How to Verbalize Your Solution Before Writing Any Code
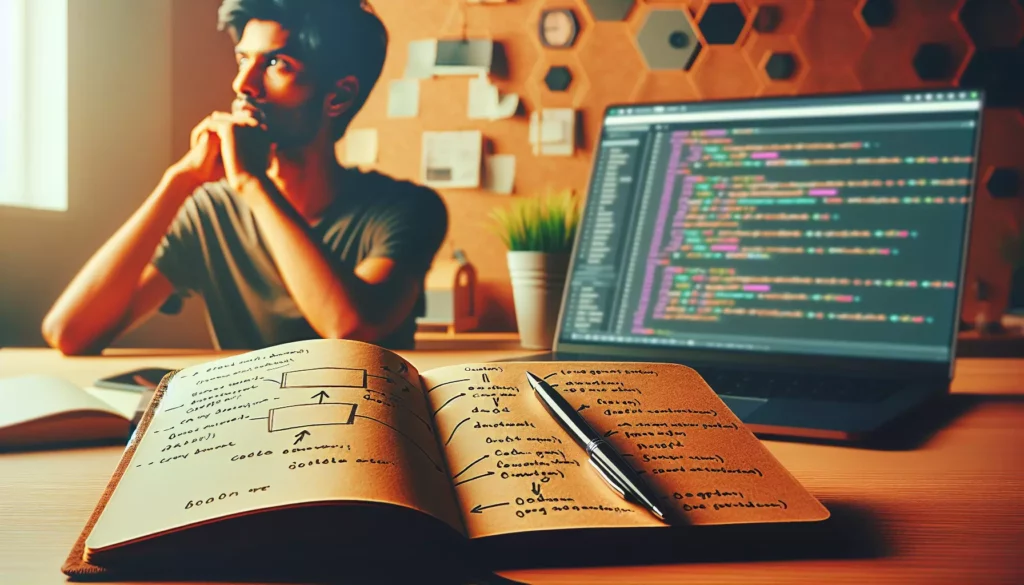
In the world of programming and software development, the ability to articulate your solution before diving into code is a crucial skill that often separates novice programmers from seasoned professionals. This practice, known as verbalizing or explaining your solution, is not just a formality but a powerful technique that can significantly improve your problem-solving abilities, code quality, and overall efficiency as a developer. In this comprehensive guide, we’ll explore the importance of verbalizing your solution, the benefits it brings, and practical steps to master this essential skill.
Why Verbalize Your Solution?
Before we delve into the how-to, let’s understand why verbalizing your solution is so important:
- Clarity of Thought: Explaining your solution out loud or in writing forces you to organize your thoughts and identify any gaps in your understanding.
- Problem Decomposition: It helps break down complex problems into smaller, manageable parts.
- Catch Logical Errors: You’re more likely to spot flaws in your logic when you articulate each step.
- Improved Communication: It enhances your ability to explain technical concepts to others, a valuable skill in team environments.
- Better Code Structure: A well-verbalized solution often translates into well-structured, readable code.
- Time-Saving: While it may seem time-consuming initially, it often saves time in the long run by reducing debugging and refactoring efforts.
The Process of Verbalizing Your Solution
Now that we understand the importance, let’s break down the process of verbalizing your solution into actionable steps:
1. Understand the Problem
Before you can verbalize a solution, you need to fully grasp the problem at hand. This involves:
- Reading the problem statement carefully, multiple times if necessary.
- Identifying the inputs and expected outputs.
- Noting any constraints or special conditions.
- Asking clarifying questions if anything is ambiguous.
For example, if you’re tackling a problem to find the maximum subarray sum, you might verbalize your understanding as:
“The problem asks to find the contiguous subarray within a one-dimensional array of numbers that has the largest sum. The input is an array of integers, which can be positive or negative. The output should be a single integer representing the maximum sum. There are no specific constraints on the array size or the range of integers.”
2. Break Down the Problem
Once you understand the problem, break it down into smaller, manageable parts. This step is crucial for complex problems. Verbalize each part of the problem and how they relate to each other. For our maximum subarray sum example:
“To solve this, we need to:
1. Iterate through the array.
2. Keep track of the current sum as we iterate.
3. Compare the current sum with the maximum sum we’ve seen so far.
4. Handle cases where the current sum becomes negative.
5. Return the maximum sum found.”
3. Outline Your Approach
Now, describe your high-level approach to solving the problem. This doesn’t involve code yet, but rather a general strategy. For our example:
“I’ll use Kadane’s algorithm to solve this problem efficiently. The idea is to maintain two variables: ‘current_sum’ and ‘max_sum’. We’ll iterate through the array once, updating these variables. If ‘current_sum’ becomes negative, we’ll reset it to zero, as any negative sum is not worth keeping in a contiguous subarray aiming for the maximum sum.”
4. Detail the Algorithm
With your high-level approach in mind, detail the step-by-step algorithm. Be as specific as possible without writing actual code. For our maximum subarray sum problem:
“1. Initialize two variables: ‘current_sum’ and ‘max_sum’ to the first element of the array.
2. Iterate through the array starting from the second element:
a. Add the current element to ‘current_sum’.
b. If ‘current_sum’ is greater than ‘max_sum’, update ‘max_sum’ to ‘current_sum’.
c. If ‘current_sum’ becomes negative, reset it to 0.
3. After the iteration, return ‘max_sum’.”
5. Consider Edge Cases
Verbalize any edge cases or special scenarios your solution needs to handle. This step often reveals overlooked aspects of the problem. For our example:
“We need to consider the following edge cases:
1. An empty array: We should return 0 or null, depending on the problem specification.
2. An array with all negative numbers: The algorithm should still work and return the least negative number.
3. An array with a single element: The algorithm should return that element.”
6. Analyze Time and Space Complexity
Before coding, it’s crucial to verbalize the efficiency of your solution. This helps you understand if your approach is optimal or if you need to consider alternatives. For our Kadane’s algorithm example:
“Time Complexity: O(n), where n is the length of the array. We’re making a single pass through the array.
Space Complexity: O(1), as we’re using only two variables (current_sum and max_sum) regardless of the input size.”
7. Pseudo-code (Optional)
For more complex problems, it can be helpful to write pseudo-code before actual coding. This bridges the gap between your verbal explanation and the final code. Here’s how it might look for our example:
function maxSubarraySum(arr):
if arr is empty:
return 0 // or null, based on problem specification
current_sum = max_sum = arr[0]
for i from 1 to arr.length - 1:
current_sum = max(arr[i], current_sum + arr[i])
max_sum = max(max_sum, current_sum)
return max_sum
Benefits of Verbalizing in Different Scenarios
The process of verbalizing your solution is beneficial in various programming scenarios:
1. Technical Interviews
In technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), verbalizing your solution is often expected and highly valued. It allows the interviewer to:
- Understand your thought process
- Assess your problem-solving skills
- Evaluate your communication abilities
- Provide guidance if you’re heading in the wrong direction
By clearly articulating your approach before coding, you demonstrate not just coding ability, but also the crucial soft skills that make a great team member.
2. Pair Programming
When engaged in pair programming, verbalizing your solution helps:
- Align both programmers on the approach
- Facilitate discussion and idea sharing
- Catch potential issues early in the development process
- Improve code quality through collaborative thinking
3. Code Reviews
When submitting code for review, including a verbal explanation of your solution can:
- Make it easier for reviewers to understand your code
- Justify design decisions
- Highlight areas where you’re unsure and need feedback
- Speed up the review process
4. Self-Learning and Problem-Solving
Even when coding alone, verbalizing your solution:
- Helps clarify your thoughts
- Forces you to think through the problem thoroughly
- Can reveal alternative approaches you hadn’t considered
- Improves your ability to explain technical concepts, which is valuable for documentation and future discussions
Common Pitfalls to Avoid
While verbalizing your solution is incredibly beneficial, there are some common pitfalls to be aware of:
1. Jumping to Code Too Quickly
It’s tempting to start coding immediately, especially if you think you see a solution. Resist this urge. Take the time to fully verbalize your approach first. This often reveals flaws or optimizations you might have missed.
2. Being Too Vague
Avoid general statements like “I’ll use a loop to solve this.” Instead, be specific about what the loop does, what conditions it checks, and how it contributes to the solution.
3. Ignoring Edge Cases
Don’t forget to verbalize how your solution handles edge cases. This demonstrates thorough thinking and helps prevent bugs in your final code.
4. Overlooking Time and Space Complexity
Always verbalize the efficiency of your solution. This shows you’re thinking about performance, not just functionality.
5. Not Considering Alternative Approaches
Even if your first idea seems solid, verbalize any alternative approaches you’ve considered. This shows depth of thought and can lead to more optimal solutions.
Practicing Verbalization
Like any skill, verbalizing your solution improves with practice. Here are some ways to hone this skill:
1. Solve Problems Aloud
When working through coding problems, practice explaining your thought process out loud, even if you’re alone. This gets you comfortable with articulating your ideas.
2. Use a Rubber Duck
The “rubber duck debugging” technique involves explaining your code to an inanimate object (traditionally a rubber duck). This forces you to verbalize your solution in detail and often helps you spot issues on your own.
3. Participate in Coding Challenges
Platforms like LeetCode, HackerRank, or AlgoCademy offer coding challenges. Practice not just solving the problems, but writing out your approach before coding.
4. Teach Others
Teaching a concept to someone else is one of the best ways to solidify your understanding. Look for opportunities to explain coding concepts or solutions to peers or in online forums.
5. Record Yourself
Try recording yourself as you verbalize solutions. This allows you to review your explanation, identify areas for improvement, and get more comfortable with the process.
Conclusion
Verbalizing your solution before writing code is a powerful technique that can significantly improve your problem-solving skills, code quality, and efficiency as a programmer. It’s a skill valued highly in technical interviews, especially for positions at top tech companies, and is equally beneficial in collaborative coding environments and personal projects.
By following the steps outlined in this guide – from understanding the problem to detailing your algorithm and considering edge cases – you’ll develop a structured approach to problem-solving that will serve you well throughout your programming career. Remember, the goal is not just to solve the problem, but to solve it thoughtfully and communicatively.
As you continue to practice and refine this skill, you’ll likely find that your ability to tackle complex coding challenges improves, your code becomes more robust and efficient, and your capacity to work effectively in team environments enhances. So the next time you face a coding problem, take a step back, verbalize your solution, and watch how it transforms your approach to programming.