How to Use Recursion Effectively in Algorithmic Problem Solving
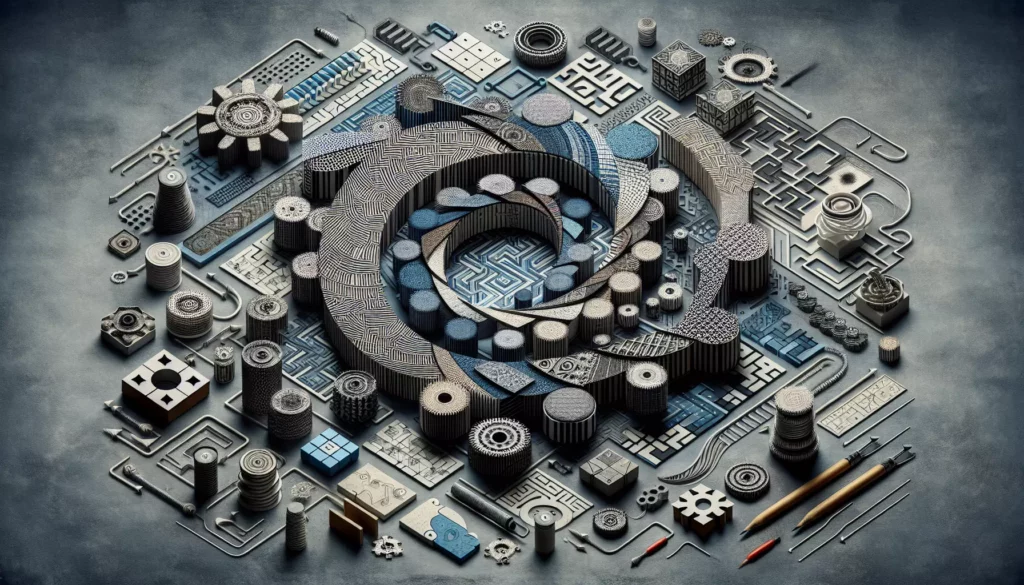
Recursion is a powerful programming technique that can be used to solve complex problems elegantly and efficiently. It’s a fundamental concept in computer science and a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore how to use recursion effectively in algorithmic problem solving, with a focus on its application in coding interviews and real-world scenarios.
Understanding Recursion
Before diving into the practical applications of recursion, it’s essential to have a solid understanding of what recursion is and how it works.
What is Recursion?
Recursion is a method of solving a problem where the solution depends on solutions to smaller instances of the same problem. In programming, recursion occurs when a function calls itself directly or indirectly.
The Anatomy of a Recursive Function
A typical recursive function consists of two main parts:
- Base case(s): The simplest scenario(s) where the function can return a result without making any recursive calls.
- Recursive case(s): The scenario(s) where the function calls itself with a simpler or smaller input.
Here’s a simple example of a recursive function that calculates the factorial of a number:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1)
When to Use Recursion
Recursion can be an excellent tool for solving certain types of problems. Here are some scenarios where recursion shines:
1. Problems with a Recursive Structure
Many problems in computer science have a naturally recursive structure. These include:
- Tree traversals
- Graph traversals
- Divide-and-conquer algorithms
- Backtracking algorithms
2. Problems That Can Be Broken Down into Smaller Subproblems
If a problem can be divided into smaller, similar subproblems, recursion might be a good fit. Examples include:
- Sorting algorithms (e.g., Merge Sort, Quick Sort)
- Dynamic programming problems
- Combinatorial problems
3. When Simplicity is Preferred Over Performance
In some cases, a recursive solution might be more intuitive and easier to understand than an iterative one, even if it’s not the most efficient approach.
Effective Recursion Techniques
To use recursion effectively in algorithmic problem solving, consider the following techniques:
1. Identify the Base Case(s)
The base case is crucial in preventing infinite recursion. Always start by identifying the simplest scenario(s) where the function can return a result without making any recursive calls.
2. Define the Recursive Case
Determine how to break down the problem into smaller subproblems. Ensure that each recursive call moves towards the base case.
3. Trust the Recursion
When writing recursive functions, it’s important to trust that the recursive calls will work correctly. Focus on solving the current problem assuming the recursive calls will handle the rest.
4. Use Helper Functions
Sometimes, it’s useful to create a helper function that does the actual recursion, while the main function sets up the initial state or handles edge cases.
5. Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Some programming languages and compilers can optimize tail-recursive functions to use constant stack space.
Common Recursive Patterns
Several common patterns emerge when solving problems recursively. Familiarizing yourself with these patterns can help you recognize when and how to apply recursion effectively.
1. Linear Recursion
In linear recursion, each function call makes at most one recursive call. This is the simplest form of recursion.
Example: Calculating the sum of an array
def array_sum(arr):
if len(arr) == 0:
return 0
else:
return arr[0] + array_sum(arr[1:])
2. Binary Recursion
Binary recursion involves making two recursive calls in each function call. This is common in divide-and-conquer algorithms.
Example: Merge Sort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
3. Multiple Recursion
Multiple recursion involves making more than two recursive calls in each function call. This is often seen in problems involving combinatorics or dynamic programming.
Example: Calculating Fibonacci numbers
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
4. Mutual Recursion
Mutual recursion occurs when two or more functions call each other recursively. This can be useful for problems with alternating states or conditions.
Example: Determining if a number is even or odd
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
Recursion in Tree and Graph Traversals
Recursion is particularly useful when working with tree and graph data structures. Let’s look at some common examples:
1. Binary Tree Traversal
Recursive functions can elegantly traverse binary trees in various orders:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if not root:
return []
return (inorder_traversal(root.left) +
[root.val] +
inorder_traversal(root.right))
def preorder_traversal(root):
if not root:
return []
return ([root.val] +
preorder_traversal(root.left) +
preorder_traversal(root.right))
def postorder_traversal(root):
if not root:
return []
return (postorder_traversal(root.left) +
postorder_traversal(root.right) +
[root.val])
2. Depth-First Search (DFS) in Graphs
Recursion is a natural fit for implementing depth-first search in graphs:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
dfs(graph, 'A')
Recursion in Dynamic Programming
Dynamic programming problems often have a recursive structure, but naive recursive solutions can be inefficient due to redundant calculations. By combining recursion with memoization, we can create efficient solutions to dynamic programming problems.
Example: Fibonacci Sequence with Memoization
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
Common Pitfalls and How to Avoid Them
While recursion can be a powerful tool, it’s important to be aware of potential pitfalls:
1. Stack Overflow
Recursive functions can lead to stack overflow errors if the recursion depth is too large. To avoid this:
- Ensure your base cases are correct and reachable.
- Consider using tail recursion where possible.
- For very deep recursions, consider an iterative approach or increase the stack size.
2. Redundant Calculations
Naive recursive implementations can lead to exponential time complexity due to redundant calculations. To mitigate this:
- Use memoization or dynamic programming techniques.
- Consider bottom-up iterative solutions for some problems.
3. Incorrect Base Cases
Failing to properly define base cases can lead to infinite recursion or incorrect results. Always carefully consider and test your base cases.
Recursion vs. Iteration
While recursion can lead to elegant solutions, it’s not always the best choice. Here are some factors to consider when deciding between recursion and iteration:
Pros of Recursion:
- Can lead to cleaner, more intuitive code for certain problems.
- Natural fit for problems with recursive structure (e.g., tree traversals).
- Can be easier to prove correctness for some algorithms.
Cons of Recursion:
- Can lead to stack overflow for deep recursions.
- Often less efficient in terms of time and space complexity.
- May be harder to debug and trace.
When to Choose Recursion:
- When the problem has a natural recursive structure.
- When the recursive solution is significantly clearer than the iterative one.
- When performance is not critical, and the recursion depth is manageable.
When to Choose Iteration:
- When performance is critical.
- When dealing with large inputs that could lead to deep recursion.
- When the iterative solution is just as clear and intuitive.
Practice Problems
To master recursion, practice is key. Here are some problems to help you hone your recursive problem-solving skills:
- Implement a recursive function to calculate the power of a number (x^n).
- Write a recursive function to reverse a string.
- Implement a recursive binary search algorithm.
- Write a recursive function to generate all permutations of a string.
- Implement the Tower of Hanoi problem using recursion.
- Write a recursive function to find the longest common subsequence of two strings.
- Implement a recursive solution for the n-queens problem.
- Write a recursive function to solve the subset sum problem.
- Implement a recursive solution for the edit distance problem.
- Write a recursive function to generate all valid parentheses combinations given n pairs of parentheses.
Conclusion
Recursion is a powerful technique in algorithmic problem solving, offering elegant solutions to complex problems. By understanding when and how to use recursion effectively, you can tackle a wide range of coding challenges, from tree traversals to dynamic programming problems.
Remember, the key to mastering recursion is practice. As you work through more problems, you’ll develop an intuition for when recursion is the right tool and how to implement it effectively. Keep in mind the common patterns and pitfalls we’ve discussed, and don’t be afraid to experiment with both recursive and iterative approaches to find the best solution for each problem.
As you prepare for coding interviews or work on real-world projects, recursion will be an invaluable tool in your programming arsenal. With a solid understanding of recursion and plenty of practice, you’ll be well-equipped to tackle complex algorithmic challenges and impress potential employers at top tech companies.