How to Use Git Tags for Versioning Releases: A Comprehensive Guide
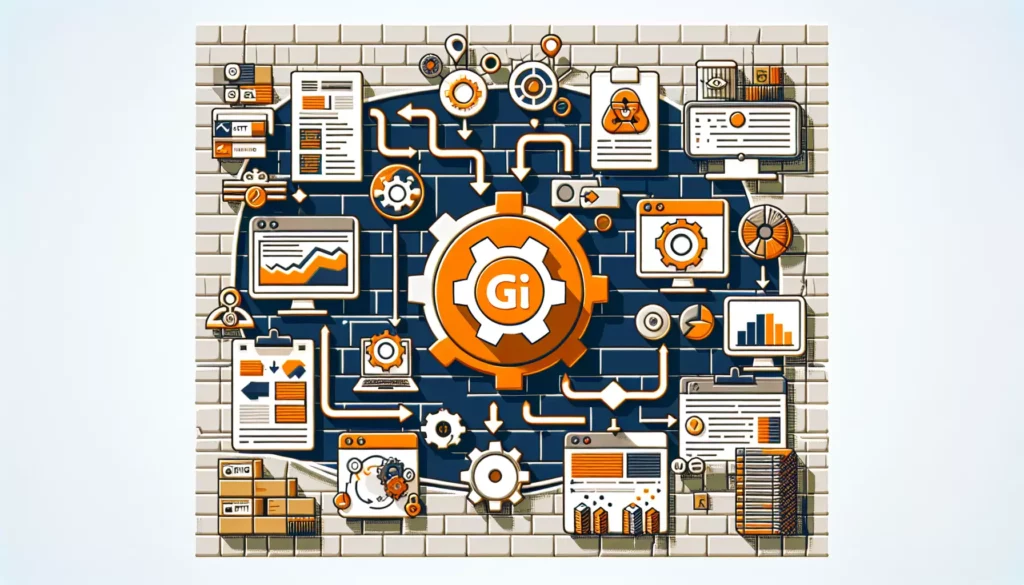
In the world of software development, version control is crucial for managing and tracking changes in your codebase. Git, one of the most popular version control systems, offers a powerful feature called “tags” that can greatly simplify the process of versioning releases. In this comprehensive guide, we’ll explore how to effectively use Git tags for versioning releases, providing you with the knowledge and skills to streamline your development workflow.
Table of Contents
- What Are Git Tags?
- Types of Git Tags
- Creating Git Tags
- Listing and Viewing Tags
- Pushing Tags to Remote Repositories
- Deleting Tags
- Checking Out Tags
- Best Practices for Using Git Tags
- Semantic Versioning with Git Tags
- Automating Tagging in CI/CD Pipelines
- Conclusion
1. What Are Git Tags?
Git tags are references that point to specific points in Git history. They are typically used to mark release points (v1.0, v2.0, etc.) or other significant milestones in a project’s development. Unlike branches, which move as new commits are added, tags are attached to a specific commit and do not change.
Tags serve several important purposes in Git:
- Marking release points
- Creating stable references to specific versions of your code
- Facilitating easy rollback to previous versions
- Improving collaboration by providing clear version references
2. Types of Git Tags
Git supports two types of tags:
Lightweight Tags
Lightweight tags are simply a pointer to a specific commit. They are created with the git tag
command followed by a name. These tags don’t store any additional information and are primarily used for temporary or private tagging.
Annotated Tags
Annotated tags are stored as full objects in the Git database. They contain additional information such as the tagger’s name, email, date, and a tagging message. Annotated tags are recommended for public releases and long-term version tracking.
3. Creating Git Tags
Creating a Lightweight Tag
To create a lightweight tag, use the following command:
git tag v1.0.0
This creates a tag named “v1.0.0” pointing to the current HEAD.
Creating an Annotated Tag
To create an annotated tag, use the -a
flag:
git tag -a v1.0.0 -m "Release version 1.0.0"
This creates an annotated tag with the message “Release version 1.0.0”.
Tagging a Specific Commit
You can also tag a specific commit by providing its hash:
git tag -a v1.0.0 9fceb02 -m "Release version 1.0.0"
This creates an annotated tag for the commit with the hash “9fceb02”.
4. Listing and Viewing Tags
Listing Tags
To list all tags in your repository, use:
git tag
For a more detailed list including the annotated tag messages, use:
git tag -n
Viewing Tag Details
To view detailed information about a specific tag, use:
git show v1.0.0
This command displays the tagger information, date, and commit message associated with the tag.
5. Pushing Tags to Remote Repositories
By default, the git push
command doesn’t transfer tags to remote repositories. To push tags, you have two options:
Pushing a Specific Tag
git push origin v1.0.0
This pushes the tag “v1.0.0” to the remote repository named “origin”.
Pushing All Tags
git push origin --tags
This command pushes all tags to the remote repository.
6. Deleting Tags
Deleting a Local Tag
To delete a tag from your local repository:
git tag -d v1.0.0
Deleting a Remote Tag
To delete a tag from a remote repository:
git push origin --delete v1.0.0
7. Checking Out Tags
To check out the code at a specific tag, you can use:
git checkout v1.0.0
This will put your repository in a “detached HEAD” state. If you want to make changes, it’s best to create a new branch:
git checkout -b new-branch v1.0.0
8. Best Practices for Using Git Tags
- Use Annotated Tags for Releases: Annotated tags provide more information and are better suited for marking significant points like releases.
- Follow a Consistent Naming Convention: Adopt a clear and consistent naming scheme for your tags, such as “v1.0.0” for major releases.
- Tag After Thorough Testing: Only create release tags after your code has been thoroughly tested and is ready for deployment.
- Include Relevant Information in Tag Messages: For annotated tags, include useful information in the tag message, such as key features or changes in the release.
- Use Tags in Conjunction with Branches: Create tags on stable branches (like master or main) to mark releases, while using feature branches for ongoing development.
- Automate Tagging in CI/CD Pipelines: Implement automated tagging in your continuous integration and deployment processes to ensure consistency.
9. Semantic Versioning with Git Tags
Semantic Versioning (SemVer) is a versioning scheme that provides meaning to version numbers. It follows the format MAJOR.MINOR.PATCH:
- MAJOR version for incompatible API changes
- MINOR version for backwards-compatible functionality additions
- PATCH version for backwards-compatible bug fixes
Using SemVer with Git tags can greatly improve the clarity of your release history. Here’s an example of how to create a tag using SemVer:
git tag -a v1.2.3 -m "Release version 1.2.3 - Added feature X and fixed bug Y"
10. Automating Tagging in CI/CD Pipelines
Automating the tagging process in your Continuous Integration/Continuous Deployment (CI/CD) pipeline can ensure consistency and reduce human error. Here’s a basic example of how you might automate tagging in a CI/CD pipeline using a bash script:
#!/bin/bash
# Get the latest version tag
latest_tag=$(git describe --tags --abbrev=0)
# Increment the version
IFS='.' read -ra ADDR <<< "$latest_tag"
major=${ADDR[0]}
minor=${ADDR[1]}
patch=${ADDR[2]}
# Increment patch version
new_patch=$((patch + 1))
# Create new tag
new_tag="v$major.$minor.$new_patch"
# Create and push the new tag
git tag -a $new_tag -m "Automated release $new_tag"
git push origin $new_tag
This script gets the latest tag, increments the patch version, creates a new tag, and pushes it to the remote repository. You can integrate this script into your CI/CD pipeline to automatically create tags for each build or release.
11. Conclusion
Git tags are a powerful feature for versioning releases and marking significant points in your project’s history. By following the best practices outlined in this guide and leveraging tools like Semantic Versioning and automated tagging in CI/CD pipelines, you can create a robust and efficient versioning system for your software projects.
Remember, effective use of Git tags not only helps in managing releases but also improves collaboration within your team and provides clear references for users of your software. As you continue to develop your coding skills and work on larger projects, mastering Git tags will become an invaluable asset in your software development toolkit.
Whether you’re a beginner just starting out with version control or an experienced developer looking to refine your Git workflow, incorporating tags into your development process can significantly enhance your project management and version tracking capabilities. So start tagging your releases today, and enjoy the benefits of clearer, more organized version control in your software development journey!