How to Use Pseudocode Effectively in Technical Interviews: A Comprehensive Guide
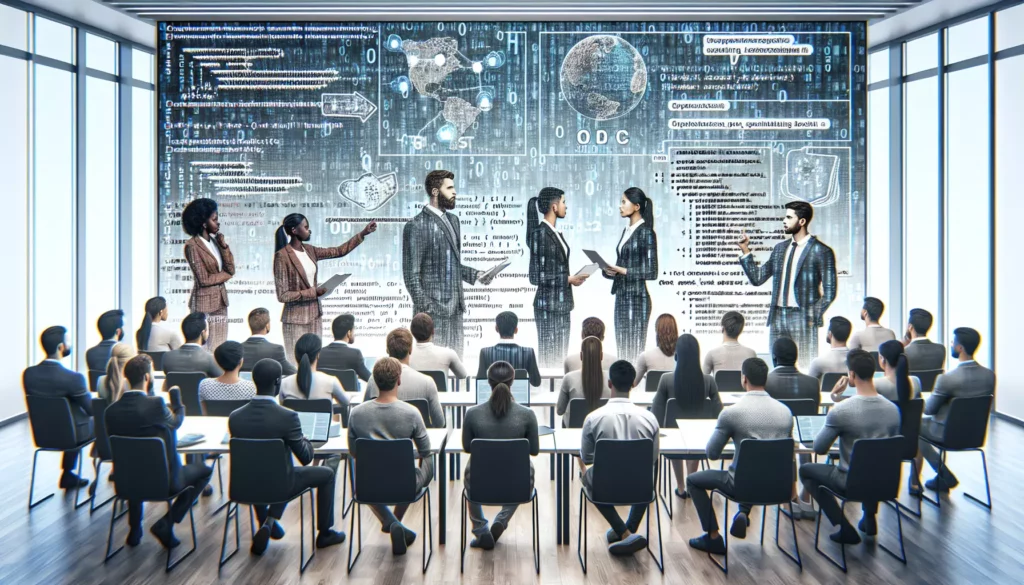
In the competitive world of technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to communicate your problem-solving process is just as crucial as your coding skills. This is where pseudocode comes into play. Pseudocode serves as a bridge between your thought process and the actual code, allowing you to outline your approach clearly before diving into implementation details. In this comprehensive guide, we’ll explore how to use pseudocode effectively in interviews, helping you showcase your algorithmic thinking and problem-solving abilities.
What is Pseudocode?
Before we dive into the specifics of using pseudocode in interviews, let’s clarify what pseudocode is. Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a programming language but is intended for human reading rather than machine reading. Essentially, it’s a way to plan out your code using plain language mixed with some basic coding structures.
Why Use Pseudocode in Interviews?
Using pseudocode in technical interviews offers several advantages:
- Clarifies Your Thinking: Writing pseudocode helps you organize your thoughts and approach to the problem before you start coding.
- Demonstrates Problem-Solving Skills: It shows the interviewer your ability to break down complex problems into manageable steps.
- Facilitates Communication: Pseudocode allows you to explain your approach clearly to the interviewer, fostering discussion and feedback.
- Reduces Coding Errors: By planning your solution in pseudocode, you’re less likely to make logical errors when you start actual coding.
- Saves Time: It’s quicker to write and modify pseudocode than to write and debug actual code, especially under interview pressure.
How to Write Effective Pseudocode for Interviews
Here are some key strategies for writing effective pseudocode during technical interviews:
1. Keep It Simple and Clear
Your pseudocode should be easy to read and understand. Use plain English and avoid unnecessary complexity. The goal is to communicate your algorithm clearly, not to impress with fancy terminology.
Example of clear pseudocode for finding the maximum number in an array:
function findMaximum(array):
set max to first element of array
for each element in array:
if element > max:
set max to element
return max
2. Use Consistent Indentation
Proper indentation helps to show the structure of your algorithm, making it easier for both you and the interviewer to follow the logic.
function binarySearch(array, target):
set left to 0
set right to length of array - 1
while left <= right:
set mid to (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
set left to mid + 1
else:
set right to mid - 1
return -1 // Target not found
3. Include Key Programming Constructs
While pseudocode doesn’t need to be in any specific programming language, including basic programming constructs can help clarify your logic. Common constructs to use include:
- Loops (for, while)
- Conditional statements (if, else, elif)
- Function definitions
- Variable assignments
4. Focus on the Algorithm, Not Implementation Details
Pseudocode should focus on the high-level logic of your algorithm rather than low-level implementation details. Avoid getting bogged down in language-specific syntax or built-in functions.
Example of algorithm-focused pseudocode for merging two sorted arrays:
function mergeSortedArrays(arr1, arr2):
initialize result as empty array
initialize pointers i and j to 0
while i < length of arr1 and j < length of arr2:
if arr1[i] < arr2[j]:
add arr1[i] to result
increment i
else:
add arr2[j] to result
increment j
add any remaining elements from arr1 to result
add any remaining elements from arr2 to result
return result
5. Use Descriptive Variable Names
Choose variable names that clearly describe their purpose. This makes your pseudocode more self-explanatory and easier to follow.
function calculateAverageGrade(studentGrades):
set totalGrade to 0
set numberOfStudents to length of studentGrades
for each grade in studentGrades:
add grade to totalGrade
set averageGrade to totalGrade / numberOfStudents
return averageGrade
6. Comment on Complex Parts
If a part of your algorithm is particularly complex or not immediately obvious, add a brief comment to explain your reasoning. This shows the interviewer that you’re considering edge cases and potential optimizations.
function findLongestPalindrome(string):
initialize maxLength to 0
initialize start to 0
for i from 0 to length of string - 1:
// Check for odd-length palindromes
length1 = expandAroundCenter(string, i, i)
// Check for even-length palindromes
length2 = expandAroundCenter(string, i, i+1)
// Update maxLength and start if a longer palindrome is found
if max(length1, length2) > maxLength:
maxLength = max(length1, length2)
start = i - (maxLength - 1) / 2
return substring of string from start to start + maxLength
// Helper function to expand around center
function expandAroundCenter(string, left, right):
while left >= 0 and right < length of string and string[left] == string[right]:
decrement left
increment right
return right - left - 1 // Length of palindrome
Common Pitfalls to Avoid When Using Pseudocode in Interviews
While pseudocode can be a powerful tool in technical interviews, there are some common mistakes to avoid:
1. Being Too Vague
While pseudocode doesn’t need to be as detailed as actual code, it should still provide a clear outline of your algorithm. Avoid overly general statements that don’t convey your problem-solving approach.
Bad example:
function sortArray(array):
sort the array
return sorted array
Better example:
function quickSort(array):
if length of array <= 1:
return array
choose pivot as last element of array
initialize left and right as empty arrays
for each element in array except pivot:
if element < pivot:
add element to left
else:
add element to right
return concatenate(quickSort(left), pivot, quickSort(right))
2. Including Too Much Detail
On the flip side, including too much detail can make your pseudocode difficult to read and understand quickly. Remember, the goal is to communicate your high-level approach, not to write actual code.
Too detailed:
function reverseString(str):
initialize empty string result
for i from str.length - 1 to 0 step -1:
result = result + str.charAt(i)
return result
Better balance:
function reverseString(str):
initialize empty result string
for each character from end to start of str:
add character to result
return result
3. Neglecting Edge Cases
While pseudocode doesn’t need to handle every possible scenario, it’s important to show that you’re considering edge cases. This demonstrates your thoroughness and attention to detail.
function findElementInSortedArray(array, target):
if array is empty:
return -1 // Handle empty array case
set left to 0
set right to length of array - 1
while left <= right:
set mid to (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
set left to mid + 1
else:
set right to mid - 1
return -1 // Element not found
4. Forgetting to Explain Your Reasoning
While writing pseudocode, don’t forget to explain your thought process to the interviewer. This can include why you chose a particular approach, any trade-offs you’re considering, or potential optimizations you’re thinking about.
Pseudocode in Different Types of Interview Questions
The way you use pseudocode may vary depending on the type of problem you’re solving. Let’s look at how to apply pseudocode effectively in different common interview scenarios:
1. Array Manipulation Problems
For array problems, your pseudocode should clearly show how you’re iterating through the array and what operations you’re performing. Here’s an example for finding the two numbers in an array that sum to a target value:
function findTwoSum(array, target):
create empty hash map
for each number in array:
complement = target - number
if complement exists in hash map:
return [complement, number]
else:
add number to hash map with its index
return null // No solution found
2. Tree Traversal Problems
When dealing with tree problems, your pseudocode should clearly indicate how you’re traversing the tree (e.g., depth-first, breadth-first) and what operations you’re performing at each node. Here’s an example for finding the maximum depth of a binary tree:
function maxDepth(root):
if root is null:
return 0
leftDepth = maxDepth(root.left)
rightDepth = maxDepth(root.right)
return 1 + max(leftDepth, rightDepth)
3. Dynamic Programming Problems
For dynamic programming problems, your pseudocode should outline your recurrence relation and how you’re building your solution. Here’s an example for the classic fibonacci sequence problem:
function fibonacci(n):
if n <= 1:
return n
initialize dp array of size n+1 with all zeros
dp[0] = 0
dp[1] = 1
for i from 2 to n:
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
4. Graph Problems
For graph problems, your pseudocode should clearly show how you’re representing the graph and how you’re traversing it. Here’s an example for depth-first search (DFS) on a graph:
function DFS(graph, start):
initialize empty stack
initialize empty set for visited nodes
push start onto stack
while stack is not empty:
node = pop from stack
if node not in visited:
add node to visited
process node
for each neighbor of node in graph:
if neighbor not in visited:
push neighbor onto stack
return visited
Transitioning from Pseudocode to Actual Code
Once you’ve written your pseudocode and discussed your approach with the interviewer, you’ll likely need to transition to writing actual code. Here are some tips for making this transition smooth:
1. Use Your Pseudocode as a Guide
Your pseudocode should serve as a roadmap for your actual code. Follow it step by step as you write your implementation.
2. Start with the Main Structure
Begin by translating the high-level structure of your pseudocode (function definitions, main loops, etc.) into code. This gives you a skeleton to fill in with more detailed implementations.
3. Implement One Section at a Time
Don’t try to translate everything at once. Implement one section of your pseudocode at a time, testing and explaining as you go.
4. Keep Talking
Continue to explain your thought process as you translate your pseudocode into actual code. This keeps the interviewer engaged and shows your problem-solving skills in action.
Practice Makes Perfect
Like any skill, using pseudocode effectively in interviews takes practice. Here are some ways to improve your pseudocode skills:
- Solve Problems Regularly: Practice solving algorithmic problems and write pseudocode for each solution before implementing it.
- Review and Refine: After solving a problem, review your pseudocode. Is it clear? Could it be more concise? More detailed?
- Study Good Examples: Look at pseudocode examples in algorithm textbooks or coding websites to understand what makes them effective.
- Mock Interviews: Practice explaining your pseudocode out loud in mock interview settings.
- Teach Others: Explaining algorithms using pseudocode to others can help solidify your understanding and improve your communication skills.
Conclusion
Mastering the art of writing effective pseudocode can significantly enhance your performance in technical interviews. It allows you to demonstrate your problem-solving skills, communicate your thoughts clearly, and approach coding challenges in a structured manner. Remember, the goal of pseudocode in an interview is not just to outline your solution, but to facilitate a productive discussion about your approach to problem-solving.
By following the guidelines and strategies outlined in this guide, you’ll be well-equipped to use pseudocode as a powerful tool in your technical interviews. Whether you’re aiming for a position at a FAANG company or any other tech role, effective use of pseudocode can set you apart as a thoughtful, organized, and skilled problem solver.
Keep practicing, stay curious, and approach each problem as an opportunity to showcase your algorithmic thinking. With time and dedication, you’ll find that pseudocode becomes an invaluable asset in your coding interview toolkit.