How to Use Git Stash for Temporary Code Changes
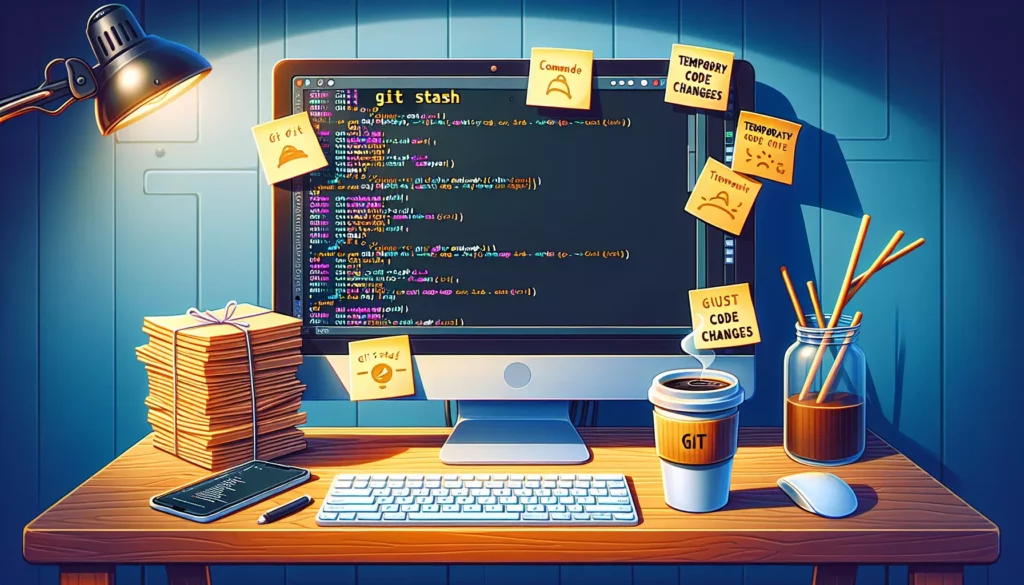
In the world of software development, version control is an essential skill. Git, one of the most popular version control systems, offers a powerful feature called “git stash” that allows developers to temporarily save changes without committing them. This feature is particularly useful when you need to switch tasks quickly or when you want to experiment with code without affecting your main work. In this comprehensive guide, we’ll explore how to use git stash effectively, its various commands, and best practices for managing your temporary code changes.
What is Git Stash?
Git stash is a command that takes your modified tracked files and saves them on a stack of unfinished changes that you can reapply at any time. It’s like putting your work-in-progress in a drawer for safekeeping while you work on something else.
The primary use cases for git stash include:
- Switching branches without committing half-done work
- Applying a stash to multiple branches
- Quickly hiding changes when pulling updates from a remote repository
- Experimenting with different solutions without affecting your current work
Basic Git Stash Commands
1. Stashing Your Changes
To stash your current changes, simply run:
git stash
This command will save your modified tracked files and staged changes, and then revert them to match the HEAD commit. Your working directory will be clean, allowing you to switch branches or perform other operations.
2. Listing Stashes
To see a list of your stashes, use:
git stash list
This command will show you all the stashes in your stack, with the most recent stash at the top.
3. Applying a Stash
To apply the most recent stash and remove it from the stack:
git stash pop
If you want to apply a specific stash, you can use:
git stash apply stash@{n}
Where ‘n’ is the index of the stash you want to apply.
4. Removing a Stash
To remove the most recent stash without applying it:
git stash drop
To remove a specific stash:
git stash drop stash@{n}
Advanced Git Stash Techniques
1. Stashing Untracked Files
By default, git stash only stores tracked files. To include untracked files in your stash, use:
git stash -u
This command will stash both tracked and untracked files.
2. Creating a Branch from a Stash
If you want to create a new branch and apply your stashed changes to it, use:
git stash branch <new-branch-name> stash@{n}
This command creates a new branch, checks it out, and then applies the stash, dropping it if the application was successful.
3. Partial Stashing
Sometimes you may want to stash only part of your changes. You can do this interactively using:
git stash -p
This command will prompt you to choose which changes you want to stash and which to keep in your working directory.
4. Viewing Stash Contents
To see the contents of a stash without applying it, use:
git stash show -p stash@{n}
This command shows the diff of the stash against the commit it was based on.
Best Practices for Using Git Stash
1. Use Descriptive Stash Messages
When creating a stash, it’s helpful to add a descriptive message:
git stash save "Your descriptive message here"
This makes it easier to identify specific stashes later, especially if you have multiple stashes.
2. Clean Up Your Stash Stack
Regularly review and clean up your stash stack to avoid confusion and clutter. Remove stashes that are no longer needed using git stash drop
.
3. Be Cautious When Applying Stashes
When applying a stash, be aware that it may cause conflicts with your current work. Always make sure your working directory is clean before applying a stash, or consider using git stash apply --index
to try to reinstate the staging area’s state as well.
4. Use Stashes for Short-Term Storage
While git stash is a powerful tool, it’s best used for short-term storage of changes. For longer-term work or more complex changes, consider using feature branches instead.
Common Scenarios and Solutions
Scenario 1: Switching Branches with Uncommitted Changes
Imagine you’re working on a feature branch and need to quickly switch to the main branch to fix a bug. Here’s how you can use git stash:
git stash save "WIP: Feature XYZ"
git checkout main
# Fix the bug and commit the changes
git checkout feature-branch
git stash pop
Scenario 2: Experimenting with Code
If you want to try out a different approach without affecting your current work:
git stash
# Experiment with your code
# If you like the changes:
git stash drop
# If you want to revert to your original code:
git stash pop
Scenario 3: Applying a Stash to Multiple Branches
Sometimes you might want to apply the same changes to multiple branches:
git stash
git checkout branch1
git stash apply
git checkout branch2
git stash apply
git stash drop # If you're done with the stash
Troubleshooting Common Issues
1. Merge Conflicts When Applying a Stash
If you encounter merge conflicts when applying a stash, Git will pause the operation. You’ll need to resolve the conflicts manually, then stage the resolved files and run git stash drop
if you used git stash pop
.
2. Lost Stashes
If you accidentally drop a stash, you might be able to recover it using Git’s reflog:
git fsck --no-reflog | awk '/dangling commit/ {print $3}'
This command will show you a list of dangling commits, which may include your lost stash. You can then use git show
to examine these commits and potentially recover your work.
3. Stash Apply Not Working as Expected
If git stash apply
isn’t applying your changes as expected, it might be due to changes in your working directory since you created the stash. Try using git stash apply --index
to attempt to restore both the working tree and the index.
Git Stash in Team Collaboration
While git stash is primarily a local operation, it can be useful in team collaboration scenarios:
1. Code Reviews
When reviewing a colleague’s pull request, you can use git stash to temporarily store your current work, check out their branch, and then return to your work:
git stash
git checkout colleagues-branch
# Review the code
git checkout your-branch
git stash pop
2. Pair Programming
During pair programming sessions, you can use git stash to quickly switch between different ideas or approaches:
git stash save "Approach A"
# Try a different approach
git stash save "Approach B"
# Discuss and decide which approach to use
git stash pop stash@{1} # Apply the chosen approach
3. Handling Interruptions
In a team environment, interruptions are common. Git stash allows you to quickly save your work and switch contexts:
git stash save "Feature X progress"
# Handle the interruption
git stash pop # Resume work on Feature X
Git Stash and Continuous Integration
Git stash can be particularly useful in continuous integration (CI) workflows:
1. Pre-Commit Hooks
You can use git stash in pre-commit hooks to temporarily store changes, run tests or linters, and then reapply the changes:
#!/bin/sh
git stash -q --keep-index
npm test
RESULT=$?
git stash pop -q
[ $RESULT -ne 0 ] && exit 1
exit 0
2. CI Pipeline Debugging
When debugging CI pipeline issues, you can use git stash to quickly switch between the failing commit and your current work:
git stash
git checkout <failing-commit>
# Debug the issue
git checkout -
git stash pop
Git Stash Alternatives
While git stash is a powerful tool, there are alternatives that might be more suitable in certain situations:
1. Feature Branches
For longer-term work or more significant changes, creating a feature branch is often a better choice than using git stash:
git checkout -b feature-branch
# Work on your feature
git commit -m "Feature implementation"
git checkout main
git merge feature-branch
2. Git Worktree
Git worktree allows you to check out multiple branches simultaneously in separate directories:
git worktree add ../feature-branch feature-branch
cd ../feature-branch
# Work on your feature
cd -
git worktree remove ../feature-branch
3. Commit and Amend
For quick changes, you can commit your work and then amend the commit later:
git commit -m "WIP: Feature implementation"
# Make more changes
git commit --amend
Conclusion
Git stash is a powerful and flexible tool that can significantly improve your workflow when dealing with temporary code changes. By mastering git stash, you can easily switch between tasks, experiment with code, and manage your work-in-progress more effectively. Remember to use descriptive stash messages, clean up your stash stack regularly, and consider alternatives like feature branches for longer-term work.
As you continue to develop your programming skills, tools like git stash become invaluable in managing complex projects and collaborating with team members. Practice using git stash in your daily workflow, and you’ll soon find it an indispensable part of your development toolkit.
Whether you’re preparing for technical interviews, working on personal projects, or contributing to open-source software, a solid understanding of git stash and other version control techniques will serve you well in your journey as a software developer.