How to Use Test Cases to Improve Your Coding Challenge Performance
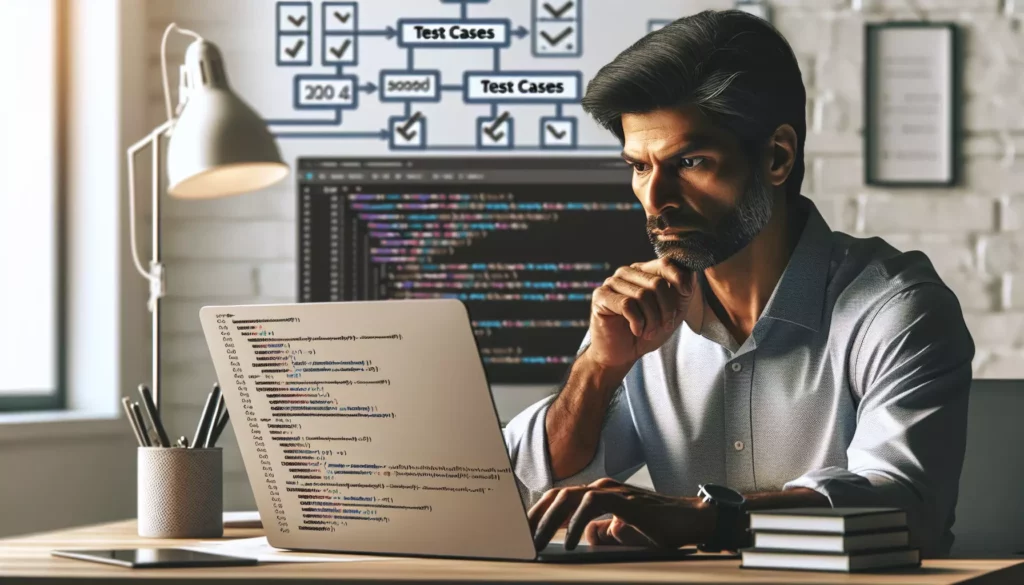
In the world of coding interviews and technical assessments, mastering the art of using test cases can be a game-changer. Whether you’re a beginner coder or an experienced developer preparing for interviews with top tech companies, understanding how to leverage test cases effectively can significantly boost your performance in coding challenges. This comprehensive guide will walk you through the importance of test cases, how to create them, and strategies to use them for improving your problem-solving skills.
Understanding the Importance of Test Cases
Before diving into the specifics of using test cases, it’s crucial to understand why they are so important in the context of coding challenges and interviews.
1. Validation of Code Correctness
Test cases serve as a primary means of validating whether your code produces the expected output for given inputs. They help ensure that your solution works correctly across various scenarios.
2. Edge Case Identification
Well-crafted test cases can help you identify edge cases and corner scenarios that you might have overlooked in your initial implementation.
3. Performance Evaluation
Test cases can be designed to evaluate the performance of your code, helping you understand if your solution meets the required time and space complexity constraints.
4. Debugging Aid
When your code fails a test case, it provides valuable information for debugging, allowing you to pinpoint where and why your solution is failing.
Creating Effective Test Cases
Now that we understand the importance of test cases, let’s explore how to create effective ones for coding challenges.
1. Start with the Given Examples
Most coding challenges come with example inputs and expected outputs. Always start by converting these into test cases. For instance:
def test_example_cases():
assert solution([1, 2, 3, 4, 5]) == 15
assert solution([]) == 0
assert solution([-1, -2, -3]) == -6
2. Consider Edge Cases
Think about extreme or unusual inputs that could potentially break your code. Some common edge cases include:
- Empty input (e.g., empty array, empty string)
- Very large or very small numbers
- Negative numbers (if applicable)
- Single element input
- Input with all identical elements
def test_edge_cases():
assert solution([1000000000]) == 1000000000
assert solution([-2147483648, 2147483647]) == -1
assert solution([42] * 100000) == 4200000
3. Test Boundary Conditions
If the problem specifies certain constraints (e.g., array length between 1 and 10^5), create test cases that check these boundaries:
def test_boundary_conditions():
assert solution([1] * 100000) == 100000
assert solution([1]) == 1
4. Include Random Test Cases
Generate random inputs to test your solution more thoroughly. This can help uncover unexpected issues:
import random
def test_random_cases():
for _ in range(100):
arr = [random.randint(-1000, 1000) for _ in range(random.randint(1, 1000))]
expected = sum(arr)
assert solution(arr) == expected
Strategies for Using Test Cases Effectively
Having a good set of test cases is just the beginning. Here are strategies to use them effectively in improving your coding challenge performance:
1. Test-Driven Development (TDD)
Adopt a TDD approach where you write test cases before implementing your solution. This helps you clarify the problem requirements and think through different scenarios.
- Write a failing test case
- Implement the minimum code to pass the test
- Refactor your code
- Repeat with the next test case
2. Incremental Testing
As you implement your solution, run your test cases frequently. This helps catch errors early and makes debugging easier.
def solution(arr):
# Initial implementation
return sum(arr)
# Run tests after initial implementation
test_example_cases()
test_edge_cases()
# Refine implementation if needed
def solution(arr):
if not arr:
return 0
return sum(arr)
# Run tests again
test_example_cases()
test_edge_cases()
test_boundary_conditions()
3. Use Test Cases for Debugging
When your solution fails a test case, use it as a debugging tool:
- Identify the failing test case
- Add print statements or use a debugger to trace the execution for that specific input
- Analyze the intermediate results to understand where your logic is failing
def solution(arr):
print(f"Input: {arr}") # Debugging print
result = sum(arr)
print(f"Output: {result}") # Debugging print
return result
# Run the failing test case
assert solution([-2147483648, 2147483647]) == -1
4. Optimize Using Test Cases
Use your test cases to measure and improve the performance of your solution:
- Implement a basic working solution
- Run performance tests with large inputs
- Identify bottlenecks and optimize
- Re-run tests to verify improvements
import time
def performance_test(func, arr):
start_time = time.time()
result = func(arr)
end_time = time.time()
print(f"Time taken: {end_time - start_time} seconds")
return result
# Test initial solution
large_input = list(range(100000))
performance_test(solution, large_input)
# Optimize and test again
def optimized_solution(arr):
return sum(arr)
performance_test(optimized_solution, large_input)
Common Pitfalls to Avoid
While using test cases can greatly improve your coding challenge performance, there are some common pitfalls to be aware of:
1. Overfitting to Test Cases
Be cautious not to create a solution that only works for your specific test cases but fails for other valid inputs. Always consider the general problem and constraints.
2. Ignoring Time and Space Complexity
Don’t focus solely on passing test cases. Ensure your solution meets the required time and space complexity constraints, especially for large inputs.
3. Neglecting Code Readability
In the rush to pass all test cases, don’t sacrifice code readability and maintainability. Clean, well-structured code is often just as important as a correct solution.
4. Forgetting to Handle Invalid Inputs
Ensure your solution gracefully handles invalid inputs, even if they’re not explicitly mentioned in the problem statement. This shows attention to detail and robust coding practices.
def solution(arr):
if not isinstance(arr, list):
raise ValueError("Input must be a list")
return sum(arr) if arr else 0
def test_invalid_inputs():
try:
solution("not a list")
except ValueError:
print("Correctly handled invalid input")
else:
print("Failed to handle invalid input")
Advanced Test Case Techniques
As you become more proficient with test cases, consider these advanced techniques to further enhance your coding challenge performance:
1. Parameterized Tests
Use parameterized tests to run the same test logic with different inputs, reducing code duplication and improving test coverage:
import pytest
@pytest.mark.parametrize("input_arr, expected", [
([1, 2, 3], 6),
([-1, -2, -3], -6),
([0, 0, 0], 0),
([1000000000, -1000000000], 0)
])
def test_solution(input_arr, expected):
assert solution(input_arr) == expected
2. Property-Based Testing
Employ property-based testing libraries like Hypothesis for Python to generate a wide range of test cases based on specified properties:
from hypothesis import given
from hypothesis.strategies import lists, integers
@given(lists(integers()))
def test_solution_properties(arr):
result = solution(arr)
assert result == sum(arr)
assert isinstance(result, int)
3. Mutation Testing
Use mutation testing tools to evaluate the quality of your test cases by introducing small changes (mutations) to your code and checking if your tests catch these changes:
# Example using the mutmut library for Python
# Run: mutmut run --paths-to-mutate=./solution.py
def solution(arr):
return sum(arr)
def test_solution():
assert solution([1, 2, 3]) == 6
assert solution([-1, -2, -3]) == -6
assert solution([]) == 0
4. Benchmarking
Implement benchmarking to compare different solutions or optimizations:
import timeit
def benchmark(func, input_arr):
return timeit.timeit(lambda: func(input_arr), number=1000)
# Compare two implementations
result1 = benchmark(solution1, large_input)
result2 = benchmark(solution2, large_input)
print(f"Solution 1 time: {result1}")
print(f"Solution 2 time: {result2}")
print(f"Speedup: {result1 / result2:.2f}x")
Integrating Test Cases into Your Problem-Solving Workflow
To truly leverage the power of test cases in improving your coding challenge performance, integrate them into your problem-solving workflow:
1. Problem Analysis
As you read and analyze the problem statement, start jotting down potential test cases. This helps in understanding the problem requirements and constraints more thoroughly.
2. Solution Design
While designing your solution, think about how you’ll test each component or edge case. This can guide your implementation and help you catch potential issues early.
3. Implementation
Implement your solution incrementally, writing and running test cases for each part as you go. This approach helps in building a robust solution step by step.
4. Optimization
Use your comprehensive test suite to verify that optimizations don’t break existing functionality and to measure performance improvements.
5. Review and Refactor
After your solution passes all test cases, review your code and test cases. Look for opportunities to refactor for better readability or efficiency, always re-running your tests after each change.
Leveraging AI-Powered Assistance for Test Case Generation
In the era of AI, leveraging tools like ChatGPT or other AI-powered coding assistants can significantly enhance your test case generation process:
1. Generating Diverse Test Cases
Use AI to brainstorm a wide range of test cases, including edge cases you might not have considered:
Human: Generate 5 diverse test cases for a function that finds the longest palindromic substring in a given string.
AI: Here are 5 diverse test cases for the longest palindromic substring function:
1. Input: "babad"
Expected Output: "bab" or "aba"
2. Input: "cbbd"
Expected Output: "bb"
3. Input: "a"
Expected Output: "a"
4. Input: "abcdefghijklmnopqrstuvwxyz"
Expected Output: "a" (or any single character)
5. Input: "aaaaaaaaaaaaaaaaaaaaaaaaaaa"
Expected Output: "aaaaaaaaaaaaaaaaaaaaaaaaaaa"