How to Use Git Rebase for a Cleaner Project History
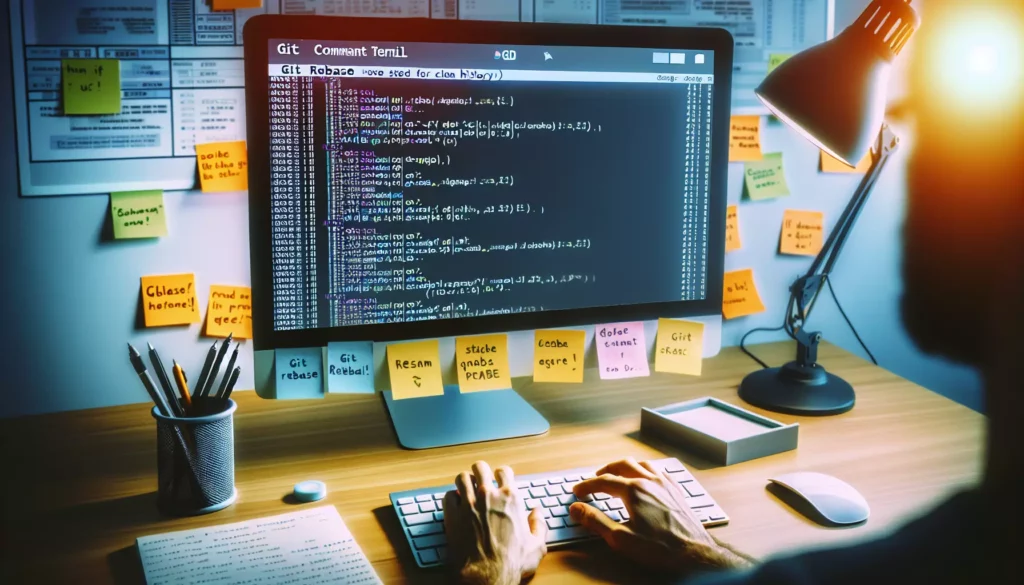
In the world of software development, version control is an essential tool for managing code changes and collaborating with team members. Git, being one of the most popular version control systems, offers a powerful feature called “rebase” that can help developers maintain a cleaner and more organized project history. In this comprehensive guide, we’ll explore how to use Git rebase effectively, its benefits, and potential pitfalls to watch out for.
What is Git Rebase?
Before diving into the specifics of using Git rebase, it’s important to understand what it is and how it differs from other Git operations like merging.
Git rebase is a command that allows you to move or combine a sequence of commits to a new base commit. In simpler terms, it enables you to change the starting point of your branch to a different commit. This can be particularly useful when you want to incorporate changes from one branch into another while maintaining a linear project history.
The main difference between rebasing and merging is that rebasing rewrites the commit history, while merging preserves it. When you merge branches, Git creates a new commit that combines the changes from both branches. On the other hand, rebasing moves the entire feature branch to begin on the tip of the main branch, effectively incorporating all of the new commits.
Why Use Git Rebase?
There are several reasons why developers choose to use Git rebase:
- Cleaner Project History: Rebasing can result in a more linear and easier-to-read project history, as it avoids the creation of unnecessary merge commits.
- Easier Code Reviews: A cleaner history makes it easier for team members to review code changes and understand the evolution of the project.
- Resolving Conflicts Earlier: Rebasing allows you to resolve conflicts on a commit-by-commit basis, which can be easier than resolving all conflicts at once during a merge.
- Keeping Feature Branches Up-to-Date: Regularly rebasing feature branches onto the main branch ensures that your feature branch incorporates the latest changes from the main branch, reducing the likelihood of conflicts when it’s time to merge.
How to Use Git Rebase
Now that we understand the benefits of Git rebase, let’s explore how to use it effectively. We’ll cover the basic rebase operation, interactive rebasing, and some advanced techniques.
Basic Rebase Operation
The simplest form of rebasing involves moving a feature branch to a new base. Here’s how you can do it:
- Ensure you’re on the branch you want to rebase:
git checkout feature-branch
- Rebase onto the desired base branch (usually the main branch):
git rebase main
This command will take all the commits from your feature branch and replay them on top of the latest commit from the main branch.
Interactive Rebasing
Interactive rebasing gives you more control over the rebasing process, allowing you to modify commits as they are moved to the new base. To start an interactive rebase, use the `-i` flag:
git rebase -i main
This will open your default text editor with a list of commits that will be rebased. You can then choose to pick, edit, squash, or drop commits as needed. Here are some common operations you can perform during an interactive rebase:
- pick: Use the commit as-is
- reword: Use the commit, but edit the commit message
- edit: Use the commit, but stop for amending
- squash: Use the commit, but meld it into the previous commit
- fixup: Like squash, but discard the commit’s log message
- drop: Remove the commit
Advanced Rebasing Techniques
1. Squashing Commits
Squashing commits is a common use case for interactive rebasing. It allows you to combine multiple commits into a single, more meaningful commit. Here’s how to do it:
- Start an interactive rebase:
git rebase -i HEAD~3
(This will show the last 3 commits)
- In the text editor, change “pick” to “squash” for the commits you want to combine:
pick abc1234 First commit message squash def5678 Second commit message squash ghi9101 Third commit message
- Save and close the file. Git will then prompt you to edit the combined commit message.
- Edit the commit message as desired, then save and close the file.
2. Splitting Commits
Sometimes you may want to split a large commit into smaller, more focused commits. Here’s how to do it:
- Start an interactive rebase, going back to the commit you want to split:
git rebase -i <commit-hash>^
- Change “pick” to “edit” for the commit you want to split:
edit abc1234 Large commit to split
- Save and close the file. The rebase will stop at the commit you marked for editing.
- Reset the last commit, keeping the changes unstaged:
git reset HEAD^
- Stage and commit the changes in smaller, logical chunks:
git add <files> git commit -m "First part of split commit" git add <more-files> git commit -m "Second part of split commit"
- Once you’ve created all the desired commits, continue the rebase:
git rebase --continue
Best Practices for Using Git Rebase
While Git rebase is a powerful tool, it’s important to use it responsibly. Here are some best practices to keep in mind:
1. Don’t Rebase Shared Branches
The golden rule of Git rebase is: never rebase branches that others are working on. Rebasing rewrites history, which can cause problems for other developers who have based their work on the original branch. Stick to rebasing your local branches or feature branches that only you are working on.
2. Communicate with Your Team
If you’re working in a team, make sure everyone is on the same page regarding the use of rebase. Some teams prefer a merge-only workflow, while others might be comfortable with rebasing feature branches before merging.
3. Use Feature Flags for Long-Running Features
For long-running feature branches, consider using feature flags instead of relying solely on branching. This allows you to merge code more frequently and reduces the need for complex rebases.
4. Regularly Rebase Feature Branches
If you’re working on a feature branch, regularly rebasing onto the main branch can help you stay up-to-date with the latest changes and reduce the likelihood of conflicts when it’s time to merge.
5. Write Good Commit Messages
Clear and descriptive commit messages become even more important when rebasing, as they help you and your team understand the purpose of each commit during the rebase process.
Potential Pitfalls and How to Avoid Them
While Git rebase is a powerful tool, it’s not without its challenges. Here are some common pitfalls and how to avoid them:
1. Losing Commits
If you’re not careful, it’s possible to lose commits during a rebase. To avoid this:
- Always create a backup branch before rebasing:
git branch backup-branch
- Use
git reflog
to recover lost commits if necessary
2. Merge Conflicts
Rebasing can sometimes lead to merge conflicts, especially if there have been significant changes to the base branch. To handle this:
- Resolve conflicts on a commit-by-commit basis
- Use
git rebase --abort
if you need to start over - Consider using a visual merge tool to help resolve complex conflicts
3. Force Pushing
After rebasing, you may need to force push your changes to the remote repository. This can be dangerous if not done carefully:
- Only force push to branches that you own and that aren’t shared
- Use the
--force-with-lease
option instead of--force
for an extra layer of safety
Alternatives to Rebasing
While rebasing can be useful in many situations, it’s not always the best choice. Here are some alternatives to consider:
1. Merging
Traditional merging is still a valid and often preferred method for integrating changes. It preserves the full history of your project and is generally safer for shared branches.
2. Cherry-Picking
If you only want to apply specific commits from one branch to another, cherry-picking might be a better option than rebasing. Use git cherry-pick <commit-hash>
to apply individual commits.
3. Creating a New Branch
Sometimes, the simplest solution is to create a new branch with the desired starting point and re-apply your changes manually.
Git Rebase in Popular IDEs and Tools
Many popular Integrated Development Environments (IDEs) and Git clients provide graphical interfaces for performing Git operations, including rebasing. Here’s how you can use Git rebase in some common tools:
Visual Studio Code
VS Code has built-in Git support and can be extended with the “Git Graph” extension for a more visual representation of your Git history.
- Open the Source Control view (Ctrl+Shift+G)
- Click on the three dots (…) to open the menu
- Select “Rebase” and choose the branch to rebase onto
JetBrains IDEs (IntelliJ IDEA, PyCharm, WebStorm, etc.)
JetBrains IDEs offer comprehensive Git integration:
- Go to Git -> Rebase
- Select the branch to rebase onto
- Choose between “Rebase” and “Interactive Rebase”
GitKraken
GitKraken is a popular Git client with a user-friendly interface:
- Right-click on the branch you want to rebase
- Select “Rebase [branch] onto…”
- Choose the target branch
Git Rebase and Continuous Integration/Continuous Deployment (CI/CD)
Using Git rebase effectively can have a positive impact on your CI/CD pipeline:
1. Cleaner Build History
A linear history created by rebasing can make it easier to track which commits triggered specific builds or deployments.
2. Easier Rollbacks
With a clean, linear history, rolling back to a previous state in case of issues becomes more straightforward.
3. Improved Code Review Process
Rebased branches with squashed commits can make the code review process more efficient, as reviewers can focus on the overall changes rather than incremental updates.
Conclusion
Git rebase is a powerful tool that, when used correctly, can help maintain a clean and organized project history. By understanding how to perform basic and interactive rebases, as well as following best practices and avoiding common pitfalls, you can leverage this feature to improve your Git workflow.
Remember that while rebasing has its advantages, it’s not always the best solution. Consider your team’s workflow, the nature of your project, and the potential risks before deciding to use rebase. When in doubt, communicate with your team and choose the approach that best suits your project’s needs.
As you continue to develop your Git skills, experimenting with rebasing in a safe environment (like a personal project) can help you become more comfortable with the process. With practice, you’ll be able to confidently use Git rebase to maintain a clean and efficient project history, ultimately contributing to better collaboration and code management in your development projects.