How to Use Libraries and Frameworks in Your Code
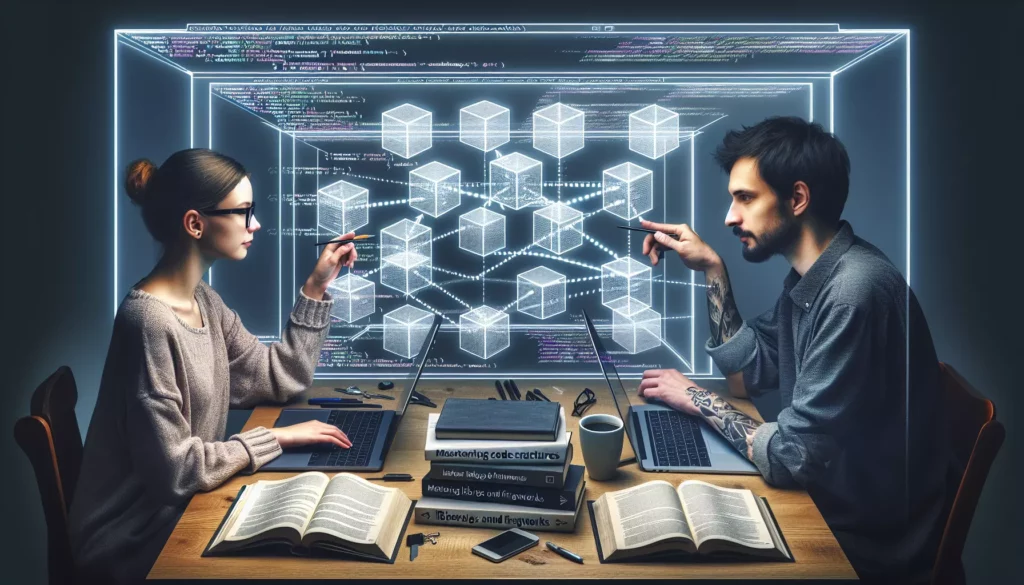
In the world of programming, efficiency and productivity are key. As you progress in your coding journey, you’ll quickly realize that reinventing the wheel for every project is not only time-consuming but also counterproductive. This is where libraries and frameworks come into play. They are essential tools that can significantly streamline your development process, allowing you to focus on solving unique problems rather than implementing common functionalities from scratch. In this comprehensive guide, we’ll explore how to effectively use libraries and frameworks in your code, providing you with the knowledge to supercharge your programming skills.
Understanding Libraries and Frameworks
Before diving into the how-to, it’s crucial to understand what libraries and frameworks are and how they differ.
What is a Library?
A library is a collection of pre-written code that you can use in your projects. Libraries typically focus on providing specific functionality or a set of related functions. For example, jQuery is a popular JavaScript library that simplifies DOM manipulation and event handling.
What is a Framework?
A framework, on the other hand, is a more comprehensive tool that provides a structure for developing software applications. It often includes libraries, APIs, and conventions that guide how you should organize and write your code. Examples include React for building user interfaces and Django for web development in Python.
Key Differences
The main difference between libraries and frameworks lies in the concept of inversion of control:
- With a library, you are in control. You choose when and where to call the library code.
- With a framework, the control is inverted. The framework defines the structure of the application and calls your code when needed.
Benefits of Using Libraries and Frameworks
Incorporating libraries and frameworks into your projects offers numerous advantages:
- Efficiency: Save time by using pre-written, tested code for common functionalities.
- Reliability: Many libraries and frameworks are well-maintained and thoroughly tested by large communities.
- Standardization: Frameworks especially help in maintaining consistent coding practices across projects.
- Community Support: Access a wealth of resources, documentation, and community help.
- Focus on Core Logic: Spend more time on unique aspects of your project rather than reinventing basic functionalities.
How to Choose the Right Library or Framework
Selecting the appropriate library or framework is crucial for the success of your project. Here are some factors to consider:
- Project Requirements: Assess your project’s needs and choose tools that align with these requirements.
- Learning Curve: Consider the time and effort required to learn and implement the library or framework.
- Community and Support: Look for active communities, comprehensive documentation, and regular updates.
- Performance: Evaluate the performance impact, especially for resource-intensive applications.
- Licensing: Ensure the licensing terms are compatible with your project’s needs.
- Integration: Check if the library or framework integrates well with your existing tech stack.
Steps to Incorporate Libraries and Frameworks into Your Code
Now that we understand the basics, let’s dive into the practical steps of using libraries and frameworks in your projects.
1. Installation
The first step is to install the library or framework. This process can vary depending on the programming language and the specific tool you’re using. Here are common methods:
- Package Managers: Many languages have package managers that simplify the installation process. For example:
For JavaScript (using npm):
npm install library-name
For Python (using pip):
pip install library-name
- Manual Download: Some libraries can be downloaded directly from their official websites and included in your project.
- CDN (Content Delivery Network): For web development, you can often include libraries directly from a CDN.
2. Importing or Including the Library
Once installed, you need to import or include the library in your code. The method varies by language:
In JavaScript:
import Library from 'library-name';
In Python:
import library_name
In HTML (for CDN-hosted libraries):
<script src="https://cdn.example.com/library.js"></script>
3. Using the Library’s Functions or Classes
After importing, you can start using the library’s functions or classes in your code. Let’s look at an example using the popular JavaScript library, Lodash:
import _ from 'lodash';
const numbers = [1, 2, 3, 4, 5];
const sum = _.sum(numbers);
console.log(sum); // Output: 15
4. Following Framework Conventions
When using a framework, it’s important to follow its conventions and structure. For instance, if you’re using React, you might create a component like this:
import React from 'react';
function MyComponent() {
return <h1>Hello, World!</h1>;
}
export default MyComponent;
5. Customization and Configuration
Many libraries and frameworks allow for customization. This might involve creating configuration files or passing options when initializing. For example, configuring a Vue.js application:
import Vue from 'vue';
import App from './App.vue';
new Vue({
render: h => h(App),
data: {
// Custom data
},
methods: {
// Custom methods
}
}).$mount('#app');
Best Practices for Using Libraries and Frameworks
To make the most of libraries and frameworks, follow these best practices:
- Keep Dependencies Updated: Regularly update your libraries and frameworks to benefit from bug fixes and new features. However, be cautious of breaking changes in major version updates.
- Read the Documentation: Thoroughly read the official documentation to understand the correct usage and best practices specific to each library or framework.
- Don’t Overuse: While libraries and frameworks are helpful, don’t include them unnecessarily. Each addition increases your project’s complexity and potentially its load time.
- Understand the Core Concepts: Don’t just copy-paste code. Understand how the library or framework works under the hood to use it more effectively.
- Test Thoroughly: Ensure that your use of libraries doesn’t introduce unexpected behaviors or bugs in your application.
- Consider Performance: Be mindful of the performance impact, especially when working on resource-constrained environments or large-scale applications.
Common Pitfalls to Avoid
While libraries and frameworks can greatly enhance your development process, there are some common pitfalls to be aware of:
- Overreliance: Don’t become overly dependent on libraries for simple tasks. It’s important to understand the underlying principles.
- Version Conflicts: Be cautious of version conflicts when using multiple libraries. Ensure compatibility between different dependencies.
- Bloated Applications: Including too many libraries can lead to unnecessarily large applications. Only use what you need.
- Neglecting Security: Always verify the security of third-party code you’re incorporating into your project.
- Ignoring Licensing Terms: Be aware of the licensing requirements of the libraries you use, especially for commercial projects.
Advanced Techniques
As you become more comfortable with using libraries and frameworks, consider these advanced techniques:
1. Creating Your Own Libraries
Once you’ve gained experience, you might find yourself repeating certain code patterns across projects. This is an excellent opportunity to create your own library. Here’s a simple example of creating a utility library in JavaScript:
// myUtils.js
export const capitalize = (str) => str.charAt(0).toUpperCase() + str.slice(1);
export const sum = (arr) => arr.reduce((a, b) => a + b, 0);
// Using your library
import { capitalize, sum } from './myUtils';
console.log(capitalize('hello')); // Output: Hello
console.log(sum([1, 2, 3, 4])); // Output: 10
2. Extending Existing Libraries
Many libraries allow you to extend their functionality. For instance, you can create custom plugins for jQuery:
(function($) {
$.fn.highlight = function(color) {
return this.css('background-color', color);
};
}(jQuery));
// Usage
$('#myElement').highlight('yellow');
3. Integrating Multiple Libraries
Sometimes, you may need to integrate multiple libraries to achieve your desired functionality. For example, you might use Axios for HTTP requests within a React application:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
axios.get('https://api.example.com/users')
.then(response => setUsers(response.data))
.catch(error => console.error('Error fetching users:', error));
}, []);
return (
<ul>
{users.map(user => <li key={user.id}>{user.name}</li>)}
</ul>
);
}
The Future of Libraries and Frameworks
As technology evolves, so do libraries and frameworks. Here are some trends to watch:
- Micro-Frontends: Breaking down front-end applications into smaller, more manageable pieces.
- Serverless Architectures: Frameworks that support serverless development are gaining popularity.
- AI and Machine Learning Integration: More libraries are incorporating AI capabilities, making it easier for developers to implement intelligent features.
- Web Assembly: Libraries that leverage Web Assembly for high-performance web applications are on the rise.
- Cross-Platform Development: Frameworks that allow for simultaneous development across multiple platforms (web, mobile, desktop) are becoming more sophisticated.
Conclusion
Mastering the use of libraries and frameworks is a crucial skill for any developer. They can significantly enhance your productivity, allowing you to build more complex and robust applications in less time. However, it’s important to use them judiciously, understanding their strengths and limitations.
Remember, the key to effectively using libraries and frameworks lies in understanding your project’s needs, staying updated with the latest developments, and continuously learning. As you grow as a developer, you’ll develop an intuition for when to use existing tools and when to craft custom solutions.
By following the guidelines and best practices outlined in this article, you’ll be well-equipped to leverage the power of libraries and frameworks in your coding projects. Whether you’re building a simple website or a complex enterprise application, these tools will be invaluable allies in your development journey.
Keep exploring, keep learning, and most importantly, keep coding!