How to Use Hash Maps to Optimize Your Solutions
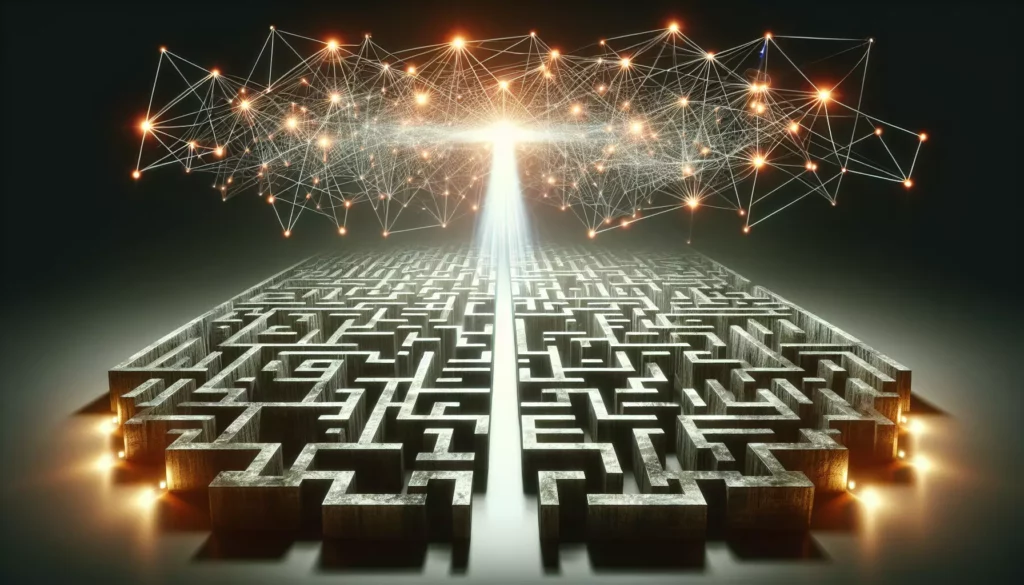
In the world of coding and algorithm design, efficiency is key. As you progress from basic programming concepts to more advanced problem-solving techniques, you’ll encounter various data structures that can significantly improve the performance of your solutions. One such powerful tool is the hash map, also known as a hash table or dictionary in some programming languages. In this comprehensive guide, we’ll explore how to use hash maps to optimize your solutions, making your code faster and more efficient.
What is a Hash Map?
Before diving into the optimization techniques, let’s first understand what a hash map is. A hash map is a data structure that implements an associative array abstract data type, a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
The main advantage of using a hash map is its ability to provide constant-time average complexity for basic operations like insertion, deletion, and lookup. This makes hash maps extremely efficient for tasks that require frequent access or modification of data based on unique keys.
Key Features of Hash Maps:
- Fast lookups: O(1) average time complexity for search, insert, and delete operations
- Key-value pairs: Allows storing and retrieving data based on unique keys
- Dynamic size: Can grow or shrink as needed
- Versatility: Can store various data types as values
When to Use Hash Maps
Hash maps are particularly useful in scenarios where you need to:
- Quickly access or update data based on a unique identifier
- Count occurrences of elements in a collection
- Implement caching mechanisms
- Detect duplicates in a dataset
- Optimize time-consuming lookups in large datasets
Now, let’s explore some common problem-solving scenarios where hash maps can significantly optimize your solutions.
1. Two Sum Problem
The Two Sum problem is a classic coding interview question that asks you to find two numbers in an array that add up to a specific target sum. While a brute-force approach would involve nested loops with O(n^2) time complexity, using a hash map can optimize this to O(n).
Problem Statement:
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Optimized Solution using Hash Map:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
In this solution, we use a hash map to store each number as we iterate through the array, with the number as the key and its index as the value. For each number, we calculate its complement (target – num) and check if it exists in the hash map. If it does, we’ve found our pair and return their indices. If not, we add the current number and its index to the hash map.
This approach reduces the time complexity from O(n^2) to O(n), as we only need to iterate through the array once.
2. First Non-Repeating Character
Another common problem where hash maps shine is finding the first non-repeating character in a string. This problem often appears in coding interviews and real-world scenarios like data compression or string processing.
Problem Statement:
Given a string s
, find the first non-repeating character in it and return its index. If it does not exist, return -1.
Optimized Solution using Hash Map:
def first_uniq_char(s):
char_count = {}
for char in s:
char_count[char] = char_count.get(char, 0) + 1
for i, char in enumerate(s):
if char_count[char] == 1:
return i
return -1
In this solution, we use a hash map to count the occurrences of each character in the string. We then iterate through the string again, checking the count for each character. The first character with a count of 1 is our answer.
This approach has a time complexity of O(n), where n is the length of the string, as we iterate through the string twice. Without a hash map, we might need nested loops, resulting in O(n^2) time complexity.
3. LRU Cache Implementation
An LRU (Least Recently Used) Cache is a data structure that maintains a fixed-size collection of the most recently used items. It’s commonly used in computer science for caching frequently accessed data. Implementing an efficient LRU Cache often involves using a combination of a hash map and a doubly linked list.
Problem Statement:
Design and implement a data structure for Least Recently Used (LRU) cache. It should support the following operations: get and put.
get(key)
– Get the value (will always be positive) of the key if the key exists in the cache, otherwise return -1.put(key, value)
– Set or insert the value if the key is not already present. When the cache reached its capacity, it should invalidate the least recently used item before inserting a new item.
Optimized Solution using Hash Map and Doubly Linked List:
class Node:
def __init__(self, key=0, value=0):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity: int):
self.cache = {}
self.capacity = capacity
self.head = Node()
self.tail = Node()
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key: int) -> int:
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.value
return -1
def put(self, key: int, value: int) -> None:
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self._add(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
lru = self.head.next
self._remove(lru)
del self.cache[lru.key]
def _remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _add(self, node):
node.prev = self.tail.prev
node.next = self.tail
self.tail.prev.next = node
self.tail.prev = node
In this implementation, we use a hash map (self.cache
) to store key-node pairs for quick access, and a doubly linked list to maintain the order of elements based on their recent use. The hash map allows O(1) access to cache items, while the doubly linked list enables O(1) updates to the order of elements.
This design ensures that both get
and put
operations have O(1) time complexity, making it highly efficient for large-scale caching scenarios.
4. Group Anagrams
Grouping anagrams is another classic problem where hash maps can significantly optimize the solution. This problem involves grouping strings that are anagrams of each other.
Problem Statement:
Given an array of strings strs
, group the anagrams together. You can return the answer in any order.
Optimized Solution using Hash Map:
from collections import defaultdict
def group_anagrams(strs):
anagram_groups = defaultdict(list)
for s in strs:
sorted_s = ''.join(sorted(s))
anagram_groups[sorted_s].append(s)
return list(anagram_groups.values())
In this solution, we use a hash map (implemented as a defaultdict
in Python) to group anagrams. The key insight is that anagrams will have the same sorted string representation. We use this sorted string as the key in our hash map, and append each original string to the list of anagrams for that key.
This approach has a time complexity of O(n * k * log(k)), where n is the number of strings and k is the maximum length of a string. The sorting step for each string contributes the log(k) factor. Without a hash map, we might need to compare each string with every other string, resulting in a much higher time complexity.
5. Longest Substring Without Repeating Characters
Finding the longest substring without repeating characters is a problem that can be significantly optimized using a hash map. This problem is often encountered in string processing and data compression scenarios.
Problem Statement:
Given a string s
, find the length of the longest substring without repeating characters.
Optimized Solution using Hash Map:
def length_of_longest_substring(s):
char_index = {}
max_length = 0
start = 0
for i, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
else:
max_length = max(max_length, i - start + 1)
char_index[char] = i
return max_length
In this solution, we use a hash map (char_index
) to store the most recent index of each character. We maintain a sliding window using the start
variable, which represents the starting index of the current substring without repeating characters.
As we iterate through the string, we update the start
index if we encounter a repeating character, and update the max_length
if the current substring is longer than the previous maximum.
This approach has a time complexity of O(n), where n is the length of the string, as we only need to iterate through the string once. Without a hash map, we might need nested loops or more complex logic, potentially leading to higher time complexity.
Best Practices for Using Hash Maps
While hash maps are powerful tools for optimization, it’s important to use them effectively. Here are some best practices to keep in mind:
- Choose appropriate keys: Ensure that your keys are unique and efficiently hashable. Strings and integers are commonly used as keys.
- Handle collisions: Be aware that hash collisions can occur. Most built-in hash map implementations handle this, but if you’re implementing your own, consider using techniques like chaining or open addressing.
- Consider space complexity: While hash maps often improve time complexity, they do use additional space. Ensure that the space trade-off is worthwhile for your specific use case.
- Use language-specific optimizations: Many programming languages have optimized hash map implementations. For example, in Python, consider using
collections.defaultdict
orCounter
for specific use cases. - Be mindful of iteration order: In most implementations, the iteration order of hash maps is not guaranteed. If order matters, consider using an ordered dict or maintaining a separate list for order.
Conclusion
Hash maps are versatile and powerful data structures that can significantly optimize many common algorithmic problems. By providing constant-time average complexity for basic operations, they enable efficient solutions to a wide range of challenges, from the classic Two Sum problem to more complex scenarios like implementing LRU Caches.
As you continue to develop your coding skills and prepare for technical interviews, mastering the use of hash maps will be invaluable. They not only help in solving problems more efficiently but also demonstrate to interviewers your ability to optimize solutions and think critically about data structure choices.
Remember, the key to becoming proficient with hash maps, like any programming concept, is practice. Try implementing the solutions we’ve discussed, and look for opportunities to apply hash maps in your own projects. As you gain experience, you’ll develop an intuition for when and how to leverage hash maps to create more efficient and elegant solutions.
Keep exploring, keep coding, and keep optimizing!