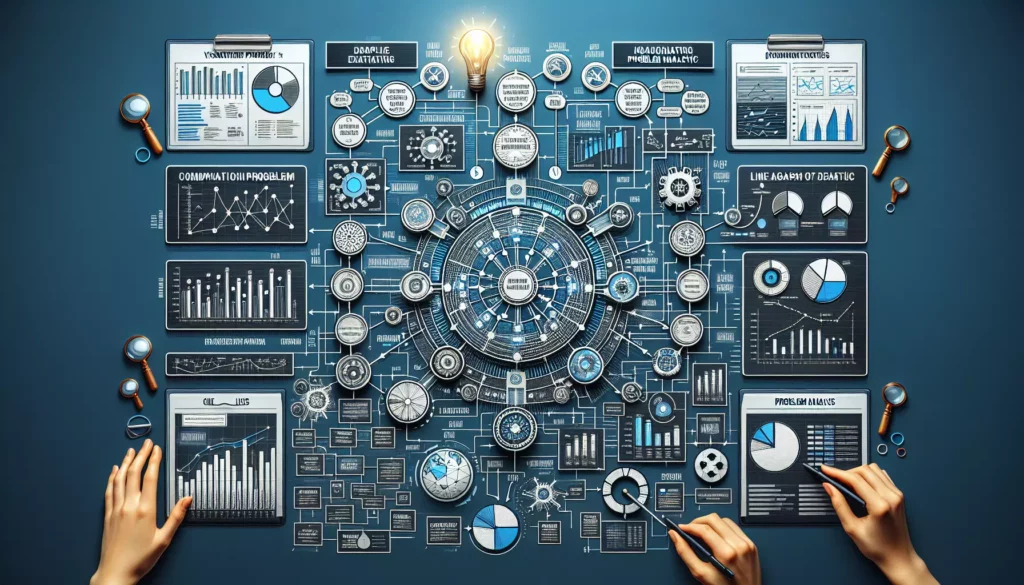
In the world of programming and problem-solving, the ability to visualize complex problems is an invaluable skill. As we dive into the realm of coding education and algorithmic thinking, it’s crucial to understand how diagrams and charts can significantly enhance our problem-solving capabilities. This comprehensive guide will explore various techniques to visualize problems, making them more manageable and easier to solve.
1. Introduction to Problem Visualization
Before we delve into specific diagrams and charts, let’s understand why visualization is so important in problem-solving:
- Clarity: Visual representations can simplify complex problems.
- Communication: Diagrams make it easier to explain problems to others.
- Pattern Recognition: Visuals help identify patterns and relationships.
- Memory Aid: Visual information is often easier to remember than text.
- Problem Decomposition: Diagrams can break down large problems into smaller, manageable parts.
2. Flowcharts: The Backbone of Problem Visualization
Flowcharts are perhaps the most fundamental and versatile tool for visualizing problems, especially in programming. They represent a process or algorithm as a series of steps, decisions, and actions.
Key Components of a Flowchart:
- Start/End: Oval shapes indicate the beginning and end of a process.
- Process: Rectangles represent actions or operations.
- Decision: Diamonds show points where a decision must be made.
- Arrows: Lines with arrowheads show the flow direction.
Example: Creating a Simple Flowchart
Let’s create a flowchart for a simple “Guess the Number” game:
<!-- Flowchart representation in ASCII -->
(Start)
|
v
[Generate Random Number]
|
v
[Ask User for Guess]
|
v
<Is Guess Correct?>
/ \
Yes No
/ \
[Display [Give Hint]
"You Win!"] |
| |
| v
| <Try Again?>
| / \
| Yes No
| / \
| | |
| | v
| | (End)
| |
|<-----
|
v
(End)
This flowchart clearly shows the game’s logic, including the main process, decision points, and possible outcomes.
3. Mind Maps: Brainstorming and Concept Organization
Mind maps are excellent for brainstorming and organizing ideas around a central concept. They’re particularly useful in the early stages of problem-solving when you’re trying to understand all aspects of a problem.
How to Create a Mind Map:
- Start with a central idea or problem in the middle of your page.
- Branch out with main themes or categories related to the central idea.
- Add subtopics to each main branch.
- Use colors, images, or symbols to enhance visual appeal and memory retention.
Example: Mind Map for a Web Development Project
<!-- ASCII representation of a mind map -->
+-----------------+
| Web Project |
+-----------------+
|
+--------+--------+
| |
+-------v------+ +------v------+
| Front-end | | Back-end |
+--------------+ +-------------+
| | | |
| | | |
+-v--+ +--v-+ +-v-+ +--v-+
|HTML| |CSS | |API| |DB |
+----+ +----+ +---+ +----+
This mind map quickly outlines the main components of a web development project, helping to organize thoughts and identify key areas to focus on.
4. UML Diagrams: Visualizing Object-Oriented Systems
Unified Modeling Language (UML) diagrams are essential for visualizing object-oriented systems and relationships between classes. They’re particularly useful in software design and analysis.
Types of UML Diagrams:
- Class Diagrams: Show static structure of the system.
- Sequence Diagrams: Illustrate interactions between objects over time.
- Use Case Diagrams: Depict system functionality from a user’s perspective.
- Activity Diagrams: Similar to flowcharts but focus on the flow of control in a system.
Example: Simple Class Diagram
<!-- ASCII representation of a class diagram -->
+-------------------+
| Animal |
+-------------------+
| - name: String |
| - age: int |
+-------------------+
| + makeSound(): void |
+-------------------+
^
|
+---------+---------+
| |
+----------+ +----------+
| Dog | | Cat |
+----------+ +----------+
| - breed | | - color |
+----------+ +----------+
| + bark() | | + meow() |
+----------+ +----------+
This class diagram shows the relationships between an Animal superclass and its Dog and Cat subclasses, clearly illustrating inheritance and class-specific attributes and methods.
5. Data Flow Diagrams: Visualizing Data Movement
Data Flow Diagrams (DFDs) are perfect for visualizing how data moves through a system. They’re particularly useful in systems analysis and database design.
Components of a DFD:
- External Entities: Sources or destinations of data (rectangles).
- Processes: Actions that transform data (circles).
- Data Stores: Where data is held (open-ended rectangles).
- Data Flows: Movement of data (arrows).
Example: Simple DFD for an Online Shopping System
<!-- ASCII representation of a Data Flow Diagram -->
[Customer] --> (Place Order) --> [Order Database]
|
v
(Process Payment) <-- [Payment Gateway]
|
v
(Update Inventory) --> [Inventory Database]
|
v
(Ship Order) --> [Shipping Company]
This DFD clearly shows how data flows through an online shopping system, from the customer placing an order to the final shipping process.
6. Entity-Relationship Diagrams: Modeling Database Structures
Entity-Relationship Diagrams (ERDs) are crucial for visualizing database structures. They show the relationships between different entities (tables) in a database.
Key Components of an ERD:
- Entities: Represented by rectangles (e.g., Customer, Order).
- Attributes: Characteristics of entities (e.g., customer_id, name).
- Relationships: How entities are related (e.g., one-to-many, many-to-many).
Example: Simple ERD for an E-commerce Database
<!-- ASCII representation of an Entity-Relationship Diagram -->
+-------------+ +-----------+
| Customer | | Order |
+-------------+ +-----------+
| customer_id |<--->o| order_id |
| name | | date |
| email | | total |
+-------------+ +-----------+
|
|
v
+--------------+
| Order_Item |
+--------------+
| order_id |
| product_id |
| quantity |
+--------------+
^
|
+--------------+
| Product |
+--------------+
| product_id |
| name |
| price |
+--------------+
This ERD shows the relationships between customers, orders, order items, and products in an e-commerce database system.
7. Gantt Charts: Visualizing Project Timelines
While not directly related to coding problems, Gantt charts are invaluable for visualizing project timelines and task dependencies. They’re especially useful in project management and planning complex coding projects.
Key Components of a Gantt Chart:
- Tasks: Listed vertically.
- Timeline: Horizontal axis showing dates or time periods.
- Bars: Represent the duration of each task.
- Dependencies: Arrows showing how tasks relate to each other.
Example: Simple Gantt Chart for a Software Development Project
<!-- ASCII representation of a Gantt Chart -->
Task Week 1 Week 2 Week 3 Week 4
Planning ====
Design --------====
Development --------========
Testing --------====
Deployment ----
This Gantt chart clearly shows the timeline and overlaps of different phases in a software development project.
8. Decision Trees: Visualizing Complex Decision-Making Processes
Decision trees are excellent for visualizing complex decision-making processes, especially in algorithms involving multiple conditions and outcomes.
Components of a Decision Tree:
- Root Node: The starting point of the decision process.
- Internal Nodes: Represent tests on attributes or conditions.
- Branches: Outcomes of the tests.
- Leaf Nodes: Final decisions or outcomes.
Example: Decision Tree for a Simple Classification Problem
<!-- ASCII representation of a Decision Tree -->
[Is it raining?]
/ \
Yes No
/ \
[Is it windy?] [Temperature?]
/ \ / | \
Yes No <0°C 0-20°C >20°C
/ \ | | |
[Stay in] [Take | | |
umbrella] | | |
[Stay in] | [Go out]
[Take jacket]
This decision tree visualizes the process of deciding whether to go out based on weather conditions.
9. Venn Diagrams: Visualizing Set Theory and Logic
Venn diagrams are useful for visualizing relationships between different sets of data or concepts. They’re particularly helpful in set theory, logic, and database queries.
Key Components of a Venn Diagram:
- Circles: Represent sets or categories.
- Overlaps: Show commonalities between sets.
- Labels: Describe the sets and their elements.
Example: Venn Diagram for Database Query Visualization
<!-- ASCII representation of a Venn Diagram -->
+----------+
/ Table A \
/ +---+ \
| / \ |
| | C | |
| \ / |
\ +---+ /
\ Table B /
+----------+
This Venn diagram could represent a JOIN operation in SQL, where C is the intersection of Tables A and B.
10. Sequence Diagrams: Visualizing Object Interactions
Sequence diagrams are part of UML and are excellent for visualizing how objects in a system interact over time. They’re particularly useful for understanding and designing complex systems with multiple interacting components.
Key Components of a Sequence Diagram:
- Lifelines: Vertical lines representing different objects or actors in the system.
- Messages: Horizontal arrows showing interactions between lifelines.
- Activation Boxes: Rectangles on lifelines showing when an object is active or processing.
Example: Sequence Diagram for a Simple Login Process
<!-- ASCII representation of a Sequence Diagram -->
User LoginUI AuthService Database
| | | |
|--login()-->| | |
| |--validate()-->| |
| | |--query()-->|
| | |<--result--|
| |<--response--| |
|<--result---| | |
| | | |
This sequence diagram shows the interactions between different components during a login process, clearly illustrating the flow of actions and data.
11. State Diagrams: Visualizing System States and Transitions
State diagrams, also part of UML, are used to visualize the different states a system or object can be in and how it transitions between these states. They’re particularly useful for modeling the behavior of complex systems or algorithms.
Key Components of a State Diagram:
- States: Represented by rounded rectangles.
- Transitions: Arrows showing how the system moves between states.
- Events: Labels on transitions indicating what causes the state change.
- Actions: Activities that occur when entering, exiting, or during a state.
Example: State Diagram for a Simple Order Processing System
<!-- ASCII representation of a State Diagram -->
+----------+
| Pending |
+----------+
|
[Payment Received]
|
v
+--------------+
| Processing |
+--------------+
|
[Items Packed]
|
v
+------------+
| Shipped |
+------------+
|
[Delivery Confirmed]
|
v
+-------------+
| Completed |
+-------------+
This state diagram clearly shows the different states an order can be in and the events that trigger transitions between these states.
12. Network Diagrams: Visualizing Interconnected Systems
Network diagrams are crucial for visualizing interconnected systems, whether they’re computer networks, social networks, or any other type of interconnected structure. They’re particularly useful in network design, graph theory problems, and social network analysis.
Key Components of a Network Diagram:
- Nodes: Represent entities in the network (computers, people, etc.).
- Edges: Lines connecting nodes, representing relationships or connections.
- Labels: Descriptions of nodes or edges.
- Directionality: Arrows on edges to show direction of relationship (if applicable).
Example: Simple Network Diagram for a Small Office Network
<!-- ASCII representation of a Network Diagram -->
[Internet]
|
|
[Router]
/ | \
/ | \
[PC1] [PC2] [Printer]
\ | /
\ | /
[Switch]
| |
[Server] [NAS]
This network diagram shows the basic structure of a small office network, including the connections between different devices.
13. Histogram and Bar Charts: Visualizing Data Distribution
While more commonly associated with data analysis, histograms and bar charts can be valuable tools for visualizing certain types of programming problems, especially those dealing with data distribution or frequency analysis.
Key Components:
- X-axis: Categories or ranges of data.
- Y-axis: Frequency or count.
- Bars: Represent the frequency for each category or range.
Example: Histogram of Array Element Frequencies
<!-- ASCII representation of a Histogram -->
Frequency |
10 | ##
9 | ##
8 | ## ##
7 | ## ##
6 | ## ## ##
5 | ## ## ##
4 | ## ## ## ##
3 | ## ## ## ## ## ##
2 | ## ## ## ## ## ##
1 | ## ## ## ## ## ##
0 +-------------------------
1 2 3 4 5 6 7 8 9
Array Elements
This histogram visualizes the frequency distribution of elements in an array, which can be useful for understanding data patterns or identifying outliers in certain algorithms.
14. Conclusion: The Power of Visual Problem-Solving
Visualizing problems using diagrams and charts is a powerful technique that can significantly enhance your problem-solving skills in programming and beyond. By representing complex ideas visually, you can:
- Gain a clearer understanding of the problem at hand.
- Communicate ideas more effectively to team members or stakeholders.
- Identify patterns and relationships that might not be apparent in text or code alone.
- Break down complex problems into more manageable components.
- Develop more efficient and effective solutions.
As you continue your journey in coding education and skills development, remember that these visualization techniques are valuable tools in your problem-solving toolkit. Whether you’re tackling algorithmic challenges, designing systems, or preparing for technical interviews, the ability to visualize problems can give you a significant advantage.
Practice incorporating these various diagrams and charts into your problem-solving process. Start with simpler visualizations like flowcharts and mind maps, and gradually work your way up to more complex representations like UML diagrams and ERDs. With time and practice, you’ll find that visual problem-solving becomes an integral and natural part of your approach to coding and software development.
Remember, the goal of visualization is not just to create pretty pictures, but to gain deeper insights into the problems you’re solving. Use these tools strategically to enhance your understanding, improve your solutions, and ultimately become a more effective and efficient problem solver in the world of programming.